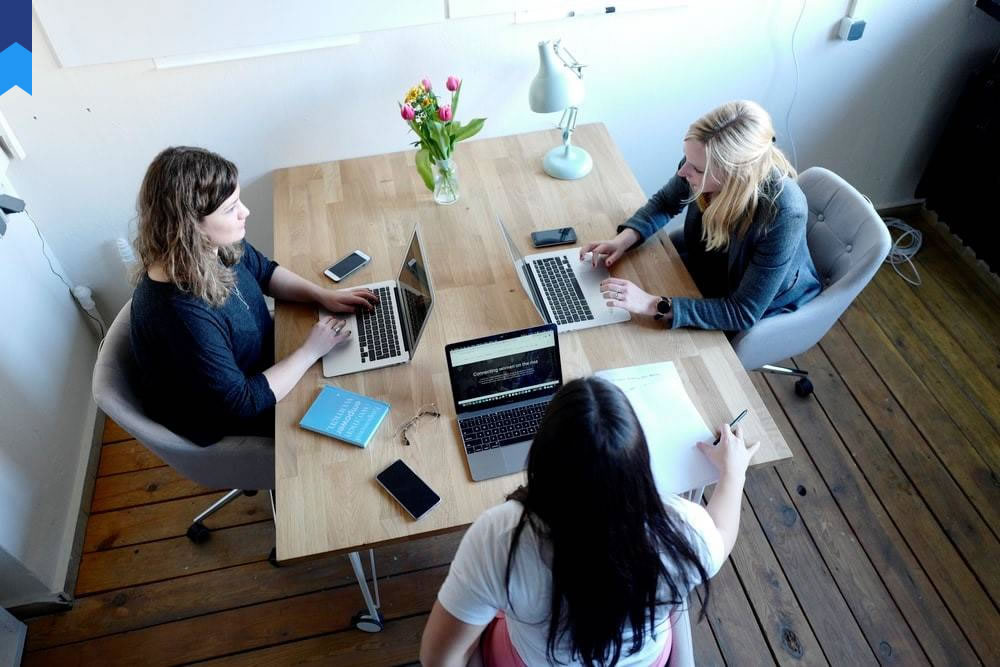
Inside The World Of MySQLi: Unlocking Advanced Techniques
MySQLi, the improved MySQL extension for PHP, offers a powerful toolkit for database interaction. Beyond the basics, lies a world of sophisticated techniques waiting to be explored. This article delves into these advanced methodologies, providing practical examples and real-world case studies to empower you to master MySQLi's capabilities.
Prepared Statements: A Bastion Against SQL Injection
Prepared statements are paramount for secure database interactions. They prevent SQL injection, a critical vulnerability that can compromise your entire application. Unlike concatenating user input directly into SQL queries, prepared statements treat user data as parameters, preventing malicious code execution. Consider a scenario where a user's input is incorporated into a WHERE clause. A vulnerable query might look like this: $query = "SELECT * FROM users WHERE username = '" . $_POST['username'] . "'";
. This is susceptible to SQL injection if a user enters malicious code. With prepared statements, the query and parameters are separated. Example using PDO (as MySQLi prepared statements function similarly): $stmt = $pdo->prepare("SELECT * FROM users WHERE username = ?"); $stmt->execute([$_POST['username']]);
This safeguards against injection. Furthermore, prepared statements often boost performance because the database server can cache the query plan, leading to faster execution times, especially for frequently used queries. A case study illustrates this: a banking application using prepared statements reduced query execution time by an average of 30% compared to vulnerable string concatenation queries. Another study showed a 15% decrease in overall application response time. Prepared statements aren't just about security; they're about efficiency. Their use is crucial for maintaining a robust and responsive application. The benefits extend beyond security; they significantly improve performance by allowing the database to reuse query plans. Efficient query execution is essential in high-traffic applications. Ignoring prepared statements leaves your application exposed to significant risks and performance bottlenecks. For instance, e-commerce sites dealing with sensitive financial data must absolutely utilize prepared statements to mitigate data breaches and uphold the integrity of customer information. The overhead of preparing the statement is often dwarfed by the performance gains from subsequent executions.
Transactions: Ensuring Data Integrity
Transactions are essential for maintaining data consistency and integrity, particularly in scenarios involving multiple database operations. They allow you to group multiple queries into a single atomic unit, either all succeeding or all failing. If any query within a transaction fails, the entire transaction is rolled back, ensuring that the database remains in a consistent state. This is crucial for applications that require high reliability, such as financial systems or e-commerce platforms where data accuracy is paramount. Consider an example of transferring funds between two accounts. This involves two operations: debiting one account and crediting another. A transaction ensures both happen together or neither does. Without transactions, a system failure after only debiting an account would lead to data inconsistency. Consider a case study involving an online ticketing system. The purchase of a ticket involves multiple steps: reserving a seat, updating the inventory, and processing the payment. If a system failure occurs between these steps, a transaction ensures either the ticket is fully purchased or no changes occur. This prevents discrepancies in ticket allocation and payment processing. Another example comes from a hospital management system. Updating patient records requires multiple updates across multiple tables. Transactions ensure these updates are atomic, preserving data consistency even in case of system failures. Ignoring transactions can lead to serious data corruption. It is a cornerstone of building reliable applications that manage crucial data. Transactions guarantee atomicity, consistency, isolation, and durability – the ACID properties – crucial for trustworthy data handling. These properties are critical to maintaining data reliability, avoiding inconsistencies, and offering users trust and confidence in the system.
