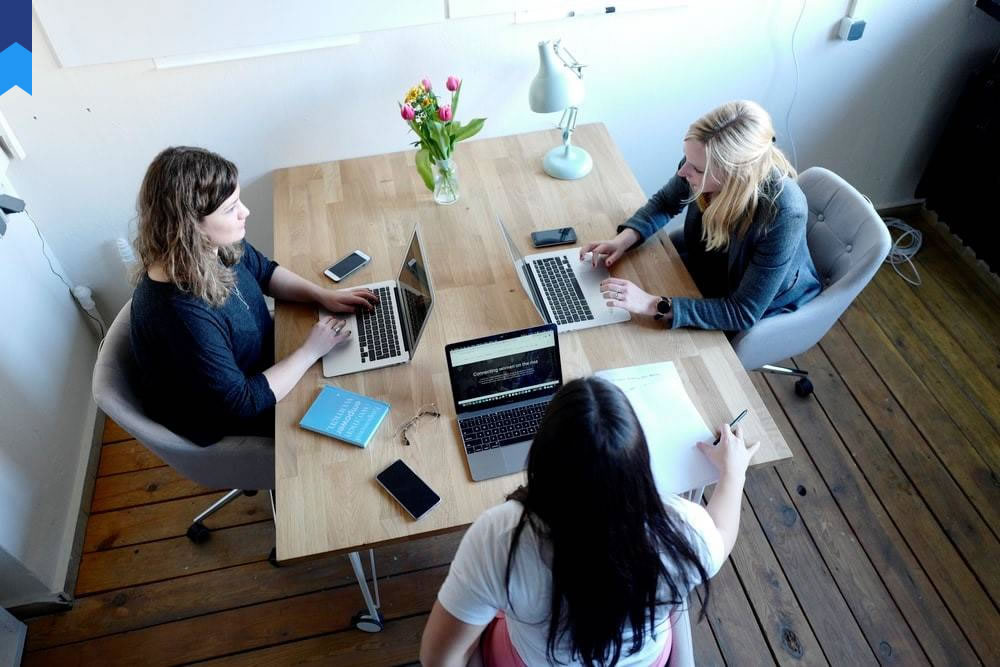
How Effectively To Manipulate Complex Word Documents Using Apache POI
Efficiently manipulating complex Word documents is a crucial task in many applications. Apache POI, a powerful Java library, provides the tools to accomplish this. However, mastering its intricacies requires a deep dive beyond basic tutorials. This article explores advanced techniques and best practices for tackling sophisticated Word document processing with Apache POI.
Advanced Table Manipulation
Apache POI offers robust capabilities for handling tables within Word documents. Beyond simple cell value modifications, advanced techniques involve dynamically adding rows and columns, merging cells, applying styles consistently across multiple tables, and handling complex table structures with nested tables or merged cells spanning multiple rows and columns. Consider a scenario where you need to generate reports with dynamically sized tables based on data retrieved from a database. Apache POI's flexibility allows you to create these tables programmatically, adjusting their dimensions based on the data's length. This eliminates the need for manual table creation and ensures consistency across reports. A poorly designed table handling approach, on the other hand, can lead to improperly formatted documents, data loss and significant debugging time.
Case Study 1: An e-commerce company uses Apache POI to generate personalized product catalogs. The number of products in each catalog varies, so POI dynamically creates tables, adjusts column widths, and applies formatting to present the information clearly. This approach saves hours of manual work and guarantees consistent presentation across all catalogs.
Case Study 2: A financial institution uses POI to generate reports with complex tables summarizing financial data. Tables within the reports need to accommodate varying numbers of rows and columns. Through the use of POI's advanced table manipulation features, the institution can ensure reports are accurate and visually appealing, reducing manual formatting effort significantly. The result is greater speed and accuracy in generating reports, improving efficiency and potentially reducing error.
Beyond simple cell modifications, consider more complex scenarios like dynamically inserting tables based on conditional logic, programmatically applying intricate table styles (including borders, shading, and cell alignment) and handling exceptions during table processing (like attempting to access a non-existent cell).
Proper error handling and robust validation are essential to prevent unexpected issues when working with complex tables. Employing best practices, including explicit type checking and utilizing exception handling mechanisms, reduces risk of application crashes.
Efficiently managing memory consumption is crucial when working with extensive data sets. Employing techniques like object pooling and efficient data structures help avoid memory leaks and performance degradation. This approach allows for processing large datasets efficiently, resulting in faster report generation and reduced server load.
Mastering Styles and Formatting
Effective document styling goes beyond basic font changes. Apache POI allows manipulating paragraph styles, character styles, lists, numbering, and even custom styles for advanced formatting. Consider the creation of a company newsletter that requires consistency in styling across various sections. Apache POI's style management features ensure adherence to branding guidelines and maintain a professional appearance across all parts of the document. An inconsistent approach to styling can lead to a less professional product and an inconsistent user experience.
Case Study 1: A marketing firm employs POI to create marketing materials with consistent branding. By defining and applying custom styles, they ensure consistent use of logos, fonts, and color schemes across all documents. This boosts brand recognition and creates a professional image.
Case Study 2: A law firm uses POI to generate standardized legal documents. The firm utilizes custom styles to ensure consistency in formatting and typography, facilitating easy readability and adherence to legal formatting guidelines. This consistency prevents errors caused by inconsistent formatting, enhancing clarity and precision.
