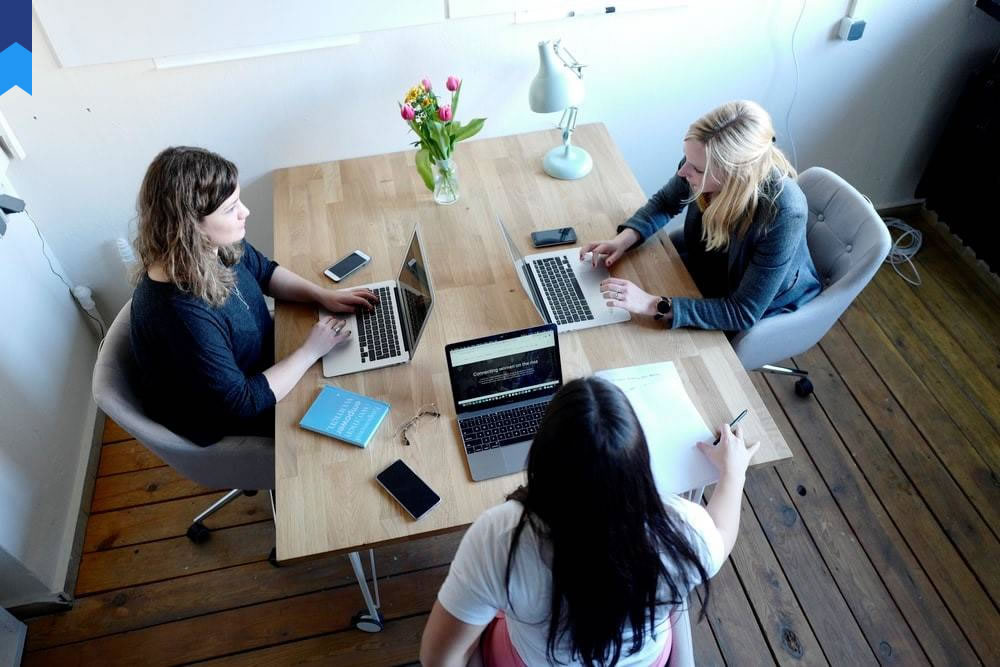
Mastering PySpark DataFrames: A Comprehensive Guide To Data Manipulation
PySpark DataFrames are a fundamental component of big data processing with PySpark. This guide provides a comprehensive walkthrough of their capabilities, focusing on efficient data manipulation techniques.
Creating and Loading DataFrames
Creating PySpark DataFrames is straightforward. You can load data from various sources like CSV files, JSON files, or even directly from databases. Let's start with loading a CSV file.
from pyspark.sql import SparkSession spark = SparkSession.builder.appName("DataFrameExample").getOrCreate() Load data from a CSV file df = spark.read.csv("path/to/your/file.csv", header=True, inferSchema=True) Display the schema df.printSchema() Show the first few rows df.show()
The inferSchema=True
option automatically infers the data types of each column. This is crucial for efficiency. Failing to infer the schema can lead to performance bottlenecks. Let's consider a case study: A large e-commerce company uses PySpark to process millions of customer transaction records daily. Using schema inference significantly reduces processing time and resource consumption. Another example, a financial institution leverages PySpark for fraud detection. Inferring the schema ensures data integrity and rapid analysis. Understanding data types is essential; misinterpreting them can lead to inaccurate analyses, especially in scenarios involving complex data.
Alternatively, you can create DataFrames from Python lists or dictionaries.
data = [("Alice", 30), ("Bob", 25), ("Charlie", 35)] columns = ["Name", "Age"] df = spark.createDataFrame(data, columns) df.show()
This approach offers flexibility, particularly for testing and prototyping. The ability to quickly create sample DataFrames is crucial in development and debugging. This is particularly valuable when working with complex transformations and aggregations. Consider a data scientist using PySpark to model customer behavior. Creating a smaller DataFrame from a subset of their data allows quick experimentation before scaling up to the full dataset. Similarly, a software engineer testing a new data processing pipeline can leverage this feature for robust validation.
Data loading performance can be significantly improved using optimized techniques like partitioning and bucketing. A properly partitioned DataFrame enables parallel processing, dramatically reducing query execution time. For instance, partitioning a DataFrame by date allows parallel processing of data for each day, improving the efficiency of time-series analyses. This is vital for real-time applications like financial market analysis and social media sentiment tracking. Another beneficial practice is to use optimized data formats like ORC or Parquet instead of CSV which greatly reduces storage size and improves query performance. ORC and Parquet formats compress data efficiently, reducing the I/O overhead. This is especially beneficial when dealing with terabytes or petabytes of data. Consider a large scale data warehousing application. Using ORC or Parquet reduces storage costs and improves query response times which is crucial for business operations.
