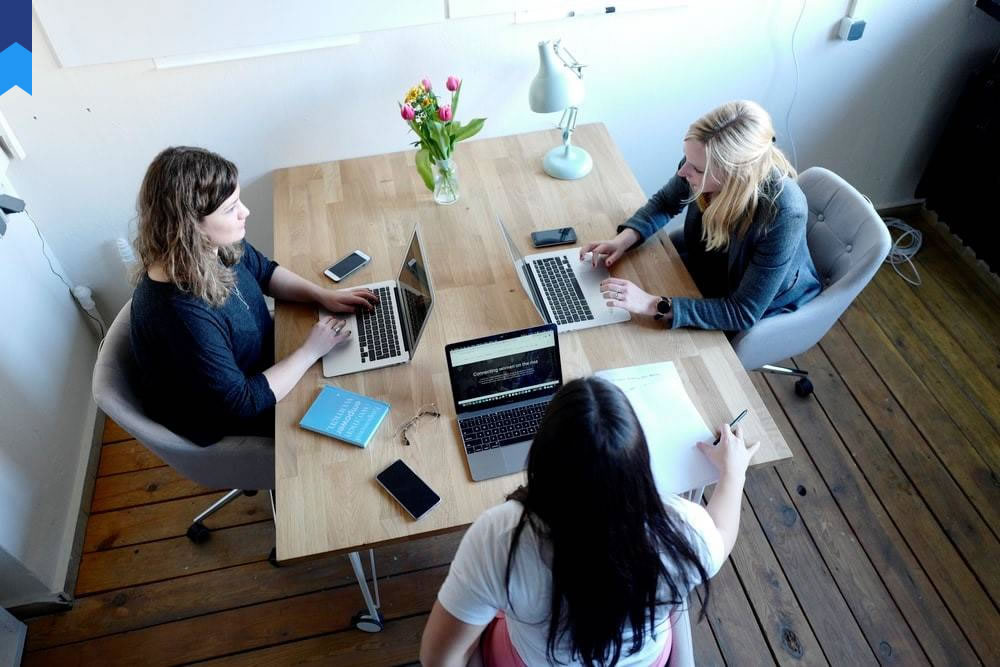
Mastering The Art Of Asynchronous JavaScript: Promises And Async/Await
Asynchronous JavaScript is crucial for building responsive and efficient web applications. This article delves into Promises and Async/Await, two powerful tools for handling asynchronous operations in JavaScript, explaining their functionalities, benefits, and practical applications.
Understanding JavaScript Promises
Promises are a fundamental building block of asynchronous JavaScript. They represent the eventual result of an asynchronous operation, which might be successful or fail. A Promise can be in one of three states: pending (initial state), fulfilled (operation completed successfully), or rejected (operation failed). The core of a Promise lies in its ability to handle the outcome of an asynchronous task gracefully, without blocking the main thread. This prevents freezing the user interface, ensuring a smoother user experience. Consider fetching data from an API: A Promise encapsulates this operation, allowing you to handle the response (fulfilled) or the error (rejected) in a structured manner.
A common use case is handling API calls. If you attempt to access a resource that does not exist, the promise will be rejected, allowing you to display an appropriate message to the user rather than crashing the application. Proper error handling using promises reduces unexpected application behavior and improves stability. For example, imagine an e-commerce application. Using promises, you can handle the case where a payment gateway fails to process a transaction without disrupting the entire checkout flow.
Promises provide methods like `.then()` for handling success and `.catch()` for handling failure, enabling a cleaner and more readable code structure. This structured approach makes it much easier to debug and maintain asynchronous operations in your application. Furthermore, Promises can be chained, allowing you to perform a sequence of asynchronous operations. Consider a scenario where you need to fetch data, process it, and then update the UI. Chaining Promises allows you to handle these operations sequentially and elegantly.
Case Study 1: A large social media platform uses promises extensively for handling user interactions, such as posting updates, liking content, and sending messages. This enables real-time updates to the UI without disrupting the user's flow. Case Study 2: An online banking application utilizes promises to handle transactions securely, ensuring that each step is handled properly and errors are gracefully managed, leading to improved security and stability.
Async/Await: A More Elegant Approach
Async/Await builds upon Promises, providing a more synchronous-like syntax for handling asynchronous operations. The `async` keyword transforms a function into an asynchronous function, allowing it to use the `await` keyword. `await` only works inside an `async` function and pauses execution until a Promise resolves (fulfilled or rejected). This simplifies the code significantly, making asynchronous operations easier to read and understand. It allows you to write asynchronous code that looks and behaves a lot more like synchronous code, thereby reducing complexity.
Async/Await significantly enhances code readability, particularly in situations involving multiple chained Promises. It eliminates the need for nested `.then()` blocks, which can become cumbersome to manage. The synchronous-like structure is far more intuitive for developers accustomed to traditional synchronous programming styles. This results in improved developer productivity and reduced debugging time. Error handling within async/await is also straightforward; using a `try...catch` block elegantly handles any rejected Promises.
