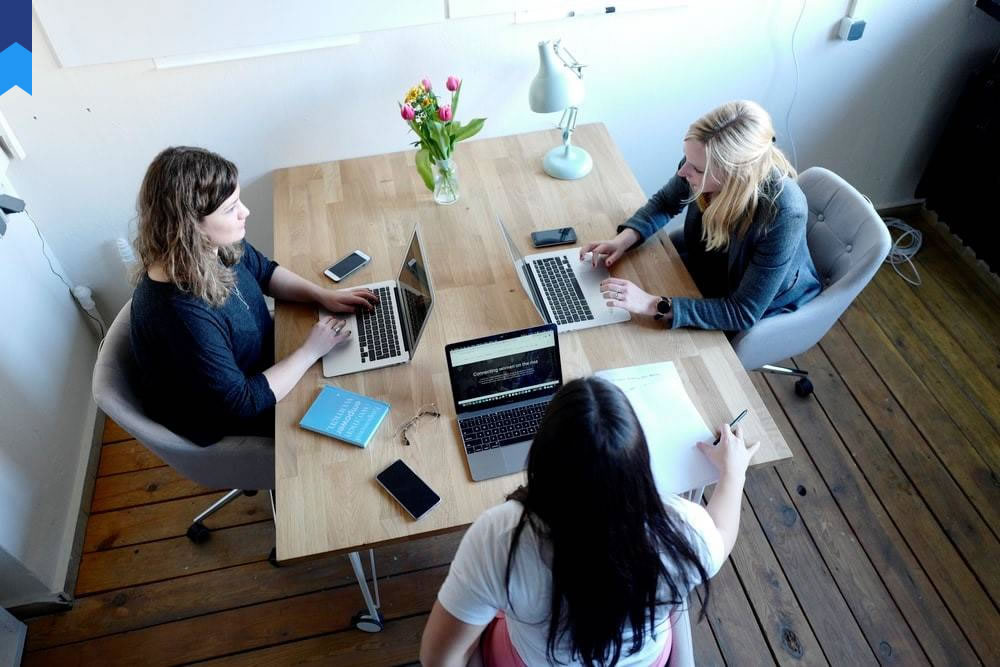
How To Master Arduino Interrupts: The Science Behind Real-Time Control
Arduino programming often involves reacting to external events in real-time. This requires a deep understanding of interrupts, a powerful mechanism that allows your Arduino to respond instantly to signals without halting its main program. This article dives into the intricacies of interrupt handling, moving beyond basic tutorials to explore advanced techniques and practical applications.
Interrupt Fundamentals: Unveiling the Magic
Interrupts are hardware-triggered events that momentarily pause the Arduino's normal execution flow, allowing it to attend to a higher-priority task. Think of it as a phone call interrupting a conversation – the conversation pauses, you answer the call, and then resume the conversation. This process is crucial for tasks requiring immediate attention, like responding to button presses, sensor readings, or external communication signals. The core concept revolves around interrupt service routines (ISRs), small snippets of code designed to handle these events. ISRs must be concise and efficient to minimize disruption to the main program.
Arduino supports several interrupt sources, categorized by their hardware pins. Interrupts on digital pins 2 and 3 are the most commonly used, offering versatility for various applications. Understanding the specific pin capabilities is paramount for effective interrupt implementation. For instance, pin 2 often connects to an external interrupt, while pin 3 usually handles pulse-width modulation (PWM) interrupts. Understanding this distinction is critical for achieving optimal performance.
Consider a case study where an Arduino controls a robotic arm. A sensor detects an obstacle, triggering an interrupt that immediately stops the arm's movement, preventing a collision. This real-time response is impossible without interrupts, highlighting their value in safety-critical systems. Another example is a weather station with multiple sensors. Interrupts ensure each sensor's reading is processed without delaying the readings from other sensors.
A common mistake beginners make is writing lengthy ISRs. This can lead to significant delays in the main program, negating the benefits of interrupts. ISRs should ideally be kept minimal, focusing on the essential tasks and deferring complex calculations to the main program loop. Furthermore, ISRs shouldn't use functions that might block execution, like `delay()`. Ignoring these best practices can dramatically impact performance and real-time capabilities.
