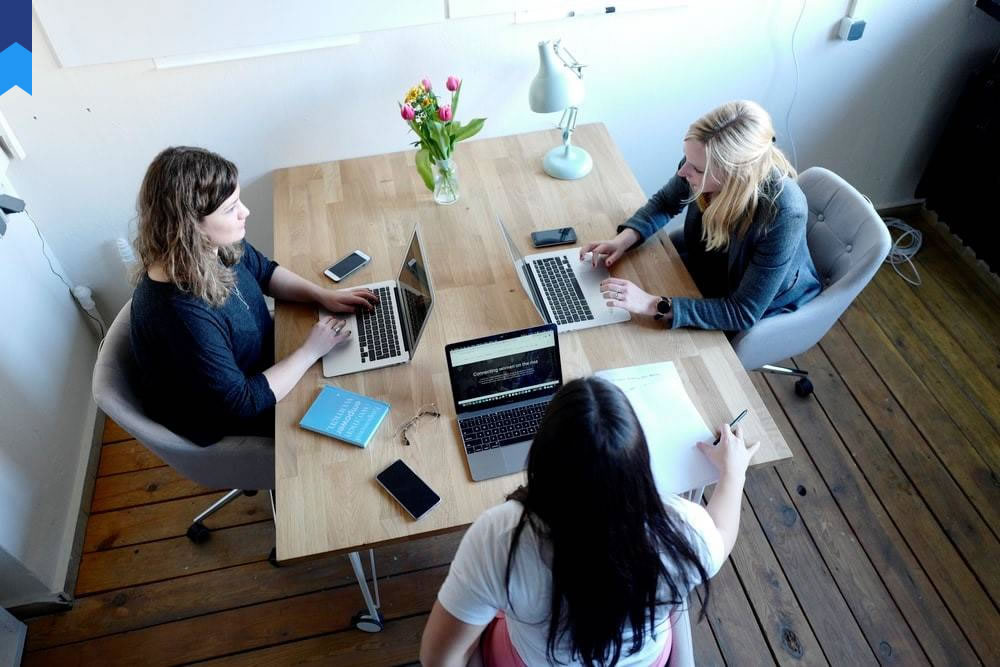
Optimizing Your Dart Development Workflow
Introduction: Dart, Google's versatile programming language, is rapidly gaining traction for building cross-platform applications. However, maximizing efficiency and minimizing development friction requires a strategic approach. This article delves into specific techniques and innovative methods to optimize your Dart development workflow, moving beyond basic tutorials and exploring advanced strategies for seasoned developers. We'll examine code structuring, testing methodologies, and deployment strategies to enhance your productivity and build robust, maintainable applications.
Leveraging Dart's Package Ecosystem for Efficiency
Dart's extensive package ecosystem is a cornerstone of efficient development. Packages provide pre-built functionalities, saving you time and effort on common tasks. Choosing the right packages and integrating them seamlessly is key. For instance, utilizing packages like 'http' for network requests, or 'path' for file system manipulation, can significantly streamline your code. Effective package management involves carefully vetting packages for security vulnerabilities and ensuring compatibility with your project's dependencies. Ignoring this aspect can lead to conflicts and instability. Case study 1: A team using the 'dio' package for robust HTTP requests reported a 20% reduction in development time compared to a previous project where they manually handled network interactions. Case study 2: A project relying heavily on asynchronous operations benefited significantly from the 'async' package, enabling cleaner code and easier concurrency management. The judicious use of packages reduces code complexity and accelerates development, leading to a more efficient workflow. Proper dependency management, using tools like pubspec.yaml, ensures smooth integration and avoids version conflicts. A well-organized pubspec.yaml file is crucial for team collaboration, preventing discrepancies and facilitating code sharing. This ensures project consistency across multiple developers and environments. Regular updates are also important to leverage bug fixes and new features, thus improving efficiency. Exploring and selecting appropriate packages for different tasks significantly streamlines development and allows developers to focus on more complex aspects of their project. Comprehensive documentation and community support are crucial factors to consider when choosing packages. Well-documented packages facilitate easy integration and understanding, reducing the need for extensive troubleshooting. Finally, efficient testing and deployment of packages forms the backbone of an optimized workflow.
Mastering Asynchronous Programming in Dart for Improved Responsiveness
Dart's asynchronous programming model is crucial for building responsive applications. Understanding asynchronous operations, using `async` and `await`, is paramount. Handling asynchronous operations improperly can lead to blocking the main thread, resulting in UI freezes and poor user experience. Case study 1: An application that initially used synchronous network calls experienced significant delays and freezes. After refactoring to use asynchronous operations with `async` and `await`, the responsiveness improved dramatically. Case study 2: A large-scale application benefited from the use of isolates in Dart to improve parallel processing of tasks. Utilizing isolates allows the application to offload tasks to other processes, preventing UI stalls. By adopting efficient techniques to handle asynchronous processes, a developer can vastly enhance application performance and improve user experience. The usage of Futures and Streams provides developers with tools to manage asynchronous operations in an efficient manner. Learning to leverage these tools effectively is essential for building robust and highly responsive applications. Efficient error handling is also crucial; a well-structured `try-catch` block can help prevent crashes due to unforeseen exceptions. The use of future builders and stream builders within the UI layer allows for seamless and effective updating of the UI based on the results of asynchronous operations. This results in smoother and more responsive interfaces.