Furthermore, understanding your data's schema is crucial before any data manipulation. Improperly defined schemas lead to errors and inefficient computations. For example, if a column that should be numeric is mistakenly interpreted as a string, computations on that column will fail. Data validation and cleaning are integral to data manipulation. Identify and correct data inconsistencies before processing, such as missing values, outliers, or duplicate entries, to ensure data quality and reliability. By carefully cleaning and validating your data, you will achieve more robust and accurate results.
Data Transformations
PySpark DataFrames offer a rich set of functions for transforming data. These functions allow you to filter, select, aggregate, and reshape data efficiently. Let's illustrate with some examples.
Filter data filtered_df = df.filter(df["Age"] > 30) Select columns selected_df = df.select("Name", "Age") Add a new column df = df.withColumn("AgeSquared", df["Age"] * df["Age"]) Rename a column df = df.withColumnRenamed("Age", "Years") Drop a column df = df.drop("AgeSquared") Group and aggregate grouped_df = df.groupBy("Name").agg({"Years": "max"})
Filtering allows you to select only the relevant data. For example, a marketing team might filter customer data to identify users aged between 25 and 40. Selecting specific columns reduces the data volume processed, leading to improved performance. This is particularly beneficial for large datasets where processing all columns would be inefficient. Consider a financial analyst needing to focus on specific financial indicators for analysis. Column selection allows focusing on these specific aspects only.
Adding new columns is useful for creating calculated fields. This is crucial for feature engineering in machine learning or generating summary statistics in data analysis. For instance, a retail analyst might create a new column to calculate the total purchase amount from individual transaction data. Similarly, calculating a customer's average purchase frequency requires adding a derived column to the DataFrame. Renaming columns enhances clarity and readability, especially in collaborative projects. Consistent naming conventions are pivotal for understanding and maintaining data quality. Clear and consistent names are essential for collaborative data analysis.
Dropping irrelevant columns reduces data storage and improves query performance. Unnecessary columns add overhead and can slow down processing. For instance, removing temporary or intermediate columns after they've served their purpose improves efficiency. This is common when performing multiple stages of data transformation. In a large-scale data processing pipeline, removing intermediate columns is crucial for resource management and optimizes the entire process. Efficient data manipulation reduces storage and processing costs, which is especially relevant in resource-intensive environments.
Aggregation involves summarizing data. For instance, grouping data by city and calculating the average income in each city provides valuable insights. Grouping and aggregation are essential for summarizing and interpreting large amounts of data effectively. This is crucial for business intelligence and reporting purposes where extracting key metrics is essential. Consider a market research firm compiling average consumer spending trends. Aggregation efficiently summarizes vast amounts of transactional data.
Window Functions
Window functions allow calculations across a set of rows related to the current row. This is different from traditional aggregate functions that summarize entire groups.
from pyspark.sql.functions import rank, lag, lead Rank users by age df = df.withColumn("rank", rank().over(Window.orderBy("Years"))) Calculate the lag and lead of age df = df.withColumn("lag", lag("Years", 1).over(Window.orderBy("Years"))) df = df.withColumn("lead", lead("Years", 1).over(Window.orderBy("Years")))
Ranking is a frequently used window function. It assigns a rank to each row based on a specified order. This is used in scenarios such as leaderboard generation or ranking customers based on purchasing power. For instance, an e-commerce site might use ranking to showcase top-selling products or highlight high-value customers. Ranking helps to order users by criteria. It finds its uses in various applications including those focused on consumer behavior, financial market performance, and other areas.
Lag and lead functions provide access to previous and subsequent row values. This is useful for calculating changes over time or detecting trends. For example, financial analysts might use lag and lead to calculate percentage changes in stock prices day over day. Tracking metrics over time provides insight into trends and patterns, whether in social media engagement, financial markets, or sales figures.
Window functions excel at analyzing sequential data, as commonly encountered in time series analysis. They efficiently calculate running totals, moving averages, and other time-based aggregates without requiring complex joins. In applications like fraud detection, window functions can detect unusual patterns by comparing current transactions with preceding ones. The ability to analyze sequential data is pivotal in understanding dynamic processes in areas like finance, healthcare, and logistics. Consider analyzing patterns in website traffic or evaluating the efficiency of a production line. Window functions empower the examination of data sequences.
Moreover, advanced window functions can handle complex scenarios involving partitions and ordering. Partitioning allows for independent calculations within subsets of data. This is useful when performing calculations within specific groups or categories. Consider an application that requires independent analysis of sales figures for each product category. Partitions allow us to perform window function calculations separately for each category.
