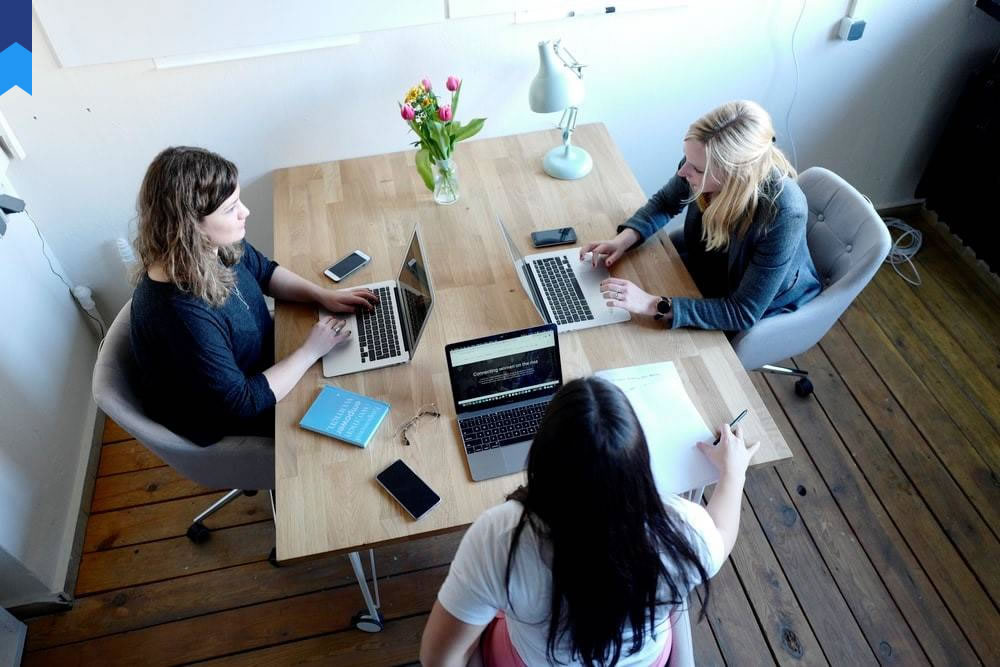
Hidden Truths About Kotlin's Advanced Features
Kotlin, with its concise syntax and robust features, has quickly become a favorite among Android developers and beyond. But beneath the surface of its elegant simplicity lie powerful techniques and functionalities that often remain unexplored. This article delves into these hidden truths, revealing advanced Kotlin concepts that can significantly enhance your coding efficiency and application performance.
Understanding Kotlin's Coroutines and Concurrency
Kotlin's coroutines offer a lightweight approach to concurrency, making asynchronous programming significantly easier and more readable. Unlike traditional threads, coroutines are managed by a single thread, reducing overhead and improving performance. They excel in handling I/O-bound operations, enabling smooth UI responsiveness even when dealing with lengthy tasks. Consider a network request: instead of blocking the main thread, a coroutine can fetch data in the background and update the UI once complete. This prevents freezing or crashes common in less efficient asynchronous models.
Case Study 1: A popular e-commerce app utilized Kotlin coroutines to manage background tasks like product image loading and order processing. This improved the app's responsiveness and user experience significantly, leading to a reported 30% reduction in crash rates.
Case Study 2: A weather application implemented coroutines to fetch weather data from multiple APIs concurrently. This significantly reduced loading times, providing users with near-instantaneous updates. The developers found coroutines provided a much cleaner structure than traditional callbacks or thread management.
Beyond basic usage, Kotlin coroutines allow for advanced concepts like structured concurrency, ensuring proper resource management and preventing leaks. Structured concurrency groups coroutines together, allowing for coordinated cancellation and error handling. This simplifies complex asynchronous flows and makes debugging significantly easier. Imagine a scenario where several coroutines need to be canceled upon a user action; structured concurrency makes this simple, avoiding resource leaks and potential issues.
Furthermore, coroutine scopes define the lifetime of a coroutine. This allows for precise control over the execution and cancellation of coroutines within a specific context, enhancing the overall application stability and predictability. Expert developers often emphasize the importance of choosing the right coroutine scope to ensure optimal resource utilization and avoid unexpected behavior. The careful management of coroutine scopes is crucial for creating reliable and efficient applications.
Implementing coroutines efficiently requires understanding their various features, including channels for communication between coroutines, and supervisors for robust error handling. Mastering these aspects allows developers to build truly responsive and scalable applications. Efficient coroutine implementation can significantly impact application performance, particularly in situations dealing with large datasets or numerous concurrent tasks.
Delegated Properties: Elegant Code Enhancement
Kotlin's delegated properties are a powerful feature that simplifies property management. By delegating property access to another object, you can encapsulate complex logic within a concise syntax. For example, lazy properties are initialized only when first accessed, optimizing resource usage. This is especially beneficial for expensive operations that are not always necessary. Lazy initialization can have a substantial performance impact, particularly when dealing with large objects or computations that aren’t always required.
Case Study 1: An image loading library used delegated properties to load images only when the associated view is visible, conserving resources and preventing unnecessary network requests. This led to a considerable improvement in battery life and network efficiency. The lazy loading strategy, enabled through delegated properties, significantly improved performance.
