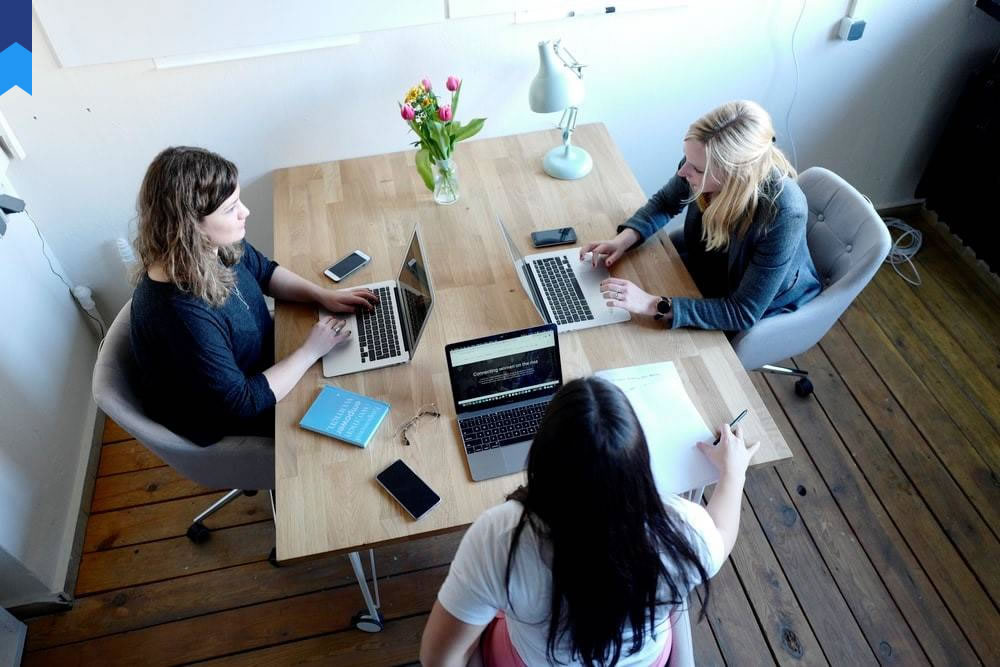
Inside The World Of Kotlin Coroutines: Mastering Asynchronous Programming
Kotlin coroutines have revolutionized asynchronous programming, offering a powerful and elegant solution for handling concurrent tasks. This article delves into the intricacies of coroutines, moving beyond basic introductions to explore advanced techniques and practical applications that will significantly enhance your Kotlin development skills.
Understanding the Fundamentals of Kotlin Coroutines
Coroutines provide a lightweight concurrency model that allows you to write asynchronous code in a sequential manner. Unlike threads, which are heavyweight and resource-intensive, coroutines are managed by a single thread, minimizing overhead and improving efficiency. This is particularly beneficial for I/O-bound operations, such as network requests or database queries. The key components include launch
, which starts a new coroutine, and async
, which returns a Deferred
value, allowing you to await the result. For example, launch { println("Hello from a coroutine!") }
will execute the code block concurrently. Consider a scenario involving multiple network calls. With coroutines, you can launch each call concurrently using async
, then collect the results using awaitAll
. This significantly reduces overall execution time compared to sequential execution. A common mistake is forgetting to handle exceptions within coroutines. Proper exception handling is crucial to ensure the robustness of your applications. Using try-catch
blocks within coroutine scopes is essential.
Case Study 1: A web application uses coroutines to handle multiple user requests concurrently, ensuring responsiveness and scalability. By using coroutines, the application can handle a larger number of concurrent requests without compromising performance.
Case Study 2: A mobile application uses coroutines to fetch data from a remote server. This allows the UI to remain responsive while the data is being fetched. The use of coroutines in this case is crucial for maintaining the user experience.
