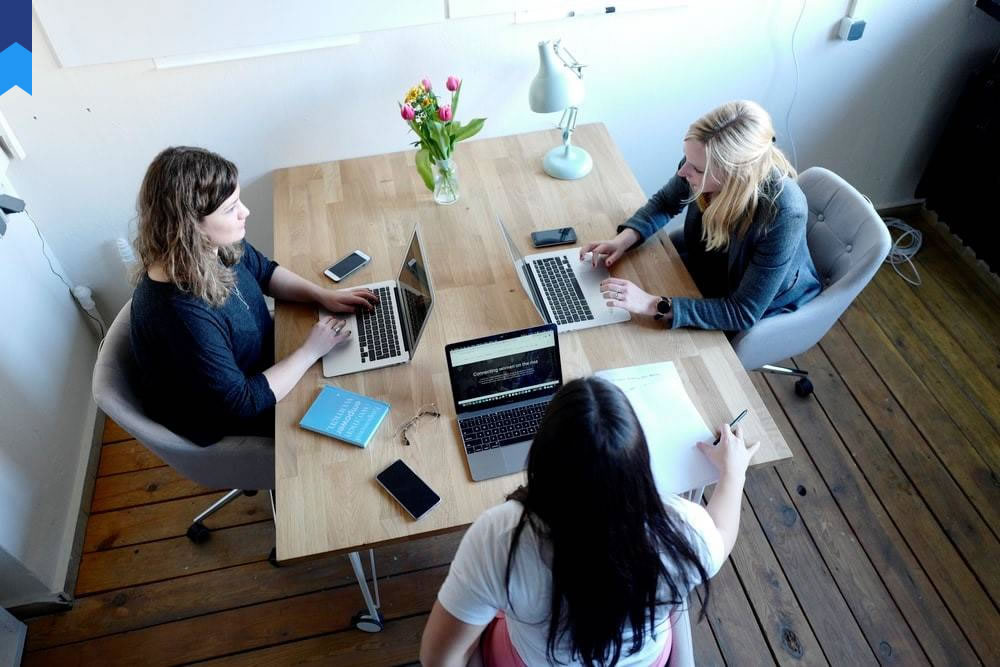
Rethinking Parallel Computing: A Fresh Perspective on Julia
Julia's rise as a high-performance computing language has been significant. Its innovative design blends the ease of use of interpreted languages like Python with the speed of compiled languages like C++. This unique combination makes it attractive for complex scientific computing, machine learning, and data analysis tasks. However, merely acknowledging its speed isn't enough; a deeper dive into its parallel computing capabilities reveals a new paradigm for efficiency and scalability.
Unlocking Julia's Parallel Power: Threads and Processes
Julia's strength lies in its seamless integration of parallel programming constructs. Unlike many languages where parallel programming requires significant code restructuring, Julia offers multiple approaches, including threads and processes, each with its strengths and weaknesses. Threads share memory space, making inter-thread communication fast but limiting scalability due to the Global Interpreter Lock (GIL) in some implementations. Processes, on the other hand, have independent memory spaces, offering better scalability but at the cost of slower inter-process communication. The optimal choice depends on the specific application and its memory requirements. For instance, a computationally intensive task with minimal data exchange between threads benefits from threading, while a task involving significant data sharing might be better suited to processes. Consider a large-scale numerical simulation – threads might excel at handling individual computations within the simulation, while processes could manage separate sub-simulations with independent datasets, combining results afterward. Another example is machine learning model training: parallelizing training across multiple datasets is efficiently handled using processes. A robust system requires a comprehensive understanding of both approaches and often involves a hybrid strategy.
Case Study 1: A financial modeling firm uses Julia's threading capabilities to accelerate risk assessment calculations by distributing the workload across available CPU cores. This drastically reduces computation time compared to serial processing, enhancing efficiency and enabling real-time analysis. Case Study 2: A research team analyzing climate data utilizes Julia's distributed computing features, leveraging processes to handle massive datasets spread across multiple servers. The parallel processing capability ensures efficient data analysis despite the scale.
