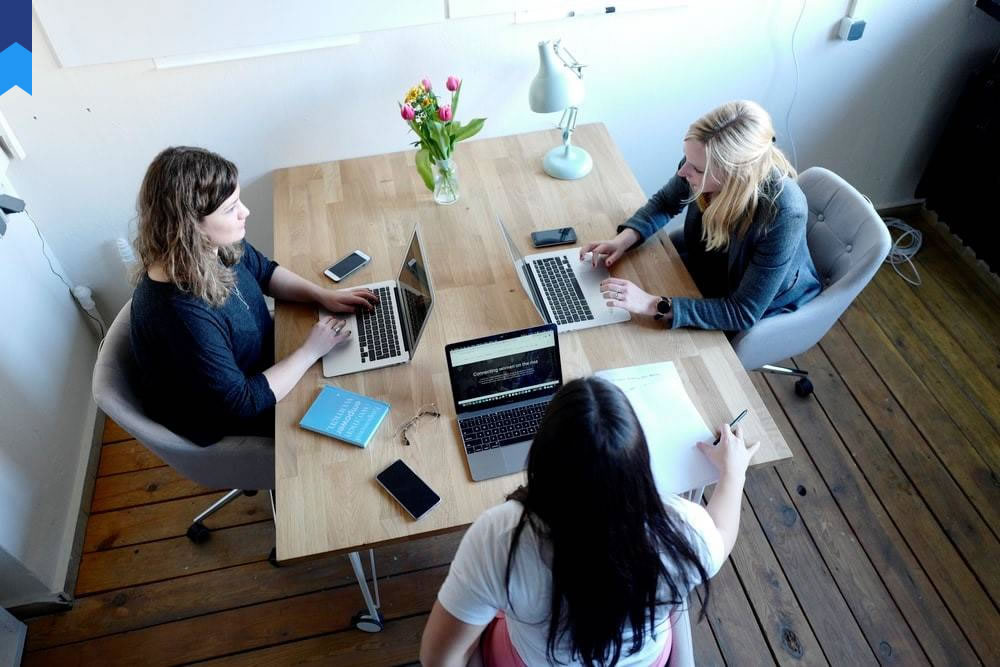
Kotlin's Hidden Depths: Unveiling The Science Behind Advanced Programming
Kotlin, with its concise syntax and powerful features, has rapidly become a favorite among developers. But beneath the surface of its elegant design lie profound principles and techniques that unlock its true potential. This article delves into the “science†of advanced Kotlin programming, exploring practical applications beyond the basics.
Coroutine Concurrency: Mastering Asynchronous Operations
Kotlin's coroutines offer a revolutionary approach to concurrency, enabling asynchronous programming without the complexities of traditional threads. They provide a lightweight and efficient mechanism to handle long-running operations without blocking the main thread, ensuring a responsive user interface. Understanding coroutines involves grasping concepts like suspension, continuation, and scope.
Consider a scenario involving network requests. Without coroutines, each request would block the main thread, leading to a frozen UI. With coroutines, requests can run concurrently, freeing the main thread to handle other tasks. This is achieved by suspending the coroutine at the point of the network call, resuming it only when the response arrives.
Case Study 1: A popular mobile game uses coroutines to handle concurrent data loading, providing a smooth and lag-free experience for users. Without coroutines, loading times would be significantly longer, negatively impacting the user experience.
Case Study 2: An e-commerce application uses coroutines to manage concurrent database operations, ensuring fast and responsive responses to user requests. This results in a more efficient and scalable application that can handle a larger number of users.
The launch function initiates a new coroutine, while withContext allows for switching between different dispatchers. Dispatchers dictate where the coroutine runs (e.g., main thread, background threads). Careful use of dispatchers is crucial for performance and responsiveness.
Error handling within coroutines requires structured concurrency. Techniques like try-catch blocks are crucial to handle exceptions without interrupting the execution of other coroutines. Coroutine scope management ensures that resources are properly released and exceptions are handled correctly. Understanding these intricate details forms the core of advanced coroutine programming.
Advanced techniques include using coroutine builders like async and await for more complex asynchronous scenarios, managing coroutine context and propagation for greater flexibility, and utilizing flow for streamlined data processing.
Experts emphasize the importance of understanding the underlying mechanisms of coroutines to avoid common pitfalls and to write efficient, robust code. Mastering coroutines is not just about writing asynchronous code; it's about writing highly concurrent and performant applications.
Functional Programming in Kotlin: Beyond the Basics
Kotlin embraces functional programming paradigms, allowing for cleaner, more maintainable code. This involves using immutable data structures, higher-order functions, and lambda expressions. While basic understanding focuses on lambda expressions and higher-order functions, advanced application dives deeper into concepts like monads and functors for more robust error handling and data transformation.
Case Study 1: A large-scale data processing pipeline benefits from functional programming's ability to compose functions, enabling modularity and reusability. This results in clearer and easier-to-maintain code.
Case Study 2: A financial modeling application uses immutable data structures to prevent accidental data modification, ensuring the integrity of financial calculations. This leads to more reliable and predictable results.
Higher-order functions, which take functions as arguments or return functions as results, are a cornerstone of functional programming. They enable code reusability and abstraction. For instance, functions like `map`, `filter`, and `reduce` provide concise ways to manipulate collections.
Lambda expressions provide a concise way to define anonymous functions, enhancing the readability of functional code. These anonymous functions are commonly used with higher-order functions. Understanding how to effectively utilize lambda expressions is critical to leveraging the power of functional programming in Kotlin.
Functional programming also significantly enhances testability. The purity of functions (absence of side effects) makes them easier to test in isolation. This contributes to building more robust applications.
