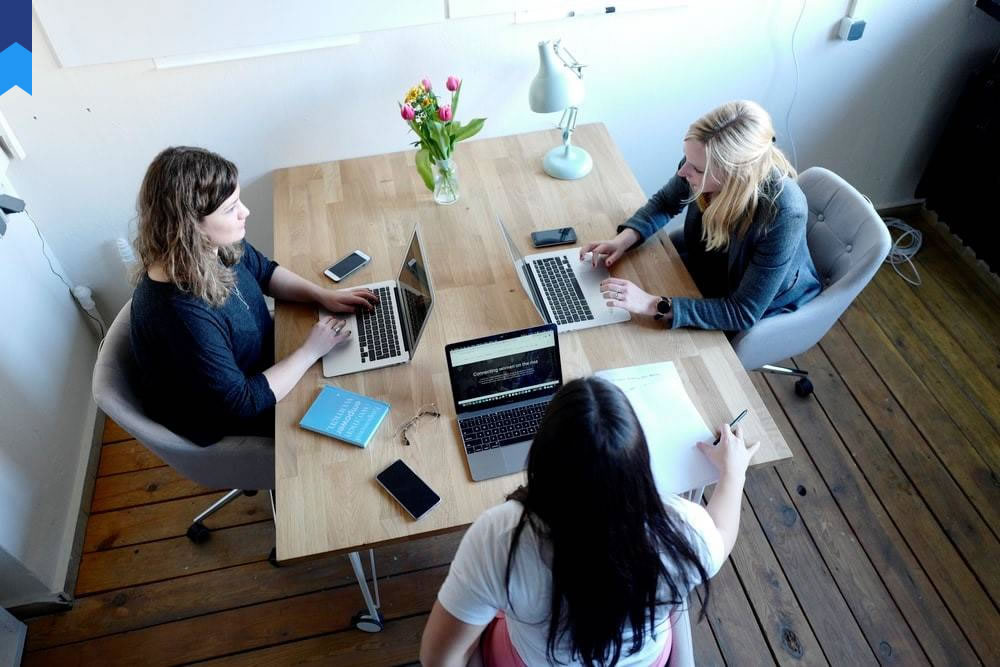
Mastering Asynchronous Programming In Dart: A Comprehensive Guide
Introduction
Dart, Google's versatile programming language, excels in building cross-platform applications. Its asynchronous programming capabilities are crucial for handling I/O-bound operations efficiently, preventing UI freezes, and ensuring responsiveness. Understanding asynchronous programming is vital for any Dart developer, whether building mobile apps with Flutter, server-side applications, or command-line tools. This guide will delve into the core concepts of asynchronous programming in Dart, exploring various methods, best practices, and real-world scenarios to help you master this essential skill. We'll examine futures, async/await, streams, and error handling, providing practical examples and case studies to solidify your understanding.
Futures: Handling Single Asynchronous Operations
Futures are the fundamental building blocks of asynchronous operations in Dart. A Future represents a value that may not be available immediately but will be at some point in the future. They are typically used to handle single asynchronous tasks, such as network requests or file I/O. The Future
class provides methods like then
for handling successful results and catchError
for managing exceptions. For example, a network request might be represented by a Future
, indicating that it will eventually resolve to a string containing the response. Consider a scenario where you need to fetch user data from a remote server. A Future
would gracefully handle the asynchronous nature of this operation, preventing the application from freezing while waiting for the response. Effective error handling is crucial; a well-structured catchError
block can prevent crashes and provide graceful fallback mechanisms. Case Study 1: Imagine a weather application; fetching weather data from an API using a Future
ensures the UI remains responsive while the request is in progress. Case Study 2: A mobile game could use Futures
to load game assets asynchronously, preventing lag during startup.
Async/Await: Simplifying Asynchronous Code
While Futures provide the foundation, the async
and await
keywords dramatically improve the readability and maintainability of asynchronous code. The async
keyword marks a function as asynchronous, allowing the use of await
within its body. await
pauses execution until a Future
completes, effectively making asynchronous code look and behave like synchronous code. This significantly simplifies complex asynchronous workflows. Let's consider a scenario where you need to fetch data from multiple APIs sequentially. Using async/await
, you can write the code in a clear, linear fashion without nested callbacks. This enhances code clarity and reduces the risk of errors associated with deeply nested callbacks. Case Study 1: An e-commerce application fetching product details and customer reviews could utilize async/await
to streamline the data retrieval process, improving user experience. Case Study 2: A build system could use async/await
to execute multiple tasks concurrently, significantly reducing build times.
Streams: Handling Sequences of Asynchronous Events
Streams are used to handle sequences of asynchronous events. Unlike Futures, which represent a single asynchronous operation, Streams represent a sequence of values over time. They are ideal for scenarios involving continuous data streams, such as real-time data updates, sensor readings, or network connections. Streams provide methods for listening to new events, handling errors, and managing cancellations. Consider a chat application. The incoming messages would be represented by a Stream, allowing the app to react to new messages as they arrive without blocking the main thread. This ensures responsiveness and a smooth user experience. The flexibility of Streams allows for efficient management of various data flows. Case Study 1: A stock trading app could use Streams to update stock prices in real-time. Case Study 2: A social media feed could utilize Streams to display new posts as they are published.
Error Handling in Asynchronous Code
Robust error handling is crucial in asynchronous programming. Unhandled exceptions can lead to application crashes and unexpected behavior. Dart offers various mechanisms for handling errors in asynchronous code. try-catch
blocks can be used to catch exceptions within asynchronous functions. Futures provide the catchError
method for handling exceptions that occur during the execution of a future. Streams offer similar mechanisms for handling errors within the stream flow. It's essential to handle potential errors at each stage of an asynchronous operation to ensure application stability. Consider a scenario where a network request fails. Proper error handling would inform the user of the failure and provide a graceful fallback mechanism, rather than crashing the application. Effective error management significantly improves the robustness and reliability of applications. Case Study 1: An online banking app must carefully handle network errors and data validation to ensure security and prevent data loss. Case Study 2: A weather forecast application should gracefully handle API errors, possibly displaying a default message or fallback data.
Conclusion
Mastering asynchronous programming in Dart is essential for building high-performance and responsive applications. By understanding Futures, async/await, Streams, and robust error handling techniques, developers can create applications that handle I/O-bound operations efficiently and gracefully. The techniques discussed in this guide, combined with practical experience, will equip you to build sophisticated and reliable Dart applications that excel in responsiveness and user experience. Asynchronous programming is not merely a technical detail; it is a fundamental aspect of building modern applications, and its mastery significantly impacts the overall quality and performance of your work. Further exploration of advanced concepts, such as isolates for parallel processing, will further enhance your skills and enable the creation of even more efficient and robust applications.