Consider a scenario where you need to retrieve data from multiple API endpoints. Without Async/Await, managing the promises would involve deeply nested `.then()` calls. However, using async/await simplifies this process by awaiting each promise sequentially, resulting in cleaner and more manageable code. This improves maintainability and makes it easier for other developers to understand the code's logic.
Case Study 1: A popular streaming service utilizes async/await for managing video playback. This allows for seamless transitions between videos and efficient handling of buffering events. Case Study 2: A large e-commerce company employs async/await to manage shopping cart updates and order processing, optimizing speed and preventing conflicts during high-traffic periods.
Error Handling in Asynchronous Operations
Robust error handling is crucial for building reliable asynchronous applications. While Promises provide `.catch()` for error handling, Async/Await integrates with the `try...catch` block, making error handling more intuitive. This allows you to use familiar error handling mechanisms, simplifying the process and enhancing code readability. The `try...catch` block catches exceptions thrown during the execution of the asynchronous function, ensuring that errors are handled gracefully without crashing the application.
Effective error handling goes beyond simply catching exceptions. It includes logging errors for debugging purposes, providing informative error messages to users, and implementing retry mechanisms for transient errors, such as network connectivity issues. Logging errors provides vital information for identifying and resolving bugs, while informative messages to users help maintain a positive user experience. Retry mechanisms allow the system to attempt the operation again after a suitable delay in case of temporary errors.
It’s crucial to distinguish between recoverable and unrecoverable errors. Recoverable errors might be temporary issues, such as network glitches, which could be handled by retrying the operation after a delay. Unrecoverable errors, such as invalid input data or critical system failures, should be handled gracefully, informing the user and potentially logging the error for debugging. Implementing a robust strategy is essential, balancing user experience with application stability.
Case Study 1: A cloud storage service utilizes a sophisticated error-handling system that logs detailed information for debugging while presenting user-friendly messages for common errors. Case Study 2: A financial application implements several error-handling strategies, including retries for network failures and custom error messages for validation errors.
Advanced Techniques and Best Practices
Beyond the basics, several advanced techniques refine asynchronous programming. These include using `Promise.all()` to concurrently execute multiple Promises, and using `Promise.race()` to resolve the first Promise that fulfills or rejects. `Promise.all()` is particularly useful for fetching data from multiple sources simultaneously, optimizing performance. `Promise.race()` is beneficial when you need the fastest response among multiple asynchronous operations.
Implementing proper cancellation mechanisms is vital for preventing resource leaks and ensuring responsiveness. This might involve using signals or timers to interrupt long-running asynchronous operations if they are no longer necessary. Efficient cancellation prevents unnecessary resource consumption and enhances the overall performance and stability of the application.
Careful consideration of resource management is crucial, especially when dealing with long-running asynchronous operations or a large number of concurrent operations. Memory leaks can occur if resources are not properly released. Regularly reviewing and optimizing code helps avoid these issues and ensure application reliability. Performance monitoring is also vital to identify and address performance bottlenecks, which might stem from inefficient asynchronous operations.
Case Study 1: A mapping application uses `Promise.all()` to load map tiles concurrently, significantly reducing rendering time. Case Study 2: A real-time chat application employs advanced cancellation techniques to avoid resource leaks and guarantee responsive messaging.
Future Trends in Asynchronous JavaScript
As JavaScript continues to evolve, asynchronous programming remains a critical component of modern web development. Future trends focus on enhancing performance, simplifying development, and improving error handling. Expect to see more tools and libraries emerging that streamline asynchronous programming, further reducing the complexity of handling asynchronous operations. Better tooling and frameworks will simplify the development process and make asynchronous code easier to write and maintain.
The focus on performance optimization will continue, with innovations in asynchronous programming potentially leading to substantial improvements in application responsiveness and efficiency. Research is underway to discover novel approaches to improve performance and reduce overhead related to asynchronous tasks. More robust error handling techniques and improved debugging tools are also anticipated.
The increased complexity of modern web applications will drive innovation in asynchronous programming, with new patterns and techniques emerging to simplify complex asynchronous workflows. The rise of new JavaScript frameworks and libraries will continue to shape asynchronous practices, incorporating best practices and pushing performance boundaries. Expect more integration with other technologies to enhance the capabilities of asynchronous JavaScript, such as tighter integration with server-side technologies.
Case Study 1: Ongoing research into asynchronous programming is exploring innovative approaches to optimize performance and reduce overhead, particularly in handling a large number of concurrent operations. Case Study 2: The development of new JavaScript frameworks incorporates improved asynchronous handling, simplifying the development process and reducing common pitfalls.
Conclusion
Mastering asynchronous JavaScript, specifically using Promises and Async/Await, is crucial for modern web developers. These tools provide elegant and efficient ways to handle asynchronous operations, leading to improved application performance, responsiveness, and maintainability. Understanding error handling, advanced techniques, and keeping an eye on future trends are essential for building robust and high-performing applications. By embracing these best practices, developers can create exceptional user experiences and maintain the stability of their applications. This detailed examination of asynchronous JavaScript provides a strong foundation for building complex, yet efficient, applications.