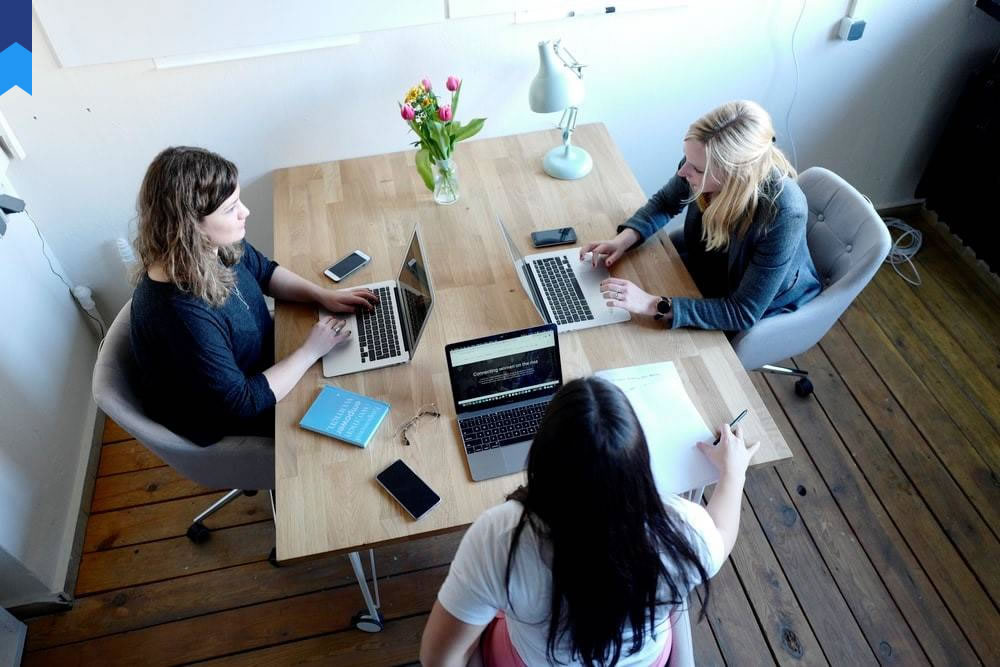
Master Apache POI: A Comprehensive Guide To Working With Word Documents
In the realm of software development, the ability to manipulate and automate document processing is a highly sought-after skill. Apache POI, a powerful Java library, empowers developers to seamlessly interact with various file formats, including Microsoft Word documents (.docx). This comprehensive guide delves into the intricacies of working with Apache POI for Word, equipping you with the knowledge and practical examples to tackle a wide range of document-related tasks.
Introduction
Apache POI, a cornerstone of Java's document processing capabilities, has become an indispensable tool for developers working with Microsoft Office files. This open-source library, renowned for its robust functionality and extensive support for various file formats, provides a gateway to manipulate Word documents programmatically, unlocking a world of automation possibilities.
From creating and modifying documents to extracting data and generating reports, Apache POI offers a versatile arsenal of tools. Its intuitive API, coupled with its ability to handle complex document structures, makes it a favored choice for a diverse range of applications, including data migration, report generation, and document templating.
Whether you're a seasoned Java developer or a newcomer to the world of document manipulation, this guide serves as your comprehensive companion to mastering the art of working with Word documents using Apache POI.
Getting Started with Apache POI
Embarking on your journey with Apache POI is a straightforward process. The first step involves incorporating the library into your Java project. This can be achieved through various dependency management tools, such as Maven or Gradle.
For Maven users, the following dependency declaration in your pom.xml file will set the stage: ```xml
Replace "YOUR_VERSION" with the desired Apache POI version. Similar dependency configurations exist for Gradle and other build systems. Once the library is integrated, you're ready to unleash the power of Apache POI.
A simple code example demonstrates the fundamental steps of creating a new Word document and adding content: ```java import org.apache.poi.xwpf.usermodel.XWPFDocument; import org.apache.poi.xwpf.usermodel.XWPFParagraph; public class WordDocumentCreation { public static void main(String[] args) throws Exception { // Create a new Word document XWPFDocument document = new XWPFDocument(); // Create a new paragraph XWPFParagraph paragraph = document.createParagraph(); // Set the paragraph text paragraph.createRun().setText("This is a sample Word document created using Apache POI."); // Save the document document.write(new FileOutputStream("sample.docx")); document.close(); } } ```
This code snippet demonstrates the core functionality of creating a new Word document, adding a paragraph with text, and saving the document to a file.
Manipulating Text and Formatting
Apache POI provides granular control over text manipulation and formatting within Word documents. You can seamlessly add, modify, and delete text, apply various formatting styles, and control the appearance of your document.
To illustrate text manipulation, let's modify the existing paragraph with a bold, italicized, and underlined style: ```java import org.apache.poi.xwpf.usermodel.XWPFDocument; import org.apache.poi.xwpf.usermodel.XWPFParagraph; import org.apache.poi.xwpf.usermodel.XWPFRun; public class TextFormatting { public static void main(String[] args) throws Exception { // Load an existing Word document XWPFDocument document = new XWPFDocument(new FileInputStream("sample.docx")); // Get the first paragraph XWPFParagraph paragraph = document.getParagraphs().get(0); // Get the first run in the paragraph XWPFRun run = paragraph.getRuns().get(0); // Apply bold, italic, and underline formatting run.setBold(true); run.setItalic(true); run.setUnderline(XWPFRun.UUNDERLINE_SINGLE); // Save the document document.write(new FileOutputStream("formatted.docx")); document.close(); } } ```
In this example, we retrieve the first paragraph and its associated run, then apply bold, italic, and underline styles to the text. The modified document is subsequently saved as "formatted.docx".
