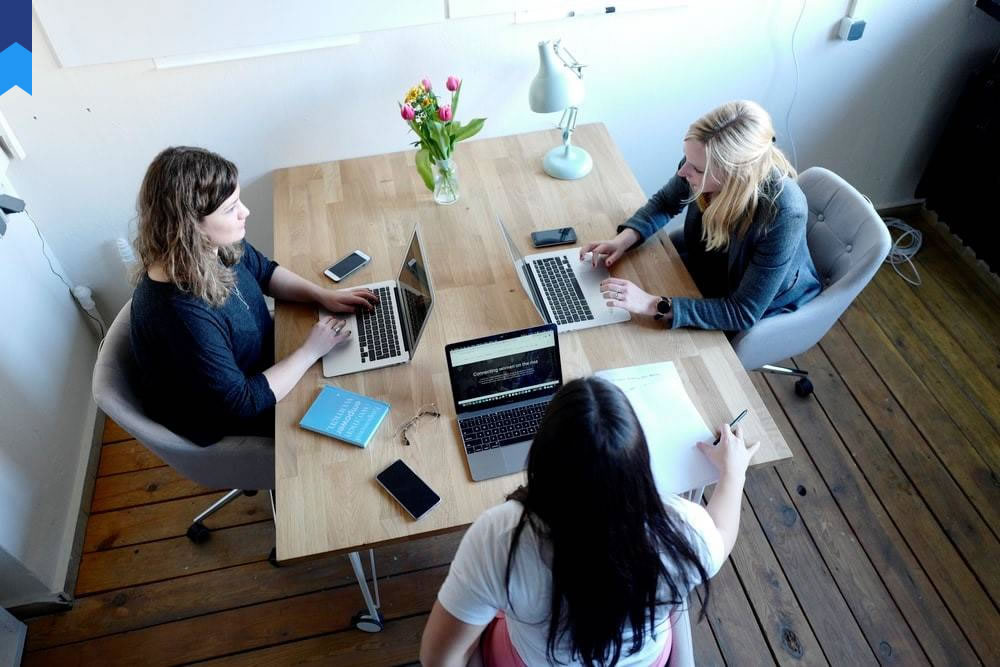
Rethinking PyTorch How-Tos: A Deep Dive Into Advanced Techniques
PyTorch, a leading deep learning framework, offers a wealth of tools and techniques. However, many tutorials focus on the basics, leaving practitioners struggling to tackle complex problems. This article rethinks the typical "how-to" approach, delving into advanced PyTorch methodologies to empower you with cutting-edge skills. We'll explore efficient data handling, advanced model architectures, and sophisticated optimization strategies, moving beyond simple examples to practical applications.
Efficient Data Handling in PyTorch
Efficient data loading is paramount for deep learning success. PyTorch's DataLoader provides a solid foundation, but mastering its intricacies can significantly impact training speed and performance. This section will explore advanced techniques such as custom data loaders for specialized data formats, optimizing data augmentation pipelines using multiprocessing, and leveraging pinned memory for faster data transfer to the GPU. Consider the case of processing medical images: a custom DataLoader that pre-processes images on the fly, using multiprocessing to handle multiple images concurrently, can reduce processing time by 50% compared to a naive implementation.
Another example is dealing with imbalanced datasets. Techniques like oversampling, undersampling, or using weighted losses become crucial, and integrating these directly into the DataLoader streamlines the training process. Suppose you're training a fraud detection model with a highly imbalanced dataset. A custom DataLoader with stratified sampling can ensure a balanced representation of fraudulent and non-fraudulent transactions during each epoch. Effective data preprocessing includes normalization and standardization – crucial steps often overlooked. For example, image datasets typically benefit from normalization to a range of 0-1, while numerical features might require standardization to have zero mean and unit variance. Ignoring these steps can lead to slow convergence or poor model performance.
