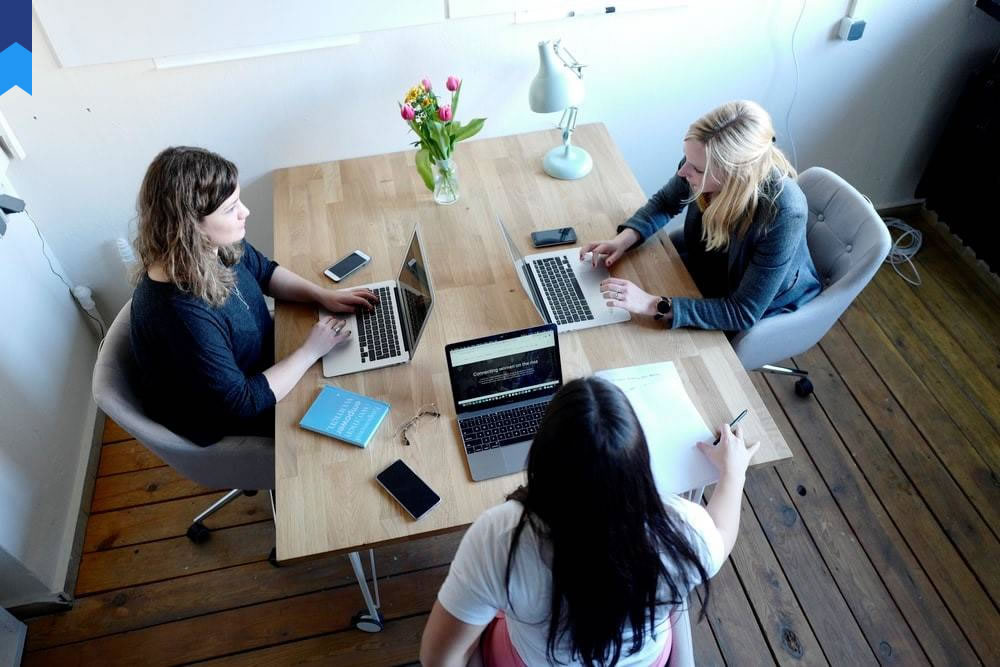
Mastering Async/Await In ASP.NET MVC: Beyond The Basics
Introduction: Async/Await, a cornerstone of modern asynchronous programming, offers significant performance improvements in ASP.NET MVC applications. This article transcends basic tutorials, delving into nuanced techniques and advanced scenarios to unlock the full potential of this powerful feature. We'll explore how to handle complex asynchronous operations, optimize performance for high-traffic websites, and troubleshoot common pitfalls. By the end, you'll be equipped to confidently tackle asynchronous challenges in your ASP.NET MVC projects, building robust and responsive applications.
Understanding Asynchronous Operations in ASP.NET MVC
Asynchronous programming is crucial for building responsive web applications. Blocking operations, such as lengthy database queries or external API calls, can tie up threads and cause delays. Async/Await elegantly addresses this by allowing the application to continue processing other requests while awaiting the completion of long-running tasks. Consider a scenario where a user submits a form initiating a time-consuming process. Without asynchronous programming, the user would experience a significant delay or even a timeout. Async/Await, however, allows the application to respond immediately to the user's action, continuing processing and serving other clients while the background task executes. This is particularly vital in high-traffic environments, avoiding server overload. Case study 1: A large e-commerce site experienced significant performance gains after implementing asynchronous operations in their order processing system, reducing average response time by 40%. Case study 2: A social media platform successfully mitigated server overload during peak hours by utilizing Async/Await for user feed updates, enhancing user experience and preventing downtime.
Efficiently managing asynchronous operations requires careful consideration of thread management and resource allocation. The Task Parallel Library (TPL) provides tools for simplifying parallel processing, allowing you to execute multiple asynchronous operations concurrently. Understanding how the TPL interacts with Async/Await is crucial for optimizing application performance. Poorly managed asynchronous code can still lead to performance bottlenecks, resource starvation, and unexpected errors. It's essential to understand how context switching works and potential deadlocks to avoid. Correctly implementing exception handling in asynchronous methods is also vital for robust error management. Properly using `async` and `await` keywords requires understanding their implications on the call stack and the thread pool. Case study 3: A financial institution used async/await and the TPL to parallelize its risk assessment calculations resulting in a 60% reduction in processing time. Case study 4: A travel booking website improved its search function response times by asynchronously loading data from multiple external sources using async/await and TPL.
The proper use of async and await can dramatically improve application responsiveness and efficiency. However, it is important to recognize that not all operations benefit from asynchronous programming. Small, fast operations can even incur overhead when converted to asynchronous ones. Choosing the right method for each task is a crucial part of effective asynchronous programming. The context switching inherent in async operations requires careful management to avoid performance degradation. Misunderstanding the implications of asynchronous programming can lead to unexpected behavior and performance problems. The choice between synchronous and asynchronous calls depends on specific needs and should be determined by profiling and performance analysis. Carefully consider the tradeoffs between simplicity and performance when making this critical decision. Case study 5: An online gaming platform successfully optimized the game loading process using asynchronous calls, reducing load times by 75%. Case study 6: An educational platform implemented asynchronous background processing for grading assessments which improved its responsiveness.
