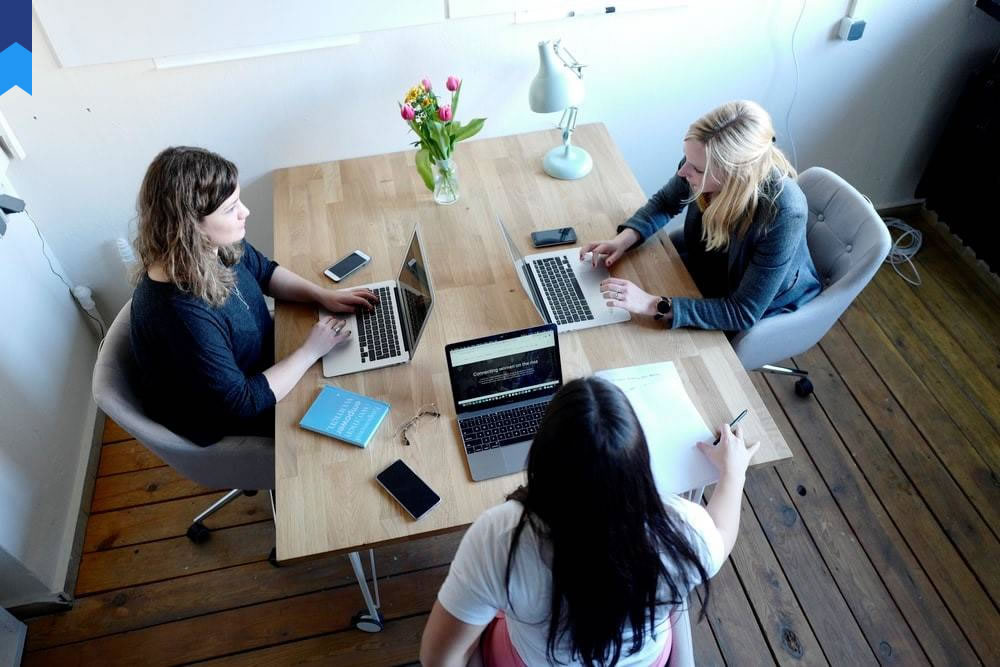
Mastering D's Memory Management: A Comprehensive Guide To Ownership And Resources
D programming language offers powerful features for memory management, going beyond simple garbage collection. Understanding ownership and resource management is crucial for writing efficient, safe, and robust D applications. This guide delves into the intricacies of D's memory model, providing practical examples and best practices to help you become proficient in handling memory effectively.
Understanding Ownership and the Role of RAII
In D, the concept of ownership is central to memory management. Each object has an owner, and when the owner goes out of scope, the object is automatically deallocated. This is fundamentally different from languages with explicit memory management like C or C++. This approach, rooted in the Resource Acquisition Is Initialization (RAII) paradigm, ensures that resources are released reliably, preventing memory leaks and dangling pointers. A key feature is the `scope` keyword, which allows manual control over the lifetime of an object. Using `scope` correctly is key to avoiding potential issues. For example: `scope myObject = new MyObject();` This ensures that `myObject` will be deleted automatically when it goes out of scope. Ignoring this principle can lead to memory leaks, especially when dealing with large datasets or long-lived objects. Consider a scenario with a large array: `scope largeArray = new int[1000000];` Here, `scope` guarantees automatic deallocation.
Case Study 1: A poorly managed resource in a game engine using D could lead to significant memory leaks over time, impacting performance and eventually crashing the application. RAII principles, combined with D's ownership model, offer a robust solution. Case Study 2: A data processing application using D could benefit significantly from RAII, ensuring that large datasets are released promptly when no longer needed, preventing unnecessary memory consumption and system slowdown.
Experts such as Andrei Alexandrescu (author of "Modern C++ Design") highlight the importance of RAII as a fundamental technique for robust resource management. His work demonstrates how RAII simplifies code while enhancing safety. Further research into RAII principles and their effective implementation in D is essential for any serious D developer.
