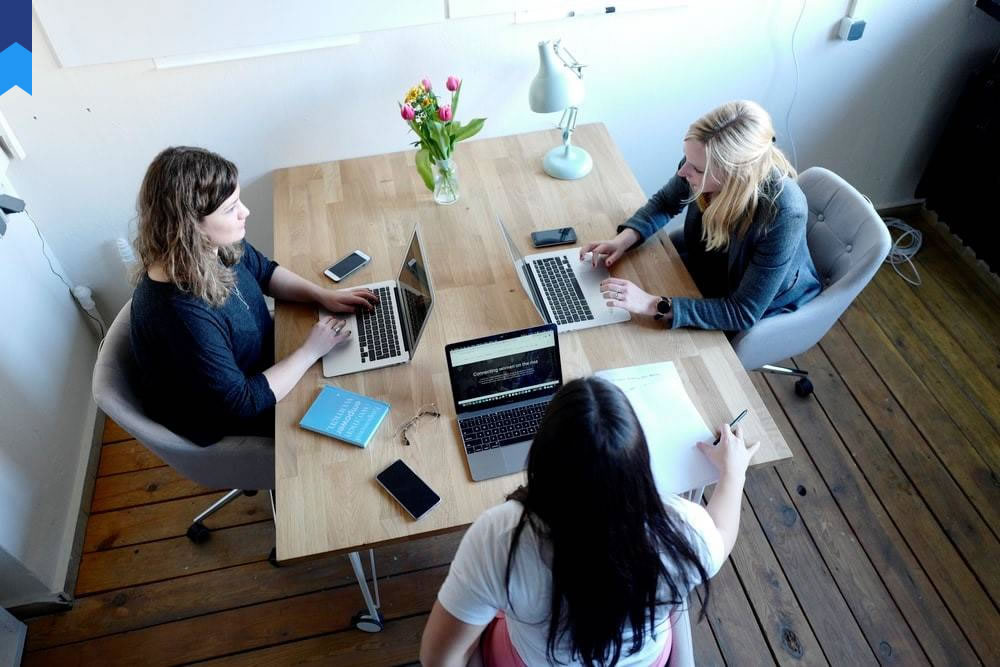
How To Create Excel Charts With Apache POI: A Comprehensive Guide
Introduction
Apache POI is a powerful Java library that allows developers to interact with various Microsoft Office file formats, including Excel spreadsheets. One of the key functionalities of Apache POI is its ability to create and manipulate charts within Excel documents. This capability opens doors to a world of data visualization opportunities, enabling developers to build dynamic and interactive charts within their Java applications.
This article serves as a comprehensive guide to navigating the intricacies of chart creation using Apache POI. We'll delve into the essential steps involved, exploring best practices and providing practical examples to illustrate the concepts. From basic bar charts to more complex pie charts and scatter plots, we'll equip you with the knowledge to generate compelling visualizations within your Excel documents.
Creating a Chart with Apache POI
The process of creating a chart using Apache POI involves a series of steps, each contributing to the final visualization. Let's break down these steps and provide illustrative examples:
1. **Setting up the Spreadsheet:** Begin by creating a new workbook and a sheet within it. This forms the foundation for your chart. Here's how to achieve this:
java Workbook workbook = new XSSFWorkbook(); // Create a new Excel workbook Sheet sheet = workbook.createSheet("ChartSheet"); // Create a sheet named "ChartSheet"
2. **Populating Data:** The next step involves filling your sheet with the data you want to visualize. This is achieved using rows and cells. Let's assume you want to create a simple bar chart representing sales data:
```java Row row1 = sheet.createRow(0); // Create the first row row1.createCell(0).setCellValue("Month"); // Set column header row1.createCell(1).setCellValue("Sales"); // Set column header
Row row2 = sheet.createRow(1); // Create the second row row2.createCell(0).setCellValue("January"); // Set data point row2.createCell(1).setCellValue(1000); // Set corresponding sales value
Row row3 = sheet.createRow(2); // Create the third row row3.createCell(0).setCellValue("February"); // Set data point row3.createCell(1).setCellValue(1200); // Set corresponding sales value ```
3. **Defining the Chart:** Now, you need to specify the type of chart you want to create. Apache POI supports various chart types, such as bar charts, line charts, pie charts, and scatter plots. Let's create a bar chart for our sales data:
java Chart chart = sheet.createDrawingPatriarch().createChart(new ClientAnchor(0, 0, 0, 0, 1, 1, 10, 20)); ChartLegend legend = chart.getOrCreateLegend(); legend.setPosition(LegendPosition.TOP_RIGHT);
4. **Setting the Data Source:** The next step involves linking your chart to the data you've populated in the sheet. This defines the data range that will be used for visualization:
```java // Create a category axis CategoryAxis categoryAxis = chart.getChartAxisFactory().createCategoryAxis(AxisPosition.BOTTOM); ValueAxis valueAxis = chart.getChartAxisFactory().createValueAxis(AxisPosition.LEFT); valueAxis.setCrosses(AxisCrosses.AUTO_ZERO);
// Define the data range AreaReference areaReference = new AreaReference(sheet.getSheetName(), new CellReference(1, 0), new CellReference(2, 1));
// Create a chart dataset ChartDataSource
// Create the chart series ChartSeries series = chart.createSeries(dataSource, new ChartData(categoryAxis, valueAxis));
// Set the chart type series.setSeriesType(ChartTypes.BAR);
// Add the chart series to the chart chart.getChartSeries().add(series); ```
5. **Formatting and Refinement:** The final step involves customizing your chart to enhance its visual appeal and readability. This includes formatting the chart title, axes, data labels, and overall appearance. Apache POI provides various methods for achieving this level of customization:
```java // Set chart title chart.setTitleText("Monthly Sales");
// Customize data labels series.setDataLabels(DataLabels.of().setIncludeCategory(true));
// Modify axis appearance categoryAxis.setTitle("Month"); valueAxis.setTitle("Sales"); ```
By following these steps, you can create a variety of charts using Apache POI. Remember to experiment with different chart types, data ranges, and formatting options to achieve the best visualization for your specific needs.
