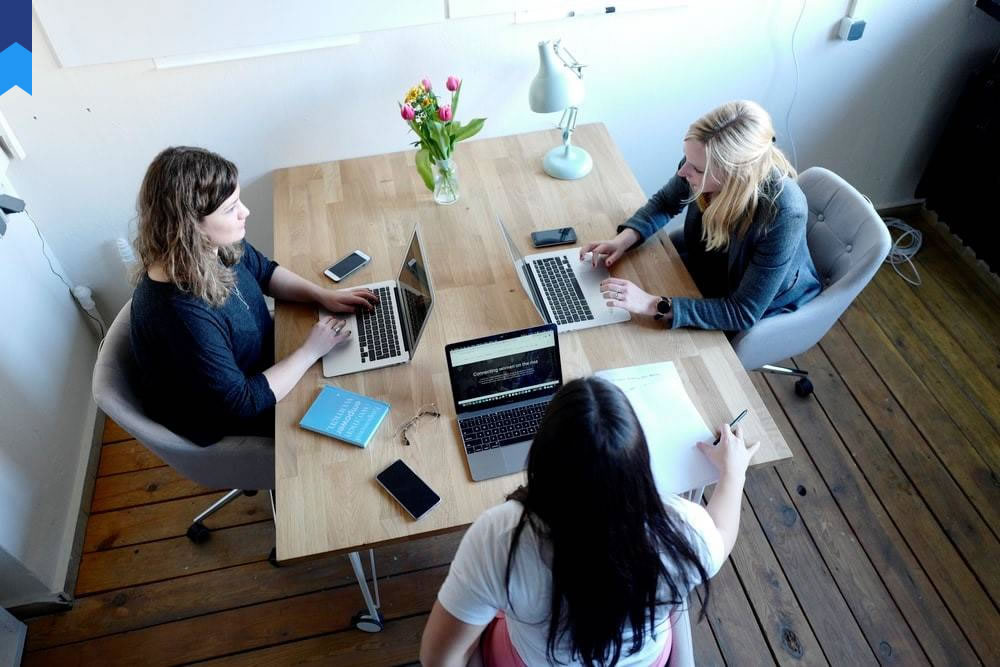
Mastering Regular Expressions: A Comprehensive Guide For Developers
Regular expressions, often abbreviated as regex, are powerful tools used to search, match, and manipulate text. They provide a concise and flexible way to define patterns that can be used to find, extract, and replace specific strings within larger bodies of text. Whether you're a seasoned developer or just starting out, understanding regular expressions is essential for a wide range of tasks, from data validation and text processing to web scraping and security analysis.
This comprehensive guide will delve into the intricacies of regular expressions, covering their fundamental concepts, syntax, and practical applications. We'll explore various techniques for constructing effective patterns, examine real-world use cases, and showcase how regex can streamline your development workflows.
Introduction
Regular expressions are a fundamental tool in the arsenal of any programmer. They allow you to search, match, and manipulate text data in a powerful and flexible way. Whether you're working with large datasets, processing user input, or performing text analysis, regex can significantly improve your efficiency and accuracy.
At its core, a regular expression is a sequence of characters that defines a search pattern. These patterns can be simple, like matching a single character or a specific word, or complex, encompassing multiple patterns, quantifiers, and special characters. The power of regex lies in its ability to represent a wide range of patterns with a compact and expressive syntax.
Understanding regular expressions is essential for anyone involved in software development, particularly for tasks involving data manipulation, text processing, and web development. Mastering regex can significantly improve your coding skills, allowing you to write more efficient and robust code.
Understanding the Basics of Regular Expressions
Regular expressions are based on a simple but powerful concept: defining patterns to match text. The core elements of regex include:
- Literals: These are the basic building blocks of a regex pattern. They represent specific characters that must match exactly. For example, "a" will match the letter "a", and "123" will match the number "123".
- Metacharacters: These special characters have a specific meaning within a regex and allow you to create more complex patterns. For example, "." matches any single character, "*" matches zero or more occurrences of the preceding character, and "+" matches one or more occurrences.
- Character Classes: These allow you to define a range of characters that you want to match. For example, "[a-z]" matches any lowercase letter, "[0-9]" matches any digit, and "[A-Za-z0-9]" matches any alphanumeric character.
- Quantifiers: These specify the number of times a preceding character or group should occur. For example, "{3}" matches exactly three occurrences, "{2,}" matches at least two occurrences, and "{2,5}" matches between two and five occurrences.
Here are some basic examples:
- "cat" matches the word "cat" exactly.
- ".at" matches any three-letter word ending in "at", like "cat", "bat", or "hat".
- "[A-Za-z]+" matches any string containing one or more letters.
- "[0-9]{3}" matches any three-digit number.
