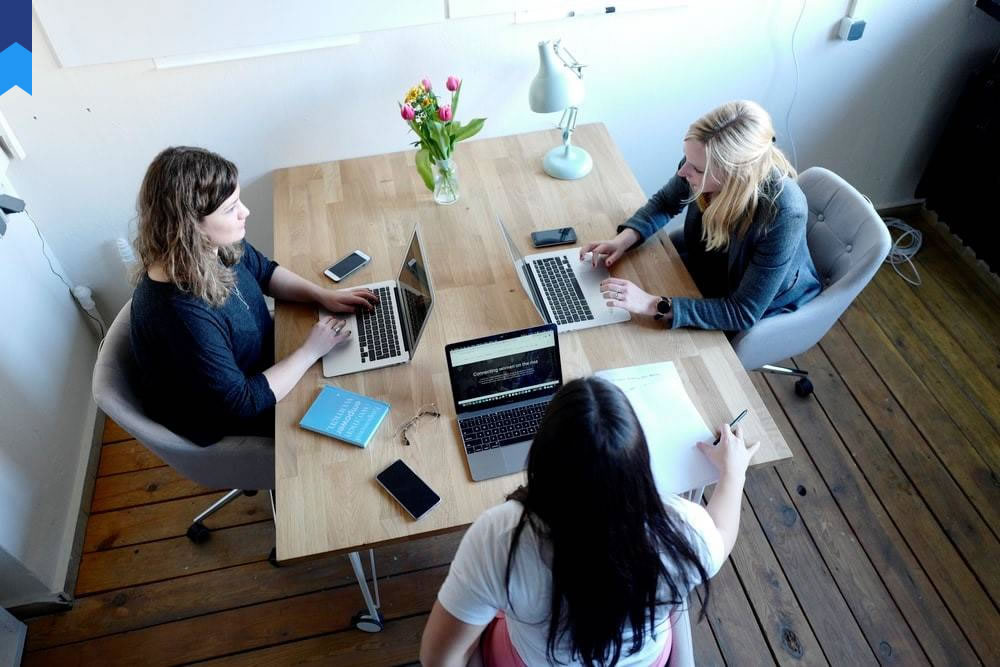
How Effectively To Master D's Advanced Features For Robust Applications Using Modern Techniques
Introduction: D, a systems programming language, offers a powerful blend of performance and safety. This article delves into advanced features, moving beyond basic tutorials to explore practical applications and innovative techniques. We'll examine memory management, metaprogramming, and concurrency features, providing real-world examples and case studies to demonstrate their effectiveness in building robust and high-performance applications. Mastering these features is crucial for any developer aiming to leverage D's full potential. We will explore how to effectively utilize these advanced capabilities, focusing on best practices and addressing potential pitfalls. This guide will empower you to write safer, more efficient, and ultimately, more successful D applications.
Mastering D's Memory Management
D's memory management system is a cornerstone of its strength. Unlike C or C++, D offers garbage collection, significantly reducing the risk of memory leaks and dangling pointers. However, understanding how to effectively utilize D's garbage collection is crucial to writing efficient and robust applications. Manual memory management, using techniques like `scope` and `new` / `delete`, is still possible and sometimes beneficial for performance-critical sections. This allows for fine-grained control and optimization. Consider the use of `std.gc.stacktrace` to investigate unexpected behavior. One case study involves a high-frequency trading application where controlled memory allocation within specific loops proved crucial for minimizing latency. Another example shows a game engine using a custom allocator to improve the performance of object pool management. Proper use of `@nogc` further enhances performance in specific contexts. Understanding the trade-offs between garbage collection and manual memory management is essential for efficient D programming. Developers must weigh the convenience of garbage collection against potential performance gains from manual control, especially in highly optimized projects. Improper use of `@nogc` can lead to performance degradation. Analyzing memory profiling data from tools like Valgrind is vital for identifying potential memory-related issues.