Furthermore, suspending functions, a core element of coroutines, allow you to pause the execution of a coroutine without blocking the thread. This enables writing asynchronous code that looks and behaves like synchronous code. Properly structured suspending functions make the asynchronous code easier to reason about and debug. Incorrectly structured suspending functions can lead to performance degradation and unhandled exceptions.
Effective use of coroutines necessitates an understanding of coroutine scopes. Scopes control the lifecycle of coroutines, allowing you to manage their creation and cancellation. Incorrect scope management can result in resource leaks and unexpected behavior. It's recommended to use structured concurrency whenever possible, as this approach simplifies error handling and resource management.
Advanced Coroutine Techniques: Flow and Channels
Kotlin Flows build upon coroutines, providing a powerful mechanism for handling streams of data asynchronously. Flows are cold streams, meaning they only start emitting values when a collector subscribes. They are ideal for handling events, data streams, and asynchronous operations that generate multiple results. For example, a flow can be used to continuously monitor sensor data, reacting to changes as they occur. A typical flow operation might involve transforming the data stream, filtering elements, and collecting the results. Misunderstanding flow operators, such as map
, filter
, and reduce
can lead to unexpected behavior or performance problems. Understanding backpressure is crucial when working with flows; managing backpressure correctly ensures that consumers are not overwhelmed by the producer.
Case Study 1: A real-time data visualization application uses flows to process incoming data streams and update the UI in real time.
Case Study 2: A financial trading application uses flows to handle price updates from a market data feed, efficiently processing and displaying price changes.
Channels provide a way for coroutines to communicate by sending and receiving values. They offer a more robust solution than shared mutable variables for inter-coroutine communication, preventing race conditions and ensuring thread safety. Channels offer various modes of operation, including buffered and unbuffered, which impacts the behavior of send and receive operations. Choosing the wrong channel type can impact performance and concurrency, so understanding the characteristics of each type is vital. For instance, an unbuffered channel ensures that senders wait for receivers, creating a synchronization point, while a buffered channel allows for asynchronous communication.
Consider a scenario where multiple coroutines need to process tasks concurrently and share the results. Using channels, each coroutine can send its results to the channel, and another coroutine can read and process the results from the channel. This approach provides a clear separation of concerns and improves code readability and maintainability. Improper use of channels can lead to deadlocks, where coroutines wait indefinitely for each other. It is important to carefully manage channel capacity and communication patterns to avoid such scenarios.
Error Handling and Concurrency Control in Coroutines
Robust error handling is essential in any application, and coroutines are no exception. try-catch
blocks are used within coroutines to handle exceptions, but the nature of asynchronous operations requires careful consideration. Using CoroutineExceptionHandler
allows for global exception handling across multiple coroutines. This enables centralized error logging and recovery strategies. Failing to handle exceptions appropriately can lead to application crashes or data inconsistencies. The choice between local and global exception handling depends on the context and the desired level of control over error management.
Case Study 1: A banking application utilizes CoroutineExceptionHandler
to catch and log exceptions during transaction processing, ensuring data integrity and preventing failures from cascading. This proactive approach ensures resilience and data consistency.
Case Study 2: An e-commerce application employs local exception handling within individual coroutines, enabling specific error responses for different operations, thereby improving the user experience.
Concurrency control becomes critical when multiple coroutines access shared resources. Kotlin offers various synchronization mechanisms, including mutexes and atomic operations, to ensure thread safety. Incorrect usage of these mechanisms can lead to race conditions and unpredictable behavior. Understanding the trade-offs between different concurrency control techniques is vital to optimize performance and maintain data integrity. A common pitfall is the overuse of synchronization primitives, which can introduce bottlenecks and reduce concurrency.
Consider a scenario where multiple coroutines update a shared database. Without proper concurrency control, race conditions can occur, leading to data corruption or inconsistencies. Using a mutex to protect access to the database ensures that only one coroutine can access it at a time, thus maintaining data integrity. In contrast, using atomic operations is more efficient for simpler update scenarios, offering a performance advantage over mutexes. For more complex scenarios, a combination of different concurrency control mechanisms might be necessary. Each mechanism has strengths and weaknesses related to its performance and complexity.
