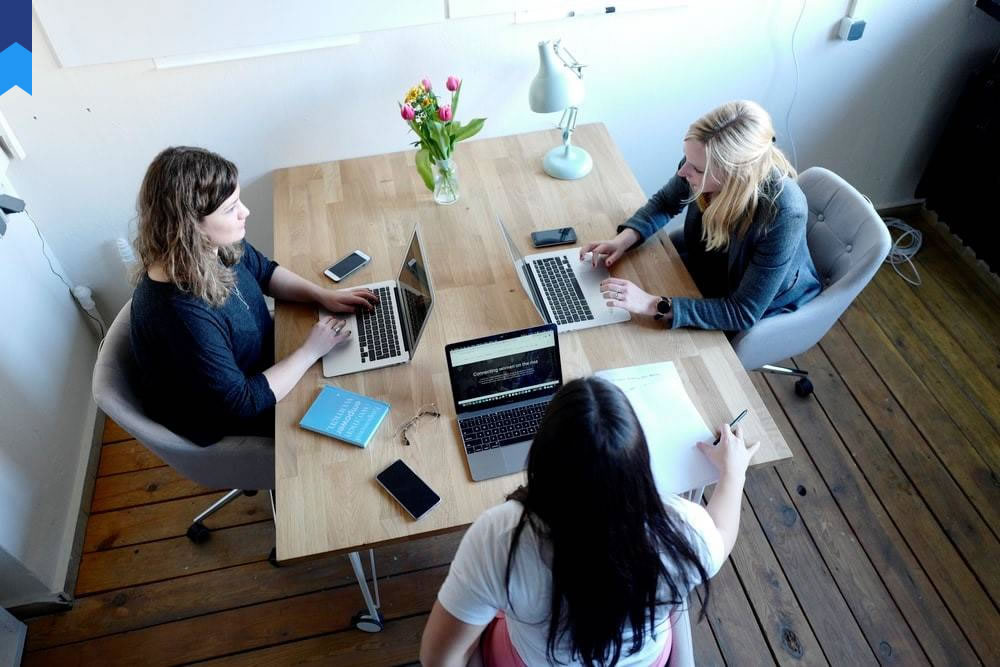
Rethinking jQuery: A Modern Approach to Dynamic Web Development
jQuery, once the undisputed king of JavaScript frameworks, continues to hold a significant place in web development. However, its dominance has waned somewhat in the face of newer, more specialized libraries and frameworks. This article delves into how jQuery remains a relevant and powerful tool for modern web developers, presenting a fresh perspective on its capabilities beyond the basics.
Harnessing jQuery's Power for DOM Manipulation
jQuery's core strength lies in its elegant and efficient DOM manipulation capabilities. Traditionally used for tasks like selecting elements, adding classes, and manipulating attributes, jQuery continues to excel in these areas, even with the rise of other frameworks. Its concise syntax reduces development time, leading to cleaner and more readable code. For instance, selecting elements with complex CSS selectors becomes straightforward: `$("#myElement").addClass("highlight");` This simple line of code would require significantly more verbose JavaScript without jQuery. Consider a complex scenario: dynamically updating a product list based on user filters. jQuery's selectors and methods make this process manageable and efficient, allowing for intricate filtering and sorting operations.
Case study 1: An e-commerce platform uses jQuery to dynamically update product listings based on user-selected filters (price range, brand, etc.). This enhances user experience by providing instant feedback without full page reloads. Case study 2: A news website uses jQuery to manage and update news articles displayed in a carousel on the homepage, ensuring smooth animations and transitions without the complexities of direct JavaScript DOM manipulation.
Moreover, jQuery's chaining functionality streamlines DOM manipulation sequences, enabling developers to execute multiple operations on the same element in a single, readable line. The use of `$.each()` allows elegant traversal of collections, improving workflow by simplifying looping operations, a key advantage for handling large datasets or complex structures. Furthermore, jQuery's animation capabilities provide streamlined ways to add visual appeal to websites, a feature still highly relevant.
Let's delve into the details of how jQuery simplifies DOM traversing and manipulation using the `.find()`, `.parent()`, `.children()`, and `.siblings()` methods. These allow for navigating complex DOM structures with greater efficiency than vanilla Javascript. For example, finding a specific element within a nested structure is as simple as `$("#parentContainer").find(".specificElement");`. This simplicity enhances code readability and maintainability, especially in large projects. Its intuitive methods like `append()`, `prepend()`, `after()`, and `before()` add elements to the DOM efficiently. These offer precise control over element placement, simplifying the task of dynamic content insertion and management.
