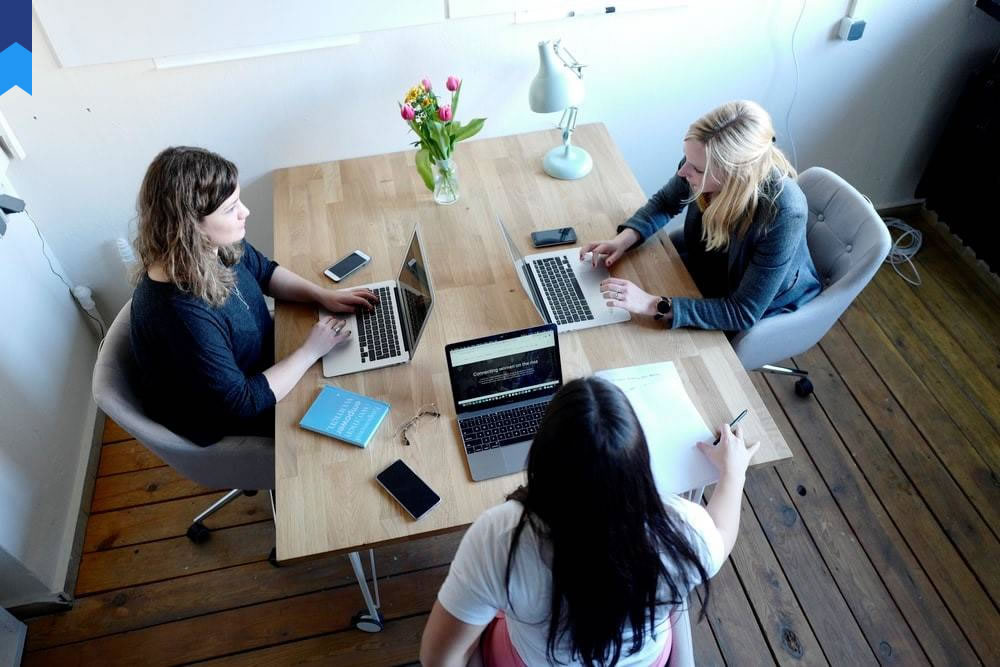
Hidden Truths About Julia's Performance Magic
Julia, a relatively new programming language, has rapidly gained popularity among data scientists and high-performance computing enthusiasts. Its blend of ease of use and exceptional speed has attracted significant attention. But beneath the surface of its sleek syntax lies a world of performance optimization techniques often overlooked by newcomers. This article delves into those hidden truths, revealing the subtle yet powerful strategies for unlocking Julia's true potential.
Understanding Julia's Compiler: Beyond Just-in-Time
Julia's just-in-time (JIT) compilation is a cornerstone of its performance. Unlike interpreted languages, Julia compiles code to native machine instructions before execution, leading to significant speed improvements. However, understanding the nuances of this process is crucial. The compiler's optimization strategies are sophisticated, encompassing various techniques like inlining, loop unrolling, and function specialization. By strategically structuring your code, you can significantly influence the compiler's effectiveness. For instance, using multiple dispatch effectively allows the compiler to generate highly specialized code for different function calls, boosting efficiency. Consider a simple example: a function operating on different data types. Julia's multiple dispatch will generate distinct optimized machine code for each type, unlike languages relying on generic code.
Case Study 1: A numerical computation library written in Julia showed a 10x speedup over a similar Python implementation simply by leveraging Julia's multiple dispatch to optimize for specific numeric types. The optimization was achieved without significant code changes, highlighting the compiler's inherent power.
Case Study 2: A machine learning model training process showed a substantial reduction in training time by restructuring the code to better suit Julia's compiler. The key change involved pre-allocating arrays and using specialized array operations, which allowed the compiler to avoid runtime allocation overhead.
Effective type annotations are another key. Providing clear type information allows the compiler to make more aggressive optimizations, resulting in faster code. Consider the difference between a function with no type annotations versus one where the input and output types are explicitly declared. The latter gives the compiler significantly more information, enabling superior performance. The implications extend to large-scale scientific computing, where efficient resource utilization is paramount. Ignoring type stability can lead to substantial performance penalties.
Understanding the interaction between Julia's compiler and your code is crucial. Profiling tools can help identify performance bottlenecks, enabling targeted optimization efforts. A thorough grasp of the compiler's inner workings allows programmers to write code that is not only correct but also highly efficient.
Memory Management: Avoiding the Pitfalls
Efficient memory management is crucial for high-performance computing. Julia, while offering automatic garbage collection, presents opportunities for manual optimization. Understanding how Julia handles memory allocation and deallocation is essential. Pre-allocating arrays is a simple yet effective technique to significantly reduce memory allocation overhead. This is because dynamic allocation can introduce delays as the runtime system searches for suitable memory blocks. By pre-allocating, you provide the runtime system with the necessary memory up front, eliminating this delay. This is especially impactful in loops where repeated allocations are a common performance bottleneck.
Case Study 1: A high-frequency trading algorithm experienced a marked reduction in latency by pre-allocating memory for data structures used within critical loops. The reduction in latency resulted in improved order execution speed and enhanced profitability.
Case Study 2: A scientific simulation demonstrated significant speed improvements after refactoring its memory management. The key was identifying memory-intensive operations and using pre-allocation strategies to eliminate runtime memory management overhead. The result was faster simulation runs and efficient resource utilization.
