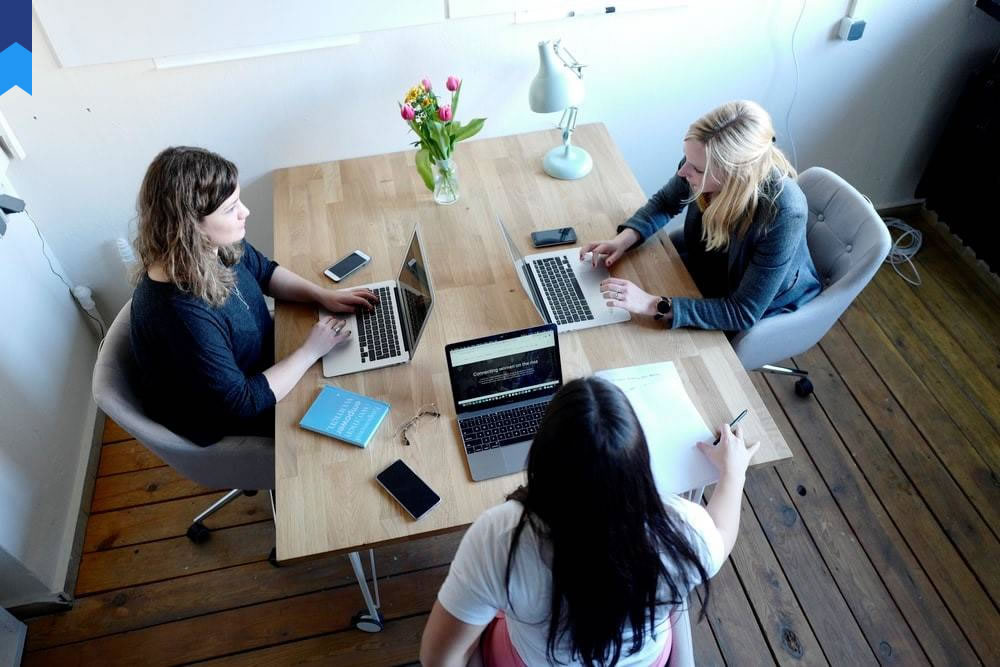
Mastering Ethereum Smart Contract Development: A Comprehensive Guide
Ethereum smart contracts are self-executing contracts with the terms of the agreement between buyer and seller being directly written into lines of code. These contracts are stored on the blockchain and automatically execute when predefined conditions are met. This eliminates the need for intermediaries and significantly streamlines various processes. This guide delves into the intricacies of developing robust and secure Ethereum smart contracts, offering a comprehensive understanding for both beginners and experienced developers.
Choosing the Right Development Environment
Selecting a suitable integrated development environment (IDE) is crucial for efficient smart contract development. Popular choices include Remix, a browser-based IDE perfect for beginners, and Truffle, a more comprehensive framework ideal for larger projects. Each IDE offers unique features and functionalities. Remix's simplicity allows for quick prototyping and testing, while Truffle’s advanced features facilitate the management of complex projects. The choice depends on the project’s complexity and the developer’s experience level. Case Study 1: A small-scale decentralized application (dApp) utilizing a simple token transfer might be perfectly suited for Remix. Case Study 2: A complex DeFi protocol requiring extensive testing and deployment strategies would greatly benefit from using Truffle's more sophisticated tools. Consider factors like debugging capabilities, extension support, and community support when making your decision. The active community surrounding Truffle provides substantial support through forums and documentation, making it a favored choice among experienced developers. The ongoing development of Remix ensures its continuous improvement, making it a viable option for those starting their smart contract development journey. Choosing the right IDE significantly impacts development speed and project success.
Beyond IDE selection, the choice of programming language significantly affects the development process. Solidity, the most popular language for Ethereum smart contract development, is known for its user-friendly syntax and extensive community support. However, other languages like Vyper, known for its security features, and Yul, a lower-level language, offer unique advantages for specific use cases. Solidity's popularity stems from its relatively simple syntax which enables faster learning for new developers. This is supported by a large and active community providing tutorials and resources. Case Study 3: A decentralized exchange (DEX) might benefit from Solidity’s flexibility for complex functionalities. Case Study 4: A critical financial application might use Vyper to benefit from its focus on security and prevent potential vulnerabilities. Understanding the trade-offs between each language is crucial for building successful Ethereum contracts. The choice between these languages will depend on project requirements and developer expertise. Each language features different security properties and levels of abstraction. The decision is best made with a deep understanding of these factors. The evolution of the Ethereum ecosystem is resulting in new languages and tools, requiring continuous adaptation and learning.