Furthermore, jQuery's `toggleClass()` method dynamically adds or removes classes from an element, useful for handling responsive design and user interface interactions. Efficiently managing CSS classes within jQuery promotes code flexibility and adaptability to different screen sizes and conditions. The ability to easily handle events and manipulate DOM elements in response to user interactions remains one of jQuery's significant advantages. This empowers developers to create interactive elements that respond dynamically to user input, a key feature in modern web development.
Event Handling and User Interactions
Beyond DOM manipulation, jQuery simplifies event handling. Attaching event listeners to elements is straightforward, improving the developer experience. Instead of writing lengthy JavaScript event handlers, developers can concisely manage events using jQuery's methods. For example, binding a click event to an element is reduced to: `$("#myButton").click(function() { /* your code here */ });`. This streamlined syntax improves code readability and reduces development time. Furthermore, jQuery's ability to handle events efficiently remains a key advantage, especially when dealing with complex interactions.
Case study 1: A social media website uses jQuery to handle real-time updates, displaying new posts or notifications dynamically without full page reloads. This improves the user experience by presenting instant feedback. Case study 2: An online gaming platform uses jQuery to manage user input and update game states smoothly in response to player actions. This ensures seamless user interaction without performance issues.
jQuery offers advanced event handling capabilities that simplify complex interactions. It allows developers to easily handle custom events and efficiently manage event propagation, leading to robust and reliable event handling. Handling multiple events using a single function can streamline code and make it easier to maintain. Efficiently delegating events, particularly for dynamically added elements, addresses a common challenge encountered in interactive web development. This ensures that events are correctly bound to dynamically created elements, improving code robustness.
Consider the challenge of handling events on dynamically added elements. With jQuery, developers can easily delegate events to a parent container, ensuring that all elements added to that container will inherit the relevant event handlers. This provides elegant solutions for managing interactions in dynamic environments, such as a chat application where messages are constantly added. Advanced event handling also includes namespace functionality to prevent naming conflicts in large projects, improving maintainability and scalability. Through methods such as `on()` and `off()`, jQuery offers significant control over event binding, enhancing flexibility and code organization.
Moreover, jQuery's event handling supports custom events, adding significant flexibility and power. These provide a powerful tool for creating reusable components and handling internal application communication. The capability to trigger custom events enables sophisticated internal messaging systems, making the application more robust and easier to maintain. This feature addresses a challenge commonly encountered when creating complex JavaScript applications where internal communication and synchronization are crucial.
AJAX and Asynchronous Operations
jQuery significantly simplifies AJAX requests. Its `$.ajax()` method provides a high-level abstraction, hiding much of the complexity of asynchronous communication. This results in concise code that is easier to write, read, and maintain. For example, making a GET request to a server becomes a simple matter of: `$.get("myUrl", function(data) { /* process data */ });`. This reduces the development time and increases the reliability of asynchronous operations, a significant advantage when working with remote data sources.
Case study 1: An online travel agency utilizes jQuery's AJAX capabilities to dynamically update flight availability and pricing information without requiring full page reloads. This provides a seamless user experience. Case study 2: A social networking platform uses jQuery's AJAX features to fetch and display updated posts or messages without interrupting the user's current activity. This ensures a fluid and responsive user experience.
jQuery's AJAX methods handle various HTTP methods (GET, POST, PUT, DELETE), streamlining communication with back-end servers. This comprehensive support for HTTP methods allows developers to create robust applications capable of handling diverse data manipulation requirements. The ability to easily handle JSON responses further enhances jQuery's usefulness in modern web development. Modern applications often use JSON as a primary data exchange format, and jQuery's efficient JSON handling makes working with these responses seamless.
Furthermore, jQuery's AJAX functionality includes error handling capabilities, making asynchronous operations more robust and resilient to network issues. The inclusion of error handling mechanisms enhances the reliability and stability of the application. By incorporating appropriate error handling, developers can ensure a smooth user experience and prevent application crashes due to network interruptions or server-side errors. This robust error handling is essential for creating applications that are reliable and responsive even under adverse network conditions.
