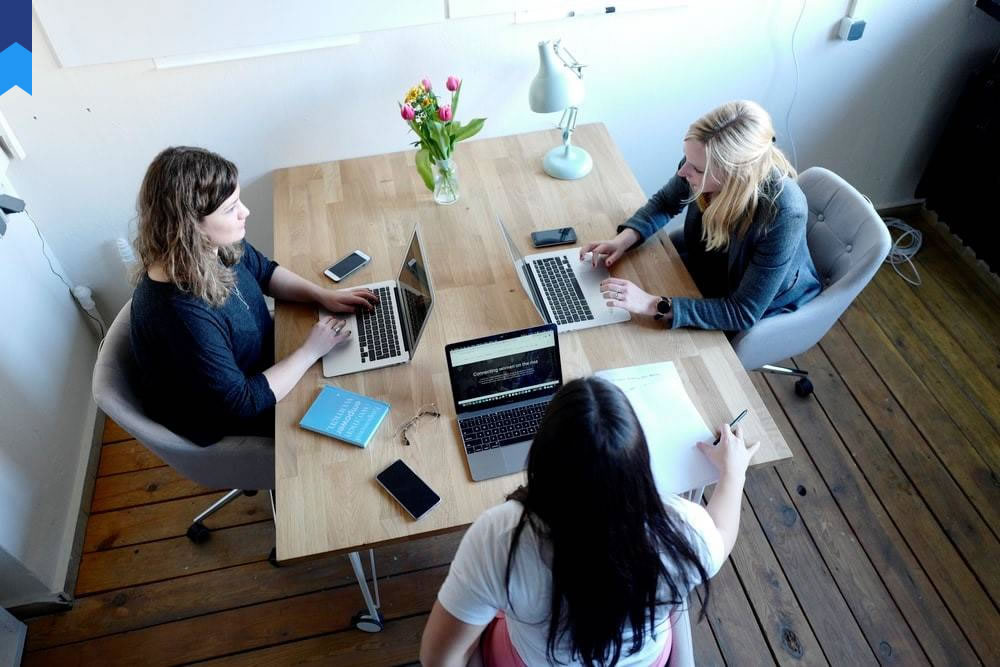
How Effectively To Manage ADO.NET Datasets?
Efficiently managing ADO.NET datasets is crucial for building robust and scalable applications. This article delves into advanced techniques beyond the basics, exploring strategies for optimal performance, data integrity, and efficient resource utilization. We will examine various aspects, including data caching, connection pooling, transaction management, and asynchronous operations, and showcase real-world examples to illustrate best practices.
Understanding ADO.NET Dataset Optimization
Optimizing ADO.NET dataset performance is paramount for smooth application operation. Inefficient data handling leads to sluggish response times and resource exhaustion. Careful consideration of data retrieval and manipulation is critical. Effective strategies include minimizing the amount of data retrieved from the database, using stored procedures to leverage the database's optimized execution plans, and utilizing data caching mechanisms for frequently accessed data. For example, consider an e-commerce application where product information is frequently accessed. Caching this information in a local data structure reduces database load, boosting application responsiveness. Another effective technique involves using parameterized queries to prevent SQL injection vulnerabilities and improve performance by allowing the database engine to reuse query plans. This is especially important in applications handling user-submitted data.
Case Study 1: A large financial institution experienced significant performance improvements by implementing a caching strategy for frequently accessed account balances. The reduction in database queries translated to a 30% decrease in response times for their online banking application.
Case Study 2: An online retailer optimized its product catalog browsing functionality by using stored procedures and parameterized queries. This resulted in a 20% reduction in database load and a noticeable improvement in page load times.
Leveraging connection pooling is another vital aspect. Connection pooling reduces the overhead associated with establishing new database connections for each request. Connection pools create a pool of pre-established connections, improving performance and scalability. Careful management of connection pool size and timeout settings is crucial to avoid resource exhaustion and maintain optimal responsiveness. Furthermore, implementing proper error handling and exception management is important to ensure data integrity and gracefully handle unexpected situations. By utilizing try-catch blocks and appropriate exception handling mechanisms, the application can prevent data corruption and ensure a stable user experience. Data validation is also critical in ensuring data integrity and preventing unexpected behavior. Validation rules, implemented before data is persisted to the database, guarantee that only valid data is accepted, improving application reliability.
