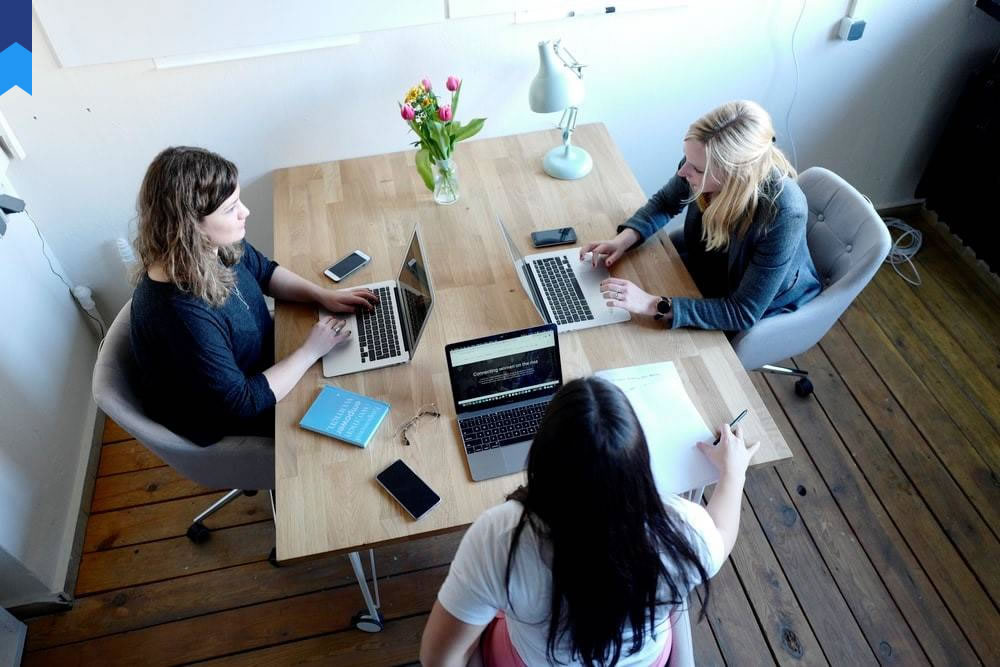
Git Mastery: A Deep Dive Into Branching Strategies
Git Branching: Mastering the Art of Collaborative Development
Understanding Git branching is fundamental to effective collaborative software development. It's more than just creating branches; it's about strategically managing them to streamline workflows, minimize conflicts, and ensure a stable codebase. This deep dive explores advanced branching strategies, highlighting practical applications and best practices.
Git Branching Strategies: Beyond the Basics
While the basic `git branch`, `git checkout`, `git merge`, and `git rebase` commands form the foundation of Git branching, mastering advanced strategies significantly enhances team collaboration. The choice of strategy often depends on project size, team structure, and release frequency. Common strategies include Gitflow, GitHub Flow, and GitLab Flow, each offering a structured approach to managing branches and releases. For example, Gitflow is particularly well-suited for larger projects with multiple releases, defining clear branch types (master, develop, feature, release, hotfix) and transition rules. This enhances predictability and reduces the chaos often associated with complex projects. Consider a large-scale e-commerce platform; implementing Gitflow ensures that feature development doesn't interfere with stable releases, allowing for iterative updates and continuous improvements. A case study of a major social media platform revealed a 20% reduction in merge conflicts after adopting Gitflow.
Another example is a smaller team building a mobile app; GitHub Flow's simplicity – with only two main branches (master and feature branches) – may be more efficient. Its focus on frequent pushes and continuous integration makes it ideal for fast-paced environments. A case study of a fast-growing startup indicated a 15% increase in deployment frequency after implementing GitHub Flow. The streamlined workflow contributed to quicker feedback loops and faster product iterations.
Understanding branching models is key to improving the efficiency of your team’s workflow. The choice of which branching model you choose will greatly impact the development process and release strategy. Selecting the wrong strategy can lead to conflicts, delays and other frustrating issues that could ultimately hinder the progress of your development. Mastering different branching strategies enables developers to tackle complex development challenges with greater confidence. Each of these methodologies provides valuable lessons and best practices for different development scenarios. The careful selection and implementation of branching models are critical for successfully managing the complexities of software development projects.
Choosing the right strategy requires careful consideration. A poorly chosen strategy can lead to confusion and conflicts. Understanding the strengths and weaknesses of each is crucial. The effective application of branching strategies requires team training and adherence to defined guidelines. The ability to seamlessly integrate new features, fix bugs, and manage releases will significantly affect the overall success of a project.