In addition, jQuery's AJAX methods provide progress tracking, enabling developers to display progress indicators to users during long-running operations. Displaying progress indicators is crucial for user experience, preventing frustration caused by long waits for data. This provides users with feedback during data processing, keeping them informed about the progress of their request and improving their overall experience.
Plugins and Extensions
jQuery's vast ecosystem of plugins extends its functionality dramatically. Thousands of plugins provide ready-made solutions for common web development tasks, significantly accelerating development. From image sliders and carousels to form validators and date pickers, plugins offer pre-built components that save developers considerable time and effort. This rich ecosystem makes jQuery a highly adaptable framework capable of addressing a broad range of web development needs.
Case study 1: A photography website utilizes a jQuery image slider plugin to create an attractive and engaging presentation of photographs. This enhances the user experience by creating an interactive and visually appealing presentation. Case study 2: An e-commerce platform incorporates a jQuery form validation plugin to ensure users submit accurate and complete data. This enhances the reliability of data collection and reduces errors.
Choosing the right plugins for a project is crucial for maximizing efficiency and performance. Developers should carefully evaluate plugins based on their functionality, performance, and security. Considering factors such as code quality, community support, and maintenance history is crucial in ensuring the chosen plugins provide optimal benefits without compromising the stability or security of the project. Moreover, careful selection of plugins is a key step in optimizing the application's overall performance and maintaining a streamlined user experience.
Integrating plugins smoothly into an existing jQuery project is critical for maintaining code consistency and readability. Proper plugin integration involves understanding the plugin's documentation and utilizing the plugin’s API effectively. This process significantly affects the overall maintainability and scalability of the project. The consistent application of best practices in plugin integration ensures that the project's overall structure remains organized and its functionality remains coherent. This approach is essential for building maintainable and scalable applications.
Furthermore, security is a paramount concern when using plugins. Developers should carefully vet plugins for security vulnerabilities before incorporating them into their projects. Regularly updating plugins is crucial to mitigate any discovered vulnerabilities. These measures contribute to the overall security and integrity of the application, safeguarding user data and protecting the application from malicious attacks. This security consideration is fundamental in building trustworthy and secure web applications.
Modern jQuery Best Practices
While jQuery simplifies development, adopting best practices is crucial for maintainable and performant applications. Minimizing jQuery usage where vanilla JavaScript offers a simpler solution improves overall efficiency. Using vanilla JavaScript when appropriate makes the code cleaner and potentially faster. This balanced approach leverages the strengths of both approaches, enhancing performance and maintainability. This pragmatic approach avoids unnecessary framework overhead and enhances development efficiency.
Case study 1: A developer optimizes a website's performance by replacing jQuery's DOM manipulation functions with native JavaScript equivalents where appropriate. This improves the site's speed and responsiveness. Case study 2: A development team streamlines their codebase by using vanilla JavaScript for simple DOM manipulations while employing jQuery's strengths for more complex tasks involving AJAX and animation.
Efficiently managing jQuery's inclusion in a project is critical for performance. Employing techniques like minimizing and bundling jQuery code can significantly reduce the application's load time. These optimization strategies enhance the overall user experience by reducing delays. This is essential, especially on mobile devices and slow connections. Efficient management of jQuery code contributes to a faster loading time and a better user experience.
Furthermore, following modern JavaScript standards, such as using ES6 features alongside jQuery, enhances code clarity and maintainability. Employing contemporary JavaScript features enhances the overall quality and sophistication of the project. This creates cleaner, more organized, and more modern JavaScript code. This approach combines the best of jQuery's capabilities with modern JavaScript programming practices.
Moreover, rigorously testing code ensures that jQuery integrations function as expected and that performance issues are identified and resolved promptly. Regular testing provides confidence in the stability and reliability of the application. This process helps ensure a high-quality and well-functioning application. By thoroughly testing the integrated jQuery code, developers maintain a high-quality product.
Conclusion
jQuery, despite the rise of newer frameworks, remains a valuable tool for many web developers. Its concise syntax, powerful DOM manipulation capabilities, robust AJAX handling, extensive plugin ecosystem, and mature community support continue to make it a relevant choice for specific tasks. By adopting modern best practices, developers can harness jQuery's power while maintaining clean, efficient, and performant applications. Focusing on its strengths and integrating it strategically alongside vanilla JavaScript or other frameworks empowers developers to build sophisticated and effective web applications.