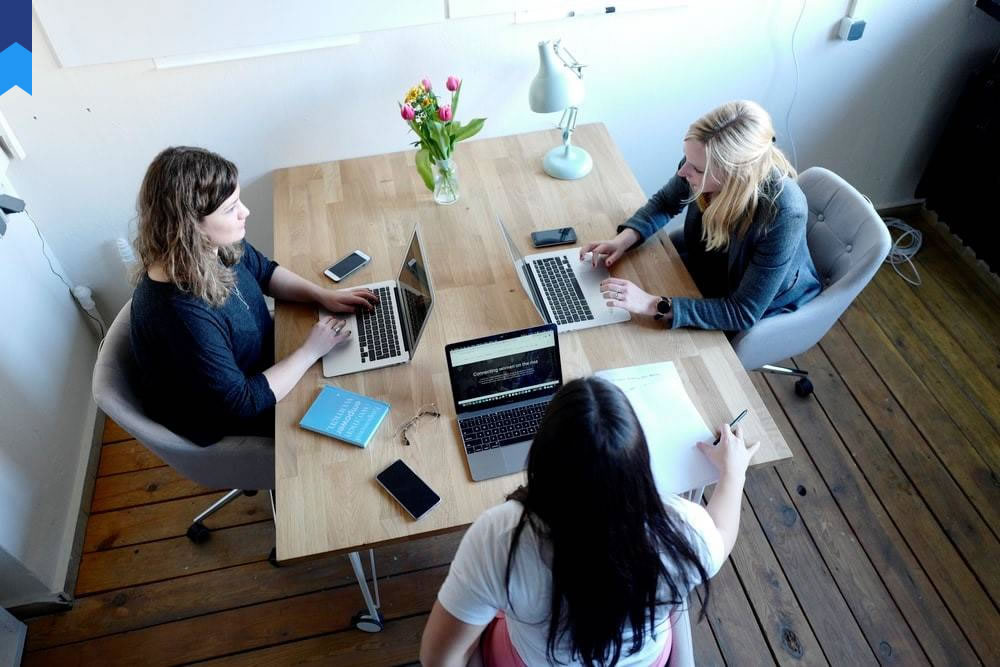
Mastering ASP.NET Core: A Comprehensive Guide To Building Dynamic Web Applications
ASP.NET Core, a powerful and versatile framework, empowers developers to build robust and scalable web applications. From simple websites to complex enterprise applications, ASP.NET Core offers a comprehensive set of tools and features to streamline development and enhance performance. This comprehensive guide delves into the intricacies of ASP.NET Core, providing a roadmap for mastering its capabilities and building exceptional web applications.
Understanding ASP.NET Core Fundamentals
ASP.NET Core stands as a modern, open-source framework designed for building web applications. Its modular architecture and cross-platform compatibility make it a preferred choice for developers seeking flexibility and efficiency. ASP.NET Core leverages the power of .NET, a comprehensive development platform, providing access to a vast library of tools and components. Key features of ASP.NET Core include:
- Cross-platform Compatibility: ASP.NET Core embraces cross-platform development, running seamlessly on Windows, macOS, and Linux. This flexibility allows developers to choose their preferred operating system and development environment.
- Modular Architecture: ASP.NET Core's modular structure enables developers to select only the components required for their project, minimizing resource consumption and streamlining development.
- Lightweight and Fast: Designed for speed and efficiency, ASP.NET Core boasts a lightweight architecture, resulting in rapid application startup times and improved performance.
- Built-in Security Features: ASP.NET Core incorporates robust security features, including cross-site scripting (XSS) protection, cross-site request forgery (CSRF) prevention, and authentication and authorization mechanisms, ensuring the security of web applications.
A strong foundation in ASP.NET Core principles is essential for effective application development. To illustrate, consider the development of an e-commerce platform using ASP.NET Core. The platform would utilize ASP.NET Core's MVC (Model-View-Controller) architecture, separating concerns into distinct layers: the model responsible for data handling, the view handling the user interface, and the controller managing interactions between the model and view. This modular approach enhances code organization and maintainability, facilitating collaboration among developers.
Moreover, ASP.NET Core's dependency injection system enables the easy management of dependencies, improving code reusability and simplifying testing. For instance, in the e-commerce platform, the dependency injection system could be used to inject a database context into controllers and services, allowing them to access and manipulate data without needing to manually create or manage database connections.
