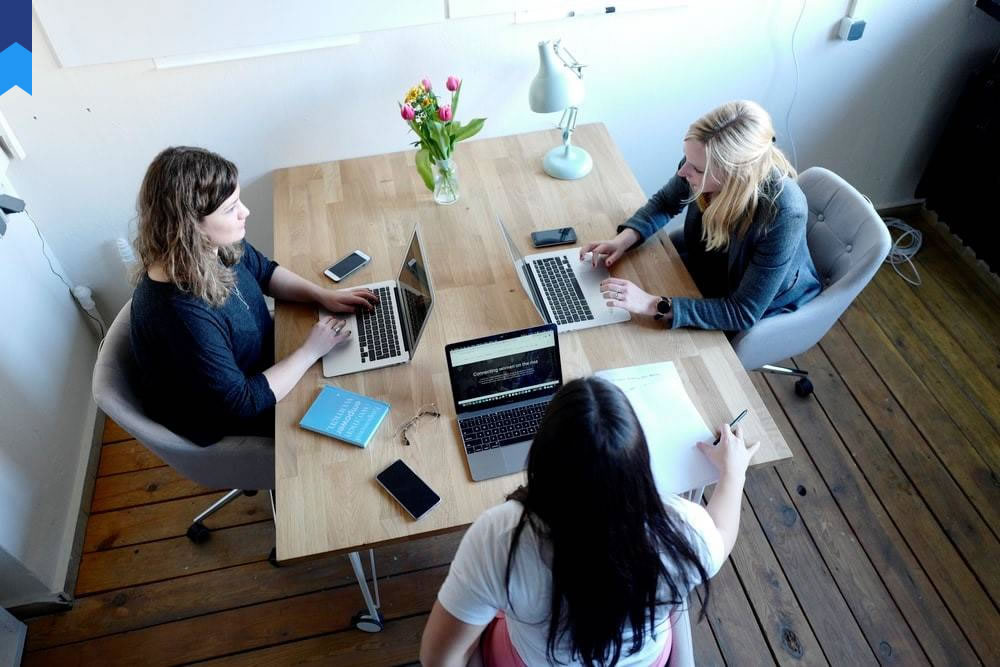
How Effectively To Master D's Advanced Features Using Modern Techniques
D is a systems programming language known for its power and efficiency. However, truly mastering its advanced features requires more than just basic tutorials. This article dives into specific, practical, and innovative techniques to elevate your D programming skills to a professional level.
Introduction
This article serves as a guide to help you navigate the intricacies of advanced D programming. We'll explore techniques beyond the fundamentals, focusing on practical applications and problem-solving scenarios. The goal is to empower you with the skills to write efficient, robust, and maintainable D code. We will dissect complex concepts and illustrate them with clear examples, case studies, and industry best practices. This journey will cover crucial aspects that many developers overlook, ultimately helping you become a more proficient D programmer.
Mastering advanced D features is essential for creating high-performance applications and systems. It requires a deep understanding of the language's capabilities, including memory management, metaprogramming, and concurrency. This article will serve as your comprehensive roadmap to achieve this mastery, offering practical strategies and real-world examples.
Throughout this article, we will emphasize practical application. Theoretical knowledge is important, but practical application solidifies understanding and fosters true mastery. We will explore effective methods for optimizing code, debugging efficiently, and leveraging D's unique strengths to solve complex programming challenges. The path to mastery is paved with consistent practice and a dedicated approach to understanding the underlying principles of D.
Mastering Memory Management in D
D's memory management capabilities are a cornerstone of its power. Understanding its features allows for precise control, preventing common pitfalls and boosting performance. Techniques like manual memory management with `new` and `delete`, RAII (Resource Acquisition Is Initialization) using destructors, and understanding the garbage collector's behavior are critical. The garbage collector, while helpful, is not a silver bullet; understanding its limitations and potential performance implications is crucial for optimal code.
Case Study 1: Consider a scenario involving large data structures. By carefully managing memory allocation and deallocation, we can significantly reduce memory footprint and improve application responsiveness. Using RAII for resource management ensures that resources are properly released even during exceptions.
Case Study 2: In high-performance computing, manual memory management, combined with compiler optimizations, can yield significant speed gains. By avoiding unnecessary heap allocations, we reduce the overhead associated with garbage collection.
Understanding D's memory models is crucial for writing efficient and reliable code. This includes appreciating the differences between stack and heap allocation and how these choices impact performance. Furthermore, using techniques like scoped allocation, where objects are automatically deallocated when they go out of scope, is highly recommended for improved code clarity and memory safety.
Employing sophisticated techniques like custom allocators or memory pools, suitable for particular use cases such as game development or embedded systems programming, can further refine your memory management skills. These advanced techniques demand a thorough understanding of the underlying memory architecture and potential trade-offs involved.
The key lies in balancing automatic garbage collection with manual memory management when needed. Understanding this delicate balance leads to creating programs that are both efficient and easy to maintain.