Beyond pre-allocation, using immutable data structures where appropriate can help the garbage collector's performance. Immutable structures don't change after creation, simplifying garbage collection. Understanding when to use mutable versus immutable data structures directly impacts the overall performance of the application. Julia’s features allow developers to fine-tune the balance between mutability and performance based on specific application needs. Incorrectly managing mutable structures can severely impact efficiency. In large-scale applications, this can translate to significant resource savings and improved runtime performance.
Careful consideration of memory usage is crucial, especially in memory-constrained environments. Tools for memory profiling help identify memory leaks and inefficient memory utilization, facilitating effective optimization.
Parallel and Distributed Computing: Unleashing Julia's Power
Julia's inherent support for parallel and distributed computing sets it apart from many other languages. Leveraging multiple cores or even clusters of machines significantly speeds up computations, particularly when dealing with large datasets. Julia's built-in mechanisms, like `Threads.@threads` for multi-threading and `Distributed` for distributed computing, greatly simplify parallelization. However, understanding the nuances of parallel programming is crucial to avoid common pitfalls like race conditions and deadlocks. For instance, improper synchronization in multi-threaded code can lead to incorrect results and unexpected behavior.
Case Study 1: A large-scale weather simulation drastically reduced computation time by parallelizing its core algorithms using Julia's `Threads.@threads` macro. The parallelization allowed the simulation to leverage multiple cores, yielding significant performance gains.
Case Study 2: A genetic algorithm for optimization problems saw substantial speedup when implemented using Julia’s `Distributed` package, distributing the computation across multiple machines in a cluster. This approach efficiently leveraged the combined processing power, leading to faster convergence and improved results.
Effective parallelization requires careful consideration of data partitioning, communication overhead, and synchronization mechanisms. A well-designed parallel algorithm minimizes communication overhead, maximizing the benefits of parallel execution. Improper data partitioning can lead to load imbalances, significantly hindering performance. Thorough testing and profiling are crucial in this context to ensure optimal performance.
Choosing the appropriate parallelization strategy depends on the application’s characteristics. For some problems, multi-threading within a single machine might be sufficient, while others might require distributed computing across multiple machines. Julia provides the flexibility to choose the most efficient approach for specific tasks.
Leveraging Packages and Libraries: Building on Existing Work
Julia's vibrant ecosystem of packages offers pre-built solutions for many common tasks. Using these packages can save development time and improve code quality. However, choosing the right packages and understanding their performance characteristics is crucial. Not all packages are created equal; some are highly optimized while others might have performance limitations. Benchmarking different packages is essential to determine which performs best for your needs. Julia's package manager makes this process straightforward.
Case Study 1: A data science project leveraging Julia's `DataFrames` package for data manipulation experienced significant performance improvements compared to similar projects using other languages. `DataFrames`’ optimized data structures and algorithms facilitated efficient data processing and analysis.
Case Study 2: A machine learning application used Julia’s `Flux.jl` deep learning library, achieving comparable or even superior performance compared to Python’s TensorFlow or PyTorch. `Flux.jl`’s integration with Julia's compiler and its emphasis on performance contributed to the observed gains.
Understanding the strengths and weaknesses of different packages allows developers to make informed decisions. Careful consideration of package dependencies is also vital to avoid compatibility issues and performance bottlenecks. Dependencies can influence performance significantly; a poorly performing dependency can degrade the overall application speed.
The Julia community actively contributes to and maintains packages, ensuring the availability of well-tested and high-performance tools. Staying updated with new package releases and improvements can lead to continuous performance enhancements.
Advanced Optimization Techniques: Fine-tuning for Peak Performance
Beyond the basic techniques, advanced optimization methods can further enhance Julia's performance. These techniques often involve lower-level manipulations of code, taking advantage of specific hardware features or compiler optimizations. Techniques like loop fusion (combining multiple loops into a single one), vectorization (using SIMD instructions for parallel processing), and manual memory management in performance-critical sections can yield substantial speed improvements. These methods demand a deep understanding of both the Julia language and the underlying hardware architecture.
Case Study 1: A computational fluid dynamics simulation using Julia was optimized by carefully analyzing its inner loops, identifying opportunities for loop fusion and vectorization. The restructuring of the loops significantly improved the simulation's speed. A considerable gain in efficiency was realized through a deeper level of code manipulation.
Case Study 2: A graphics rendering application implemented manual memory management in its most performance-sensitive sections, significantly reducing memory allocation overhead. The judicious application of manual memory management resulted in noticeably faster rendering times. Manual control is not advisable without extensive expertise.
Advanced optimization is a complex undertaking, requiring specialized expertise and in-depth profiling. Profiling tools help pinpoint bottlenecks, providing insights into where optimization efforts should be focused. Premature optimization should be avoided; focusing optimization efforts on the most computationally intensive parts of the code yields the largest gains. The trade-off between optimization effort and performance gains must be carefully considered. While these techniques can bring significant improvements, the investment in time and expertise should align with the potential returns.
Julia's advanced features allow for fine-grained control over performance, enabling developers to squeeze out maximum efficiency from their code. However, such techniques must be applied carefully, lest they introduce unintended side effects or complicate maintenance.
Conclusion
Julia’s performance capabilities extend far beyond what’s immediately apparent. Mastering the subtleties of its compiler, memory management, parallel processing capabilities, and leveraging its rich package ecosystem unlocks unprecedented speed and efficiency. By understanding and applying the techniques discussed, developers can transform their Julia code from merely functional to truly exceptional, achieving levels of performance that rival or surpass those of even the most optimized compiled languages. The journey to mastering Julia's performance potential is ongoing, but the rewards—in speed, efficiency, and ultimately, success—are substantial.