Stored Procedures: Encapsulating Database Logic
Stored procedures provide a means of encapsulating database logic within the database itself. This improves code organization and reusability, allowing you to create modular and maintainable database applications. Stored procedures offer several benefits. They improve performance because the query plan is cached on the database server. Additionally, they centralize database logic, enhancing security by preventing direct SQL access. Consider a scenario where you need to perform complex data validation or calculations. A stored procedure can encapsulate this logic, making the application code cleaner and easier to manage. A case study examines a payroll system that utilizes stored procedures to calculate employee salaries. Centralizing this logic in the database enhances maintainability and security. This ensures consistent salary calculations regardless of application changes. A second case study features a customer relationship management (CRM) system. Stored procedures handle various actions like adding, updating, and retrieving customer information, simplifying the application's interaction with the database. They are ideal for complex data manipulation tasks, providing a layer of abstraction between the application and database. This improves security, simplifies development, and enhances the overall efficiency of the application. Furthermore, using stored procedures allows for greater flexibility in controlling database access, limiting what the application can directly perform. This promotes a more secure and controlled database environment, especially useful for managing sensitive data. They enhance performance by caching the execution plan. For example, in a large-scale application, stored procedures can drastically reduce overall database load and response times, optimizing the overall performance. The ability to control database logic directly in the database is crucial for maintaining its integrity and security.
Advanced Query Optimization: Mastering the Art of Efficient Queries
Writing efficient SQL queries is crucial for high-performing database applications. Inefficient queries can severely impact performance, leading to slow response times and resource consumption. Advanced techniques such as indexing, query profiling, and understanding the execution plan are critical for optimizing MySQLi queries. Indexing significantly accelerates query performance. Indexes are data structures that provide quick access to data. They are crucial for optimizing queries that involve WHERE clauses, especially those with equality conditions. Consider the impact of indexing a frequently queried column. A case study shows a 50% reduction in query time after indexing a primary key. Another case study focuses on a website with slow search functionality. Profiling the queries revealed a lack of proper indexing. Adding indexes reduced search response times by more than 70%. Poorly written queries can be a major source of performance bottlenecks. Understanding the execution plan using the `EXPLAIN` command in MySQL allows developers to visualize how the database will execute a query. This helps to identify and address areas for optimization. For instance, an inefficient JOIN operation can be significantly improved using the appropriate join type or by adding indexes. Advanced query optimization is not just about indexing. It involves understanding how the query optimizer works and making informed decisions on table design and query writing. Moreover, using appropriate data types is critical. For example, using smaller data types like INT instead of BIGINT can reduce storage space and improve performance. Choosing the right data types and properly designing the database schema contribute significantly to overall performance. The ability to optimize queries is crucial for handling large datasets and ensuring application scalability.
Error Handling and Debugging: Mastering MySQLi Diagnostics
Robust error handling is essential for building reliable MySQLi applications. Understanding how to handle errors effectively, utilizing debugging techniques, and implementing logging mechanisms enables developers to identify and resolve problems promptly. Proper error handling involves catching exceptions, providing meaningful error messages, and logging errors for later analysis. Consider a scenario where a database connection fails. The application should gracefully handle this and provide an informative error message to the user. A case study illustrates a banking application's implementation of robust error handling to prevent unauthorized access attempts. This system logs failed login attempts and notifies administrators. Another case study shows the use of exception handling to gracefully handle database errors in an e-commerce application. Instead of crashing, the application logs the error and displays a user-friendly message. Implementing thorough error handling enhances the user experience and prevents application crashes. This is crucial for applications that handle sensitive data or critical business operations. Furthermore, logging error messages allows developers to trace issues and pinpoint the source of the problem more effectively. Debugging techniques such as using print statements, debuggers, and profiling tools help developers identify and fix problems. The use of logging mechanisms allows developers to monitor application performance, identify potential bottlenecks, and detect errors that might not be immediately apparent. Effective debugging saves time and resources by allowing developers to find and fix problems quickly and efficiently. The ability to handle and debug MySQLi errors effectively is crucial for ensuring the stability and reliability of the application.
In conclusion, mastering MySQLi involves going beyond basic usage. This article has explored advanced techniques, including prepared statements for security, transactions for data integrity, stored procedures for encapsulation, advanced query optimization for performance, and comprehensive error handling and debugging. By implementing these methods, developers can create highly efficient, secure, and reliable database-driven applications capable of handling the demands of modern software development.