Proper data sanitization is equally crucial for security and integrity, protecting against potential SQL injection attacks. This process removes or neutralizes potentially harmful elements from the data before it's used in database queries. Thorough testing and validation of the data before it enters the database are essential to prevent data corruption and maintain the integrity of the entire system. Regular system monitoring is also essential in identifying and addressing potential issues that may affect the performance and reliability of the application. This includes monitoring database query execution times, resource utilization, and error rates. This allows for proactive identification and resolution of performance bottlenecks.
Advanced Transaction Management
Transactional integrity is a cornerstone of database management. Ensuring that data modifications are processed atomically, consistently, and durably is vital for maintaining data integrity. ADO.NET provides robust transaction management capabilities. Using transactions ensures that a series of database operations either succeed completely or fail entirely, preventing partial updates that can lead to inconsistencies. For instance, in a banking application, transferring money between two accounts requires an atomic transaction. If one part of the transfer fails, the entire operation should be rolled back, preventing inconsistencies in account balances. Transactions also help maintain data consistency in concurrent access scenarios. Multiple users may access and modify the same data simultaneously. Transactions manage concurrent access, ensuring that data is consistent even with concurrent updates.
Case Study 3: An airline reservation system implemented transactions to ensure that seat reservations are atomic. If a seat is already booked, the transaction prevents overbooking. This guarantees data consistency and avoids issues caused by concurrent access.
Case Study 4: An e-commerce platform uses transactions to manage order processing. This prevents inconsistencies, such as an order being marked as complete without the corresponding inventory update. This ensures data integrity in a high-traffic environment.
Proper isolation levels are essential in transaction management. They define how transactions interact with each other. For example, a read uncommitted isolation level allows a transaction to see data changes made by other uncommitted transactions, leading to potential dirty reads. Conversely, a serializable isolation level guarantees that transactions are executed as if they were performed serially, preventing all anomalies. Choosing the right isolation level is crucial to balance concurrency and data consistency. Implementing proper rollback mechanisms is also essential. In case of errors or exceptions, the rollback mechanism should revert the database to its previous state before the transaction began, maintaining data integrity. This is particularly critical in error-prone operations or those prone to failure.
Efficient error handling and logging mechanisms are vital. Logging transaction-related events allows for comprehensive monitoring and debugging. This makes it easier to identify and troubleshoot potential issues. Detailed error logs provide valuable information for tracing the causes of transaction failures. Furthermore, efficient resource management, including connection handling and proper disposal of objects, is crucial for optimal resource utilization. Failing to properly close connections and dispose of objects can lead to resource leaks and performance degradation. Careful management of database resources is essential for maintaining application performance and stability.
Asynchronous Operations and Performance
In today's demanding applications, responsiveness is key. Asynchronous operations enhance performance by allowing the application to continue processing other requests while waiting for long-running database operations to complete. ADO.NET supports asynchronous operations through the `async` and `await` keywords (introduced in C# 5.0). Using asynchronous methods prevents blocking the main thread while waiting for database results. This improves responsiveness and user experience, particularly in applications handling multiple concurrent requests.
Case Study 5: A social media platform uses asynchronous database operations to handle user updates and interactions. This maintains a fast and responsive user experience even under heavy load.
Case Study 6: An online gaming platform utilizes asynchronous database calls for game state updates. This ensures the game remains responsive and free from lag, despite the high volume of data updates.
Efficiently utilizing asynchronous programming requires careful consideration of task scheduling and concurrency. Properly managing tasks and threads is essential to maximize performance and avoid bottlenecks. This includes understanding how to handle exceptions and errors arising from asynchronous operations. Implementing robust error handling mechanisms and logging ensures that errors are correctly handled and tracked. Understanding the nuances of asynchronous programming, such as task cancellation and timeout management, is also essential. Properly handling these aspects prevents unexpected behavior and resource leaks.
Asynchronous operations significantly improve scalability by enabling the application to handle more concurrent requests without compromising responsiveness. This is particularly important in high-traffic applications that receive a large number of simultaneous requests. The ability to manage these requests efficiently is crucial for providing a smooth user experience and maintaining application stability. Moreover, efficient queuing mechanisms can further improve the scalability of asynchronous operations. Queueing allows for handling requests efficiently even during peak loads, minimizing delays and improving application responsiveness. The selection of an appropriate queuing system is also an important design decision that impacts the scalability and performance of asynchronous operations.
