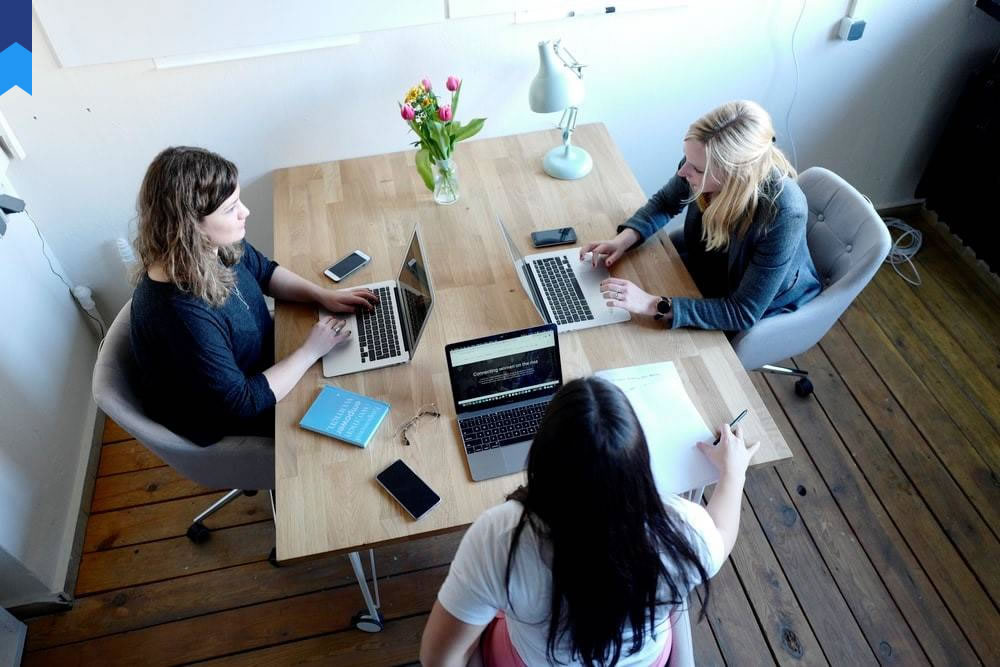
Mastering Arduino Serial Communication: A Comprehensive Guide
Arduino Serial Communication: A Deep Dive into Effective Data Transfer
Understanding Serial Communication Fundamentals
Serial communication forms the backbone of many Arduino projects, enabling the microcontroller to interact with computers, sensors, and other devices. This fundamental method of data transfer involves sending data bit by bit over a single wire, a process governed by protocols such as UART (Universal Asynchronous Receiver/Transmitter). Understanding these protocols is crucial for efficient communication. A common misconception is that serial communication is inherently slow; however, modern microcontrollers can achieve impressive data rates. The actual speed depends on factors like baud rate, data bit length, stop bits, and parity. For instance, a common baud rate is 9600 bps, meaning 9600 bits per second. However, higher rates are achievable, allowing for rapid data transmission in applications like real-time sensor readings. Different data formats, such as ASCII and binary, can influence the efficiency of the transmission. Choosing the right format based on your application is key to optimization.
Consider a project that involves reading temperature data from a sensor and transmitting it to a computer. Using serial communication, the Arduino can read the temperature, convert it into a digital value, and transmit it serially to the computer. The computer, using a suitable program (e.g., Processing, Python), then receives and processes the data. Understanding how to format this data—whether as an integer or a floating-point number—is essential for error-free transmission and interpretation. Error checking mechanisms can also be incorporated, providing robustness to the communication channel. These mechanisms involve techniques such as parity bits, checksums, or more sophisticated error correction codes. The choice depends on the application’s required reliability and the complexity that can be tolerated.