Testing Coroutines: Strategies and Best Practices
Testing asynchronous code presents unique challenges. Standard testing frameworks may not be directly suitable for testing coroutines due to their asynchronous nature. Kotlin provides tools such as runBlockingTest
and TestCoroutineDispatcher
that facilitate testing coroutines. These tools allow you to control the execution of coroutines within a test environment, enabling more predictable and reliable tests. Without appropriate testing techniques, the asynchronous nature of coroutines can make it hard to reproduce issues. Thorough testing is crucial to ensure code reliability and identify potential concurrency issues.
Case Study 1: A team uses runBlockingTest
to test a coroutine that performs a network request, verifying the correct data is returned and that exceptions are handled appropriately.
Case Study 2: A developer utilizes TestCoroutineDispatcher
to simulate various scenarios, such as network delays or failures, to ensure the resilience and reliability of a coroutine-based application under various conditions.
Effective testing involves creating unit tests for individual coroutines, ensuring that they function correctly in isolation. Integration tests are then used to verify the interaction between multiple coroutines and shared resources. This layered testing approach helps to identify both isolated issues and integration problems. Ignoring test coverage can lead to critical failures when running in a real-world environment, therefore comprehensive testing is recommended.
Various strategies exist for testing coroutines. Techniques like mocking network responses or using in-memory databases can facilitate testing without relying on external services or databases. This makes tests faster and more reliable. These practices ensure the isolation of individual coroutines and components, facilitating more accurate and focused testing. Overlooking the importance of isolation can lead to unexpected test results, making it hard to detect and solve issues. These testing strategies are integral to building robust and reliable coroutine applications.
Practical Applications and Future Trends of Kotlin Coroutines
Kotlin coroutines find extensive use in various domains. In Android development, coroutines are crucial for handling long-running operations on the main thread without blocking the UI. This prevents application freezes and ensures a responsive user experience. The adoption of coroutines in Android development is rapidly increasing due to their efficiency and ease of use. Many Android libraries now embrace coroutines, further accelerating their adoption. The simplicity and efficiency of coroutines have led to their widespread use in Android development, significantly improving application performance and responsiveness.
Case Study 1: A popular Android application utilizes coroutines to fetch and display data from a remote API, ensuring a smooth user experience even with slow network connections.
Case Study 2: An Android game utilizes coroutines for game logic and update cycles, ensuring smooth game performance and responsiveness, even with complex game mechanics.
Beyond Android, coroutines find applications in server-side development, enabling efficient handling of concurrent requests in web applications and microservices. This helps to improve the scalability and responsiveness of these systems. The asynchronous nature of coroutines allows for handling numerous concurrent requests without exceeding system resources. This is especially important in high-traffic environments. The growing adoption of Kotlin as a backend language is further fueling the adoption of coroutines in server-side development.
Future trends suggest an increasing reliance on coroutines for complex asynchronous operations. Improved tooling and library support will likely make coroutines even more accessible and easier to use. New features and optimizations could enhance the efficiency and performance of coroutines even further, driving their widespread adoption in various programming contexts. Asynchronous programming is becoming increasingly important, making coroutines an even more critical tool for developers. This is particularly true given the rise of highly concurrent applications and the demand for efficient resource utilization.
Conclusion
Kotlin coroutines represent a significant advancement in asynchronous programming, offering a more elegant and efficient way to handle concurrency. Mastering coroutines, including their advanced features like flows and channels, is crucial for any Kotlin developer seeking to build robust, scalable, and high-performance applications. By understanding the nuances of error handling, concurrency control, and effective testing strategies, developers can leverage the full power of coroutines and create sophisticated applications that deliver optimal performance and a seamless user experience. Continuous learning and adoption of best practices are key to keeping pace with the evolving landscape of asynchronous programming and realizing the full potential of Kotlin coroutines.