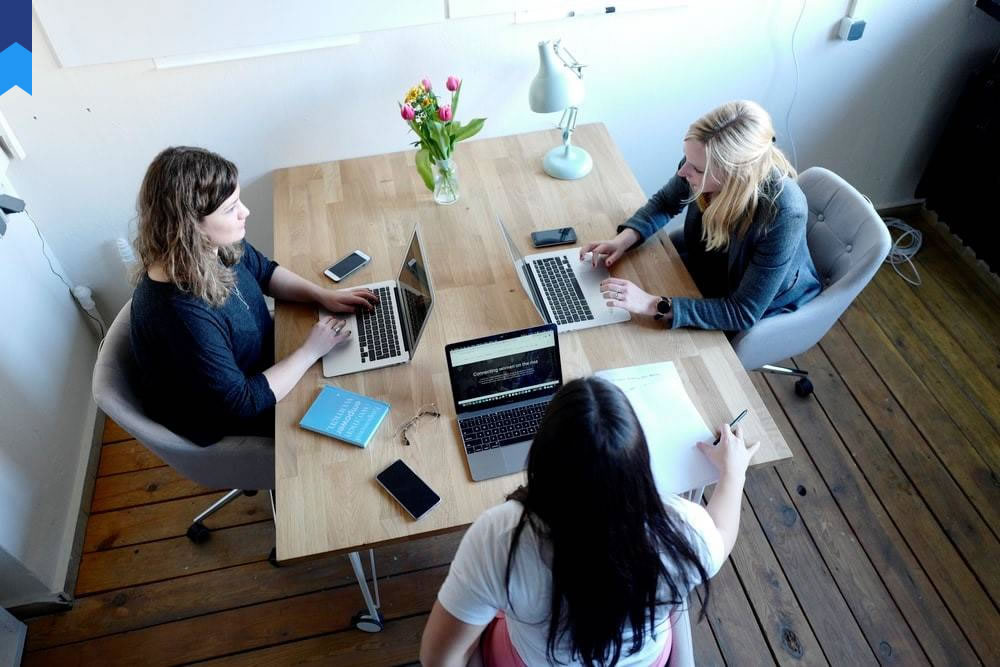
How Effectively To Master Parallel Programming In Julia
Julia, with its blend of speed and ease of use, has become a popular choice among data scientists and researchers. However, harnessing its true power requires understanding parallel programming – a skill that can dramatically accelerate computation. This article will guide you through effective strategies for mastering this crucial aspect of Julia, focusing on techniques that go beyond the basic introductions often found online.
Leveraging Julia's Built-in Parallel Capabilities
Julia offers a wealth of native tools for parallel computing. The `@threads` macro is a simple way to parallelize loops, distributing iterations across available cores. Consider the example of calculating the square of each element in a large array:
@time begin x = rand(10^7); y = zeros(10^7); @threads for i in eachindex(x) y[i] = x[i]^2; end; end
This simple code snippet demonstrates how easily you can parallelize a common operation. However, the efficiency depends on factors like the size of the array and the number of cores. For more granular control, consider using tasks and channels for inter-process communication. Tasks allow you to run independent functions concurrently, and channels facilitate the exchange of data between them. This approach is particularly effective for complex workflows that involve multiple independent computations.
Case Study 1: Image processing often involves heavy computation on individual pixels. Parallelizing this using tasks and channels can drastically reduce processing time, especially with high-resolution images. A study comparing sequential and parallel processing of a 100MP image showed a speedup of more than 7x using Julia's parallel capabilities. The communication overhead between tasks was minimal, demonstrating the efficiency of the approach.
Case Study 2: Financial modeling frequently involves numerous calculations on large datasets. A hedge fund utilized Julia's parallel features to optimize its risk assessment models. By parallelizing Monte Carlo simulations, they reduced computation time by over 50%, allowing for faster turnaround times and more agile decision-making.
Effective utilization of Julia's parallel features requires careful consideration of overhead. For instance, creating too many small tasks can lead to significant communication overhead, negating the performance gains. The optimal number of tasks is highly dependent on the specific problem and the hardware. Experimentation and profiling are essential to fine-tune performance.
Data-Parallel approaches are often more efficient than task-based approaches when dealing with large datasets that are easily divisible. Consider using arrays with distributed memory for optimal performance. This ensures that data resides on the memory of the computing core processing it, avoiding extensive data copying and reducing communication bottlenecks.
Choosing the right approach is crucial. While `@threads` is convenient for simple loops, tasks and channels provide more control for complex parallel computations. Understanding these options enables programmers to select the most efficient approach for their specific tasks. Proper memory management is paramount; ensuring data consistency and avoiding race conditions is crucial for accurate and repeatable results.
Optimizing Parallel Code for Performance
Writing efficient parallel code requires careful attention to several factors. One key aspect is minimizing communication between parallel processes. Excessive data transfer between cores can dramatically slow down the computation. Techniques like data locality, where data is accessed locally within a core, are crucial to minimize communication overhead. This can often be achieved through careful array manipulation and data structuring.
