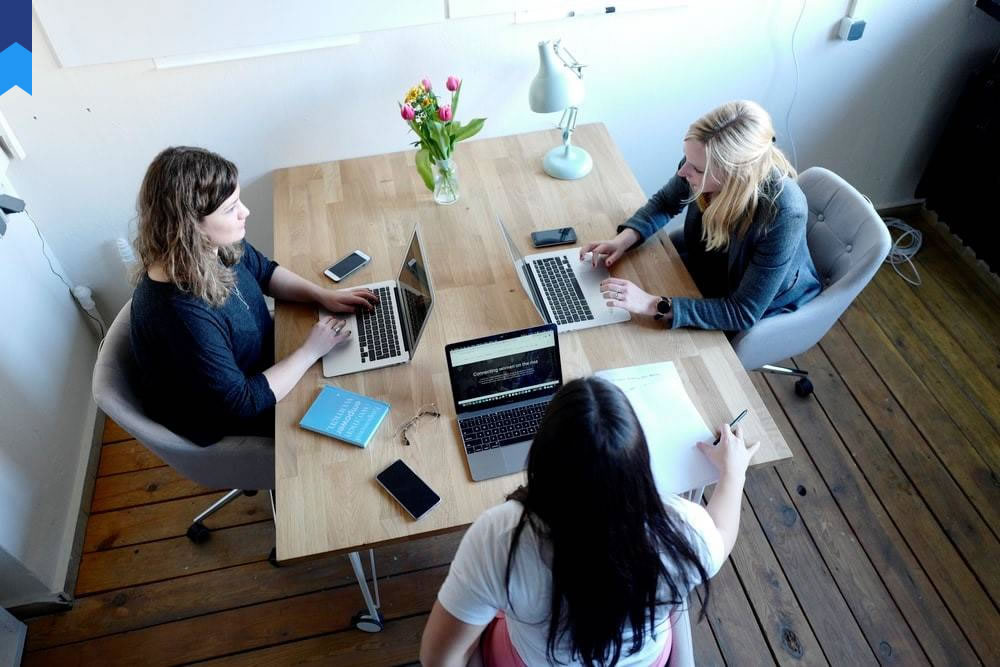
Inside The World Of Julia's Advanced Metaprogramming
Julia, a high-performance, dynamically-typed language, offers a powerful metaprogramming system allowing programmers to manipulate code as data. This goes beyond simple macros; it delves into generating code at runtime, altering compiler behavior, and even creating domain-specific languages (DSLs) within Julia itself. This article explores the depths of Julia's metaprogramming capabilities, moving past basic introductions to uncover its advanced techniques and their practical applications.
Unveiling Julia's Macro System: Beyond the Basics
Julia's macro system, built upon Lisp-inspired hygienic macros, allows for sophisticated code manipulation. Unlike simple text substitution, hygienic macros prevent accidental name clashes, ensuring predictable and maintainable code. Consider the common task of creating verbose logging statements. A macro can simplify this. Instead of writing multiple lines for logging, you can create a macro that handles the formatting and output. For instance, a `@log` macro could automatically timestamp and format messages, streamlining debugging. This is a fundamental application, but the power extends much further. Case study 1: Imagine a scenario where you need to profile specific code sections. A macro can automatically instrument the code with timing functions, reducing boilerplate and enhancing performance analysis. Case study 2: A common problem is the repetitive nature of writing getters and setters for structs. A macro can automate this process, significantly reducing development time and code size. The core principle is to abstract repetitive tasks into reusable macro definitions. Further enhancement involves leveraging Julia’s powerful type system to create macros that operate on specific types or generate type-specific code, adding a layer of safety and optimization.