The success of ASP.NET Core can be attributed to its versatility. It is employed across various industries, from e-commerce and finance to healthcare and education. Notable case studies include:
- Stack Overflow: Stack Overflow, a renowned online community for programmers, leverages ASP.NET Core to power its platform, showcasing its scalability and resilience in handling massive traffic.
- Microsoft Azure: Microsoft Azure, a cloud computing platform, utilizes ASP.NET Core extensively, demonstrating its ability to build complex, distributed applications.
Building Secure ASP.NET Core Applications
Security is paramount in modern web application development. ASP.NET Core provides a comprehensive set of security features, enabling developers to protect applications from vulnerabilities and threats. Key aspects of secure ASP.NET Core development include:
- Authentication and Authorization: ASP.NET Core offers built-in authentication and authorization mechanisms, allowing developers to control user access to resources based on roles and permissions. These mechanisms leverage industry-standard protocols like OAuth 2.0 and OpenID Connect, simplifying the integration of external identity providers.
- Input Validation and Sanitization: Preventing malicious input is crucial for application security. ASP.NET Core provides tools for input validation and sanitization, ensuring that user input is safe and sanitized before processing.
- Cross-Site Request Forgery (CSRF) Protection: CSRF attacks exploit user trust to perform unauthorized actions on their behalf. ASP.NET Core includes built-in CSRF protection mechanisms, making it difficult for attackers to carry out such attacks.
- Cross-Site Scripting (XSS) Prevention: XSS attacks involve injecting malicious scripts into websites to steal user data or disrupt functionality. ASP.NET Core employs XSS prevention techniques to escape or encode user input, mitigating the risk of such attacks.
Secure ASP.NET Core development requires a proactive approach, adopting best practices to mitigate risks. For example, when building a web application that handles sensitive user data, it's imperative to use strong encryption to protect data both in transit and at rest. This includes implementing HTTPS (Hypertext Transfer Protocol Secure) for secure communication between the client and server and using robust encryption algorithms to safeguard data stored in databases or other storage systems.
The importance of security cannot be overstated. Statistics highlight the rising threat of cyberattacks. The Ponemon Institute reported that the average cost of a data breach in reached $4.24 million. By implementing robust security measures, organizations can protect their assets and minimize the risk of costly breaches. Case studies demonstrate the effectiveness of secure ASP.NET Core development:
- PayPal: PayPal, a leading online payment processor, uses ASP.NET Core to power its platform. PayPal's robust security measures have earned it the trust of millions of users and businesses worldwide, showcasing the effectiveness of ASP.NET Core in securing sensitive financial transactions.
- Dropbox: Dropbox, a popular cloud storage service, relies on ASP.NET Core to ensure the security of its users' data. Dropbox's commitment to data security has fostered user confidence and contributed to its success.
Working with Data in ASP.NET Core
Data is the lifeblood of web applications. ASP.NET Core provides a rich set of tools and libraries for working with data, enabling developers to store, retrieve, and manipulate data efficiently. Core data access methods in ASP.NET Core include:
- Entity Framework Core: Entity Framework Core (EF Core) is an object-relational mapper (ORM) that simplifies data access by providing an object-oriented approach to interacting with relational databases. EF Core allows developers to define database entities as classes, enabling seamless interaction with data through these classes.
- ADO.NET: ADO.NET is a low-level data access technology that provides direct access to databases through connections and commands. While more complex than EF Core, ADO.NET offers finer-grained control over data access, suitable for scenarios requiring specific database interactions.
Choosing the right data access technology depends on project requirements and developer preferences. For projects requiring a simplified, object-oriented approach to data access, EF Core is often the preferred choice. Conversely, projects requiring more granular control or compatibility with specific database features may opt for ADO.NET.
To illustrate, consider a blog application built using ASP.NET Core. The application would need to store blog posts, comments, and user information. EF Core could be used to create entities for blog posts, comments, and users, mapping these entities to tables in a relational database. This simplifies data access, allowing developers to easily create, read, update, and delete blog posts and user data. Alternatively, if the application requires specific database features or optimizations, ADO.NET could be utilized to handle data access directly.
Effective data management is crucial for application performance. Case studies demonstrate how ASP.NET Core facilitates efficient data access:
- GitHub: GitHub, a code hosting platform, employs ASP.NET Core to manage a vast amount of data, including repositories, commits, and user profiles. GitHub's performance and scalability are a testament to ASP.NET Core's ability to handle large-scale data operations efficiently.