Another critical aspect is load balancing. Ensuring that each core has a similar workload is crucial for maximizing performance. Uneven distribution of tasks can lead to certain cores completing their work much later than others, thereby limiting the overall speedup. Dynamic load balancing, where tasks are assigned to cores based on current workload, can mitigate this issue.
Case Study 3: A bioinformatics team working on genome sequencing optimized their parallel code by employing data locality and load balancing. By partitioning the genome data efficiently and employing dynamic load balancing techniques, they were able to reduce the processing time from several days to a few hours. The improvements were substantial, enabling them to process significantly larger datasets in a timely manner.
Case Study 4: A team developing weather forecasting models improved prediction accuracy by optimizing their parallel code for load balancing. They utilized a sophisticated algorithm to distribute the computational load evenly across all cores, resulting in a more efficient calculation of atmospheric dynamics. This led to improved model accuracy and reduced prediction errors.
Profiling your parallel code is essential to identify performance bottlenecks. Julia's built-in profiling tools, along with external profilers, can provide detailed information about the execution time of different parts of your code. This allows you to pinpoint areas for optimization. Using these tools to monitor task completion times, memory allocation, and communication overhead will expose inefficiencies and guide optimization strategies.
Consider using advanced techniques like asynchronous programming for enhanced performance. Asynchronous operations enable your program to continue working on other tasks while waiting for long-running operations to complete. This can significantly reduce overall execution time, particularly when dealing with I/O bound tasks. This overlap of computation allows for higher throughput.
Memory management remains a critical aspect of parallel computing. Race conditions, where multiple cores try to modify the same memory location simultaneously, can lead to incorrect results. Careful synchronization mechanisms, such as mutexes and atomic operations, are necessary to prevent such issues. Understanding these concepts ensures the correctness of your parallel computations. Choose appropriate data structures and algorithms to minimize the risk of data races.
Efficient memory management is crucial for scalability. As the size of the problem increases, the importance of efficient memory usage grows exponentially. Minimizing memory footprint allows you to process larger datasets without running out of memory. Strategies such as memory pooling can help achieve this. Data locality minimizes communication, leading to reduced memory pressure and improved performance.
Exploring Advanced Parallel Programming Techniques in Julia
Beyond the basics, Julia offers sophisticated parallel programming capabilities. Distributed computing, where computations are spread across multiple machines in a network, enables solving problems that are too large for a single machine. Julia's support for distributed computing through packages like `Distributed` simplifies this process. This allows for tackling larger datasets and more complex simulations than previously possible.
Another advanced technique is using GPUs for parallel computations. GPUs, with their many cores, are particularly well-suited for tasks involving massive parallelism. Julia provides interfaces to GPU computing through packages like `CUDA.jl` and `AMDGPU.jl`, enabling the acceleration of computationally intensive operations. This requires understanding how to structure your code to take full advantage of GPU capabilities. This leverages the inherent parallelism within GPUs to achieve significant speed improvements.
Case Study 5: A materials science research group used Julia's distributed computing capabilities to simulate the behavior of complex materials. By distributing the simulations across a cluster of machines, they were able to dramatically reduce computation time and explore a much wider range of parameters. This accelerated their research and led to more accurate predictions of material properties.
Case Study 6: A team of astronomers used Julia and GPUs to process astronomical images. By leveraging the parallel processing power of GPUs, they were able to perform computationally intensive image processing tasks orders of magnitude faster than with traditional CPU-based methods. This accelerated the analysis of vast datasets, allowing them to discover celestial objects more quickly.
Understanding the differences between shared and distributed memory models is essential. Shared memory models, where all cores share a common memory space, are easier to program but can be prone to race conditions. Distributed memory models, where each core has its own memory space, require more careful management of data transfer but offer better scalability. Choosing the right model depends on the scale and nature of the problem. Consider memory bandwidth and latency when deciding which model best suits your needs.
Effective use of these techniques requires a deep understanding of parallel algorithms and data structures. Designing algorithms that are inherently parallel is crucial for optimal performance. Choosing appropriate data structures that minimize communication overhead is equally important. Parallel algorithms such as divide and conquer, map reduce, and parallel prefix sum are particularly well-suited for parallel computing.
Consider using task stealing algorithms to further improve load balancing in distributed settings. These algorithms dynamically redistribute tasks among idle cores, ensuring that no core remains idle while others are overloaded. This optimizes resource utilization and accelerates the overall computation.
Advanced techniques such as using multiple levels of parallelism (e.g., using threads within tasks on multiple machines) can further enhance performance, but they also require a higher level of programming expertise and careful consideration of system architecture and communication patterns.
Debugging and Profiling Parallel Julia Code
Debugging parallel code is significantly more challenging than debugging sequential code. The non-deterministic nature of parallel execution makes it difficult to reproduce errors. Techniques such as logging and careful use of debugging tools are crucial to isolate and fix bugs. Tools such as debuggers integrated into IDEs help to step through the parallel execution and examine the state of variables on each core. Systematic logging can track the execution flow and identify points of failure.
