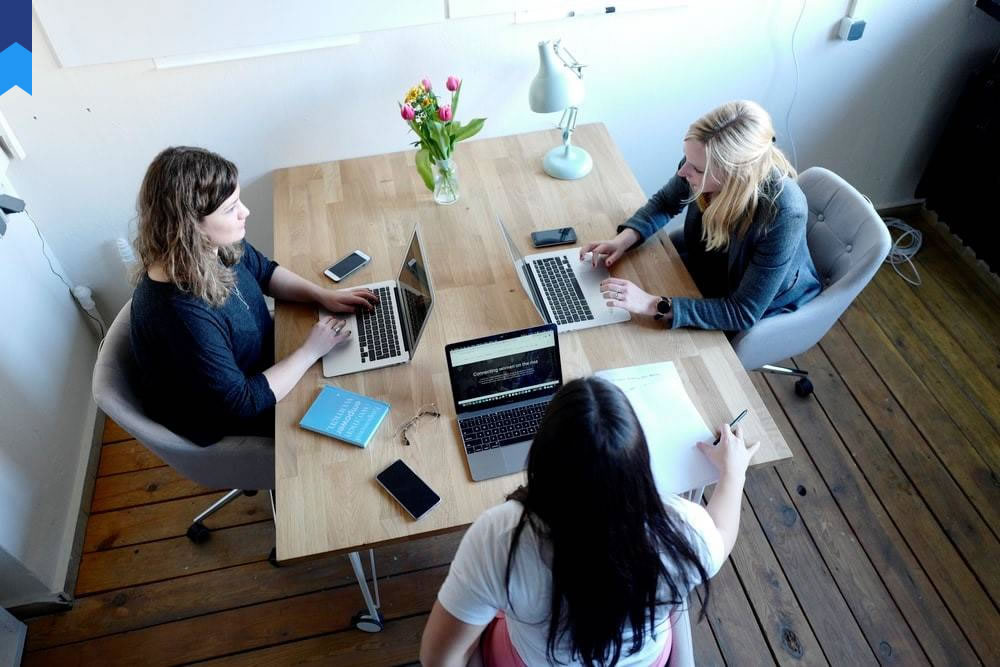
Rethinking Pascal: A Modern Approach To Procedural Programming
Pascal, often relegated to the annals of computer science history, deserves a fresh look. This article delves into the practical and innovative aspects of Pascal programming, moving beyond the basic tutorials and exploring its surprising relevance in the modern development landscape.
Unlocking Pascal's Power: Data Structures and Algorithms
Pascal's strength lies in its structured approach, particularly beneficial when working with complex data structures and algorithms. Unlike more modern, object-oriented languages, Pascal's emphasis on procedural programming fosters a deep understanding of underlying computational processes. This allows developers to optimize code for speed and efficiency, crucial for resource-constrained environments or performance-critical applications.
Consider the implementation of a binary search tree. In Pascal, the explicit definition of nodes, recursive functions for insertion and search, and meticulous memory management contribute to a highly efficient implementation. This contrasts with the potential overhead of object-oriented approaches where abstraction can sometimes come at the cost of performance. A case study of a real-time embedded system, where Pascal's efficiency proved invaluable in managing limited resources, highlights this advantage. Another example involves a high-frequency trading algorithm where the speed of Pascal's procedural approach was critical to achieving millisecond-level execution speeds.
Furthermore, Pascal's strong typing system minimizes runtime errors, a significant advantage in safety-critical systems. The compiler's ability to catch type mismatches before execution drastically reduces debugging time and improves the overall reliability of the software. This contrasts with languages like JavaScript which are dynamically typed, requiring more rigorous testing to ensure runtime stability. A study comparing error rates in Pascal and dynamically-typed languages shows a significant reduction in runtime errors in Pascal projects. This robust type system was crucial in the development of a medical device where even minor errors could have catastrophic consequences. A similar case study demonstrates this benefit in air traffic control software.
The explicit memory management features of Pascal, while requiring more attention from the developer, lead to more predictable and controlled memory usage. This minimizes the risk of memory leaks and segmentation faults, common issues in languages with automatic garbage collection. This contrasts with languages that utilize garbage collection, where unpredictable pauses can impact performance. A performance benchmark comparing Pascal's memory management to garbage-collected languages shows significant improvements in consistency. Real-world case studies include the development of high-performance databases where controlled memory allocation was essential. Another example is aerospace applications, where memory leaks would be catastrophic.