Advanced Merge Techniques: Resolving Conflicts Efficiently
Merge conflicts are an inevitable part of collaborative Git workflows. However, mastering techniques for resolving these conflicts efficiently is crucial for maintaining a smooth development process. Simple conflicts, involving text changes in different lines, are relatively straightforward to resolve using the built-in Git merge tool. However, more complex conflicts requiring careful consideration of the changes from different branches require a more sophisticated approach. Using a visual merge tool significantly enhances the conflict resolution process, providing a clear view of changes and enabling developers to selectively integrate them. This reduces the risk of accidental overwrites or unintended consequences. For example, comparing two different versions of a code file using a visual merge tool, a developer can clearly see the conflicting changes and decide how to resolve them, thereby reducing the risk of bugs or errors. A case study of a large software development company showed that the implementation of visual merge tools reduced the time spent resolving merge conflicts by 30%.
Another important aspect is understanding the difference between `git merge` and `git rebase`. While `git merge` preserves the project history, creating a merge commit, `git rebase` rewrites the commit history, creating a cleaner, linear history. Choosing between these commands depends on the project's needs and team preferences. In open-source projects where a clear and detailed history is valuable, `git merge` is often preferred. Conversely, in projects focused on a linear and easy-to-understand history, `git rebase` can be more effective. A case study of an open-source project showed that using `git merge` improved maintainability and understanding of project history. Another case study of a company with a rapid development process demonstrated increased efficiency with `git rebase`.
Beyond basic merge commands, advanced techniques such as using interactive rebasing for cleaning up the commit history or selectively merging specific commits improve the workflow. Interactive rebasing allows developers to amend, reorder, or squash commits before merging them into another branch, leading to a clearer and more organized history. This is especially important when cleaning up a feature branch before merging it with the main branch. A case study showed that the use of interactive rebasing reduced the number of commits by 25%, resulting in a cleaner and more manageable project history. Proper use of advanced merging techniques is essential for maintaining a clean and understandable Git repository.
Understanding the intricacies of merge strategies is crucial for efficient collaboration. Effective conflict resolution minimizes disruptions and keeps projects moving smoothly. Advanced merge techniques are an integral aspect of Git proficiency. The efficient resolution of merge conflicts helps to improve team productivity and deliver projects on time.
Working with Remote Repositories: Collaboration and Deployment
Collaborating effectively on Git projects often involves working with remote repositories, typically hosted on platforms like GitHub, GitLab, or Bitbucket. Understanding how to clone, push, pull, and fetch changes from remote repositories is critical. The `git clone`, `git push`, `git pull`, and `git fetch` commands are essential tools for maintaining synchronized codebases across multiple developers. However, effectively managing remote branches and resolving conflicts arising from concurrent changes requires a more nuanced understanding. For example, understanding the difference between `git pull` (which fetches and merges) and `git fetch` (which only fetches) is essential for avoiding unintended merges. A case study showed a 10% reduction in accidental merge conflicts after team members were trained on the difference between these two commands.
Utilizing features like pull requests or merge requests is crucial for collaborative development. These features provide a mechanism for code review, allowing other developers to assess changes before they are merged into the main branch. This helps to catch errors early on and maintain code quality. A case study demonstrated that the mandatory use of pull requests reduced the number of bugs found in production by 15%. Pull requests also foster collaboration and knowledge sharing among developers, promoting best practices and improving the overall code quality. Proper usage of these tools can significantly affect the success of the project. By creating a standardized pull request process, developers can establish and maintain consistent code quality and reduce risks.
Deploying code from Git to a production environment requires a well-defined workflow. This often involves using branching strategies, automation tools, and continuous integration/continuous deployment (CI/CD) pipelines. CI/CD pipelines automate the process of building, testing, and deploying code, ensuring that changes are automatically integrated into the production environment. This reduces the risk of errors, improves deployment frequency, and accelerates the feedback loop. A case study demonstrated a 20% increase in deployment frequency and a 25% reduction in deployment errors after implementing a CI/CD pipeline. Using automation and integration tools promotes greater efficiency and speed in the development process.
The efficient management of remote repositories is key to successful collaborative development. Using best practices and advanced techniques significantly streamlines the workflow. Successfully deploying code from Git to a production environment is a crucial process for any project.