Working with Tables
Tables are an integral part of many Word documents. Apache POI empowers you to create, modify, and manipulate tables with ease. You can define table dimensions, add rows and columns, populate cells with data, and apply cell styles to enhance the visual presentation.
Let's create a simple table with two rows and two columns, populate it with data, and add some basic formatting: ```java import org.apache.poi.xwpf.usermodel.XWPFDocument; import org.apache.poi.xwpf.usermodel.XWPFTable; import org.apache.poi.xwpf.usermodel.XWPFTableRow; import org.apache.poi.xwpf.usermodel.XWPFTableCell; public class TableManipulation { public static void main(String[] args) throws Exception { // Create a new Word document XWPFDocument document = new XWPFDocument(); // Create a new table with 2 rows and 2 columns XWPFTable table = document.createTable(2, 2); // Set table cell text XWPFTableRow row1 = table.getRow(0); XWPFTableCell cell11 = row1.getCell(0); XWPFTableCell cell12 = row1.getCell(1); XWPFTableRow row2 = table.getRow(1); XWPFTableCell cell21 = row2.getCell(0); XWPFTableCell cell22 = row2.getCell(1); cell11.setText("Name"); cell12.setText("Age"); cell21.setText("John Doe"); cell22.setText("30"); // Apply cell border formatting for (XWPFTableRow row : table.getRows()) { for (XWPFTableCell cell : row.getCells()) { cell.getCTTc().addNewTcPr().addNewTcBorders().addNewBottom().setVal(STBorder.SINGLE); } } // Save the document document.write(new FileOutputStream("table.docx")); document.close(); } } ```
In this code, we define a table with two rows and columns, add text to each cell, and apply simple border formatting to the cells. Apache POI provides a wide range of options for customizing table appearance, including cell alignment, background colors, and more.
Advanced Features: Images and Lists
Beyond basic text and tables, Apache POI enables you to incorporate images and lists into your Word documents, enriching their content and presentation. You can seamlessly embed images from local or remote sources, while lists provide structured ways to organize information.
To illustrate image insertion, let's add a picture to our document: ```java import org.apache.poi.xwpf.usermodel.XWPFDocument; import org.apache.poi.xwpf.usermodel.XWPFParagraph; import org.apache.poi.xwpf.usermodel.XWPFPictureData; import org.apache.poi.xwpf.usermodel.XWPFRun; import org.apache.poi.util.IOUtils; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.InputStream; public class ImageInsertion { public static void main(String[] args) throws Exception { // Create a new Word document XWPFDocument document = new XWPFDocument(); // Create a new paragraph XWPFParagraph paragraph = document.createParagraph(); // Insert an image XWPFRun run = paragraph.createRun(); InputStream imageStream = new FileInputStream("image.jpg"); XWPFPictureData pictureData = document.addPictureData(imageStream, XWPFPictureData.PICTURE_TYPE_JPEG); run.addPicture(pictureData.getPictureData(), document.getNextPicNameNumber(), "image.jpg", 100, 100); // Save the document document.write(new FileOutputStream("image.docx")); document.close(); } } ```
In this code, we add a picture from "image.jpg" to our document. The "addPictureData" method adds the image data to the document, while the "addPicture" method inserts the image within a paragraph, specifying its dimensions.
For list creation, Apache POI supports both numbered and bulleted lists. Here's an example of creating a bulleted list: ```java import org.apache.poi.xwpf.usermodel.XWPFDocument; import org.apache.poi.xwpf.usermodel.XWPFParagraph; import org.apache.poi.xwpf.usermodel.XWPFRun; public class ListCreation { public static void main(String[] args) throws Exception { // Create a new Word document XWPFDocument document = new XWPFDocument(); // Create a new paragraph XWPFParagraph paragraph = document.createParagraph(); // Create a bulleted list paragraph.getCTP().addNewPPr().addNewNumPr().addNewIlvl().setVal(0); // Add list items XWPFRun run1 = paragraph.createRun(); run1.setText("Item 1"); paragraph = document.createParagraph(); paragraph.getCTP().addNewPPr().addNewNumPr().addNewIlvl().setVal(0); XWPFRun run2 = paragraph.createRun(); run2.setText("Item 2"); // Save the document document.write(new FileOutputStream("list.docx")); document.close(); } } ```
This code generates a bulleted list with two items. The "Ilvl" element within the paragraph properties controls the list level. By setting "Ilvl" to 0, we create top-level list items.
Conclusion
Apache POI, with its comprehensive API and versatile functionality, empowers developers to programmatically interact with Word documents, opening up a world of possibilities for document automation, manipulation, and analysis. This guide has provided a comprehensive exploration of key Apache POI concepts, covering topics such as document creation, text formatting, table manipulation, and the inclusion of images and lists.
By leveraging the power of Apache POI, you can streamline document-related processes, automate report generation, extract valuable data, and enhance your software applications with rich document integration capabilities. As you embark on your journey with Apache POI, remember to leverage its extensive documentation, explore the vast community resources, and embrace the endless potential it offers for your document-centric projects.