Case Study 1: A weather monitoring station uses serial communication to transmit temperature, humidity, and pressure data to a central server. The baud rate is carefully chosen to ensure reliable data transmission without exceeding the capabilities of the communication channel. The data format is structured using a comma-separated value (CSV) format for easy parsing on the server-side. Error detection is implemented using checksums to verify data integrity during transmission. Case Study 2: An automated irrigation system uses serial communication to receive sensor data indicating soil moisture levels. The system utilizes a low baud rate to minimize power consumption and prioritizes reliability over speed. Data is transmitted in a simple binary format for efficient processing by the microcontroller controlling the irrigation valves. Error detection is minimal, since missed irrigation cycles are less critical than data corruption in the weather station example.
The selection of the appropriate baud rate is a crucial consideration. A higher baud rate facilitates faster communication but increases the risk of transmission errors, especially in noisy environments. Conversely, lower baud rates offer more robust communication but at the cost of reduced speed. The choice depends on the application's requirements for speed versus reliability. Careful consideration must also be given to the communication protocol. For example, using a hardware flow control mechanism can prevent data loss when the receiving end is unable to process data at the same rate as the transmitting end. Many factors influence the selection of hardware, such as the type of microcontroller and the nature of the connected devices. For instance, using a dedicated UART module offers improved performance and reliability compared to software-based serial communication.
Serial Communication with Arduino Libraries
The Arduino IDE provides several libraries that simplify serial communication. The Serial library is the cornerstone, offering functions for sending and receiving data. Its simplicity makes it ideal for beginners. Key functions include Serial.begin() for initializing the serial port, Serial.print() for sending data, and Serial.read() for receiving data. Understanding the nuances of these functions is essential for effective communication. For example, Serial.print() can be used to transmit various data types, such as integers, floating-point numbers, and strings, offering flexibility in data representation. The choice of data type affects the efficiency and the amount of data being transmitted. Data serialization, converting data structures into a byte stream, becomes more crucial with complex data sets.
Advanced features include using Serial.println() which adds a newline character at the end of each transmission. This is often essential for proper data parsing in receiving applications. Proper termination of data streams is a key aspect of successful serial communication, preventing data corruption. Buffering also plays a role; the Serial library utilizes buffers to store incoming and outgoing data. Understanding the size of these buffers is essential for avoiding data loss. Case Study 1: A robotics project uses the Serial library to send control commands to a robot arm. The commands are structured as simple text strings, making the code easy to understand and debug. The robot arm’s response is received via the same serial port, allowing for a two-way communication system. Case Study 2: A home automation system uses the Serial library to send sensor data wirelessly over an XBee module. The data is packaged efficiently to minimize transmission time, thereby enhancing system responsiveness. This involves careful consideration of the data types used and the efficiency of data packing.
Beyond the basic Serial library, other libraries offer enhanced functionality. For example, libraries designed for specific sensors often include functions for serial communication, further simplifying the coding process. These libraries frequently provide higher-level abstractions, hiding the complexities of the underlying serial communication protocols. Using these libraries often makes development faster and less prone to errors. However, understanding the basics of serial communication remains crucial even when using these higher-level tools. This allows for more efficient troubleshooting and adaptation to unforeseen circumstances. Consider the benefits of using dedicated serial communication chips, which often offer faster data rates and more robust error handling capabilities compared to using the microcontroller's built-in UART. Choosing between software and hardware serial communication depends on factors like the required data rate and the availability of resources.
Debugging serial communication can often be challenging. The use of serial monitors and debuggers is essential. Analyzing the transmitted and received data helps identify problems. Systematic troubleshooting techniques, such as checking baud rates, data formats, and cable connections, are paramount. Careful consideration of potential sources of error and meticulous testing are crucial steps in any serial communication project. Understanding how to interpret error messages and logs is another skill that is crucial for successful troubleshooting. Effective use of debugging tools, such as serial monitors and logic analyzers, drastically improves troubleshooting efficiency. Remember that effective communication requires a clear understanding of the intended data exchange, encompassing the data types, format, and transmission protocol.