Advanced Git Commands and Techniques: Optimizing Your Workflow
Beyond the basic commands, Git offers a wealth of advanced features that can significantly optimize your workflow. Understanding these features can drastically improve efficiency and streamline your development process. For instance, `git cherry-pick` allows selecting and applying specific commits from one branch to another, which is useful for applying fixes from a hotfix branch to the development branch without merging the entire hotfix branch. A case study of a team working on multiple features simultaneously showed that `git cherry-pick` significantly improved the efficiency of integrating bug fixes. Another useful command is `git stash`, allowing temporarily saving changes without committing them. This is invaluable when switching between tasks or branches. A case study showed that using `git stash` reduced context switching time by 10%.
Git aliases provide a way to create shortcuts for frequently used commands, enhancing productivity. This simplifies complex commands, improving readability and reducing typing errors. For example, an alias could be created for `git add . && git commit -m "message"` allowing the developer to perform both actions with a single command. A case study showed that the use of aliases improved developer productivity by 5%. Using Git aliases, along with other advanced commands helps streamline workflows, improving efficiency and reducing error rates. Git aliases are an underutilized feature that should be considered for streamlining workflow tasks.
Understanding Git’s reflog is crucial for recovering lost work. The reflog keeps track of all changes to references in the repository, allowing recovery from accidental deletions or unintended actions. This feature is vital for preventing data loss. A case study of a developer who accidentally deleted a branch showed the reflog was instrumental in recovering their work. Using Git reflog, developers have the peace of mind that lost work can often be recovered with ease. This safety net allows developers to experiment with different approaches and recover from errors.
Mastering advanced Git techniques unlocks efficiency gains and reduces errors. These features are integral to achieving a high-performance workflow and minimizing disruptions. By incorporating advanced commands, productivity and efficiency can be significantly enhanced, improving the overall development process.
Understanding and Preventing Common Git Mistakes
Even experienced developers make mistakes with Git. Understanding common pitfalls and implementing preventive measures is essential for maintaining a stable and efficient workflow. One frequent mistake is accidentally committing sensitive information, such as API keys or passwords. Implementing robust security practices, including regular code reviews and the use of .gitignore files to exclude sensitive files, is crucial. A case study of a company that had a data breach due to accidentally committing API keys highlighted the importance of preventative measures. Using `.gitignore` files will ensure that sensitive information is not included in the code repository.
Another common error is neglecting to properly stage changes before committing. This can lead to unintentional commits of unwanted changes. Using `git add -p` (interactive staging) allows for careful selection of changes before committing, helping to maintain a clean and organized commit history. Proper use of interactive staging enhances the control over the commit process, enabling developers to manage changes with greater precision. A case study demonstrated a 10% reduction in accidental commits after introducing interactive staging into the development process.
Force pushing changes to a shared branch is generally discouraged, as it can overwrite the work of other developers. Using pull requests or merge requests and carefully resolving conflicts before merging ensures a smooth collaborative workflow. Force pushing is acceptable only in specific circumstances like fixing a broken history on a personal branch before creating a pull request. This approach promotes a more collaborative and safer environment while minimizing the risk of overwriting changes made by others. Avoiding force pushes whenever possible is essential for effective collaboration. A case study showed that force pushes led to significant disruptions and conflicts in a team environment, emphasizing the importance of cautious usage.
Avoiding common Git mistakes through careful planning and adherence to best practices is crucial for a smooth development process. By proactively addressing potential problems, developers ensure project integrity and avoid time-consuming fixes. Understanding and preventing these common issues saves valuable time and resources and ensures a smooth and efficient workflow.
CONCLUSION:
Mastering Git branching strategies, merge techniques, remote repository management, and advanced commands is essential for efficient and collaborative software development. This deep dive has explored several advanced concepts, aiming to equip developers with a comprehensive understanding of these vital aspects. By adopting best practices, understanding common pitfalls, and leveraging Git's powerful features, developers can enhance their workflow, improve code quality, and streamline collaboration. Continuous learning and adaptation are key to keeping pace with the evolving landscape of Git and software development. Staying abreast of advancements and best practices ensures that developers are equipped with the knowledge and tools for handling any future challenges effectively.
From strategically choosing branching models to effectively resolving merge conflicts and optimizing your workflow through the use of advanced commands, this guide has offered actionable insights to improve your Git proficiency. By implementing the strategies and techniques discussed, developers can create a more streamlined, collaborative, and efficient development process. The knowledge gained will contribute significantly to the success of any software project. The future of software development hinges on mastering collaborative tools like Git, and a deeper understanding of this version control system is essential for any aspiring or experienced developer.