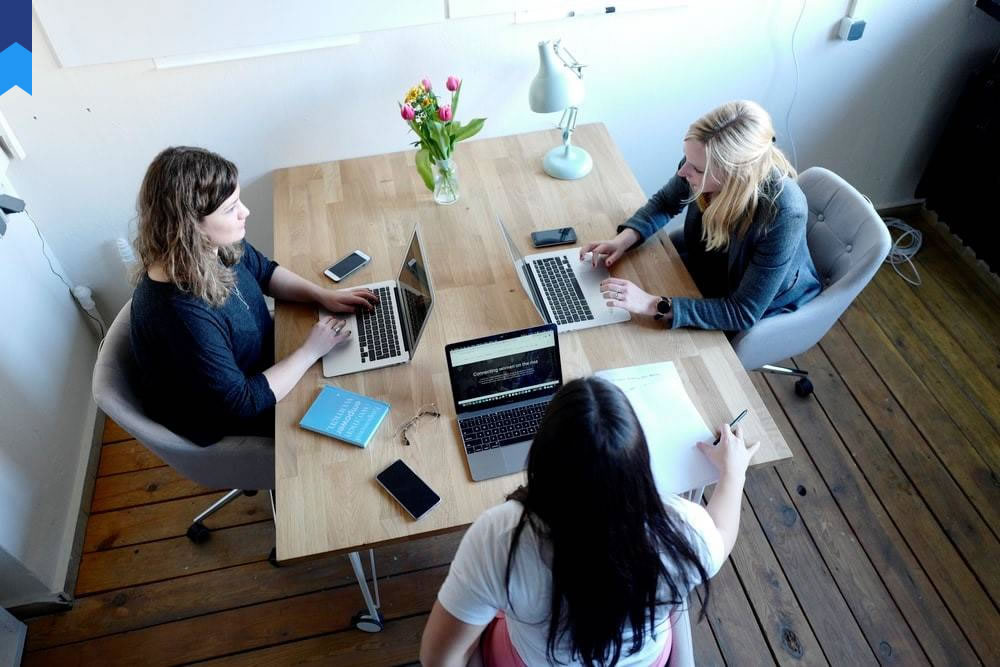
How Effectively To Manage Asynchronous Dart Streams With Firebase
Introduction: Dart, with its asynchronous capabilities, offers powerful tools for handling data streams. Firebase, a popular backend platform, often provides data in real-time streams. Effectively managing these asynchronous Dart streams interacting with Firebase requires a nuanced understanding of stream handling, error management, and efficient data processing. This article delves into practical techniques and best practices for seamless integration, ensuring robust and scalable applications. We'll explore advanced strategies beyond basic stream processing, focusing on optimizing performance and enhancing user experience.
Efficiently Handling Asynchronous Data Streams
Managing asynchronous data streams from Firebase in Dart requires careful planning and execution. The core principle lies in leveraging Dart's built-in asynchronous features, like `async` and `await`, to handle the inherent non-blocking nature of stream processing. This avoids blocking the main thread and ensures responsiveness. Using `StreamBuilder` widget efficiently updates the UI based on data changes without causing noticeable lags.
One effective approach involves creating dedicated stream controllers for managing specific data flows. This allows for better organization and easier debugging. Consider a scenario where you're managing user chat messages and online status updates. Two distinct stream controllers—one for each type of data—provide a more structured way to manage the incoming data. For example, `StreamController
Case Study 1: A real-time chat application utilizes separate stream controllers for messages, user presence updates, and typing indicators. This enables independent handling and optimization for each aspect of the chat experience. Using this structured approach prevents any single slow-loading or error-handling problem from affecting the rest of the app's functionality.
Case Study 2: A social media app uses stream controllers for managing updates to news feeds, notifications, and direct messages. Different streams allow the UI to update selectively, preventing UI overload from simultaneous data updates.
Furthermore, error handling within the stream processing pipeline is critical. Using `try-catch` blocks around stream operations prevents unexpected crashes. Implement mechanisms to gracefully handle connectivity issues or server-side errors and provide appropriate feedback to the user, such as displaying a loading indicator or an informative error message. Always prioritize robust error handling to guarantee a reliable application.
Implementing exponential backoff strategies for retry attempts when dealing with transient network problems is essential. This ensures consistent reconnections and data synchronization without overwhelming the server with repeated requests during temporary outages. This is key for providing a seamless experience to the user. Regularly checking the stream's state for errors using error handling mechanisms is also critical.
Optimizing Stream Performance
Efficiently managing asynchronous streams in Dart is crucial for building responsive and high-performing applications. A critical aspect of optimization is minimizing unnecessary data processing. Utilize Dart's stream transformations, like `map`, `where`, and `transform`, to filter and process data before it reaches the UI. This reduces load times and enhances responsiveness. Consider the scenarios below.
Scenario 1: Instead of sending a large dataset from Firebase, filter the data on the server-side using Firebase's query capabilities and only fetch the necessary subset. This greatly minimizes network traffic and processing overhead. This technique is crucial for enhancing application speed and providing a smoother user experience.
Scenario 2: Process large datasets on a background thread to avoid blocking the main UI thread. Utilize isolate libraries for creating isolated worker threads to handle computationally expensive operations, keeping the UI responsive. This ensures that the application remains usable while processing large datasets.
Case Study 1: A news aggregator app only fetches articles relevant to user preferences by applying filters at the backend before fetching data, dramatically improving load times.
Case Study 2: A data visualization tool uses background threads to process and render charts, preventing UI freezing during complex computations. This helps to improve application responsiveness, ensuring a smoother user experience.