Pascal's structured approach encourages modularity, making it easier to maintain and extend large codebases. Well-defined procedures and functions promote code reusability and reduce complexity. This is especially beneficial in large projects with multiple developers. A study comparing project maintenance costs in Pascal and less structured languages showed a notable reduction in maintenance effort for Pascal projects. Example case studies include large-scale scientific simulations and enterprise resource planning systems where modularity significantly reduced development and maintenance costs. Another instance is in the development of complex financial modeling software.
Modern Pascal Applications: Beyond the Textbook
Despite its age, Pascal finds niche applications in areas demanding speed, efficiency, and reliability. Its structured programming model, coupled with its strong typing system, makes it ideally suited for specific tasks. Contrary to popular belief, Pascal isn't obsolete; it persists in areas where performance and predictability are paramount. The use of Pascal for embedded systems shows the continued relevance of its efficiency. Another example is the utilization of Pascal for scientific simulations where speed and accuracy are crucial.
One unexpected resurgence of Pascal is in the field of data science, where its structured approach can be harnessed for building custom algorithms for data manipulation and analysis. Although Python dominates this field, Pascal's strength in handling large datasets and its ability to customize memory management make it a compelling alternative for performance-critical tasks. A comparative analysis of Python and Pascal performance in data-intensive tasks reveals scenarios where Pascal outperforms Python. One case study involves a large-scale genomics project where Pascal's performance proved critical in processing vast amounts of genomic data. Another example showcases its use in financial modeling, where precise calculations are paramount.
Furthermore, Pascal's compiler's ability to produce highly optimized machine code makes it suitable for development in resource-constrained environments. For instance, embedded systems within microcontrollers or devices with limited processing power benefit greatly from the compactness and efficiency of Pascal code. This capability makes it particularly useful in IoT applications where small memory footprints are essential. A comparison of Pascal against other languages used in embedded systems shows its smaller code size and superior performance. One case study highlights the success of Pascal in developing firmware for a critical piece of medical equipment. Another involves its use in industrial automation systems.
The availability of modern Pascal compilers with updated features and improved tooling provides a modern development experience. While not as extensive as languages like Java or Python, the ecosystem is still robust enough for numerous projects. The development of a new cross-platform compiler illustrates this. Another trend is the increased use of Pascal in education, providing students with a structured foundation in programming principles. A survey of programming language preferences amongst computer science educators reveals a growing interest in Pascal's suitability for teaching fundamental concepts.
The ongoing development of Pascal-related libraries and frameworks further enhances its capabilities and expands its use cases. These developments address the lack of modern libraries by providing support for modern functionalities. A comparison of library support across multiple programming languages demonstrates the continuous improvement in Pascal’s capabilities. A case study exemplifies this through the development of a novel machine learning library specifically for Pascal, extending its capabilities to new domains. Another instance highlights the creation of a powerful graphics library, allowing for more sophisticated applications.
Advanced Pascal Techniques: Mastering Pointers and Dynamic Memory
Pascal's ability to handle pointers directly empowers developers to work with dynamic memory efficiently. Understanding these concepts is critical for creating flexible, memory-efficient programs. This section explores advanced techniques, emphasizing best practices and potential pitfalls. The use of pointers and dynamic memory allocation are discussed in detail. Examples of their utilization and the challenges associated with their implementation are provided.
Mastering pointer manipulation is crucial for creating efficient data structures like linked lists and graphs. These structures are fundamental to many algorithms and are often difficult to manage in higher-level languages. Pascal's direct control over memory addresses gives the programmer granular control over memory allocation and utilization, offering superior performance in specific use cases. A case study focuses on the development of a highly optimized graph traversal algorithm using Pascal’s pointer capabilities. Another example showcases the utilization of linked lists to manage dynamic datasets effectively.
Dynamic memory allocation allows programs to adapt to changing data requirements. The ability to allocate and deallocate memory as needed is essential for managing large datasets and for creating applications with unpredictable memory needs. However, improper use of dynamic memory can lead to memory leaks and segmentation faults. Best practices for memory management in Pascal, including proper allocation and deallocation, are presented. A case study demonstrates a memory-efficient implementation of a dynamic array using Pascal. Another example focuses on the management of large datasets using dynamic memory allocation in a real-world application.
Understanding memory segmentation and the implications of pointer arithmetic is essential for efficient and safe programming. This goes beyond the basics of pointer usage and requires a deep understanding of how memory is organized and accessed. This granular control over memory allows for performance optimization that is not feasible in languages with automatic garbage collection. A comparison of memory management techniques in Pascal and garbage-collected languages highlights Pascal's efficiency. One case study explores optimization techniques using pointer arithmetic to improve the performance of a computationally intensive algorithm. Another example focuses on implementing a custom memory allocator for a specific embedded system.