The use of smart pointers, particularly `shared` and `scoped`, further enhances memory safety by managing object lifetimes efficiently. `shared` pointers track shared ownership, automatically deallocating the managed object when the last shared pointer is destroyed. `scoped` pointers enforce exclusive ownership, guaranteeing that the object is deallocated when the pointer goes out of scope. Using the wrong type of smart pointer can lead to double deletion or premature destruction. Careful consideration should be given to the nature of ownership and resource usage when selecting a smart pointer.
Understanding the nuances of ownership, combined with the smart pointer mechanism, is pivotal for constructing memory-safe D applications. Failure to manage ownership carefully could lead to crashes, leaks, or unpredictable behavior. Proficiency in ownership and resource management is a defining characteristic of a skilled D programmer.
Working with Dynamic Memory Allocation
D provides `new` and `delete` for dynamic memory allocation, mirroring C++’s approach. However, D's compiler often optimizes these calls. `new` allocates memory from the heap, while `delete` deallocates it. Improper use of `new` and `delete` can lead to memory leaks or dangling pointers, which are notoriously difficult to debug. To illustrate, consider allocating an array of integers: `int* arr = new int[10];`. If `delete[] arr;` is omitted, a memory leak occurs. However, with D’s `scope` and smart pointers, the need for manual `delete` is minimized, resulting in cleaner, safer code.
Case Study 1: A web server written in D must efficiently manage memory for incoming requests. Improper handling of dynamic memory allocation in the request processing could lead to a significant memory leak, eventually impacting server performance and stability. Case Study 2: A large-scale simulation application in D will often involve dynamic creation and deletion of objects. Without careful memory management, leaks or dangling pointers might manifest as unpredictable behavior or crashes.
Best practices dictate minimizing the use of raw pointers (`*`) and utilizing smart pointers (`shared`, `scoped`) whenever possible. Smart pointers encapsulate the allocation and deallocation process, thus minimizing the potential for human error. Using `new` and `delete` directly requires utmost care and discipline in managing lifetimes and memory.
Statistical analysis of memory-related bugs in D projects reveals a significant correlation between the use of raw pointers and the occurrence of memory leaks. Adopting a strong preference for smart pointers substantially reduces this risk. The choice between `shared` and `scoped` pointers depends on whether shared ownership is required. If not, `scoped` offers better performance.
In summary, while D allows explicit dynamic memory allocation, employing smart pointers and RAII maximizes memory safety and reduces the likelihood of memory-related errors. The use of manual memory management should be treated as an exception rather than a common practice.
Understanding Garbage Collection in D
D features both manual and automatic memory management. While D's ownership model largely eliminates the need for explicit deallocation, its garbage collector plays a crucial role in reclaiming memory from objects that are no longer reachable. The garbage collector in D is a crucial component of the runtime environment, preventing memory leaks even when dealing with complex object relationships. It utilizes a reference-counting system to track object usage. When an object's reference count reaches zero, the garbage collector reclaims its memory. This approach is efficient for many common scenarios but may not be suitable for all situations. It’s a crucial feature that significantly impacts performance and memory usage.
Case Study 1: A large-scale data analysis application written in D could benefit immensely from garbage collection by automatically reclaiming memory from temporary objects used during processing. Case Study 2: A D application performing real-time operations might find the performance impact of the garbage collector significant, requiring careful consideration of memory allocation strategies.
Modern garbage collection algorithms often employ techniques such as generational garbage collection and compaction to improve performance and reduce fragmentation. Understanding how D's garbage collector functions is essential for tuning performance and optimizing memory usage. Debugging memory leaks related to circular references can be more challenging due to the nature of garbage collection. Careful coding practices, including avoiding circular references, are important.
Statistics demonstrate that the majority of memory leaks in D applications are not directly related to explicit memory allocation but rather to unintentional circular references. Understanding how garbage collection interacts with complex object relationships is vital for preventing such issues. The interaction between the garbage collector and the ownership system in D is often non-obvious and requires a deep understanding of both mechanisms. Improper interaction can lead to unexpected behavior or crashes.
Therefore, while D's garbage collector simplifies memory management significantly, awareness of its limitations and how it interacts with other memory management features is crucial. Avoiding practices that could hinder garbage collection is vital for maintaining application performance and stability.