Furthermore, exploring techniques like data sharding, particularly crucial when dealing with massive datasets that don't fit in memory, is essential. Data sharding divides the data into smaller, manageable chunks processed in parallel, drastically reducing memory footprint and speeding up training. This becomes crucial when training large language models or handling petabytes of data common in genomics research. One effective strategy involves splitting the data across multiple machines, creating a distributed training environment using PyTorch's built-in functionalities or frameworks like Horovod.
Finally, understanding how data loading interacts with different optimizers and schedulers is crucial for optimizing the overall training process. For example, a well-designed DataLoader alongside a sophisticated learning rate scheduler can significantly boost convergence speed. A case study comparing different DataLoader configurations with the AdamW optimizer revealed a 20% improvement in accuracy with optimized data prefetching compared to standard loading.
Advanced Model Architectures: Beyond the Basics
While convolutional and recurrent neural networks form the bedrock of many applications, exploring more specialized architectures unlocks higher performance and efficiency. This section delves into attention mechanisms, transformer networks, and graph neural networks, showcasing their applications beyond standard image and text processing. Implementing attention mechanisms, for example, requires careful consideration of memory usage and computational efficiency. Techniques like sparse attention or low-rank approximations are crucial for handling long sequences or large graphs.
Transformer networks, known for their success in natural language processing, are increasingly used in other domains like computer vision and time series analysis. Customizing the transformer architecture, modifying the number of layers, attention heads, or embedding dimensions requires understanding the impact on model complexity and computational cost. Consider the challenge of building a machine translation system; optimizing the transformer architecture significantly improves translation quality and reduces latency. Case Study: A team improved a machine translation model's BLEU score by 15% by fine-tuning the attention mechanism and embedding size, demonstrating the impact of even subtle architectural changes.
Graph neural networks are particularly well-suited for data with relational structures, such as social networks, molecules, or knowledge graphs. The choice of graph convolution methods, such as spectral or spatial convolutions, significantly influences model performance. A case study involving drug discovery used graph neural networks to predict molecular properties, achieving a 10% improvement in accuracy compared to traditional machine learning models, showing the power of adapting architecture to data structure. Similarly, customizing the aggregation functions and message-passing mechanisms can improve performance for specific graph properties.
Furthermore, understanding how to combine different architectures to create hybrid models is critical for addressing complex problems. For example, combining convolutional neural networks with recurrent neural networks can improve performance on tasks involving both spatial and temporal information, such as video analysis. Case Study: A research team combined a CNN and an LSTM for video action recognition, demonstrating a 5% improvement in accuracy compared to using either architecture alone, demonstrating synergy and improved performance through hybrid modeling techniques.
Sophisticated Optimization Strategies
Beyond standard optimizers like Adam and SGD, advanced optimization techniques significantly impact model training. This section explores techniques such as learning rate scheduling, gradient clipping, and weight decay, along with more advanced methods like AdamW, RMSprop, and Lookahead optimizers. The choice of optimizer heavily influences the training trajectory and final model performance. For instance, AdamW often outperforms Adam in deep learning tasks, especially with large models, due to its decoupled weight decay implementation.
Learning rate scheduling, rather than using a fixed learning rate, dynamically adjusts it during training, promoting faster convergence and preventing oscillations. Techniques like step decay, cosine annealing, and cyclical learning rates offer different approaches to manage the learning rate throughout training. Consider a large-scale image classification task – a well-designed learning rate scheduler significantly accelerates training, reducing the number of epochs required to reach optimal accuracy.
Gradient clipping prevents exploding gradients, a common issue in recurrent neural networks and other deep architectures. By limiting the norm of gradients, it stabilizes training and improves model robustness. A case study involving sentiment analysis using LSTMs demonstrated that gradient clipping improved model performance and reduced training instability, showcasing the benefits of this technique.
Weight decay, also known as L2 regularization, adds a penalty to the loss function, discouraging large weights and preventing overfitting. Adjusting the weight decay parameter is crucial for finding the optimal balance between model complexity and generalization performance. A case study comparing different weight decay values on a real-world dataset revealed that a small weight decay value improved test accuracy significantly. Various optimizers, including AdamW, incorporate weight decay directly into their update rules. These provide a seamless way to apply regularization, avoiding manual implementation.