Furthermore, Julia's package ecosystem is constantly evolving, providing powerful tools for parallel computing. Libraries like `Distributed`, `ThreadsX`, and `FLoops` simplify the development of parallel applications. These tools allow developers to easily distribute tasks across multiple cores or machines without low-level programming. The `@distributed` macro, for example, simplifies the process of distributing iterations of a loop across multiple processors, significantly reducing execution time for tasks involving loop iterations. This feature makes parallel computing far more accessible, empowering a wider range of developers to exploit the benefits of multi-core processing.
Efficient parallel programming necessitates careful consideration of data partitioning and communication overhead. Poorly designed parallel algorithms can lead to performance bottlenecks. Julia's design encourages the development of efficient data structures that minimize data movement between processes or threads. Optimized data structures are vital to reducing communication delays and improving overall performance. Data structures like arrays are optimized for parallel operations, allowing for faster processing within Julia compared to more traditional languages where parallel operations might necessitate the use of more complex structures. Techniques like data locality and load balancing are critical for maximizing parallel efficiency. Proper load balancing prevents one core from being overworked, leading to more even distribution of tasks and minimized wait times.
Mastering Julia's Asynchronous Programming
Asynchronous programming provides a unique advantage in handling I/O-bound tasks. Julia's robust support for asynchronous operations allows programs to remain responsive even while waiting for network requests, file access, or other slow operations. This contrast with synchronous programming, where a program blocks while waiting for an operation to complete, is profound. Julia's `async` and `await` keywords allow developers to write concise and efficient asynchronous code, enabling concurrent execution without the complexities of traditional threading models. Consider a web server handling multiple requests. A synchronous server processes one request at a time, leading to slower response times under heavy load. An asynchronous server, however, can handle many concurrent requests simultaneously, offering significant performance improvements. The `async` functions allow the server to begin processing the next request before completing the current one, drastically increasing throughput. Another example lies in data processing pipelines, where multiple independent tasks wait for data from preceding stages. Asynchronous programming prevents blocking, keeping the pipeline flowing smoothly.
Case Study 3: A real-time data analysis system uses Julia's asynchronous capabilities to efficiently process incoming streams from various sensors. This ensures low-latency responses to changing conditions. Case Study 4: A high-frequency trading algorithm leverages Julia's asynchronous programming model to react instantly to market fluctuations, minimizing latency and enhancing profitability.
Efficient utilization of asynchronous programming involves understanding how to structure code to avoid blocking operations and to effectively manage concurrency. Properly managing resources, such as threads and network connections, is essential for optimal performance in asynchronous systems. While asynchronous operations allow for concurrent task execution, they also introduce potential challenges like race conditions and deadlocks. Understanding these concepts and implementing strategies for managing concurrency are crucial for writing robust and efficient applications. Careful consideration must be given to how tasks are organized to maximize concurrency, ensuring tasks efficiently utilize available processing resources. In many situations, asynchronous programming offers a more efficient strategy than multithreading when I/O-bound operations dominate. This distinction is important, as improper implementation can lead to performance bottlenecks, negating the advantages offered by asynchronous programming.
Combining asynchronous programming with Julia's parallel features offers even greater performance gains. Asynchronous operations can be launched in parallel, further enhancing responsiveness and throughput. Such hybrid approaches are increasingly common in demanding applications. This combination of parallelism and asynchronous operations allows for extremely high performance in systems where operations involve both I/O and computationally intensive stages. Well-structured asynchronous code combined with intelligent task partitioning delivers superior performance. Consider this hybrid approach as a powerful technique to tackle complex modern problems.
