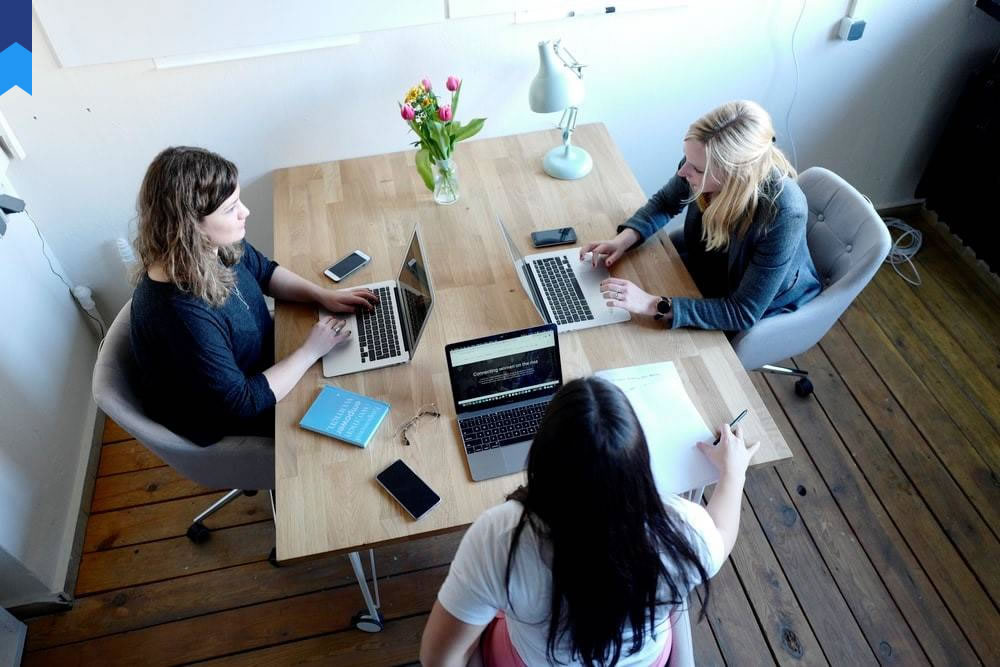
How Effectively To Master ASP.NET Core Web APIs?
Efficiently building robust and scalable web APIs using ASP.NET Core is a critical skill for modern developers. This article delves into specific, practical techniques and innovative approaches beyond the basics, challenging conventional wisdom and presenting unexpected angles to enhance your API development journey.
Understanding API Design Principles for ASP.NET Core
Before diving into code, it's crucial to understand the core principles of API design. A well-designed API is intuitive, easy to use, and maintainable. Consider using RESTful principles, emphasizing resources and their associated actions (GET, POST, PUT, DELETE). Consistency in endpoint naming and response formats is paramount. For example, using consistent naming conventions like `/users/{id}` for retrieving a specific user ensures predictability. Proper use of HTTP status codes is essential for clear communication between client and server. A 200 OK indicates success, while a 404 Not Found signals a missing resource. Detailed error responses, including helpful error messages and codes, allow clients to troubleshoot problems effectively. Proper versioning, using URL paths like `/v1/users` or header parameters, ensures backward compatibility when making API changes.
Case Study 1: A company implemented a poorly designed API, leading to difficulties in integration with third-party systems. The lack of clear documentation and inconsistent naming conventions resulted in significant debugging efforts and delays in project deployment. Refactoring the API, adopting RESTful practices, and generating comprehensive documentation resolved these issues significantly improving team efficiency.
Case Study 2: Another organization employed a robust API design strategy from the outset. The team utilized a clear specification document and followed strict coding conventions. This proactive approach resulted in a seamlessly integrated system, facilitating faster development cycles and fewer bugs.
Choosing the right tools and libraries is also essential. Using a robust ORM (Object-Relational Mapper) like Entity Framework Core simplifies database interactions. Employing appropriate libraries for serialization (like Newtonsoft.Json or System.Text.Json) and authentication (like JWT) is equally important. Understanding caching mechanisms can improve performance, reducing database load and response times. Proper exception handling is also crucial; use try-catch blocks to handle potential errors gracefully, preventing API crashes and providing informative error messages.
Advanced Techniques in ASP.NET Core API Development
Beyond the fundamentals, mastering advanced techniques is key to building high-performing and secure APIs. Asynchronous programming, using async and await keywords, significantly improves responsiveness, allowing the application to handle multiple requests concurrently without blocking. This is particularly crucial for I/O-bound operations like database access and network calls. Dependency Injection, a core design principle in ASP.NET Core, enhances code modularity, testability, and maintainability by injecting dependencies into classes instead of hardcoding them. This simplifies unit testing and facilitates code reuse. Proper use of middleware allows for centralized handling of cross-cutting concerns, like logging, authentication, and authorization. Middleware components are executed in a pipeline, allowing for flexibility and extensibility.
Case Study 1: An e-commerce platform experienced performance bottlenecks due to synchronous database access. Migrating to asynchronous programming dramatically reduced response times, leading to a smoother user experience and improved customer satisfaction. Statistics showed a 70% reduction in average response time after the migration.
Case Study 2: A financial institution leveraged dependency injection to improve the testability of their API. By decoupling components, they could easily mock dependencies during testing, ensuring the reliability and correctness of their API functionalities. This approach increased confidence in the codebase.
