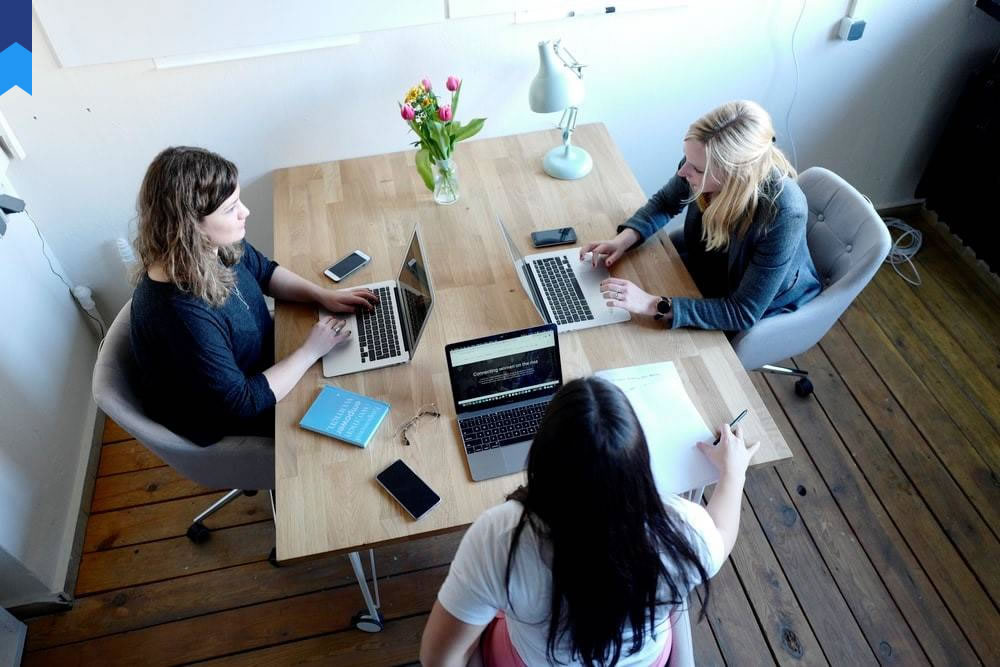
Master ASP.NET Core Security: A Comprehensive Guide To Protecting Your Applications
In today's digital landscape, cybersecurity is paramount. As applications become increasingly complex and interconnected, safeguarding them from malicious attacks is essential. ASP.NET Core, a popular framework for building web applications, provides a robust set of tools and features for implementing comprehensive security measures. This guide will delve into the core principles and best practices for securing ASP.NET Core applications, empowering developers to build secure and reliable applications.
Authentication and Authorization
Authentication and authorization are fundamental pillars of application security. Authentication verifies the identity of users attempting to access the application, while authorization determines what actions they are permitted to perform. ASP.NET Core offers a flexible and extensible authentication system that allows for seamless integration with various authentication providers.
One common approach is using cookie-based authentication. This involves storing authentication tokens in cookies on the client's browser, enabling the server to identify and verify the user's identity for subsequent requests. ASP.NET Core supports a variety of cookie authentication schemes, including the default scheme and custom schemes. The default scheme handles token creation and validation, while custom schemes allow developers to implement specific authentication logic.
Another popular method is using JSON Web Tokens (JWTs). JWTs are self-contained tokens that encode user information and authentication data. They are often used in stateless authentication scenarios, where the server doesn't need to maintain session state. ASP.NET Core provides middleware for issuing and validating JWTs, simplifying integration with JWT-based authentication providers.
Authorization is crucial for restricting access to specific resources or actions within an application. ASP.NET Core's authorization framework allows developers to define policies that determine who has access to certain parts of the application. Policies can be based on user roles, claims, or custom logic. Developers can use attributes or authorization middleware to apply policies to controllers, actions, or individual resources.
Case Study 1: E-commerce Website Security
An e-commerce website requires secure authentication and authorization to protect sensitive customer data and financial transactions. Implementing robust authentication mechanisms, such as two-factor authentication, and granular authorization policies, such as limiting access to specific payment gateways based on user roles, is essential for securing customer accounts and preventing unauthorized purchases.
Case Study 2: Enterprise Application Access Control
An enterprise application often involves managing user permissions and restricting access to specific modules or features based on roles and responsibilities. ASP.NET Core's authorization framework enables developers to define comprehensive policies that enforce access control based on user roles, claims, and custom logic, ensuring that only authorized personnel can access sensitive information and perform critical operations.