UDFs (User-Defined Functions)
User-defined functions (UDFs) extend PySpark's capabilities by allowing you to define custom functions in Python and use them within your DataFrames.
from pyspark.sql.functions import udf from pyspark.sql.types import StringType Define a UDF to convert age to a string @udf(returnType=StringType()) def age_to_string(age): if age > 30: return "Senior" else: return "Junior" Apply the UDF df = df.withColumn("AgeGroup", age_to_string(df["Years"]))
UDFs are essential when dealing with complex or domain-specific transformations not directly available in PySpark's built-in functions. For instance, natural language processing tasks or custom data validation rules often require UDFs. Consider tasks requiring sentiment analysis from textual data; a custom UDF can be developed to categorize text based on sentiment. Another example is developing UDFs for custom validation based on domain-specific business rules. UDFs enhance the flexibility of PySpark in handling diverse data manipulation needs.
UDFs provide flexibility and extensibility. However, they can impact performance if not optimized. It's crucial to carefully consider the efficiency of UDFs, especially for large datasets. Inefficient UDFs can lead to significant performance degradation. For large datasets, it is critical to optimize UDFs using techniques such as vectorization or avoiding Python loops inside the function. Optimized UDFs ensure that data manipulation remains fast even when working with terabytes of data.
UDFs empower customization and cater to specific analytical needs not readily addressed by existing functions. They provide an avenue to incorporate complex logic or domain-specific algorithms directly into PySpark pipelines. UDFs allow incorporating specialized computations based on specific rules or algorithms. For example, a financial model requiring custom calculations would benefit from the use of UDFs. This customizability is highly beneficial when integrating with external libraries or custom algorithms.
When designing UDFs, it's essential to define appropriate return types. This ensures data type compatibility with the DataFrame schema. Inconsistencies in data types between UDF outputs and the DataFrame can lead to errors and unexpected behavior. Correct data type handling is vital for maintaining data integrity throughout the data processing pipeline. Proper data type management improves the reliability and maintainability of your PySpark scripts. Clear specification of input and output types ensures smooth data flow and minimizes errors.
Data Writing and Persistence
After manipulating data, you need to save it for future use. PySpark provides various options for writing data to different formats and locations.
Write data to a CSV file df.write.csv("path/to/output/file.csv", header=True) Write data to a Parquet file df.write.parquet("path/to/output/file.parquet") Write data to a JSON file df.write.json("path/to/output/file.json")
Choosing the right data format depends on your needs. CSV is a simple, widely supported format, but it can be less efficient for large datasets compared to other formats. Parquet and ORC are columnar storage formats, which are generally more efficient for analytical queries. They provide better compression and enable efficient column-level access. This efficiency is crucial when dealing with large datasets and frequent queries. Parquet and ORC are highly efficient storage formats that minimize storage space and boost query performance.
Consider writing data to cloud storage solutions like AWS S3, Azure Blob Storage, or Google Cloud Storage. Cloud storage offers scalability, durability, and cost-effectiveness, especially for large datasets. Cloud storage solutions provide robust infrastructure and enable parallel processing capabilities. They also provide scalability for handling datasets of any size and increase the efficiency of distributed computations. Cloud storage options such as AWS S3, Azure Blob Storage, and Google Cloud Storage provide efficient and scalable solutions for storing and processing large amounts of data in the cloud.
Data partitioning and bucketing can significantly improve read and write performance. Partitioning divides data into smaller files based on a chosen column, enabling parallel processing during read operations. This is highly beneficial for large datasets. Partitions improve data organization and optimize query execution times. Bucketing further enhances query efficiency by creating smaller partitions within partitions, making it easier to access data efficiently. Bucketing provides a high level of data organization and improves the speed of queries.
Data persistence strategies are essential for managing and accessing data across sessions. Data can be written to a persistent storage location, such as a cloud storage service or a distributed file system, allowing for retrieval later. This is crucial for building data pipelines and managing data across multiple sessions. Efficient data persistence strategies are essential for improving the performance of data workflows and ensuring that data is easily accessible when needed. Reliable data persistence practices are critical for building robust and scalable big data applications.
Conclusion
Mastering PySpark DataFrames is crucial for anyone working with big data. This comprehensive guide has explored fundamental concepts, from data loading and transformations to advanced techniques like window functions and UDFs. By effectively utilizing these tools and techniques, you can efficiently process and analyze large datasets, unlocking valuable insights from your data.
Remember that understanding data types, optimizing performance, and choosing the right data formats are essential for achieving optimal results. Continuously learning and adapting to the evolving landscape of big data technologies will ensure you remain proficient and efficient in your data analysis endeavors. The practical application of the concepts discussed in this guide, through consistent practice and real-world projects, is crucial for solidifying your understanding and developing expertise in handling large datasets using PySpark. Always keep updated with the latest advancements in big data technology to enhance your efficiency and effectiveness.