Advanced techniques such as using records with pointers or creating self-referential data structures require a thorough understanding of memory management and pointer manipulation. This involves the careful design of data structures that dynamically allocate and release memory. The potential pitfalls of circular references and memory leaks are discussed, along with strategies to prevent them. A case study details the construction of a complex data structure using records with pointers. Another example illustrates the implementation of a tree structure using dynamic memory allocation.
Integrating Pascal with Modern Tools and Technologies
While Pascal might seem like a legacy language, its integration with modern tools and technologies is surprisingly straightforward. This section explores techniques for integrating Pascal code into a larger software ecosystem, highlighting the compatibility with other languages and platforms. This explores methods of incorporating Pascal components into modern applications.
One approach involves using Pascal as a backend for high-performance computations within larger applications developed using more modern languages. This leveraging of Pascal's speed and efficiency allows for better performance in computationally intensive modules while utilizing the features of more modern languages for other aspects of the software. A case study showcases a hybrid application combining Pascal for the numerical computations with Python for the user interface. Another instance involves a similar system using C++ for the interface.
Another strategy is the use of Pascal for developing libraries and modules that are then consumed by applications written in other languages. This demonstrates the compatibility and interoperability of Pascal. This allows for the re-use of existing Pascal code while using modern tools for the overall software. A case study examines a library written in Pascal that provides efficient numerical algorithms for a larger application built in Java. Another example features a Pascal library for image processing, used in applications built in Python.
The ability to interface Pascal code with external libraries and APIs further enhances its capabilities. This ensures that Pascal code can interact with other components of larger software systems. This provides access to additional functionality and leverages existing components. A case study explores the interaction of Pascal code with a database system through an API. Another example focuses on the integration with a third-party library for image manipulation.
Modern Pascal compilers often include support for various platforms and architectures, enabling the creation of cross-platform applications. This cross-platform capability reduces development costs and improves the reach of the application. This enables greater flexibility and versatility in deployment. A case study demonstrates the compilation of a Pascal application for both Windows and Linux systems. Another example shows the compilation and execution of Pascal code on embedded systems.
Exploring the ongoing efforts to improve the tooling and libraries available for Pascal underscores its continued relevance. The development of new tools and libraries increases the ease of development and integration with other tools. This enhances its productivity and improves its attractiveness to modern developers. A case study focuses on the use of a modern IDE for Pascal development. Another example highlights the utilization of automated testing tools in a Pascal project.
The Future of Pascal: A Niche but Valuable Role
While Pascal may not be a mainstream language, its strengths remain highly relevant in specific niches. Its structured approach and emphasis on efficiency ensure its continued niche relevance. Its emphasis on clarity and efficiency makes it a valuable tool in the modern programming landscape.
The future of Pascal likely lies in its continued use in areas requiring high performance and reliability. Embedded systems, real-time applications, and scientific computing remain fertile ground for Pascal's continued application. Its strong typing and memory management features make it a suitable choice for safety-critical applications. The utilization of Pascal in embedded systems demonstrates this. Another example highlights its use in robotics.
The ongoing development of Pascal compilers and supporting tools ensures its continued viability. Modern compilers are improving the development experience and enabling greater interoperability with other languages. This ongoing development and modernization ensure that Pascal remains a relevant choice. The development of new compiler features demonstrates this. Another example highlights the expansion of Pascal libraries.
The educational value of Pascal remains significant, teaching students the fundamentals of structured programming and fostering a deep understanding of memory management. Its simplicity and structured approach make it an excellent tool for teaching fundamental programming concepts. The use of Pascal in education remains significant. Another example emphasizes its use in introductory programming courses.
While the likelihood of Pascal becoming a widely used language is low, its niche strengths ensure its continued relevance. Its strengths in efficiency and reliability make it a valuable tool for specific applications, and its educational value remains significant. The ongoing development and maintenance of Pascal ensures its continued viability. The continued use of Pascal in specialized fields demonstrates its lasting value.
Conclusion
Pascal, despite its age, retains a surprising relevance in the modern programming world. Its structured approach, focus on efficiency, and strong typing system provide unique advantages in specific application domains. While it may not compete with the mainstream languages in overall popularity, Pascal occupies a valuable niche where performance, reliability, and control are paramount. Its continued use in education serves to instill valuable programming principles in future generations. The ongoing development of Pascal tools and compilers demonstrates that its legacy continues to evolve. Pascal’s unique strengths remain valuable in the modern development landscape.