Data Caching Strategies
Data caching is a vital technique for enhancing application performance by storing frequently accessed data in memory. ADO.NET doesn't directly provide caching mechanisms but integrates well with various caching solutions. Using a caching layer reduces database load, improving application responsiveness and scalability. Several caching strategies are available, such as in-memory caching (using libraries like `System.Runtime.Caching` or third-party caching systems) and distributed caching (using solutions like Redis or Memcached).
Case Study 7: A news website uses in-memory caching to store frequently accessed news articles. This significantly improves page load times, enhancing user experience.
Case Study 8: An online auction platform uses a distributed caching system to store user bidding information. This improves scalability and reduces database load during peak activity.
Selecting the appropriate caching strategy depends on several factors, including the size and frequency of accessed data, the application's scalability requirements, and the desired level of data consistency. Implementing cache invalidation strategies is crucial to prevent stale data from being returned to the application. Different invalidation strategies exist, including time-based expiration, write-through caching, and cache-aside pattern. Understanding the trade-offs between these strategies is essential for optimizing cache performance and data freshness. Moreover, efficient cache management requires careful consideration of memory usage and potential memory leaks. Properly managing cache size and implementing garbage collection mechanisms is critical for preventing memory exhaustion and maintaining application stability.
Caching mechanisms significantly improve performance and scalability, allowing applications to handle more requests without overburdening the database. The impact of caching on performance is substantial, often leading to significant reductions in database query times and overall response times. Careful monitoring of cache hit and miss ratios helps optimize caching strategies. Regular monitoring and analysis of cache usage provide insights into the effectiveness of the caching strategy and potential areas for improvement. Monitoring helps ensure that the caching layer is providing the intended performance benefits. Implementing appropriate logging and monitoring mechanisms is also important for understanding cache behavior and identifying potential issues.
Data Security and Best Practices
Data security is paramount in any application interacting with databases. ADO.NET provides several mechanisms to enhance data security. Parameterized queries are crucial for preventing SQL injection vulnerabilities. These vulnerabilities allow attackers to inject malicious SQL code into database queries, potentially compromising data integrity and security. Using parameterized queries protects against such attacks by treating user input as data rather than executable code. Input validation and sanitization are also essential to prevent malicious input from entering the database.
Case Study 9: A banking application uses parameterized queries to prevent SQL injection attacks. This protects user account information and maintains the integrity of the system.
Case Study 10: An e-commerce website uses input validation to prevent users from injecting malicious code into product descriptions or reviews. This safeguards the system against potential attacks and ensures data integrity.
Implementing proper access control mechanisms is essential to restrict access to sensitive data. Role-based access control (RBAC) allows for granular control over database access, ensuring that only authorized users can access specific data. This is particularly important in applications handling sensitive personal information or financial data. Encryption techniques play a critical role in protecting sensitive data at rest and in transit. Encrypting data before storing it in the database and during transmission protects it from unauthorized access, even if the database is compromised. Furthermore, regular security audits and penetration testing are essential for identifying potential vulnerabilities and ensuring the security of the application. These measures help to proactively address security risks and protect against potential attacks. By proactively identifying and addressing potential vulnerabilities, the organization can reduce the likelihood of data breaches and maintain the confidentiality and integrity of its data.
Staying updated with the latest security best practices is crucial for mitigating emerging threats. Regularly updating software and libraries helps protect the application from known vulnerabilities. Implementing security measures is not a one-time event, but an ongoing process that requires constant attention and updates to address new threats and vulnerabilities. Data security involves a multifaceted approach, combining technical measures with organizational policies and procedures to ensure the protection of sensitive information.
Conclusion
Mastering ADO.NET dataset management involves more than just basic CRUD operations. Efficient data handling relies on a combination of optimized data retrieval strategies, robust transaction management, asynchronous processing, effective caching techniques, and stringent security measures. By implementing the advanced techniques outlined in this article, developers can create high-performance, scalable, and secure applications. Focusing on these areas ensures efficient resource utilization, prevents data inconsistencies, and enhances application responsiveness, ultimately leading to a superior user experience.
Remember, continuous learning and adaptation to evolving technologies and best practices are essential for building robust and future-proof applications. Staying abreast of the latest advancements in database technology and security protocols is critical for maintaining the security and performance of the application. Regularly reviewing and updating the application’s architecture and design is essential to keep pace with the ever-changing technological landscape and ensure that the application remains secure, efficient, and scalable. In addition to the techniques discussed in this article, exploring other optimization techniques and leveraging advanced database features are crucial steps towards building high-performing ADO.NET applications.