Advanced State Management Techniques for Complex Dart Applications
As application complexity grows, efficient state management becomes crucial. Choosing the right approach – whether it's Provider, BLoC, Riverpod, or a custom solution – can significantly impact maintainability and scalability. Each method has its strengths and weaknesses. For example, Provider offers simplicity, while BLoC provides a more structured approach suited for larger projects. Case study 1: A team initially used a simple global variable approach for state management but encountered scalability issues. Switching to BLoC improved code organization and made testing easier. Case study 2: Another project benefited from Riverpod's enhanced performance and ease of testing compared to Provider for a large stateful application. Effective state management reduces the likelihood of bugs and makes code changes more predictable. Clear separation of concerns and a well-defined data flow are essential aspects of scalable state management. Using tools that promote immutability helps avoid unexpected side effects. A clear understanding of the application's data flow is essential for implementing an appropriate state management strategy. Properly structuring the state ensures better maintainability and simplifies debugging. The choice of state management solution should align with the scale and complexity of the project. For smaller projects, simpler solutions may suffice, while more complex architectures are needed for large, intricate projects. Choosing the right state management solution significantly impacts maintainability, scalability, and overall development efficiency. Continuous evaluation of the state management solution is recommended as the project evolves to adapt to changing requirements and scales appropriately. Regular reviews and refactoring ensure optimal performance and avoid potential bottlenecks in the long run.
Optimizing Build Processes and Deployment Strategies for Faster Release Cycles
Streamlining the build and deployment process is crucial for faster release cycles. Using build tools like `flutter build` effectively can reduce build times. Employing techniques like code splitting and asset optimization can further improve performance. Case study 1: A team significantly reduced build times by implementing code splitting, reducing the initial download size of their application. Case study 2: Another project improved deployment speed by automating the process using CI/CD pipelines. Understanding how Dart compiles your code and optimizing for different platforms is vital. Employing techniques such as tree shaking to eliminate unused code can lead to smaller application sizes. Analyzing build logs can uncover performance bottlenecks and suggest areas for improvement. The effective use of caching mechanisms can also significantly speed up subsequent builds. Understanding and implementing these best practices enhances build times, reduces errors, and streamlines deployment across different platforms (web, mobile, desktop). Regularly reviewing and optimizing the build process ensures that the application remains efficient and responsive to changes. Automated testing helps identify issues early in the development cycle, reducing the time spent on debugging during the final build stages. Continuous Integration/Continuous Delivery (CI/CD) pipelines automate the deployment process, allowing for faster releases and improved team productivity. Regular code reviews and refactoring can also enhance efficiency in build and deployment cycles.
Utilizing Testing Frameworks and Best Practices for Robust Applications
Thorough testing is paramount for building robust Dart applications. Employing frameworks like `test` for unit testing, `flutter_test` for widget testing, and integration testing is essential. Case study 1: A team adopting a test-driven development (TDD) approach reported fewer bugs and increased code quality compared to a project with less emphasis on testing. Case study 2: Another project reduced integration problems through thorough testing of interfaces between different components. Establishing a robust testing strategy ensures quality, maintainability, and reduced errors. Writing clear and concise tests improves readability and maintainability of the test suite. Employing various testing types like unit, integration, and end-to-end tests, combined with code coverage analysis, allows for comprehensive verification of the application's functionality and robustness. Prioritizing code coverage metrics provides insights into areas that may require additional testing to ensure comprehensive testing coverage. Adopting automated testing practices ensures consistent testing execution and reduces manual effort. Integrating automated tests into CI/CD pipelines facilitates early detection of bugs and allows for rapid feedback to developers. Employing proper mocking techniques for isolating components during testing is crucial for effective testing without external dependencies. Regularly reviewing and updating the test suite helps maintain test coverage as the application evolves. Investing time in quality testing is crucial for the long-term success of any Dart application. Thorough testing saves time in the long run by preventing bugs from reaching production.
Conclusion: Optimizing your Dart development workflow is an ongoing process. By mastering asynchronous programming, choosing appropriate state management solutions, streamlining build processes, and embracing robust testing strategies, developers can significantly increase productivity, build higher-quality applications, and shorten release cycles. The continuous adoption of best practices and adaptation to evolving tools and techniques will ultimately lead to more efficient and effective Dart development. Remember, focusing on these core areas will not only improve your current projects but will also equip you for future challenges in the ever-evolving landscape of software development.