Cross-Site Scripting (XSS) Prevention
Cross-site scripting (XSS) is a common web security vulnerability that occurs when malicious scripts are injected into a website, potentially compromising user data or executing unauthorized actions. ASP.NET Core provides several mechanisms for mitigating XSS attacks, including data sanitization, input validation, and output encoding.
Data sanitization involves removing or modifying potentially harmful characters from user input before it is processed by the server. Input validation enforces rules on the types and formats of user input, preventing the injection of malicious scripts. Output encoding converts special characters in user input to their HTML-safe equivalents, preventing the execution of scripts in the browser.
ASP.NET Core's built-in Anti-XSS library helps developers sanitize and validate user input, reducing the risk of XSS attacks. Additionally, the framework automatically encodes output by default, providing a layer of protection against XSS vulnerabilities. Developers should be aware of XSS prevention techniques and implement them consistently throughout the application.
Case Study 1: Social Media Platform Security
A social media platform is vulnerable to XSS attacks, as users can potentially inject malicious scripts into their posts or comments. By implementing robust input validation and output encoding mechanisms, developers can prevent malicious scripts from being executed, safeguarding user data and protecting the platform from exploitation.
Case Study 2: Healthcare Application Security
A healthcare application handles sensitive patient information, making it a prime target for XSS attacks. By using ASP.NET Core's anti-XSS library and implementing strict input validation and output encoding policies, developers can protect patient data from unauthorized access and manipulation, ensuring confidentiality and integrity.
SQL Injection Prevention
SQL injection is a common attack that exploits vulnerabilities in database queries to manipulate data, compromise data integrity, or gain unauthorized access to sensitive information. ASP.NET Core provides mechanisms for preventing SQL injection attacks, including parameterized queries and data sanitization.
Parameterized queries use placeholders to represent data values in SQL statements, preventing malicious code from being injected into the query string. Data sanitization ensures that data passed to the database is properly encoded and escaped, preventing the execution of malicious SQL commands.
ASP.NET Core's Entity Framework Core provides built-in support for parameterized queries, simplifying SQL injection prevention. Developers should avoid using string concatenation to build SQL queries and rely on parameterized queries or ORM tools like Entity Framework Core to ensure safe and secure database interactions.
Case Study 1: Online Banking Application Security
An online banking application handles financial transactions, making it a prime target for SQL injection attacks. By using parameterized queries and data sanitization, developers can prevent attackers from manipulating database records, safeguarding customer funds and maintaining the integrity of financial transactions.
Case Study 2: E-commerce Platform Security
An e-commerce platform relies heavily on database interactions to manage product listings, customer orders, and inventory. Implementing SQL injection prevention measures is critical for protecting sensitive customer data, ensuring accurate inventory tracking, and preventing fraudulent activities.
API Security
APIs are becoming increasingly popular for building modern web applications and integrating with external services. Securing APIs is crucial for preventing unauthorized access, data breaches, and other security threats. ASP.NET Core offers several features for securing APIs, including authentication, authorization, and rate limiting.
ASP.NET Core provides a streamlined way to build APIs with robust security features. It supports both cookie-based authentication and JWT authentication, allowing developers to choose the most appropriate method for their API. Authorization policies can be applied to API endpoints, restricting access to specific resources or actions based on user roles, claims, or custom logic.
Rate limiting is an important security measure that prevents denial-of-service attacks by limiting the number of requests that can be made to an API within a specific time period. ASP.NET Core provides built-in rate limiting middleware that allows developers to configure rate limits based on specific criteria, such as IP address, user agent, or API key.
Case Study 1: Payment Gateway API Security
A payment gateway API handles sensitive financial information, making it a critical target for security threats. Implementing robust authentication, authorization, and rate limiting mechanisms is essential for preventing unauthorized access and ensuring the security of financial transactions.
Case Study 2: Social Media API Security
A social media API provides access to user data and functionality. Implementing API security measures, such as authentication, authorization, and rate limiting, is crucial for protecting user data, preventing unauthorized access, and mitigating abuse or malicious activities.
Conclusion
Building secure ASP.NET Core applications is essential for protecting sensitive data, preventing malicious attacks, and ensuring user trust. By implementing robust authentication and authorization, mitigating XSS and SQL injection vulnerabilities, and securing APIs, developers can create applications that are resilient to security threats. The framework's built-in features and best practices provide a solid foundation for building secure and reliable applications in today's digital landscape.
Staying up-to-date with the latest security best practices and vulnerabilities is crucial. Developers should leverage industry-standard security tools, libraries, and frameworks, and engage in continuous security testing and vulnerability assessments to identify and address potential security gaps.
Remember, security is an ongoing process. By embracing a proactive approach to security, developers can build secure ASP.NET Core applications that protect user data, maintain application integrity, and ensure a safe and reliable user experience.