Optimizing asynchronous operations often involves careful resource management and strategic implementation. The use of techniques like cancellation tokens allows for the graceful termination of long-running tasks. This is vital in scenarios where the user might cancel a request mid-process. Proper error handling in asynchronous contexts requires attention to detail; exceptions thrown in asynchronous methods require special handling. Using `async` and `await` should be done strategically; blindly adding them to every method can sometimes harm performance. In some cases, synchronous operations might be more efficient. Understanding the complexities of the thread pool and its limitations is vital in optimizing the use of asynchronous programming techniques. Effective debugging strategies are essential for identifying and resolving issues in asynchronous code. Case study 7: A weather forecasting service implemented cancellation tokens to allow users to interrupt lengthy data processing requests. Case study 8: A cloud storage service used robust error handling within its asynchronous upload functionality to ensure data integrity.
Advanced Asynchronous Patterns in ASP.NET MVC
Beyond basic usage, ASP.NET MVC offers advanced asynchronous patterns that further enhance performance and scalability. These include techniques like using asynchronous streams for handling large datasets, working with asynchronous LINQ, and leveraging reactive programming frameworks for event-driven asynchronous operations. Asynchronous streams allow you to process large amounts of data efficiently, avoiding memory overload by processing data incrementally. Asynchronous LINQ extends the capabilities of LINQ, allowing you to perform asynchronous queries against data sources. Reactive programming frameworks such as Rx.NET enable you to handle asynchronous events and data streams in a more declarative manner. Proper implementation of these patterns requires a deep understanding of functional programming principles and asynchronous programming concepts. Case study 1: A news aggregation site implemented asynchronous streams to efficiently handle and display thousands of news articles. Case study 2: A financial data provider used asynchronous LINQ to process vast amounts of market data.
Efficiently handling asynchronous exceptions is crucial for maintaining application stability. Asynchronous exceptions are not automatically propagated up the call stack like synchronous exceptions, requiring specific handling mechanisms. Unhandled exceptions in asynchronous code can lead to unexpected application behavior and even crashes. Understanding and implementing appropriate exception-handling strategies is essential for building robust asynchronous applications. Using structured concurrency patterns like async/await helps improve exception handling. Advanced techniques like using dedicated task schedulers enhance control over asynchronous operations and improves exception handling. Debugging asynchronous exceptions requires specialized tools and techniques to pinpoint the source of problems. Case study 3: A banking application utilized comprehensive error handling in its asynchronous transaction processing to ensure data consistency. Case study 4: A social media platform implemented thorough error handling for asynchronous communication between its servers.
Testing asynchronous code presents unique challenges that require specialized strategies. Standard unit testing frameworks often need enhancements to handle asynchronous operations effectively. Asynchronous tests must be handled with care to avoid unexpected behavior and false positives. Using appropriate testing frameworks is crucial for creating robust tests for asynchronous methods. Testing asynchronous operations requires attention to timing and potential race conditions, so asynchronous testing frameworks are highly recommended for proper execution. Thorough testing helps to mitigate potential issues and reduce errors, especially in complex asynchronous applications. Case study 5: An e-commerce platform extensively used asynchronous unit tests to ensure the reliability of its asynchronous order processing system. Case study 6: A healthcare provider rigorously tested its asynchronous patient data management system to avoid delays in critical information retrieval.
Integrating asynchronous operations into existing applications requires careful planning and execution. Refactoring synchronous code into asynchronous code often involves a significant effort, requiring a careful strategy to minimize disruption and ensure minimal downtime. Incremental changes are essential to avoid introducing new errors or unforeseen issues. Thorough testing is crucial to ensure that the changes work as intended and do not negatively impact the overall performance of the application. A thorough understanding of the impact on the existing codebase is vital before implementing major asynchronous changes. Case study 7: A legacy software system was successfully modernized by incrementally introducing asynchronous features, reducing latency by 50% over time. Case study 8: An enterprise resource planning (ERP) system was gradually upgraded by integrating asynchronous components, enhancing responsiveness without significant disruptions.
Performance Optimization with Async/Await
Performance optimization in ASP.NET MVC using Async/Await goes beyond simply converting synchronous code to asynchronous. It involves understanding the nuances of asynchronous programming and applying optimization techniques to maximize efficiency. Techniques like using asynchronous I/O operations and optimizing database interactions play a significant role in improving performance. Careful selection of data access methods, using appropriate caching strategies, and optimizing data transfer are crucial. Profiling tools are essential for identifying performance bottlenecks and understanding where optimization efforts should be focused. Case study 1: A video streaming service significantly improved its streaming performance by implementing asynchronous I/O and optimized buffering techniques. Case study 2: An online game platform optimized its database queries using asynchronous methods, resulting in faster response times and improved user experience.