Advanced Serial Communication Techniques
Beyond basic text-based communication, advanced techniques unlock the potential for more efficient data transfer. Binary data transmission offers significant advantages in terms of bandwidth efficiency, especially when dealing with large datasets or sensor readings containing high resolution. However, it requires careful consideration of data structure and parsing on both the sending and receiving ends. Using custom protocols can enable more complex communication features. For example, a custom protocol might include features such as request-response mechanisms, error handling, and data acknowledgment, making the communication more robust. These features improve the reliability and efficiency of data transfer, minimizing the chance of data loss or corruption.
Implementing error checking and correction is crucial for reliable communication, especially in environments with potential noise or interference. Techniques like checksums, parity bits, and more sophisticated error correction codes can significantly improve data integrity. The choice of error detection and correction technique depends on factors like the severity of the consequences of errors and the available resources. Balancing complexity and reliability is a key consideration. Case Study 1: A remote sensing application utilizes binary data transmission to send high-resolution sensor readings efficiently. The data is structured using a pre-defined binary format, ensuring seamless decoding on the receiving end. The system includes robust error checking to maintain data integrity despite possible interference during transmission. Case Study 2: A networked system of Arduino devices utilizes a custom communication protocol with request-response mechanisms for managing data flow and synchronization between the devices. The custom protocol incorporates features like device identification and data acknowledgment for reliable communication.
Data buffering is essential for handling varying data rates between the sender and receiver. Implementing buffers on both ends ensures that data is not lost during temporary fluctuations in transmission speed or processing capacity. Efficient buffer management is crucial for avoiding data overflow or underflow. The size of the buffer depends on the expected data rate and processing capabilities of both devices. Careful consideration must be given to how the buffer is managed to prevent buffer starvation or buffer overrun. Asynchronous communication allows for more efficient handling of multiple communication tasks concurrently. This is particularly useful when the Arduino needs to interact with multiple devices simultaneously. This approach minimizes delays and improves overall system responsiveness. Careful synchronization and appropriate communication protocols are needed to avoid conflicts.
Software-based and hardware-based serial communication have distinct advantages and disadvantages. Software serial libraries are easier to implement and require less hardware, while hardware serial ports usually provide higher data rates and are less prone to interference. The optimal choice depends on the specific project's requirements. The selection also depends on the availability of resources on the microcontroller and the desired performance characteristics. When choosing a communication approach, carefully weigh the advantages and disadvantages of each option based on project specifications and resource constraints. Consider factors like the desired data rate, reliability requirements, power consumption, and the complexity of implementation.
Troubleshooting and Best Practices
Troubleshooting serial communication often involves systematic checks. Begin by verifying the baud rate settings on both the transmitting and receiving ends. Incorrect baud rate settings are a common cause of communication failures. Next, examine the wiring and connections for any loose or faulty connections. Faulty wiring is another common source of errors. Always ensure proper grounding and shielding to minimize electrical interference. Then, check the data format on both ends to make sure both devices are using the same data format and are interpreting data correctly. Incompatible data formats lead to incorrect data interpretation. Also, review the code for any errors in data handling or transmission. Coding errors can lead to unexpected behavior. Employ debugging techniques, such as using a serial monitor to view the transmitted and received data, to identify the location of the problem.
Utilizing a logic analyzer can provide invaluable insights into the communication process. A logic analyzer can capture the signals on the serial lines and visually display the data being transmitted and received. This allows for a detailed analysis of timing and signal integrity. If problems persist, consider using a higher-level communication protocol, like I2C or SPI, which may offer improved reliability. These protocols offer more robust error detection and correction mechanisms compared to simple serial communication. Remember that the choice of communication method depends on the application's needs and complexity. Always prioritize robust code design. The implementation of robust error handling is crucial for reliable communication. For instance, adding error detection or correction mechanisms can make your system more resilient. The design of error handling mechanisms depends on the severity of consequences of errors and system complexity.