Harnessing the Power of D's Metaprogramming
D's metaprogramming capabilities allow for code generation at compile time, leading to incredibly powerful and flexible solutions. Understanding template metaprogramming, compile-time code generation, and mixins are essential for crafting elegant and efficient code. This can be used to perform complex tasks at compile time, reducing runtime overhead and increasing performance.
Case Study 1: Imagine generating highly optimized data structures at compile time based on runtime needs. Metaprogramming can adapt the data structures based on input parameters, resulting in significant performance improvements.
Case Study 2: Metaprogramming can also be used to simplify complex code patterns, improving readability and reducing the chance of errors. By generating repetitive code at compile time, we avoid manual repetition, enhancing code maintainability.
D's metaprogramming features give developers powerful tools for abstraction and code generation. For instance, you can create generic functions that operate on diverse data types without sacrificing performance, achieved by specializing the code at compile time. This enables powerful generic programming techniques without the typical runtime penalties associated with traditional polymorphism.
Furthermore, metaprogramming can be used to create Domain-Specific Languages (DSLs) within D itself. This increases expressiveness and makes the code more readable and maintainable for specific problem domains. The ability to generate code at compile time is essential for creating highly optimized and domain-specific solutions.
Mastering metaprogramming in D requires understanding its nuances, including template instantiation, template specialization, and the intricacies of the D compiler. This allows you to leverage its power responsibly, leading to more efficient and elegant solutions.
Conquering Concurrent Programming with D
Modern applications demand concurrent programming techniques. D offers powerful tools for concurrent programming. Understanding the intricacies of threads, mutexes, condition variables, and atomic operations is critical for building robust and scalable concurrent applications. Using these tools effectively requires careful planning and understanding of concurrency patterns to prevent race conditions and deadlocks.
Case Study 1: Consider a high-throughput server application. Using threads and appropriate synchronization primitives allows for handling multiple client requests concurrently, significantly improving performance and responsiveness. Incorrect synchronization can lead to data corruption and system instability.
Case Study 2: Developing a parallel algorithm for data processing. Efficiently utilizing multiple cores requires understanding how to partition data and coordinate execution, ensuring efficient use of system resources and minimizing synchronization overhead. Ignoring concurrency best practices may result in performance bottlenecks.
D provides several approaches to concurrency, including the use of threads and the more modern and often safer approaches employing coroutines. Understanding when to use each approach is crucial. Coroutines provide lightweight concurrency with less overhead than traditional threads, and they are ideal for many applications. However, threads still have their place in specific scenarios.
Memory management in concurrent programs is especially important. Careful consideration must be given to ensuring data consistency and avoiding race conditions. Techniques like atomics and mutexes are essential for protecting shared data. Using proper synchronization mechanisms is critical in preventing data corruption and ensuring program correctness.
Employing well-established concurrency patterns, such as producer-consumer or pipeline patterns, enhances code organization, simplifying both development and maintenance. Properly structured concurrent code improves readability and reduces the risk of subtle bugs.
Efficiently Utilizing D's Standard Library
D boasts a rich standard library offering a wide range of functionalities. Mastering this library significantly improves development speed and code quality. This includes utilizing data structures like `std.container.Array`, `std.container.RedBlackTree`, and `std.algorithm` modules for efficient operations on data. Understanding the strengths and weaknesses of each data structure is crucial for choosing the optimal one for a given task.
Case Study 1: Using `std.algorithm` for sorting or searching large datasets. Leveraging optimized algorithms significantly improves the performance compared to manually implementing them. Choosing the appropriate algorithm depending on the nature of the data is crucial for optimization.
Case Study 2: Utilizing `std.container.RedBlackTree` for efficient lookups in a large collection of data. This data structure offers logarithmic time complexity, making it suitable for scenarios where frequent lookups are required. Understanding the trade-offs between different container types is crucial for performance.
The standard library also includes features for input/output operations, networking, and other common tasks. Familiarizing oneself with these modules avoids reinventing the wheel, leading to increased development speed and reduced chances of errors. Understanding the provided functionalities helps leverage existing, well-tested, and efficient implementations.
Using the standard library effectively helps in writing more concise and readable code. Instead of implementing common utilities, developers can focus on the core logic of their application. Efficient use reduces development time and increases maintainability.
Furthermore, the standard library's modules are regularly updated and optimized. Staying current with the latest versions ensures access to improved performance and new features, contributing to efficient and robust applications.
Conclusion
Mastering advanced D programming demands a commitment to understanding its nuances. This article highlights crucial aspects of memory management, metaprogramming, concurrent programming, and the effective utilization of the standard library. By mastering these techniques, developers can build high-performance, reliable, and maintainable applications. The path to mastery involves constant learning, experimentation, and a dedicated approach to writing clean, efficient code. Continuous exploration of D's capabilities unlocks the potential for creating exceptional software solutions.
Remember that effective D programming is not just about knowing the syntax; it's about understanding the underlying principles and applying them creatively. The insights provided here serve as a foundation for further exploration and refinement of your D programming skills. Through consistent practice and a dedication to understanding the intricacies of the language, you can transform yourself into a highly proficient D programmer.