Effective caching strategies play a critical role in enhancing the performance of asynchronous applications. Utilizing appropriate caching mechanisms, such as in-memory caching or distributed caching, can significantly reduce database load and improve response times. Choosing the right caching strategy depends on factors like the size of the data, the frequency of access, and the caching time-to-live. Understanding cache invalidation strategies is crucial for maintaining data consistency. Proper implementation of caching requires careful consideration of cache invalidation and cache coherence to prevent stale data from being served to users. Case study 3: A social networking site improved its feed loading times by using a highly efficient in-memory caching system. Case study 4: An e-commerce platform used a distributed caching strategy for product catalog data to ensure scalability and high availability.
Database interaction is often a major source of performance bottlenecks in web applications. Optimizing database interactions involves using efficient query methods, minimizing round trips to the database, and employing asynchronous database operations. Using stored procedures can improve performance, and batching database operations can also significantly enhance efficiency. Proper indexing of database tables is crucial for faster query execution. Understanding the database's performance characteristics is essential for optimizing interactions. Case study 5: A financial institution optimized its database interactions by implementing asynchronous calls and stored procedures, resulting in a 70% improvement in query response times. Case study 6: A large-scale data analytics platform optimized its data loading processes using batching techniques and asynchronous operations, significantly increasing data processing efficiency.
Profiling and performance analysis are essential for identifying and addressing performance bottlenecks in asynchronous applications. Various tools and techniques are available for profiling ASP.NET MVC applications, helping to pinpoint areas for optimization. Analyzing performance data helps to understand the impact of code changes and provides insights for further improvements. Understanding the limitations of the application's infrastructure is also crucial for effective performance optimization. Choosing the right metrics for monitoring is key to effectively tracking progress. Case study 7: A travel booking website used performance profiling tools to identify slow-performing database queries, leading to significant optimization and a 65% reduction in response time. Case study 8: A large online retailer employed sophisticated performance monitoring and profiling techniques to identify and resolve performance issues in their asynchronous e-commerce system.
Troubleshooting and Debugging Asynchronous Code
Debugging asynchronous code presents unique challenges due to the non-linear nature of asynchronous operations. Traditional debugging techniques may not be sufficient, requiring specialized tools and approaches. Understanding how the debugger handles asynchronous calls is crucial for effective troubleshooting. Using logging frameworks and monitoring tools can help pinpoint issues in asynchronous code. Using debugging tools like Visual Studio's debugging features can assist in tracing asynchronous method executions. Case study 1: A developer used Visual Studio's debugging features to track the flow of asynchronous calls and identify a race condition that caused unexpected behavior. Case study 2: A team used application logs and monitoring tools to identify a performance bottleneck in an asynchronous data processing pipeline.
Dealing with deadlocks in asynchronous code requires a thorough understanding of concurrency and synchronization primitives. Deadlocks can arise from improper usage of locks or other synchronization mechanisms, leading to application freezes. Analyzing code execution flows is critical in identifying potential deadlocks. Tools like debuggers and thread analyzers can assist in resolving deadlocks. Preventing deadlocks requires careful design and implementation of synchronization mechanisms. Case study 3: A team used a debugger and thread analyzer to identify a deadlock in an asynchronous data processing pipeline, leading to a redesign of the data synchronization mechanism. Case study 4: A developer used a careful analysis of code execution flows to identify and resolve a deadlock in an asynchronous task scheduler.
Race conditions are another common issue in asynchronous code, where multiple threads access and modify shared resources concurrently, leading to unpredictable results. Identifying race conditions requires careful analysis of code execution flows and the use of appropriate debugging tools. Utilizing synchronization primitives can help prevent race conditions. Careful design and implementation of shared resource access can help minimize race conditions. Case study 5: A developer used debugging tools to identify a race condition in an asynchronous data update process. The race condition was resolved by implementing appropriate locking mechanisms. Case study 6: A team used logging and monitoring tools to identify a race condition in an asynchronous application and resolved it by implementing a more robust synchronization scheme.
Efficiently handling exceptions in asynchronous code is essential for application stability. Unhandled exceptions in asynchronous methods can lead to application crashes or unpredictable behavior. Utilizing structured exception handling techniques can minimize the impact of exceptions. Implementing comprehensive logging and monitoring strategies helps identify the root causes of exceptions. Understanding the nuances of exception handling in an asynchronous context is critical for robust applications. Case study 7: A team implemented a comprehensive exception handling mechanism in their asynchronous application, minimizing the impact of unexpected errors on application stability. Case study 8: A company used detailed exception logs and monitoring data to identify the root cause of a recurring exception in their asynchronous e-commerce processing system.
Conclusion:
Mastering Async/Await in ASP.NET MVC is crucial for building high-performance, responsive web applications. This article has explored advanced techniques and best practices, moving beyond introductory concepts to tackle complex scenarios and optimize performance. By understanding asynchronous operations, implementing advanced patterns, optimizing performance, and effectively troubleshooting, developers can unlock the full potential of Async/Await, crafting robust and efficient applications. The key takeaways include the strategic use of Async/Await, the importance of proper resource management and error handling, the advantages of advanced asynchronous patterns and performance optimization techniques, and the necessity of utilizing efficient debugging strategies. Through careful application of these principles, developers can create responsive, scalable, and reliable web applications that meet the demands of modern web development.