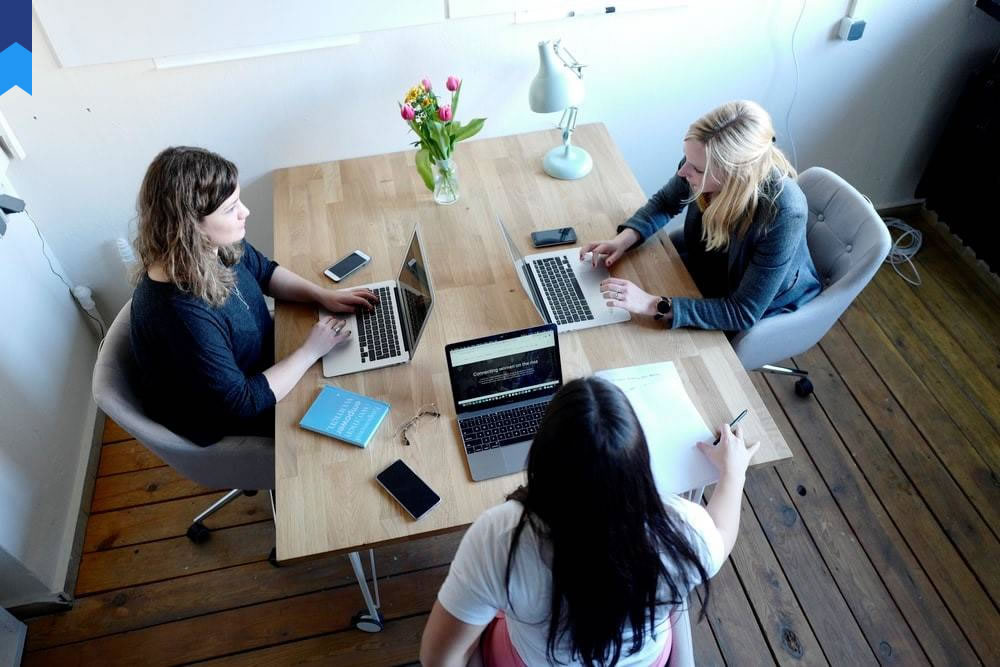
Mastering Arduino Interrupts: A Comprehensive Guide To Event-Driven Programming
In the realm of embedded systems, where time is of the essence and resources are limited, efficient code execution is paramount. Arduino, with its ease of use and powerful capabilities, has become a cornerstone for hobbyists and professionals alike. However, traditional loop-based programming often falls short when it comes to handling real-time events and asynchronous tasks. This is where interrupts come into play, empowering Arduino to respond to external stimuli promptly and efficiently.
This comprehensive guide delves into the world of Arduino interrupts, unraveling the intricacies of event-driven programming. We will explore the fundamental concepts, practical applications, and essential techniques for harnessing the power of interrupts to create sophisticated and responsive Arduino projects.
Introduction
Arduino interrupts are a powerful mechanism that allows the microcontroller to respond to external events without waiting for the main loop to cycle through its code. Think of them as a way for your Arduino to be alerted when something specific happens, such as a button press, a sensor reading a change, or a timer reaching a certain point. These events trigger pre-defined functions (called Interrupt Service Routines, or ISRs), which are executed immediately, allowing your Arduino to react instantly and dynamically.
Interrupts enable you to design projects that are more responsive, efficient, and capable of handling multiple tasks concurrently. For instance, you can use interrupts to read data from a sensor, communicate with other devices, or control external hardware without slowing down your main program.
Understanding Interrupt Fundamentals
At the heart of Arduino interrupts lies the concept of "event-driven programming". Instead of relying on a linear execution flow, the microcontroller waits for a specific event to occur. Upon detection, a designated ISR is executed, allowing the system to react promptly and efficiently. This asynchronous behavior is crucial for handling time-sensitive operations.
Let's break down the key components of Arduino interrupts:
- **Interrupt Pins:** Arduino boards offer specific pins dedicated to interrupt functionality. These pins can be configured to trigger an interrupt based on various conditions.
- **Interrupt Triggers:** You can specify how an interrupt should be triggered, using options such as:
- Rising Edge: The interrupt triggers when the pin transitions from LOW to HIGH.