Advanced styling techniques also include programmatically creating and applying custom stylesheets, managing style inheritance, and efficiently reusing styles across multiple documents. This is crucial for maintaining consistency in large-scale document generation projects.
Careful planning and organization of styles are essential for manageable code. Employing a hierarchical style structure enhances readability and makes maintenance easier.
Implementing automated testing helps prevent regressions in formatting. Automated tests ensure that styles are applied correctly, catching potential errors during development.
Thorough documentation of style definitions and their usage within the code improves code maintainability and understanding.
Working with Complex Content Controls
Content controls are powerful features in Word allowing for dynamic content insertion and user interaction. Apache POI offers ways to interact with various types of content controls, including text boxes, checkboxes, dropdowns, and date pickers. Imagine a system that needs to gather user input through interactive forms. Using POI's capabilities to handle content controls enables a seamless integration between document generation and data collection. Improper handling of content controls can result in flawed or incomplete forms.
Case Study 1: A survey platform utilizes POI to create dynamic surveys. The platform generates surveys with different content controls based on survey questions, enabling flexible and interactive questionnaires. This simplifies data collection and enhances user experience.
Case Study 2: A healthcare system uses POI to generate patient intake forms with content controls for personal information and medical history. This automated form generation improves efficiency and reduces errors. The use of content controls ensures accurate and consistent capture of data.
Advanced techniques include programmatically populating content controls with data, handling events associated with content control changes, and managing complex interactions between multiple controls. Proper error handling and data validation is crucial to safeguard data integrity.
Modular design and clear separation of concerns help in managing complex content control interactions, enhancing code organization and readability. Employing design patterns, such as the Model-View-Controller (MVC), improves code structure.
Efficient memory management is vital when dealing with many content controls, especially in large documents. The implementation of optimized data structures and memory-efficient techniques help mitigate performance issues.
Regular testing and validation are essential to ensure reliable interaction with content controls, detecting and correcting potential issues early in the development process.
Handling Images and Embedded Objects
Apache POI's ability to handle images and other embedded objects adds versatility to document manipulation. Beyond simply inserting images, advanced techniques involve dynamically resizing, repositioning, and styling images within the document. A marketing team creating a product brochure, for example, needs to precisely place images and ensure visual appeal. POI facilitates this fine-grained control, allowing for the creation of professional-looking brochures. Poor image handling can result in unprofessional or visually unappealing documents.
Case Study 1: A publishing house uses POI to automate the creation of books, placing images and figures dynamically throughout the text. This ensures consistent formatting and reduces manual effort, improving speed and accuracy.
Case Study 2: An educational institution uses POI to create presentation materials, dynamically inserting images and diagrams. This approach enhances visual appeal and clarity of presentations. POI's image handling capabilities create efficient document creation workflows.
Advanced image handling involves manipulating image properties (resolution, compression), handling different image formats, and dynamically adjusting image placement based on document layout. Consider the challenges associated with managing image scaling within the context of the surrounding text; POI addresses this through precise coordinate positioning.
Effective error handling and robust image validation are important to prevent unexpected behavior and application crashes when dealing with corrupted or incorrectly formatted images.
Thorough testing and validation ensure consistent image handling across various platforms and document types. Employing best practices, like exception handling, mitigates potential issues. Appropriate logging mechanisms aid in debugging image-related errors.
Careful consideration of image file formats and their impact on document size and loading time is crucial for optimal performance and user experience. Optimizing image files for web use enhances overall performance. This is important in scenarios where generated documents are uploaded online.
Advanced Document Structure Manipulation
Beyond simple text manipulation, Apache POI allows modification of the underlying structure of a Word document. This includes manipulating sections, headers, footers, and page numbers. A legal firm preparing complex legal documents, for instance, requires meticulous control over document structure. Apache POI provides the necessary tools to handle these structural elements efficiently. Inadequate handling can cause errors in document structure.
Case Study 1: A government agency uses POI to generate standardized reports with consistent headers, footers, and page numbering. This guarantees a uniform look and feel across reports, enhancing professionalism and readability.
Case Study 2: A research institution uses POI to prepare research papers with structured sections, headings, and footers, applying specific formatting to each section. This enhances readability and ensures consistency in presentation.
Advanced techniques include dynamically adding and removing sections, manipulating section properties (orientation, margins), and handling complex header and footer structures, including different headers/footers for odd/even pages and different sections. Proper error handling and validation are crucial for managing the document structure appropriately.
Careful planning and organization of the document structure are essential for efficient code management. This includes employing a clear hierarchical structure for sections and subsections. Use of design patterns helps in building a more robust solution.
Thorough testing and validation are essential to ensure that the changes to the document structure are applied correctly, avoiding unwanted side effects.
Regularly updating the code and testing to maintain compatibility with evolving Word document formats ensures that the application functions correctly over time and avoids potential issues with future updates to the Word format itself.
Conclusion
Mastering Apache POI for complex Word document manipulation involves understanding its advanced features and applying best practices. This article explored several critical aspects, including table manipulation, style management, content controls, image handling, and document structure manipulation. By applying these techniques, developers can build robust and efficient applications capable of generating highly customized and sophisticated Word documents. The benefits of using Apache POI for such tasks include automation, efficiency gains, improved consistency, and better error reduction compared to manual processes.
However, remember that effective use requires careful planning, code organization, and comprehensive testing. Employing best practices, including error handling and efficient memory management, are crucial for successful and reliable document manipulation. Ongoing maintenance and updates are also vital to adapt to evolving Word document standards and maintain optimal application performance.