Case Study: Sales Performance Analysis with Apache POI
Imagine a business scenario where you need to analyze sales performance across different regions and products. Apache POI can play a vital role in this process. Here's a breakdown of how you can leverage Apache POI to create insightful charts from sales data:
1. **Data Preparation:** Start by importing sales data into an Excel spreadsheet. This data could include information such as region, product, sales amount, and date. You can use Java code to automate the import process if necessary.
2. **Creating Charts:** Use Apache POI to generate different charts to analyze the sales data. For example, you might create a bar chart to compare sales across regions, a line chart to track sales trends over time, or a pie chart to show the market share of different products.
3. **Chart Customization:** Customize the charts to make them visually appealing and informative. You can add titles, legends, data labels, and format the axes for clarity. Additionally, you can utilize color schemes and chart styles to enhance the presentation.
4. **Integration and Sharing:** Integrate the generated charts into your reporting system or share them with colleagues. You can embed the charts directly into your Java application or save them as separate Excel files for distribution. This allows for a dynamic and easily accessible representation of sales data.
Real-World Applications of Apache POI for Chart Creation
The application of Apache POI extends beyond mere data visualization; it plays a crucial role in streamlining data analysis workflows and enhancing reporting processes. Let's delve into several real-world examples:
1. **Financial Reporting:** In finance, Apache POI is used to automate the creation of financial reports with dynamic charts. For instance, a financial analyst can utilize Apache POI to generate charts showcasing key performance indicators (KPIs) such as revenue growth, profit margins, and return on investment (ROI). These charts provide clear visual insights into the financial health of a company, aiding in informed decision-making.
2. **Sales and Marketing:** Sales and marketing departments often rely on Apache POI to visualize sales trends, customer demographics, and campaign performance. By generating charts using Apache POI, these departments can identify patterns, analyze customer behavior, and optimize marketing strategies to drive sales growth.
3. **Research and Development:** Research and development teams can leverage Apache POI to create scientific visualizations, illustrating experimental data, research findings, and statistical analyses. This enhances the presentation of research results and facilitates the communication of complex findings to stakeholders.
Industry Trends and Future Implications
The use of data visualization tools like Apache POI continues to grow, driven by the increasing volume of data generated by businesses and organizations. Here are some key trends shaping the landscape:
1. **Rise of Big Data and Data Analytics:** The explosion of data requires effective visualization tools to extract insights and make data-driven decisions. Apache POI, with its ability to handle large datasets and generate complex charts, is well-positioned to meet this growing need.
2. **Focus on Interactive Visualizations:** Users are increasingly demanding interactive visualizations that allow for exploration and analysis. Apache POI, while primarily focused on static chart generation, can be integrated with frameworks like JavaFX or Swing to create more interactive and dynamic charts.
3. **Cloud-based Solutions:** Cloud-based solutions are gaining popularity, offering scalability and accessibility. Apache POI, being a Java library, can be readily integrated into cloud environments, enabling developers to create and share visualizations from anywhere.
Conclusion
Apache POI provides a robust and flexible solution for generating charts within Excel documents. By mastering its capabilities, developers can create compelling visualizations that effectively convey data insights and enhance decision-making processes. As the demand for data visualization continues to rise, Apache POI remains a valuable tool for transforming raw data into actionable insights.