Consider external factors such as environmental noise. In noisy environments, electrical interference can affect signal integrity. Proper shielding and grounding are vital for mitigating this interference. External devices, such as wireless modules, can also interfere. The use of shielded cables reduces external interference. Software-based solutions for noise reduction are also available. Use appropriate shielding and grounding techniques to reduce noise and interference. These techniques improve the reliability and stability of serial communication. Case Study 1: In a robotics project, improper grounding led to intermittent communication errors. Correcting the grounding significantly improved the reliability of the system. Case Study 2: In an industrial setting, electromagnetic interference from nearby machinery caused data corruption. Shielding the serial cables solved the problem, ensuring reliable communication.
Documenting your code and communication protocols is crucial for long-term maintainability and troubleshooting. Clear documentation enhances understanding of the communication system, especially when working on complex projects or collaborating with others. Well-documented code makes future maintenance and debugging much easier. For collaboration, use version control and consistent naming conventions. Good documentation facilitates seamless collaboration. Thorough testing is vital for ensuring reliable communication. Systematic testing helps identify potential problems before deployment. Thorough testing ensures stability and reliability of the system. Remember that testing should cover various scenarios and stress conditions. Testing should include edge cases and boundary conditions.
Future Trends in Arduino Serial Communication
The field of serial communication is constantly evolving. Higher baud rates will continue to be crucial, enabling faster data transfer for increasingly demanding applications. This necessitates improvements in microcontroller capabilities and communication protocols. Low-power serial communication is becoming increasingly important for battery-powered applications. This involves developing more energy-efficient communication protocols and hardware. Improving energy efficiency will extend battery life. The integration of serial communication with other communication protocols, such as I2C and SPI, will become more prevalent. This allows for flexible and efficient communication within systems. Combined communication offers advantages in system design.
Software-defined radio (SDR) techniques are increasingly integrated with serial communication. SDR allows for flexible and reconfigurable communication systems. The use of SDR enhances flexibility and adaptability. Advances in microcontroller technology will lead to improved serial communication capabilities. Faster processors enable higher data rates and more sophisticated communication protocols. Improved processor capabilities increase communication speed. Security in serial communication will become increasingly important. This involves developing secure communication protocols and hardware. Secure communication enhances system security. Improved security features minimize vulnerabilities.
The rise of the Internet of Things (IoT) is driving demand for reliable and efficient serial communication. IoT applications often involve many devices communicating with each other. IoT increases the need for robust and efficient communication. The development of more advanced error detection and correction techniques will be crucial. This enhances the robustness of communication in challenging environments. More robust error handling enhances system stability. The integration of AI and machine learning into serial communication will lead to more intelligent and adaptive systems. This allows for self-optimizing communication systems. The use of AI improves communication efficiency and system adaptability. Artificial Intelligence assists in improving system performance.
Improved software tools and libraries will streamline serial communication development. This will make it easier for developers to create and maintain serial communication systems. Improved tools simplify development and debugging. User-friendly tools enhance development experience. The development of standardized communication protocols will improve interoperability between devices. Standardization enhances system interoperability and simplifies communication. Consistent communication standards minimize compatibility issues. Increased adoption of these standards simplifies development and improves system integration.
Conclusion
Mastering Arduino serial communication is a crucial skill for any Arduino programmer. From understanding fundamental concepts to employing advanced techniques, this guide has provided a comprehensive overview. By implementing best practices and addressing potential troubleshooting scenarios, developers can create robust and efficient communication systems. The future of serial communication in the Arduino ecosystem is bright, with ongoing advancements in speed, power efficiency, and security paving the way for innovative applications. Continuous learning and adaptation to emerging trends are essential for staying ahead in this dynamic field. The application of serial communication is vast and continuously expanding with technological advancements, solidifying its importance in the realm of embedded systems programming. Proper understanding and efficient implementation of this technique will always provide a competitive edge in various Arduino projects, large or small.