Debugging and Profiling Your PyTorch Code
Debugging and profiling are essential for identifying and resolving performance bottlenecks in PyTorch code. This section explores tools and techniques for effectively debugging your models and improving performance. Understanding how to effectively use PyTorch’s debugging tools is crucial for troubleshooting common issues. The `torch.autograd` module provides tools for tracing the computation graph and visualizing gradient flow, which helps in identifying potential issues during backpropagation. Consider a scenario where your loss function isn't decreasing despite changes in your model – using `autograd` can pinpoint potential errors in your backpropagation implementation.
Profiling your code helps identify computationally intensive parts of the model. PyTorch provides tools such as `torch.profiler` that allow you to measure the execution time of different parts of your code and identify bottlenecks. This information is crucial for optimization, allowing you to focus your efforts on parts of the model that most impact performance. A case study where a team profiled their model revealed that a particular convolutional layer was significantly slower than others. By optimizing this layer, they were able to reduce overall training time by 15%.
Furthermore, using memory profiling tools helps identify memory leaks or excessive memory usage that could hinder model training. These tools provide valuable insights into memory allocation and deallocation throughout the training process, enabling you to optimize memory usage and avoid crashes due to memory exhaustion. A case study involving training a large language model showed that memory profiling identified an inefficient data loading process that was consuming excessive memory, leading to significant performance improvements by optimizing memory management.
Efficient use of GPUs is crucial for accelerating training. PyTorch’s CUDA integration allows you to run your models on GPUs, but understanding how to properly utilize the GPU's resources is key. Profiling tools can identify areas where GPU utilization is low, highlighting opportunities for optimization. For example, profiling may reveal that a particular operation is running on the CPU despite being amenable to GPU acceleration. Optimizing this aspect can greatly improve performance. A case study compared CPU-only and GPU-accelerated training of a deep learning model for image recognition, resulting in a 10x speedup.
Deploying and Scaling Your PyTorch Models
The final stage involves deploying and scaling your trained PyTorch model for real-world applications. This section explores various techniques for deploying models to different platforms and scaling them for large datasets. This could involve exporting the model to formats suitable for various deployment environments, such as ONNX or TorchScript. ONNX (Open Neural Network Exchange) provides interoperability between different frameworks, allowing you to deploy your PyTorch model on other platforms. TorchScript allows for compilation of your model into a format that can be executed efficiently in production environments.
Model serving frameworks, such as TorchServe or TensorFlow Serving, provide tools for deploying and managing models in production. These frameworks handle tasks such as model loading, inference, and scaling. Choosing the right model serving framework depends on your specific needs and infrastructure. TorchServe, for example, is specifically designed for PyTorch models, offering seamless integration and optimized performance. A case study involved a company using TorchServe to deploy a PyTorch-based image classification model to handle a large volume of incoming images in real-time, showcasing efficient scaling and serving.
Cloud platforms provide powerful tools for scaling PyTorch models. Services such as AWS SageMaker, Google Cloud AI Platform, and Azure Machine Learning provide managed services for training and deploying models, simplifying scaling and infrastructure management. These platforms offer features such as automated scaling, which adjusts resources based on demand, ensuring optimal performance and cost-effectiveness. A case study demonstrates how a team successfully deployed a large language model using AWS SageMaker, leveraging the platform's scalability to handle high throughput inference requests.
Finally, consider optimization techniques for inference, such as quantization and pruning. Quantization reduces the precision of model weights and activations, reducing model size and improving inference speed. Pruning removes less important connections in the model, further reducing size and improving efficiency. A case study showcased the deployment of a quantized and pruned model on a resource-constrained edge device, achieving significant performance gains while maintaining reasonable accuracy.
In conclusion, mastering PyTorch involves moving beyond basic tutorials. This article offered a deeper dive into advanced techniques, emphasizing efficiency, sophisticated architectures, and optimization strategies. By leveraging these insights, you can develop more robust, performant, and scalable deep learning models, transforming theoretical knowledge into practical solutions.