- Amazon.com: Amazon.com, a global e-commerce giant, relies on ASP.NET Core for its online store, showcasing its capacity to manage millions of products and customer orders with speed and reliability.
Building Responsive User Interfaces with ASP.NET Core
User experience is a cornerstone of successful web applications. ASP.NET Core offers a variety of options for building responsive and engaging user interfaces, catering to diverse user needs and preferences.
- Razor Pages: Razor Pages provide a simpler and more structured approach to building web pages. With Razor Pages, each page corresponds to a class, allowing for better organization and code maintainability.
- MVC (Model-View-Controller): MVC is a well-established architectural pattern that separates concerns into distinct layers: the model, the view, and the controller. MVC provides a robust framework for building complex web applications.
- Blazor: Blazor, a modern UI framework, enables developers to build interactive web UIs using C. Blazor supports both client-side and server-side rendering, offering flexibility and performance optimization.
The choice of UI framework depends on project complexity and developer preferences. Razor Pages are suitable for simpler applications with straightforward UI structures. MVC is a powerful choice for complex applications requiring extensive logic and functionality. Blazor offers a modern approach to building interactive web UIs with C.
To illustrate, consider an online learning platform built with ASP.NET Core. The platform might utilize Razor Pages for simple pages like course listings or user profiles. For more complex features, such as course enrollment or video playback, MVC could be employed to handle the logic and data interaction. Alternatively, Blazor could be used to create interactive elements, such as quizzes or forums, providing a dynamic and engaging user experience.
User experience is a crucial factor in application success. Research indicates that users are more likely to abandon websites with slow loading times or poor navigation. ASP.NET Core offers tools and techniques to enhance user experience, contributing to increased engagement and satisfaction. Case studies highlight the effectiveness of ASP.NET Core in building user-friendly web applications:
- LinkedIn: LinkedIn, a professional networking platform, uses ASP.NET Core to power its website, demonstrating its ability to create a user-friendly and engaging interface for its millions of users.
- Microsoft Teams: Microsoft Teams, a collaboration platform, leverages ASP.NET Core to deliver a seamless and interactive user experience for communication and teamwork.
Deploying ASP.NET Core Applications
Once an ASP.NET Core application is developed, it needs to be deployed to a web server to be accessible to users. ASP.NET Core offers flexibility in deployment options, accommodating various hosting environments.
- Self-Hosting: ASP.NET Core applications can be self-hosted within a process, giving developers granular control over the hosting environment. This option is suitable for scenarios requiring specific configuration or customization.
- IIS (Internet Information Services): IIS is a popular web server for Windows-based platforms, offering robust features and a familiar environment for developers. ASP.NET Core applications can be seamlessly deployed to IIS.
- Azure App Service: Azure App Service is a cloud-based platform that provides a scalable and managed environment for hosting ASP.NET Core applications. App Service offers automatic scaling, load balancing, and other features, simplifying deployment and management.
The choice of deployment environment depends on project requirements and resource availability. Self-hosting offers maximum control but requires managing the hosting infrastructure. IIS is a reliable option for Windows-based applications. Azure App Service provides a managed and scalable cloud environment.
Deploying applications effectively is crucial for scalability and availability. Performance issues or deployment errors can negatively impact user experience. ASP.NET Core's deployment options and tools streamline the process, ensuring smooth transitions to production environments. Case studies illustrate the effectiveness of ASP.NET Core deployment solutions:
- Netflix: Netflix, a global streaming service, leverages ASP.NET Core to power its platform, showcasing its ability to scale its application to serve millions of users worldwide.
- GoDaddy: GoDaddy, a leading web hosting provider, employs ASP.NET Core to host its website and services, demonstrating its robustness and scalability in managing a large user base and complex applications.
Conclusion
ASP.NET Core empowers developers to build exceptional web applications. From its robust security features and efficient data access mechanisms to its diverse UI frameworks and flexible deployment options, ASP.NET Core provides a comprehensive and modern development platform. By mastering its capabilities and leveraging its tools effectively, developers can build robust, scalable, and user-friendly web applications that meet the evolving demands of the digital landscape. The future of ASP.NET Core holds exciting possibilities, with ongoing advancements in performance, security, and user experience. As the web development landscape continues to evolve, ASP.NET Core will remain a powerful and versatile framework, enabling developers to create innovative and impactful web applications.