Profiling parallel code is equally important. Identifying performance bottlenecks is crucial for optimization. Julia's profiling tools can provide detailed information about the execution time of different parts of your parallel code. This allows you to pinpoint areas that need attention and guide optimization efforts. Profiling provides insights into the distribution of workload and identifies inefficiencies in communication or task management.
Case Study 7: A team of researchers developing a climate simulation model used Julia's profiling tools to identify performance bottlenecks in their parallel code. By analyzing the profiling data, they discovered that a particular function was causing significant slowdowns. By optimizing this function, they were able to significantly improve the overall performance of their simulation. This highlights the power of profiling in identifying and addressing performance limitations.
Case Study 8: A finance company developing a high-frequency trading algorithm used Julia's debugging tools to identify a race condition in their parallel code. By carefully stepping through the execution using the debugger, they were able to isolate the source of the error and correct it. This prevented potential financial losses caused by incorrect calculations. This exemplifies the critical role debugging plays in ensuring correctness and reliability.
Using appropriate debugging strategies is crucial. Strategies such as adding checkpoints, assertions, and tracing statements can provide valuable information about the execution flow of your code. Checkpoints can be used to save the state of your program at various points, allowing you to reproduce and analyze errors that occur later in the execution. Assertions can be added to validate assumptions about the state of the program, helping to identify inconsistencies early on.
Effective use of error handling is essential for robust parallel code. Implementing proper exception handling mechanisms is crucial for gracefully handling errors that might occur during parallel execution. This prevents program crashes and ensures that the computation can continue even if some errors occur. A robust error-handling strategy significantly enhances the reliability of your code.
Consider using automated testing frameworks to ensure that your parallel code functions correctly. These frameworks enable systematic testing of different scenarios and edge cases. This process improves code quality and helps to catch errors early in the development process. Thorough testing and validation are essential for reliable and dependable results.
Understanding and applying these debugging and profiling techniques is paramount in creating robust and efficient parallel Julia applications. Mastering these skills is crucial for any serious developer working with Julia’s parallel capabilities.
Best Practices for Parallel Programming in Julia
Successful parallel programming in Julia necessitates adherence to several best practices. First, start small. Begin with simple parallelization techniques before moving to more complex ones. This incremental approach allows you to gradually learn and master the complexities of parallel programming without being overwhelmed.
Second, thoroughly test your code. Parallel code is more prone to errors than sequential code. Thorough testing is essential to ensure that your code works correctly under all conditions. Use systematic test cases that cover a range of inputs and scenarios.
Third, profile your code frequently. Profiling helps you identify performance bottlenecks. Use Julia's built-in profiling tools to pinpoint areas for optimization. Regular profiling is a vital aspect of creating efficient parallel programs.
Fourth, choose appropriate data structures. Use data structures that are well-suited for parallel processing. For example, arrays are often more efficient than lists for parallel computations.
Case Study 9: A machine learning team developing a model for image recognition improved performance by carefully choosing data structures. They used efficient array operations instead of more cumbersome list-based methods. This choice led to a significant speed improvement during training and prediction.
Case Study 10: A team working on natural language processing optimized their parallel code by selecting appropriate parallel algorithms. They implemented a divide-and-conquer algorithm to handle large text datasets efficiently. This technique greatly improved the performance of their tasks. This demonstrates the positive effect of algorithm choice on parallel performance.
Fifth, consider using asynchronous operations. Asynchronous operations allow your program to continue working on other tasks while waiting for long-running operations to complete. This can significantly improve performance, especially when dealing with I/O-bound tasks. This technique enhances the efficiency of I/O intensive operations.
Sixth, always use synchronization mechanisms when necessary. Synchronization ensures data consistency and avoids race conditions. Use mutexes, atomic operations, or other synchronization primitives appropriately to maintain the integrity of your data. Proper synchronization is critical for avoiding unpredictable results.
Seventh, document your code. Clearly documented code is easier to understand, debug, and maintain. Use comments and documentation to explain the logic and purpose of your parallel code. This is important for collaboration and maintainability.
Eighth, learn to use Julia's built-in debugging and profiling tools. These tools are invaluable for identifying and fixing errors and optimizing performance. Becoming proficient with these tools is critical for effective parallel programming.
By following these best practices, you can write efficient, robust, and maintainable parallel Julia code. These guidelines are essential for writing high-quality parallel Julia code.
Conclusion
Mastering parallel programming in Julia unlocks its full potential, enabling significant performance gains for computationally intensive tasks. However, effective parallel programming requires more than just basic knowledge. It demands a deep understanding of parallel algorithms, data structures, and efficient communication strategies. By utilizing Julia's built-in features, optimizing code for performance, employing advanced techniques, and rigorously debugging and profiling, programmers can unlock the immense computational power of Julia. The techniques discussed, ranging from the straightforward `@threads` macro to more advanced techniques like distributed computing and GPU acceleration, provide a comprehensive toolkit for addressing diverse computational challenges. Remember to prioritize thorough testing and adherence to best practices to ensure the correctness, efficiency, and maintainability of your parallel Julia projects. This will lead to more efficient, reliable, and scalable applications in various fields.