These simple examples demonstrate the basic building blocks of regular expressions. As you progress, you'll learn how to combine these elements to create more sophisticated patterns. The flexibility and power of regex lie in its ability to express a vast array of patterns with a concise and expressive syntax.
Mastering Advanced Techniques
Beyond the basic elements, regular expressions offer a rich set of advanced techniques that allow you to define more complex patterns. Some key techniques include:
- Grouping: You can group parts of a regex pattern using parentheses. This allows you to apply quantifiers and other operators to the entire group. For example, "(cat|dog){2}" matches either "cat" or "dog" repeated twice, such as "catdog" or "dogcat".
- Backreferences: Backreferences allow you to refer to a previously matched group within a regex pattern. For example, "(.)\1" matches a character followed by the same character, such as "aa" or "bb".
- Lookarounds: Lookarounds are zero-width assertions that allow you to match based on the context surrounding a pattern without actually including the context in the match itself. For example, `(?<=ABC)DEF` matches "DEF" only if it is preceded by "ABC".
- Character Classes: You can use predefined character classes, such as `\d` for digits, `\s` for whitespace, and `\w` for word characters. This provides a convenient way to match common character sets.
These advanced techniques empower you to create more complex and precise regex patterns. They allow you to match patterns based on their position within a string, their surrounding context, and their repeated occurrences.
Real-World Applications of Regular Expressions
Regular expressions have numerous real-world applications across various domains, including:
- Data Validation: Regex is widely used for validating user input. It can ensure that emails follow a specific format, passwords meet certain criteria, or phone numbers are valid. For example, `[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}` is a common regex pattern used to validate email addresses.
- Text Processing: From extracting specific information from text files to cleaning up data, regex plays a crucial role in text processing. For example, you can use regex to extract phone numbers from a large document or remove unnecessary whitespace from a string.
- Web Scraping: Web scraping involves extracting data from websites. Regex is essential for parsing HTML and extracting specific elements, such as product descriptions or prices. For example, you can use `(?<=
)(.*?)(?= )` to extract the title of a web page. - Security Analysis: Regex is used in security analysis to identify potential vulnerabilities in code or data. For example, it can be used to detect SQL injection attacks or cross-site scripting vulnerabilities by matching specific patterns in code or data.
These are just a few examples of the many ways regular expressions are used in real-world applications. Their flexibility and power make them invaluable tools for developers in various domains.
Using Regular Expressions in Your Code
Regular expressions are supported by most programming languages and tools. Here are some common ways to use regex in your code:
- Matching: You can use regex to check if a given string matches a particular pattern. For example, in Python, you can use the `re.search()` function to search for a pattern within a string.
- Extracting: Regex can be used to extract specific parts of a string. For example, you can use the `re.findall()` function to find all occurrences of a pattern within a string.
- Replacing: You can use regex to replace parts of a string based on a pattern. For example, you can use the `re.sub()` function to replace all occurrences of a pattern with a new string.
Here are some examples of using regex in different programming languages:
- Python:
import re text = "This is a sample text with phone number 123-456-7890." phone_pattern = r"\d{3}-\d{3}-\d{4}" match = re.search(phone_pattern, text) if match: print("Phone number found:", match.group()) else: print("No phone number found.")
const text = "This is a sample text with email address john.doe@example.com."; const emailPattern = /[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}/; const match = text.match(emailPattern); if (match) { console.log("Email address found:", match[0]); } else { console.log("No email address found."); }
import java.util.regex.Matcher; import java.util.regex.Pattern; String text = "This is a sample text with URL https://www.example.com."; String urlPattern = "https?://(www\\.)?[-a-zA-Z0-9@:%._\\+~=]{1,256}\\.[a-zA-Z0-9()]{1,6}\\b([-a-zA-Z0-9()@:%_\\+.~?&//=]*)"; Pattern pattern = Pattern.compile(urlPattern); Matcher matcher = pattern.matcher(text); if (matcher.find()) { System.out.println("URL found: " + matcher.group()); } else { System.out.println("No URL found."); }
These examples illustrate the basic syntax and usage of regex in various programming languages. It's important to note that specific implementation details and syntax may vary between languages. However, the fundamental principles and concepts of regex remain consistent across different programming environments.
Conclusion
Regular expressions are a powerful and versatile tool for manipulating text. They offer a concise and expressive syntax for defining complex patterns that can be used for a wide range of tasks, from data validation and text processing to web scraping and security analysis.
This guide has provided a comprehensive overview of regular expressions, covering their fundamentals, advanced techniques, real-world applications, and practical examples. By understanding the concepts and techniques discussed here, you can effectively harness the power of regex to enhance your coding skills and streamline your development workflows.
Remember that regular expressions are a vast and complex topic. This guide has only scratched the surface. Continued exploration and experimentation are essential for mastering the full potential of this powerful tool. By dedicating time to learning and practicing, you can become a proficient regex user and leverage its capabilities to solve various challenges in your development journey.