Furthermore, mastering the intricacies of the Ethereum Virtual Machine (EVM) is paramount. The EVM is a runtime environment for smart contracts, responsible for executing their code. Understanding its gas consumption model, limitations, and operational principles is critical for writing efficient and cost-effective contracts. The EVM's gas mechanism directly impacts transaction costs, emphasizing efficient code. Understanding gas optimization techniques is critical for minimizing costs and maximizing performance. Case Study 5: Inefficient code could result in high gas costs, making the contract impractical to use. Case Study 6: Optimized code can drastically reduce gas costs, making the application more efficient and accessible. The EVM's stack-based architecture presents unique challenges for developers requiring a strong understanding of data structures and algorithms. A thorough grasp of the EVM enhances the ability to write optimized and secure smart contracts. This understanding is critical for avoiding common pitfalls and optimizing contract performance.
Finally, understanding the importance of security best practices is pivotal. This involves utilizing tools like static and dynamic analysis to identify vulnerabilities. It also entails thorough testing, both unit and integration testing, to identify any flaws. Security audits are crucial, especially for high-value applications. The cost of security vulnerabilities can be extremely high, both financially and reputationally. Case Study 7: A high-profile DeFi protocol's vulnerability resulted in significant financial losses and eroded user trust. Case Study 8: A well-tested contract successfully prevented a significant exploit, protecting user funds and ensuring the protocol's stability. Employing best practices and adopting a proactive security approach is essential for building robust and dependable contracts. The security of smart contracts is paramount and requires extensive testing and audits.
Solidity Fundamentals: Data Types, Functions, and Modifiers
Mastering Solidity, the primary language for smart contract development, is crucial. This involves a deep understanding of data types, functions, and modifiers. Understanding data types is the foundation of any smart contract. Solidity offers various data types, such as integers, booleans, addresses, and arrays. Each has its specific properties and usage. Case Study 1: Choosing the wrong data type could lead to unexpected behavior or vulnerabilities. Case Study 2: Using the correct data type ensures correctness and efficiency of the smart contract. Careful selection of data types ensures precision and functionality. Mastering data types is crucial for efficient code.
Solidity functions are blocks of code that perform specific tasks. Functions can be either internal or external, and can have different parameters and return values. Understanding function visibility and modifiers is critical. Case Study 3: A function with the wrong visibility could have unexpected access control issues. Case Study 4: Correctly using modifiers improves the readability and maintainability of the contract. Functions are the building blocks of the application logic. Mastering them is necessary for building functional contracts.
Modifiers are special functions that modify the behavior of other functions. They are commonly used to implement access control, ensuring that only authorized users can interact with specific contract functions. Case Study 5: A poorly implemented access control mechanism could lead to vulnerabilities. Case Study 6: Properly using modifiers helps enforce security constraints and enhance contract integrity. Modifiers aid in refining and controlling contract functionalities. Understanding modifiers is critical for security and efficiency. They are used to control access and execute operations.
Finally, understanding inheritance and events is essential. Inheritance allows developers to create new contracts based on existing ones, promoting code reuse and efficiency. Events allow contracts to emit information, enhancing transparency and enabling applications to react to contract events. Case Study 7: Inheritance promotes modular design and code reuse. Case Study 8: Events provide a means for other applications to interact with the contract's state changes. They enhance transparency and improve real-time interaction.
Deployment and Testing: A Robust Approach
Deploying and testing smart contracts is a critical stage in the development lifecycle. This involves utilizing tools such as Truffle or Hardhat to deploy contracts to various networks, including testnets and mainnets. Rigorous testing ensures the reliability and security of the contract before deploying to production. Case Study 1: Deploying to a testnet allows developers to test their contracts in a real environment, identifying potential issues before deploying to mainnet. Case Study 2: Failing to test a contract thoroughly before deploying to mainnet could result in significant financial losses or security breaches. Thorough testing should always be prioritized.
Choosing the right deployment network is crucial. Testnets allow for cost-effective testing without the risk of losing funds, while mainnets represent the actual Ethereum network. Understanding the trade-offs between each environment is essential. Case Study 3: A developer deployed a contract to mainnet without thorough testing, resulting in significant financial losses. Case Study 4: A developer used a testnet effectively to identify bugs and vulnerabilities, leading to a more successful mainnet deployment. Choosing the right deployment environment is vital for success.
Gas optimization is a key consideration during deployment. Efficiently written code minimizes the gas costs of transactions, leading to lower fees and increased scalability. Understanding the intricacies of gas consumption and optimization techniques is critical. Case Study 5: Poorly optimized code resulted in high transaction fees, discouraging user adoption. Case Study 6: Well-optimized code significantly reduced transaction fees, leading to widespread user adoption. Efficient code is crucial for cost reduction and user satisfaction.