- Falling Edge: The interrupt triggers when the pin transitions from HIGH to LOW.
- Change: The interrupt triggers on any change in the pin state.
- Low Level: The interrupt triggers when the pin is LOW.
- High Level: The interrupt triggers when the pin is HIGH.
- **Interrupt Service Routine (ISR):** This is a special function that is executed automatically whenever an interrupt occurs. The ISR should be concise and efficient, as it runs with a higher priority than the main loop.
Consider a classic example: a button press. In a traditional loop-based approach, you would constantly poll the button state to check for a press. However, with interrupts, you can configure a pin to trigger an interrupt on a rising edge (button press). This allows your Arduino to perform other tasks while waiting for the button press, and when the button is pressed, the ISR is triggered immediately, executing the necessary code.
Common Interrupt Applications
The world of Arduino interrupts opens up a vast array of possibilities for creating innovative and responsive projects. Let's explore some of the most common applications:
- **Real-Time Data Acquisition:** Interrupts are ideal for capturing data from sensors at precise intervals. By configuring a timer interrupt to trigger at regular intervals, you can sample data from sensors without interrupting the flow of your main program.
- **Button Debouncing:** Mechanical buttons are prone to bouncing, generating multiple signals for a single press. Interrupts, with their ability to respond to rising or falling edges, can effectively debounce button signals, ensuring accurate input detection.
- **Asynchronous Communication:** Interrupts excel in handling communication protocols like SPI, I2C, and UART. When data is received, an interrupt can trigger the ISR to process the data, allowing your Arduino to communicate with other devices efficiently and seamlessly.
- **Motor Control:** In applications involving motor control, interrupts can provide precise timing for controlling motor speed and direction. By using timer interrupts, you can ensure accurate and responsive motor operation.
Practical Interrupts: Case Studies
To truly grasp the power of Arduino interrupts, let's delve into real-world case studies that illustrate their practical applications:
Case Study 1: Real-Time Data Logging with an Accelerometer
Imagine building a project that monitors vibrations in a machine using an accelerometer. Interrupts can play a pivotal role in capturing this data efficiently. By configuring a timer interrupt to trigger at a specific frequency, you can read data from the accelerometer and store it in memory. The interrupt-driven approach ensures that data acquisition is synchronized with the desired sampling rate, providing accurate insights into the machine's vibrations.
Case Study 2: Touch-Sensitive User Interface with Capacitive Sensing
Touch-sensitive interfaces have become ubiquitous in modern technology. Interrupts are the cornerstone of touch-sensitive user interfaces built with capacitive sensing. By configuring interrupt pins to trigger on changes in capacitance, you can detect touches on specific areas of a capacitive sensor. This allows you to create responsive and intuitive user interactions without constant polling, making the interface more efficient and responsive.
Advanced Interrupt Techniques
As your Arduino projects become more complex, you may find yourself needing to implement advanced interrupt techniques to optimize performance and handle intricate scenarios. Here are some techniques that will elevate your interrupt skills:
- **Nested Interrupts:** In situations where you need to respond to multiple interrupts simultaneously, nested interrupts can be employed. A nested interrupt is triggered within another interrupt, allowing you to handle multiple events in a hierarchical manner.
- **Interrupt Priorities:** In scenarios where multiple interrupts are active, you can prioritize them based on their importance. Higher-priority interrupts are processed first, ensuring that critical events are handled promptly.
- **Interrupt Disabling:** To prevent interrupts from interfering with time-critical operations, you can temporarily disable interrupts using the `noInterrupts()` function. This ensures that the critical code executes without interruptions. Once the critical code is complete, you can re-enable interrupts using the `interrupts()` function.
- **Interrupt-Driven Communication:** For advanced communication tasks, interrupts can be used to handle data transmission and reception efficiently. By configuring an interrupt to trigger on data arrival, you can process incoming data asynchronously without blocking your main program.
Conclusion
Arduino interrupts are a powerful tool in the arsenal of every embedded programmer. They unlock the potential for creating responsive, efficient, and sophisticated projects that can handle real-time events and asynchronous tasks with grace. From data acquisition and button debouncing to advanced communication and motor control, interrupts empower you to build projects that react to the world around them in an intelligent and dynamic way. By mastering the art of interrupts, you will unlock a new dimension of possibilities in your Arduino journey.