Generating Code at Runtime: Dynamic Code Construction
Julia's ability to generate code dynamically at runtime significantly expands its metaprogramming capabilities. This feature shines when dealing with situations where the exact code needed is only known at runtime, such as code generation based on user input or complex data structures. For instance, consider a scenario where you need to generate SQL queries based on user-defined filters. Rather than writing numerous if-else statements, you could generate the SQL query dynamically. Case study 1: A machine learning library could use this to optimize neural network architecture based on performance metrics obtained during training. The library could dynamically generate optimized code for the specific network topology. Case study 2: A complex simulation model might require different equations depending on the state of the system. Julia's dynamic code generation allows constructing the appropriate equations based on runtime conditions. This adaptability drastically reduces the development time and complexity of handling varied scenarios. This technique also extends to code optimization. By analyzing the runtime environment, Julia can generate optimized code tailored to the specific hardware and data. This capability allows for highly efficient programs tailored for specific hardware. The creation of DSLs (Domain-Specific Languages) within Julia itself often relies heavily on dynamic code generation, furthering the language's power and extensibility.
Manipulating the Compiler: Advanced Compiler Macros
Julia provides tools to directly interact with its compiler, allowing for fine-grained control over code compilation. This advanced level of metaprogramming enables optimization strategies beyond what's possible with standard macros. These compiler macros can perform operations such as inlining functions, optimizing data structures, or even modifying the compiler's intermediate representation (IR). Case study 1: A numerical computation library could utilize compiler macros to vectorize loops, enhancing performance on multi-core processors. This would automatically parallelize code without requiring explicit threading. Case study 2: A specialized data structure might require custom memory management. A compiler macro could optimize memory allocation and deallocation for this custom data structure, resulting in significant memory savings. Using compiler macros demands a deep understanding of Julia's internals and compiler architecture, but the rewards are immense. These techniques allow for pushing Julia’s performance boundaries beyond what standard techniques can achieve. However, careful design is paramount. Improper use can lead to unexpected compiler behavior or even code instability. This requires rigorous testing and validation.
Creating Domain-Specific Languages (DSLs) in Julia
One powerful application of Julia's metaprogramming capabilities is the creation of domain-specific languages (DSLs). DSLs are designed for specific tasks or domains, providing a more intuitive and concise syntax than general-purpose languages. By leveraging Julia's macro system and dynamic code generation, it's possible to embed custom DSLs within Julia, extending the language's functionality to accommodate specific needs. Case study 1: A DSL could be created for describing chemical reactions, allowing chemists to write reactions in a more natural notation, which is then translated by Julia into code for simulation or analysis. This allows the integration of specialized domain knowledge into a familiar programming environment. Case study 2: A financial modeling DSL can be created to represent complex financial instruments and calculations within Julia. This aids in developing highly specialized financial modeling applications. Creating a DSL involves designing a syntax, implementing the parser (often using Julia’s powerful parsing capabilities), and generating the corresponding Julia code. This process requires careful planning and a robust testing strategy.
Advanced Techniques and Best Practices
Beyond the fundamental aspects, several advanced metaprogramming techniques are crucial for building robust and maintainable code. These include techniques like using `Expr` for constructing arbitrary expressions, leveraging the `@eval` macro for dynamic code evaluation, and effectively using quotations for manipulating code as data structures. Furthermore, careful consideration of hygiene and avoiding unintended side effects is critical. Well-structured metaprograms often employ modular design principles, breaking down complex operations into smaller, more manageable components. This enhances code readability and maintainability, enabling collaborative development. Effective testing strategies are crucial. Thoroughly testing metaprograms requires more than just unit tests; it includes testing edge cases, unexpected inputs, and interactions with the compiler. Using tools and techniques for profiling and analyzing the performance of metaprograms helps identify bottlenecks and optimize code for maximum efficiency. The advanced techniques allow for greater control and flexibility but also demand a deeper understanding of Julia's underlying mechanisms.
Conclusion
Julia's metaprogramming capabilities extend far beyond basic macros. By mastering the techniques of dynamic code generation, compiler manipulation, and DSL creation, developers unlock a potent toolset for building highly efficient, specialized, and maintainable software. While the initial learning curve might be steep, the long-term benefits—in terms of code reusability, performance optimization, and the creation of specialized DSLs—make the investment worthwhile. Understanding these advanced techniques empowers Julia programmers to explore new frontiers in code manipulation and software development, pushing the boundaries of what's possible with this dynamic language. The ability to seamlessly integrate domain-specific knowledge, optimizing code for specific hardware, and creating novel problem-solving approaches makes Julia's metaprogramming a powerful asset in diverse fields.