Optimizing Julia Code for Parallel Execution
Writing efficient parallel Julia code involves optimizing both the algorithm and the implementation. Algorithm design is crucial; a poorly parallelizable algorithm will not benefit from parallel execution, no matter how efficient the implementation. Techniques like divide-and-conquer, where a problem is broken down into smaller subproblems that can be solved independently, are well-suited for parallel environments. Careful analysis of dependencies between tasks is vital in ensuring that tasks don't need to wait for other tasks, hence ensuring efficient parallelization. A well-designed algorithm will maximize the amount of work that can be performed concurrently. Poorly designed algorithms might introduce dependencies that severely limit the benefits of parallelism.
Case Study 5: A scientific simulation uses Julia's parallel capabilities to significantly reduce computation time by dividing the simulation into independent spatial regions. This task decomposition allows each region to be processed concurrently, greatly accelerating the overall computation. Case Study 6: An image processing application utilizes Julia’s parallel functionalities to divide a large image into smaller tiles, which are processed independently and combined to form the output image.
Efficient implementation requires careful attention to data structures and communication patterns. Julia's design makes it easier to work with arrays and other data structures optimized for parallel processing. Minimizing data transfer between processes or threads is essential. Proper communication patterns reduce overhead and prevent bottlenecks. Strategies like using shared memory for data exchange between threads or employing efficient message passing techniques for processes can significantly improve performance. Shared memory is often faster but only suitable for threads; message passing is more scalable for processes but introduces communication overhead.
Profiling tools are indispensable in identifying performance bottlenecks. Julia's built-in profiling capabilities, along with external tools, allow developers to pinpoint areas of code that are consuming excessive time. This targeted analysis helps to focus optimization efforts where they will have the greatest impact. Identifying bottlenecks through profiling is crucial for maximizing parallel performance; optimizing the right parts is crucial for efficiency. Performance analysis tools pinpoint computationally intensive sections, allowing developers to concentrate on optimizing critical code segments that hinder performance. Addressing these bottlenecks leads to significant performance improvements.
Leveraging Julia's Ecosystem for Parallel Applications
Julia's vibrant ecosystem provides a wealth of packages designed to facilitate parallel programming. Libraries like `MPI.jl` and `Distributed.jl` are specifically geared towards distributed computing, providing tools for running Julia code across multiple machines. These libraries simplify complex distributed programming tasks, making it accessible to a wider range of developers. They simplify the management of resources across a cluster of computers, efficiently distributing the workload and managing data exchange.
Case Study 7: A weather forecasting model leverages `MPI.jl` to distribute the workload across a high-performance computing cluster. This greatly accelerates the model's execution time, allowing for more frequent and accurate forecasts. Case Study 8: A large-scale data analysis project uses `Distributed.jl` to process a massive dataset by distributing the processing tasks among many machines, significantly reducing the time to complete the analysis.
Many other packages contribute to the parallel programming environment. Packages for linear algebra, optimization, and machine learning often include optimized parallel implementations of core algorithms. These specialized packages significantly reduce the effort needed to develop efficient parallel applications. The availability of highly optimized parallel libraries makes building high-performance applications much simpler and faster. The ease of integration with existing libraries reduces development time and maximizes the performance benefits of parallelism.
The community actively contributes to the development and improvement of these packages. This ongoing development ensures that Julia remains at the forefront of parallel computing technology. The active community support ensures that these tools remain up-to-date and efficient, constantly improving to match the demands of modern computing. This ongoing evolution makes Julia a compelling choice for anyone working on parallel applications.
Conclusion
Julia offers a compelling approach to parallel computing. Its elegant syntax, powerful features, and rich ecosystem provide a robust framework for developing high-performance applications. By understanding the nuances of threads, processes, and asynchronous programming, developers can unlock Julia's full potential. Furthermore, leveraging the existing ecosystem of packages and employing efficient coding practices will ensure that parallel applications are scalable, efficient, and effective. With its combination of ease of use and performance, Julia stands out as a key language for parallel programming in the future. The continuous growth of its community and ecosystem guarantees a vibrant and ever-improving parallel computing environment, making it a powerful tool for tackling the increasingly complex computational challenges of today and tomorrow.