Advanced functional concepts include monads and functors, which offer powerful ways to handle potential errors and perform complex data transformations. Monads, for example, encapsulate computations and their potential errors, enabling elegant error handling and chaining of operations.
Immutability, a core tenet of functional programming, minimizes bugs related to unexpected state changes, leading to more reliable and maintainable code. Immutability fosters concurrency safety as shared data cannot be unexpectedly modified by multiple threads, simplifying concurrency management.
Delegation and Extension Functions: Enhancing Code Structure
Delegation and extension functions are powerful Kotlin features that improve code organization and reusability. Delegation allows an object to delegate certain tasks to another object, promoting code modularity and reducing duplication.
Case Study 1: A UI framework utilizes delegation to handle event handling, separating UI logic from business logic. This approach enhances code maintainability and simplifies testing.
Case Study 2: A data access layer leverages delegation to manage database connections, abstracting away the underlying database implementation details. This results in greater flexibility and ease of switching database systems.
Extension functions add new functionality to existing classes without modifying their source code. This capability is particularly useful when working with third-party libraries or extending standard Kotlin classes. Extension functions are a valuable tool in enhancing code structure and promoting reusability.
Property delegation provides a convenient way to implement custom logic for property getters and setters. This mechanism simplifies common property management tasks such as lazy initialization, observable properties, and delegated properties.
Consider a scenario where you need to implement logging for every property change. Delegation simplifies this process, encapsulating the logging logic within a delegate, rather than embedding it within each property's setter. This keeps your core code clean and readable.
Using delegation effectively reduces code duplication by centralizing common functionality, which improves maintainability and reduces the chances of introducing bugs when changing that functionality. This structured approach contributes to cleaner, more organized projects.
Mastering delegation and extension functions requires a deeper understanding of how Kotlin manages object references and method calls. This knowledge is crucial for leveraging these features to their full potential, thereby enhancing overall code quality and readability.
Advanced Data Classes and Data Structures
Beyond the basics of data classes, advanced usage involves leveraging data structures and techniques optimized for specific tasks. Understanding these nuances enables efficient data manipulation and management. Kotlin's data classes simplify the creation of classes that primarily hold data. However, mastering advanced aspects involves understanding how to customize their behavior and using them in conjunction with other Kotlin features.
Case Study 1: A game development project utilizes custom data classes with operators overloaded for efficient vector math operations. This optimization improves performance and simplifies game logic.
Case Study 2: A scientific simulation utilizes custom data classes with custom serialization logic for efficient data storage and retrieval. This improves the efficiency of data management within the application.
Kotlin's collections library provides various data structures optimized for different tasks. Choosing the right data structure (e.g., List, Set, Map) is crucial for performance. Understanding the characteristics of each collection is fundamental to writing efficient code.
Efficient data manipulation often involves the use of higher-order functions like `map`, `filter`, and `reduce`, which allow concise data transformations without explicit loops. This functional approach enhances readability and reduces the likelihood of errors.
Custom data classes can be enhanced by implementing interfaces or extending abstract classes. This extends the capabilities of the data class, such as adding methods for custom validation or data processing. Such flexibility is essential for creating robust and adaptable data structures.
Deep understanding involves recognizing the performance implications of chosen data structures and algorithms. Selecting appropriate data structures based on frequency of operations (search, insertion, deletion) is critical for performance optimization.
Understanding serialization and deserialization of custom data classes is important for persistent storage and data exchange with other systems. This may involve using libraries like Gson or kotlinx.serialization. The proper handling of data serialization can significantly improve the efficiency and flexibility of the application.
Generics and Type Systems: Building Robust and Reusable Code
Kotlin's type system, including generics, is a powerful tool for building robust and reusable code. Generics allow writing code that can work with various types without losing type safety. This increases code reusability and reduces errors. However, mastering generics goes beyond basic usage, involving advanced concepts like type bounds and variance.
Case Study 1: A networking library utilizes generics to create reusable network request functions that can handle various data types. This enhances code reusability and maintainability.
Case Study 2: A data processing framework uses generics to create reusable data transformation functions that operate on different data types. This approach improves code modularity and reduces redundancy.
Type bounds restrict the types that can be used with generics, enabling more specific and type-safe code. For example, a function that operates on comparable objects can use an upper bound to specify that the generic type must implement the `Comparable` interface.
Variance describes how a generic type handles subtyping. Covariance allows using a subtype where a supertype is expected. Contravariance allows using a supertype where a subtype is expected. Invariance restricts the use of subtypes or supertypes. Understanding variance is crucial for writing flexible and type-safe generic code.
Reified type parameters allow accessing the actual type information at runtime, enabling features like runtime type checking and reflection-based operations. This functionality is particularly useful when working with frameworks or libraries that require runtime type information.
Generic functions can improve code reusability by abstracting away type-specific details. This enables writing functions that operate on various types while maintaining type safety. Generic classes provide similar benefits for creating reusable classes.
Advanced techniques include using type aliases to improve code readability and maintainability. This is particularly helpful when working with complex generic types.
Conclusion
Mastering Kotlin's advanced features isn't just about writing code; it's about understanding the underlying principles and choosing the right tools for the task. This journey requires going beyond the syntax and embracing the underlying "science" of design patterns, data structures, and concurrency. By delving into these areas, developers can create high-performing, robust, and maintainable applications. This deeper understanding transforms Kotlin programming from a skill into a craft, enabling developers to build truly exceptional software.
The exploration of coroutines, functional programming, delegation, advanced data structures, and generics reveals the true power and elegance of Kotlin. By mastering these techniques, developers can construct more efficient, robust, and maintainable applications, pushing the boundaries of what's possible. This journey of deep understanding unlocks Kotlin's full potential and distinguishes proficient developers from experts.