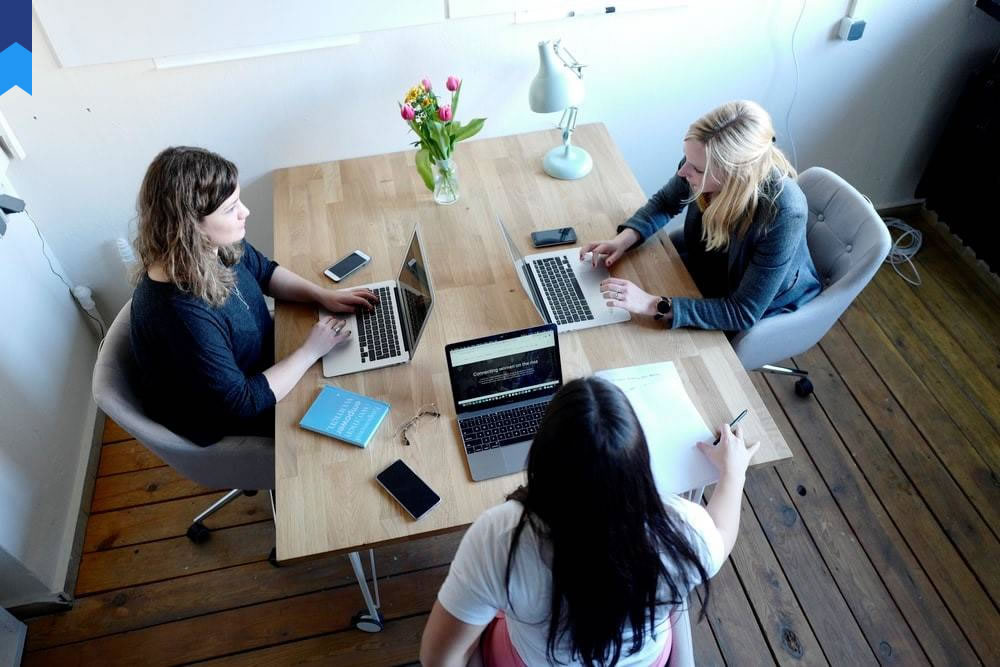
Mastering Assembly Language: A Comprehensive Guide To Memory Management
Introduction
Assembly language, a low-level programming language, offers unparalleled control over a computer's hardware. Understanding memory management in assembly is crucial for optimizing performance, writing efficient code, and developing a deep understanding of computer architecture. This guide delves into the intricacies of memory management within the context of assembly language, covering essential concepts such as segmentation, paging, stack manipulation, and heap allocation. We'll explore practical examples, real-world case studies, and current trends shaping the landscape of assembly language programming. Through this exploration, you’ll gain a robust foundation in efficiently managing memory resources in assembly language. This knowledge is essential for tasks ranging from embedded systems development to advanced system programming.
Understanding Memory Segmentation
Memory segmentation divides the computer's memory into logical blocks called segments. Each segment has a base address and a limit, defining its starting point and size. This approach simplifies memory management, allowing programs to be loaded into non-contiguous memory locations. Consider a program needing 64KB of memory. Instead of allocating a single contiguous block, segmentation can divide it into smaller segments, which can be loaded into separate, available memory regions. Case Study 1: Early DOS operating systems heavily relied on segmentation for managing memory. Case Study 2: Many embedded systems still utilize segmentation to efficiently utilize limited memory resources. A key advantage is that segments can be protected individually, improving system stability. The segmented memory model can add complexity when accessing data across segments, requiring segment selectors. However, this technique remains a crucial element in comprehending how assembly language interacts with memory.
Exploring Memory Paging
Paging is an advanced memory management technique that divides both physical and virtual memory into fixed-size blocks called pages. It addresses the limitations of segmentation by providing a more flexible and efficient memory allocation mechanism. Virtual memory allows programs to use more memory than physically available, utilizing a swap space on the hard drive. Case Study 1: Modern operating systems, such as Windows and Linux, extensively use paging for robust memory management. Case Study 2: Virtual memory, a key component enabled by paging, allows programs to run even if the available physical RAM is less than the program's memory requirements. A challenge with paging is the overhead associated with page table management. The translation lookaside buffer (TLB) significantly mitigates this overhead by caching frequently used page table entries. Understanding the intricacies of page tables and the TLB is crucial for optimizing performance in assembly.
Stack Manipulation in Assembly
The stack is a crucial data structure used for managing function calls, local variables, and temporary data. In assembly, the stack grows downwards (towards lower memory addresses). Functions typically push their parameters onto the stack, and then retrieve them after the function completes. Case Study 1: The function call mechanism in C and C++ relies heavily on the stack. Case Study 2: Exception handling in many operating systems uses the stack to store context information. Efficient stack management is essential to prevent stack overflows. Pushing large amounts of data onto the stack can lead to crashes. Therefore, careful attention should be paid to stack size limits and the appropriate use of stack-based variables. This prevents overwriting critical memory regions, which is crucial for maintaining program integrity and stability. Moreover, understanding the stack frame layout is vital for debugging and working with debuggers.
Heap Allocation and Dynamic Memory
The heap is a region of memory used for dynamically allocating memory during program execution. Unlike stack memory, heap memory is allocated and deallocated explicitly using functions like `malloc` and `free` (or their assembly language equivalents). This flexibility allows programs to adjust their memory usage as needed. Case Study 1: Data structures like linked lists and trees use heap allocation to dynamically grow and shrink their size. Case Study 2: Operating system kernel modules often dynamically allocate memory for managing device drivers and other system components. Memory leaks are a common issue when using the heap; failing to release allocated memory can lead to resource exhaustion. Best practices involve careful allocation and deallocation strategies and the use of tools to detect memory leaks. Garbage collection is an advanced approach to automatic memory management, simplifying heap management but potentially impacting performance.
Conclusion
Mastering memory management in assembly language is a critical skill for any programmer seeking to optimize performance and understand the intricacies of computer architecture. Through segmentation, paging, stack manipulation, and heap allocation, we've explored how assembly code interacts with memory. Real-world examples and case studies showcased the practical applications of these techniques. By understanding memory management principles and applying best practices, developers can create more efficient and robust assembly language programs. This expertise is highly relevant for areas like embedded systems, low-level programming, and operating system development. Continued learning and the adoption of best practices will ensure that future programs leverage memory resources effectively and efficiently.