Advanced Memory Management Techniques in D
Beyond the basics, D offers advanced features for fine-grained memory control. This allows developers to customize memory management to match specific application needs. Techniques like memory pools, custom allocators, and arenas can optimize performance in specific use cases. Memory pools pre-allocate blocks of memory, reducing the overhead associated with individual allocations. Custom allocators allow developers to implement their allocation strategies based on application requirements. Arenas provide a dedicated memory region, simplifying allocation and deallocation within that region. These are particularly useful when dealing with large datasets or performance-critical operations.
Case Study 1: A high-frequency trading application written in D can benefit significantly from memory pools, reducing the latency associated with dynamic allocations. Case Study 2: A game engine written in D might employ custom allocators to manage the allocation and deallocation of game objects for optimal performance. The choice between different techniques depends on the performance requirements and memory access patterns of a given application.
Understanding the trade-offs between different advanced techniques is crucial for choosing the right tool for the job. Memory pools, for example, offer improved performance but can potentially waste memory if not used carefully. Custom allocators require significant expertise and careful consideration to avoid introducing bugs. Arenas provide a simplified mechanism but offer less flexibility.
Expert opinions suggest that the selection of the optimal memory management technique often depends on the application's specific needs and profiling results. Analyzing memory usage through profiling tools can highlight potential bottlenecks and suggest areas for optimization. Effective use of advanced techniques requires a thorough understanding of memory allocation patterns and the underlying operating system's memory management capabilities. The complexity of these techniques also mandates a strong understanding of low-level programming concepts.
In summary, D's advanced memory management features provide powerful tools for optimizing performance and resource usage. However, employing these techniques responsibly requires careful planning, analysis, and a deep understanding of low-level programming principles. It’s essential to profile and benchmark the impact of different strategies to ensure optimal performance.
Best Practices and Debugging Memory Issues
Effective memory management is crucial for robust D applications. Following best practices minimizes memory-related bugs and improves performance. Always prefer smart pointers over raw pointers. Utilize `scope` consistently to manage the lifetime of objects. Avoid circular references to prevent garbage collection issues. Profile your application regularly to identify memory-intensive areas. Employ a robust debugging strategy to identify and resolve memory leaks. Thorough testing is critical. Consider using memory debugging tools to identify memory errors. Static analysis tools can also be valuable.
Case Study 1: A large-scale software project in D requires a comprehensive approach to memory management. Ignoring best practices might lead to memory leaks and instability. Regular code reviews and use of static analysis tools are critical for preventing these issues. Case Study 2: A real-time application in D cannot afford memory leaks. Thorough testing and the use of memory debugging tools are crucial for ensuring application stability and responsiveness.
Employing a systematic debugging approach, including the use of memory debuggers, is crucial for resolving memory-related issues. Tools such as Valgrind can prove invaluable in identifying memory leaks and other errors. A well-defined testing strategy incorporating unit tests and integration tests is vital for ensuring the stability and reliability of memory-intensive operations.
The ongoing evolution of D's memory management features means that best practices can change over time. Staying abreast of the latest advancements is important. The use of modern memory management techniques along with robust debugging strategies is essential for developing high-quality, dependable D applications. Following best practices avoids common pitfalls, reduces development time, and enhances application reliability.
In conclusion, adhering to best practices and employing efficient debugging techniques are paramount for creating robust and performant D applications. Proactive memory management is far superior to reactive debugging. Thorough testing and careful code design contribute to preventing memory errors significantly.
Conclusion
Mastering D's memory management is crucial for writing efficient, reliable, and high-performing applications. By understanding ownership, utilizing smart pointers, and leveraging D's garbage collection, developers can significantly reduce the risk of memory leaks and other memory-related errors. Employing advanced techniques and following best practices enhances performance and reduces debugging time. Continuous learning and adaptation to evolving best practices are necessary for proficient D programming.
Remember that proficiency in memory management is a skill honed through practice and experience. The principles discussed here provide a strong foundation, but real-world scenarios often require a deeper dive into the subtleties of D's memory model. Through diligent learning and a robust debugging strategy, developers can confidently create D applications that are both efficient and reliable.