Another key optimization technique is debouncing or throttling incoming data. This prevents overwhelming the application with frequent updates. Implementing debouncing delays processing until a short period of inactivity, while throttling limits the rate of updates within a specific timeframe. This is particularly useful for scenarios such as handling continuous user input or tracking rapidly changing data.
Efficiently using stream subscriptions is crucial. Avoid unnecessarily subscribing to the stream multiple times. Instead of creating multiple subscriptions, handle all the data processing within a single subscription. Ensure that streams are properly closed using `cancel()` when no longer needed to prevent memory leaks and unnecessary resource consumption. These practices are crucial for application stability and efficiency. Implement proper lifecycle management for stream subscriptions to ensure resource optimization.
Advanced Stream Management Techniques
Beyond basic stream handling, mastering advanced techniques is essential for building sophisticated applications. One such technique is the use of RxDart, a reactive programming library that extends Dart's stream capabilities. RxDart introduces operators such as `flatMap`, `switchMap`, and `combineLatest` allowing for more complex stream transformations and composition. These powerful operators provide enhanced control over data flows.
Consider an example where you need to fetch user data after receiving a user ID from a Firebase stream. Using `flatMap`, you can chain these operations, fetching user data only when the user ID changes. This elegant approach simplifies complex asynchronous operations. This reactive approach significantly reduces boilerplate code and improves code readability.
Case Study 1: An e-commerce application utilizes Rxdart to manage product updates and shopping cart data. Reactive programming ensures consistent data synchronization and efficient UI updates.
Case Study 2: A social media platform employs Rxdart to manage various data streams, including user activity, friend requests, and notifications, providing a responsive and consistent user experience.
Another crucial technique is the use of broadcast streams. Broadcast streams allow multiple listeners to simultaneously receive data from the stream without interference. This is advantageous in scenarios where multiple parts of the application need access to the same data stream. Using this prevents conflicts and ensures consistency.
Implementing mechanisms for managing multiple concurrent streams is also important. This involves creating strategies to coordinate and synchronize these streams, ensuring that data integrity is maintained. Using the `async` and `await` keywords appropriately and ensuring proper error handling when multiple streams interact is critical. For example, you may need to wait for several streams to complete before performing a specific action. This method is critical for the maintenance of data consistency across the streams.
Integration with Firebase Realtime Database
Integrating Firebase Realtime Database with Dart streams requires an understanding of Firebase's data structures and stream events. The `onValue` method provides real-time updates whenever data changes in the database. Understanding how to efficiently handle these updates is crucial. Efficient data retrieval using Firebase's query functionality and limiting the amount of data retrieved significantly improve application performance.
Consider a scenario where you're displaying a list of user comments. Instead of fetching all comments at once, you should paginate the data, fetching only a limited number of comments at a time. This improves both initial load times and subsequent data updates. It prevents unnecessary data transfer and processing overhead and thus enhances user experience.
Case Study 1: A messaging app uses Firebase's query capabilities to fetch only new messages since the user's last visit, reducing bandwidth usage and load times.
Case Study 2: A collaborative document editing tool uses Firebase's transactions to ensure data consistency across multiple users' simultaneous edits.
Properly managing disconnections and reconnections to the Firebase database is crucial for maintaining data consistency. Implement mechanisms to handle temporary network outages, ensuring that data synchronization resumes seamlessly upon reconnection. It prevents data loss and enhances the reliability of the application. Effective error handling and retry mechanisms are also crucial during network interruptions.
Data security is of utmost importance when integrating with Firebase. Securely handling sensitive data using Firebase's security rules is critical. These rules help to control access to data and prevent unauthorized access or modification. Implementing robust authentication mechanisms and authorization strategies helps to maintain data integrity and privacy. Prioritizing data protection is crucial for secure application development.
Error Handling and Robustness
Handling errors effectively is crucial for building robust and reliable applications. Unexpected issues like network outages, server-side errors, or invalid data can significantly impact user experience. Implementing comprehensive error handling strategies is crucial.
Effective error handling starts with proper exception handling. Utilize `try-catch` blocks to gracefully handle exceptions thrown during stream processing. Provide meaningful error messages to users, avoiding cryptic error codes. This enhances user experience by providing clarity.
Case Study 1: A banking application uses try-catch blocks to handle network errors, preventing the app from crashing and providing informative messages to users. This ensures application stability.
Case Study 2: A social media app handles invalid data from Firebase by gracefully recovering from errors, preventing unexpected behavior and enhancing user experience.
Implement logging mechanisms to record errors for later debugging and analysis. Detailed logs can help in identifying recurring problems and improving application stability. This enhances the debugging process significantly.
Beyond handling exceptions, build in resilience to temporary network issues. Implement retry mechanisms with exponential backoff to prevent overwhelming the server and improve the chances of successful reconnection. Implementing this ensures consistent application availability. It is crucial to consider various factors like network conditions and server availability for more reliable retry strategies.
Consider using a state management solution such as Provider, BLoC, or Riverpod to manage the application's state and handle loading and error states effectively. This approach provides a structured and manageable way to handle asynchronous operations and their outcomes. This is crucial for enhancing application organization and maintainability.
Conclusion: Effectively managing asynchronous Dart streams interacting with Firebase requires a multi-faceted approach. By leveraging advanced stream handling techniques, optimizing performance, and implementing robust error handling, developers can build high-performing, reliable, and user-friendly applications. This article has covered efficient strategies ranging from basic stream handling to advanced techniques utilizing Rxdart and integrating effectively with the Firebase Realtime Database, addressing potential challenges and offering best practices to ensure a seamless and robust user experience.