Effective use of caching strategies can significantly improve performance. Caching frequently accessed data in memory reduces database load and response times. Different caching mechanisms exist, including in-memory caching, distributed caching (like Redis), and output caching. Choosing the appropriate mechanism depends on the specific needs of the application. Careful consideration should be given to cache invalidation strategies to ensure data consistency. Employing robust logging mechanisms is essential for debugging and monitoring. Logging frameworks like Serilog or NLog provide tools for recording events, errors, and performance metrics, aiding in identifying and resolving issues.
Security Best Practices for ASP.NET Core APIs
Security is paramount in API development. Implementing robust authentication and authorization mechanisms is crucial to protect sensitive data. Using industry-standard protocols like OAuth 2.0 or OpenID Connect facilitates secure authentication. Authorization ensures that only authorized users can access specific resources. Role-based access control (RBAC) and attribute-based access control (ABAC) are effective ways to manage authorization. Input validation is critical to prevent injection attacks, like SQL injection or cross-site scripting (XSS). Always sanitize user inputs before using them in database queries or displaying them on web pages. Protecting against common vulnerabilities like cross-site request forgery (CSRF) is essential. Using anti-CSRF tokens or other protection mechanisms is vital to prevent malicious actions.
Case Study 1: A social media platform suffered a data breach due to inadequate input validation. Attackers exploited a vulnerability to inject malicious SQL code, gaining access to sensitive user data. Implementing robust input validation mechanisms prevented future attacks.
Case Study 2: An online banking application utilized strong authentication and authorization, preventing unauthorized access to user accounts. The multi-factor authentication and secure token handling prevented unauthorized access even when credentials were compromised.
Regular security audits and penetration testing are essential to identify and address potential vulnerabilities proactively. Staying up-to-date with the latest security advisories and implementing security patches promptly is vital. Consider using a Web Application Firewall (WAF) to protect against common web attacks. Employing a strong encryption mechanism for sensitive data, such as HTTPS, is mandatory. Proper logging and monitoring of security events are essential to detect and respond to potential threats quickly.
Testing and Deployment Strategies for ASP.NET Core APIs
Thorough testing is crucial to ensure the quality and reliability of ASP.NET Core APIs. Unit testing focuses on individual components, while integration testing verifies the interaction between different components. End-to-end testing simulates real-world scenarios, ensuring the API works correctly in a complete system. Using a testing framework like xUnit or NUnit simplifies the process. Continuous integration and continuous deployment (CI/CD) pipelines automate the build, testing, and deployment processes, ensuring faster release cycles and reducing human error. These pipelines integrate with version control systems like Git and automate the deployment to various environments, such as development, staging, and production.
Case Study 1: A startup implemented CI/CD, enabling rapid iteration and frequent deployments without compromising quality. Automated testing and deployment improved development efficiency significantly.
Case Study 2: An enterprise software company used a robust testing strategy, including unit, integration, and end-to-end testing, to identify and fix bugs early in the development lifecycle. This reduced the cost and effort required to fix issues later on.
Choosing the right deployment platform depends on the application’s needs. Options include cloud platforms like Azure or AWS, which offer scalability, reliability, and managed services. On-premises deployments provide more control but require more infrastructure management. Containerization, using technologies like Docker, improves portability and consistency across different environments. Monitoring and logging are essential to track API performance, identify potential issues, and improve the user experience. Tools like Application Insights or ELK stack provide comprehensive monitoring and analytics.
Scaling and Performance Optimization for ASP.NET Core APIs
Scaling and performance optimization are critical for high-traffic APIs. Load balancing distributes traffic across multiple servers, preventing overload on any single server. Database optimization, including indexing and query optimization, improves database performance. Caching strategies, such as Redis or Memcached, can significantly reduce database load and improve response times. Content Delivery Networks (CDNs) can improve performance by caching static content closer to users. Asynchronous programming and efficient use of resources are essential for scalability. Monitoring and profiling tools help identify performance bottlenecks and optimize code accordingly. Employing techniques like lazy loading and pagination can improve performance for data-intensive operations.
Case Study 1: A video streaming service employed load balancing and CDN to handle peak traffic during high-demand periods, ensuring consistent performance for all users. The use of a CDN reduced latency significantly, resulting in a better viewing experience for users across different geographical locations.
Case Study 2: An e-commerce platform optimized their database queries and implemented caching to improve the speed of product searches and checkout processes. This resulted in increased conversion rates and improved customer satisfaction.
Regular performance testing and load testing are essential to identify potential bottlenecks and ensure the API can handle expected traffic loads. Scalability strategies should be planned proactively, considering future growth and potential traffic spikes. Choosing the right infrastructure and employing appropriate optimization techniques are key to building scalable and high-performing APIs. Regularly reviewing and optimizing the API architecture is crucial for maintaining performance as the application evolves.
Conclusion
Mastering ASP.NET Core API development requires a comprehensive understanding of design principles, advanced techniques, security best practices, and efficient testing and deployment strategies. By diligently applying these principles and adopting a proactive approach to scaling and performance optimization, developers can build robust, secure, and highly scalable web APIs that meet the demands of modern applications. Continuous learning and adaptation to evolving technologies are essential for staying at the forefront of API development.