Finally, post-deployment monitoring and maintenance is crucial. Monitoring transaction activity, gas usage, and contract interactions helps identify potential issues or vulnerabilities. Regular updates and maintenance are necessary to ensure the long-term stability and security of the contract. Case Study 7: A lack of post-deployment monitoring resulted in a critical vulnerability being exploited. Case Study 8: Regular monitoring and maintenance detected and resolved a minor vulnerability, preventing a potential security breach. Constant monitoring and maintenance are crucial for long-term stability.
Interacting with Smart Contracts: Tools and Techniques
Interacting with deployed smart contracts requires specialized tools and techniques. Web3.js, a JavaScript library, allows developers to interact with the Ethereum blockchain and smart contracts programmatically. Using appropriate APIs and understanding the interaction patterns is vital for seamless communication. Case Study 1: Web3.js simplifies the process of interacting with contracts from various applications. Case Study 2: Using alternative methods to interact directly with the blockchain might require in-depth knowledge of Ethereum's technical specifications. Choosing the right interaction method is vital for ease of use.
Understanding transaction lifecycle is essential. From sending transactions to receiving responses and interpreting results, a clear understanding is necessary. Proper error handling and resilience mechanisms are critical for handling unexpected situations. Case Study 3: Poor error handling can cause issues in complex interactions. Case Study 4: Efficient error handling ensures a reliable user experience. Mastering the transaction lifecycle ensures smooth operation.
Utilizing different development frameworks is important, allowing developers to build front-end interfaces that interact with smart contracts. Frameworks like React, Angular, or Vue.js provide tools and structures for building robust and user-friendly interfaces. Case Study 5: React's component-based approach simplifies front-end development. Case Study 6: Using other front-end frameworks allows for diverse design and user experience. Choosing the right framework depends on the project's requirements and developer expertise.
Finally, securing the interaction layer is crucial. Implementing proper authentication and authorization mechanisms protects the application from unauthorized access and malicious activities. Secure coding practices are essential throughout the development process. Case Study 7: Unsecured interactions led to compromised accounts and funds. Case Study 8: Strong security measures prevented unauthorized access and protected user funds. Security is paramount in the interaction layer. A secure and robust system is needed to ensure successful and safe operation.
Advanced Concepts: Decentralized Autonomous Organizations (DAOs) and Beyond
Exploring advanced concepts such as Decentralized Autonomous Organizations (DAOs) provides a deeper understanding of smart contract applications. DAOs are autonomous organizations governed by smart contracts, offering new models for governance and decision-making. Case Study 1: A DAO successfully managed a community fund, demonstrating transparency and efficiency. Case Study 2: Challenges exist in DAOs regarding governance and security, highlighting the need for ongoing research and development. DAOs represent a new form of organizational structure.
Understanding oracle integration is essential for bridging the gap between blockchain and the real world. Oracles provide smart contracts with off-chain data, enabling real-world interactions. Case Study 3: Oracles are needed to integrate real-world data into blockchain applications. Case Study 4: Challenges exist in oracle security and reliability, requiring careful selection and integration. Oracles are crucial for real-world interactions.
Exploring different consensus mechanisms beyond proof-of-work (PoW) expands the range of possibilities. Proof-of-stake (PoS) and other consensus mechanisms offer alternatives with potential advantages in efficiency and scalability. Case Study 5: PoS offers a more energy-efficient approach compared to PoW. Case Study 6: Different consensus mechanisms provide varying levels of security and scalability. Choosing the right consensus mechanism is crucial for efficiency and security.
Finally, staying updated with the latest advancements in the Ethereum ecosystem is crucial. New tools, languages, and concepts constantly emerge, requiring developers to adapt and learn continuously. The Ethereum ecosystem is constantly evolving, demanding continuous learning and adaptation. Case Study 7: The development of new tools and libraries simplifies smart contract development. Case Study 8: Ongoing research and advancements improve the security and efficiency of Ethereum smart contracts. Staying updated is vital for success in this rapidly changing field.
Conclusion
Developing robust and secure Ethereum smart contracts requires a comprehensive understanding of various aspects, from choosing the right development environment and mastering Solidity fundamentals to deploying, testing, and interacting with contracts effectively. Understanding advanced concepts such as DAOs and oracles expands the possibilities of smart contract applications. Continuous learning and adaptation are crucial for success in this dynamic field. The future of smart contracts holds immense potential, promising revolutionary changes across various industries.
By mastering these core concepts and staying updated with the latest advancements, developers can create innovative and impactful applications. The potential for smart contracts to transform various industries remains vast. This necessitates a dedicated approach towards understanding the technical aspects and security implications involved in their development. The journey of mastering Ethereum smart contract development is continuous and rewarding, offering significant opportunities for innovation and growth.