Unlocking the Power of D's Metaprogramming
D's metaprogramming capabilities, primarily through templates and mixins, allow developers to write generic code that adapts to different types and scenarios. This can drastically improve code reusability and reduce boilerplate. Templates, similar to those in C++, enable the creation of code that's generated at compile time based on input types. Mixins allow for code injection, seamlessly adding functionality to existing classes without inheritance. Consider building a custom logging system using templates to handle different log levels and output formats. Another example involves creating a flexible data serialization library where templates accommodate various data structures. Templates can become quite complex, making debugging challenging. Understanding the compilation process and using effective debugging strategies is key to success here. A common pitfall is inadvertently creating infinite template recursion which can lead to compilation errors. Efficiently leveraging metaprogramming significantly improves code quality and maintenance. It allows for abstractions that reduce the overall complexity of the project, leading to clearer and more maintainable code. Thorough testing becomes even more important when using metaprogramming, as subtle errors can easily propagate.
Conquering Concurrency in D with Ease
Modern applications often require concurrent execution to fully utilize multi-core processors. D provides powerful concurrency features, including threads, mutexes, and atomic operations. These tools allow developers to manage multiple tasks simultaneously while maintaining data integrity and avoiding race conditions. Threads provide a straightforward way to create independent execution flows. Mutexes ensure mutual exclusion to protect shared data. Atomic operations provide lock-free synchronization. Imagine a web server using threads to handle multiple client requests concurrently. Another example is a scientific simulation efficiently performing calculations on multiple cores. Concurrency introduces its own set of problems, primarily race conditions and deadlocks. Using appropriate synchronization mechanisms and applying careful design principles is crucial. Deadlocks often arise from improper ordering of lock acquisitions. Thorough testing and proper use of debugging tools are essential in identifying and resolving these issues. The use of asynchronous operations and channels can further improve performance and scalability. Leveraging D's built-in concurrency primitives is key to creating high-performing, scalable applications.
Exploring Advanced Data Structures and Algorithms
D's standard library offers a robust collection of data structures, such as associative arrays, linked lists, and trees. However, understanding when and how to use these structures appropriately is paramount. Choosing the right data structure drastically affects the efficiency of an algorithm. For example, an associative array might be ideal for fast lookups, while a linked list might be better for frequent insertions and deletions. Consider a graph traversal algorithm where the choice of data structure (adjacency matrix vs. adjacency list) heavily impacts performance. Another example is in game development where efficient collision detection relies on using optimized spatial data structures. Poor choices can lead to performance bottlenecks. Profiling tools are indispensable for identifying areas where data structure choices need optimization. Familiarity with various algorithms and their complexities is crucial. Big O notation helps in understanding and comparing the time and space complexities of different approaches. By leveraging D's features effectively, developers can create high-performing and efficient applications. This includes advanced features like generics, which allow writing code once and applying it to diverse data types. Furthermore, understanding memory layout and alignment can significantly improve the performance of data-intensive operations.
Implementing Robust Error Handling and Exception Management
Robust error handling is crucial for creating reliable applications. D provides exception handling mechanisms, allowing developers to gracefully recover from errors and prevent crashes. Proper exception handling helps in creating applications that are more fault-tolerant and less prone to unexpected failures. Exception handling requires careful consideration of potential error scenarios, including resource leaks and the potential cascading effect of exceptions. A well-designed exception-handling strategy ensures clean and consistent handling of errors throughout the application. Let's imagine a file processing application needing to handle missing files or permission errors gracefully. Another example is a network application that needs to handle network disconnections without crashing. Untrapped exceptions can lead to program termination, resulting in data loss or corruption. Effective exception handling often involves using try-catch blocks to anticipate errors and handle them effectively. It's also crucial to log errors appropriately, providing sufficient context for debugging and identifying the root cause. Logging allows for tracking and analysis of errors over time, improving the overall reliability and maintainability of the application. This helps in the proactive identification and resolution of issues.
Conclusion: Mastering D's advanced features is essential for building robust, efficient, and high-performing applications. This article explored memory management, metaprogramming, concurrency, data structures, and exception handling, providing practical examples and case studies. By adopting best practices and understanding the potential pitfalls, developers can leverage D's strengths to create sophisticated and reliable software solutions. Further exploration of advanced topics such as compile-time code generation and contract programming would only enrich one's D programming experience. Continuing education and practical application are crucial for truly mastering D's potential.