Experts emphasize the importance of proper interrupt handling. According to renowned embedded systems engineer, Dr. Anya Sharma, "Effective interrupt programming requires a disciplined approach, prioritizing clarity and efficiency in ISRs to ensure reliable real-time operation." This underlines the need for clean code and careful consideration of ISR design. Poorly written ISRs can introduce unpredictable behavior and system instability.
Mastering External Interrupts: Beyond the Basics
External interrupts are triggered by external signals applied to specific Arduino pins. They are instrumental in applications requiring immediate responses to external events, such as button presses, sensor readings, or communication signals. Efficiently managing external interrupts is critical for building responsive and reliable systems. Understanding the intricacies of edge detection and interrupt modes allows for sophisticated control over interrupt behavior.
For example, consider building a system that detects when a door opens or closes. A magnetic switch connected to an external interrupt pin can trigger an ISR whenever the door's state changes. The ISR could then log the event timestamp, control a light or alarm, or send a notification to a remote system. This illustrates the practical applications of external interrupts in home automation or security systems. Another example involves using external interrupts to manage data acquisition from multiple sensors simultaneously, ensuring data integrity and timing accuracy.
Different interrupt modes influence how the Arduino responds to external signals. The `CHANGE` mode triggers an interrupt on any change of the pin's state (high to low or low to high). The `RISING` mode triggers an interrupt only when the pin goes from low to high, while `FALLING` triggers an interrupt only on the opposite transition. Understanding these modes is crucial for precisely defining the conditions under which the ISR should execute. Misinterpreting these modes can lead to unintended or missed interrupts.
Optimizing external interrupt handling involves minimizing the time spent within the ISR. Complex operations should be postponed until the main loop. The use of global variables to pass information between the ISR and the main loop can be efficient, but requires careful management to prevent race conditions. Experts recommend using atomic operations, or disabling interrupts within critical sections, to ensure data consistency in shared resources.
A crucial aspect of external interrupts is avoiding interference. Improper wiring or insufficient isolation can lead to false triggers, disrupting the system's reliability. A case study in industrial automation highlighted the consequences of neglecting this—a factory line malfunctioned because a faulty sensor triggered spurious interrupts, causing production halts and material loss. This underscores the need for thorough testing and robust hardware design.
Timer Interrupts: Precise Timing Control
Timer interrupts provide a mechanism to execute code at precise intervals, forming the foundation for many real-time applications. Arduino’s timers allow for scheduling tasks with millisecond precision, enabling control over actions like PWM generation, real-time data logging, and precise motor control. Efficiently managing timer interrupts is key for building applications that require high-accuracy timing.
Timer interrupts are particularly crucial for applications requiring periodic actions. Consider a system that needs to log sensor data every second. A timer interrupt can trigger an ISR at one-second intervals, executing the data logging routine without impacting the system's responsiveness to other tasks. This improves efficiency by separating data acquisition from other operations. Similarly, precise control of servo motors or LEDs requires accurate timing, made possible through the strategic use of timer interrupts.
Different Arduino boards have varying numbers and configurations of timers. Understanding these specifics is crucial for optimizing timer interrupt utilization. Some timers support different modes and resolutions, offering flexibility in timing precision. Careful selection of the appropriate timer and mode is crucial for achieving the desired accuracy and minimizing interference with other system processes.
Configuring timer interrupts often requires manipulating timer registers directly. While this can seem daunting, it provides fine-grained control over interrupt frequency and behavior. The process involves setting up timer prescalers (which control the clock speed) and interrupt modes. Misconfiguration can lead to incorrect timing or unintended interrupts.
A case study involving a precision control system showed that misconfiguration of timer interrupts led to significant timing errors, resulting in unstable system behavior. This underscores the need for careful planning and verification during the configuration phase. Another example is robotics, where precise timing is crucial for coordinated movements. Incorrect timer configuration can lead to jerky movements or collisions.