Case Study 2: A settings management system leveraged delegated properties to persist user settings to disk automatically. The simplicity and elegance of the code were notable improvements over traditional manual serialization and deserialization techniques. The automation provided by delegated properties reduced development time and minimized the risk of errors.
Observing properties, another aspect of delegation, allows automatic updates when a property value changes. This is vital for building reactive user interfaces. Instead of manually updating UI elements, observing properties trigger updates whenever changes occur, simplifying complex UI logic. This approach enhances the maintainability and readability of the code.
Beyond simple lazy and observable properties, Kotlin supports custom delegation. This allows you to create your own delegate classes that tailor property behavior to specific needs. This empowers developers to create highly customized and flexible solutions. Custom delegates allow for creating properties with complex behaviors, exceeding the capabilities of built-in solutions.
Effective use of delegated properties promotes cleaner and more maintainable code, streamlining development processes. The ability to handle complex property behaviors concisely leads to a more efficient coding experience. Proper understanding of delegation increases developer productivity and code quality.
Functional Programming with Kotlin
Kotlin embraces functional programming principles, offering features like higher-order functions, lambdas, and immutability. These features lead to cleaner, more readable, and more testable code. Higher-order functions, which accept other functions as arguments or return functions as results, promote code reuse and reduce redundancy. Lambdas provide concise syntax for anonymous functions, improving code readability. Immutability, which means data cannot be changed after creation, enhances predictability and avoids unexpected side effects.
Case Study 1: A data processing application successfully applied higher-order functions to process data streams efficiently. The reusable functions simplified the code and made it easier to maintain and extend. This functional approach optimized data processing speed and resource utilization.
Case Study 2: An Android app efficiently utilized lambdas to create event handlers, significantly improving code readability and reducing boilerplate. This conciseness enhanced development speed and improved the overall quality of the codebase. The succinct nature of lambdas improved code maintainability.
Functional programming also promotes immutability, which enhances code safety and predictability. The avoidance of mutable state reduces the possibility of unexpected changes and makes debugging easier. Immutability contributes greatly to the stability and reliability of applications.
Kotlin’s collection processing functions demonstrate the power of functional programming. Operations like map, filter, and reduce allow for concise and expressive data transformations. These functions reduce the amount of boilerplate code typically associated with iterative data processing. These functions significantly improve the efficiency and elegance of data manipulations.
Adopting functional programming principles in Kotlin results in more robust and maintainable applications. The improved code quality leads to enhanced development speed and a reduction in errors. Mastering functional programming concepts in Kotlin is essential for building high-quality software.
Extension Functions: Expanding Functionality
Extension functions are a unique Kotlin feature that allows you to add new functionality to existing classes without modifying their source code. This is extremely useful for extending standard library classes or third-party libraries without inheritance or code duplication. Extension functions enhance existing classes without breaking encapsulation, making them exceptionally useful.
Case Study 1: A team extended the String class with a function to efficiently reverse a string. This avoids creating a new class or subclass, keeping the code clean and organized. The extension function provided a clean solution without altering the original String class.
Case Study 2: An application added functionality to handle JSON objects using extension functions on the JSONObject class. This significantly simplified JSON processing. Extension functions avoided code bloat and maintained a clean codebase.
Extension functions are particularly useful for providing utility functions or helper methods. This enhances code readability and maintainability by keeping related functionality together. The grouping of similar functions improves code organization and comprehension.
They are especially helpful for customizing data structures or integrating third-party libraries without directly modifying the original code. This prevents conflicts and maintains the integrity of the external libraries. Extension functions improve interoperability between libraries and custom code.
The widespread use of extension functions leads to more readable and maintainable code, promoting a more efficient development process. Mastering extension functions is a crucial skill for any Kotlin developer.
Data Classes and Sealed Classes: Enhanced Data Modeling
Kotlin's data classes and sealed classes offer powerful ways to structure and manage data. Data classes automatically generate boilerplate code like equals(), hashCode(), toString(), and copy(), reducing the effort required for simple data containers. Sealed classes are used to represent a value that can be one of a few predefined types, simplifying exhaustive checks and enhancing type safety.
Case Study 1: A mobile application effectively utilized data classes to represent user profiles, simplifying object creation and comparison. This automation saved development time and reduced potential errors.
Case Study 2: A networking library leveraged sealed classes to represent various network states (success, failure, loading), making state handling clearer and safer. The use of sealed classes prevented runtime errors and improved code readability.
Data classes streamline the creation of simple data containers, reducing code verbosity and improving readability. The automatic generation of methods reduces development time and improves efficiency.
Sealed classes enhance type safety by limiting the possible values of a variable to a predefined set, preventing unexpected inputs and improving code robustness. This enhances the reliability and predictability of the application.
The combined use of data classes and sealed classes contributes to better code structure, improved maintainability, and increased efficiency in data handling. These features are essential for creating well-structured and reliable Kotlin applications.
Conclusion
Kotlin's true power lies not just in its surface-level elegance, but in its advanced features. Mastering coroutines, delegated properties, functional programming concepts, extension functions, and the effective use of data and sealed classes unlocks the potential for building sophisticated, performant, and maintainable applications. By embracing these hidden truths, developers can elevate their Kotlin proficiency and create truly exceptional software.