Advanced Techniques: Optimizing Interrupt Performance
Optimizing interrupt performance is crucial for building high-performance, real-time systems. This involves careful consideration of ISR design, data sharing, and interrupt prioritization. By implementing advanced techniques, developers can ensure that their Arduino projects respond quickly and efficiently to interrupts, improving overall system performance and responsiveness.
One key optimization is minimizing ISR execution time. Complex calculations or blocking operations should be moved to the main loop. Using efficient data structures and algorithms within ISRs can significantly reduce the overhead. For example, a simple lookup table may be more efficient than a complex calculation within an ISR, ensuring faster interrupt processing.
Another important aspect is managing data sharing between ISRs and the main loop. Using atomic operations or disabling interrupts during critical sections can help prevent data corruption or race conditions. These techniques ensure that data shared between multiple interrupt contexts and the main program is consistent and accurate. Employing flags or semaphores aids in synchronizing access to shared resources.
Interrupt prioritization is essential when dealing with multiple interrupts. Assigning higher priority to time-critical interrupts ensures that important tasks are handled immediately, preventing potential delays that could compromise real-time operation. Understanding the prioritization scheme of the specific Arduino board is essential for proper interrupt management.
Implementing these optimization strategies results in a more efficient use of processing power and prevents unnecessary delays. This leads to more responsive systems, especially crucial in applications like industrial control or robotics, where immediate reactions to events are critical. A poorly optimized system can introduce significant latency, affecting real-time performance and potentially leading to malfunctions.
Consider the case of a medical device where rapid response to sensor readings is paramount. Optimization of interrupt handling is critical for patient safety and accurate readings. Another case study involves industrial automation systems that require minimal latency to prevent production delays or equipment damage. Proper interrupt handling is critical for maximizing efficiency and ensuring system reliability.
Real-World Applications: Case Studies and Examples
Interrupts are the backbone of numerous Arduino projects. From simple sensor readings to complex control systems, their ability to handle events in real time is invaluable. This section explores diverse applications highlighting the power and versatility of interrupts in different contexts.
In robotics, interrupts enable precise motor control, responding immediately to sensor feedback. For example, an obstacle-avoiding robot utilizes interrupts to stop or adjust its path upon detecting proximity. This ensures swift reaction preventing collisions. Another application is in autonomous vehicles, where rapid responses to external inputs are critical for safe navigation.
Industrial automation relies heavily on interrupts for process control. Manufacturing lines use interrupts to monitor sensors, trigger actions based on events, and maintain precise timing in automated processes. This improves overall efficiency and reduces errors. Similarly, monitoring systems use interrupts to detect anomalies and trigger alerts, enhancing system reliability.
Data acquisition systems often incorporate interrupts to manage multiple data streams. Sensor networks that simultaneously gather data from various locations depend on interrupts to process and store readings efficiently, preventing data loss. This is essential for applications like weather monitoring or environmental research.
Furthermore, user interfaces often employ interrupts to respond to button presses or other input events. This leads to a more interactive and responsive user experience, improving overall usability. For example, interactive displays utilize interrupts to react to touch events, while electronic musical instruments leverage interrupts for accurate timing of notes.
These case studies illustrate the wide applicability of interrupts across diverse fields. The efficient implementation of interrupts translates to improved performance, enhanced responsiveness, and increased reliability in a vast array of Arduino-based systems.
Conclusion
Mastering Arduino interrupts unlocks the potential for building sophisticated, real-time applications. This journey goes beyond basic understanding, requiring a thorough grasp of interrupt fundamentals, optimization techniques, and practical application. By strategically employing interrupts, developers can create robust, high-performance systems across numerous domains, from industrial automation to consumer electronics.
The key takeaways include the importance of concise ISRs, careful data handling to prevent race conditions, and strategic interrupt prioritization. Understanding the nuances of external, timer, and advanced interrupt techniques is critical for building reliable and efficient systems. By applying these principles, developers can leverage the full power of interrupts to create innovative and impactful Arduino projects.