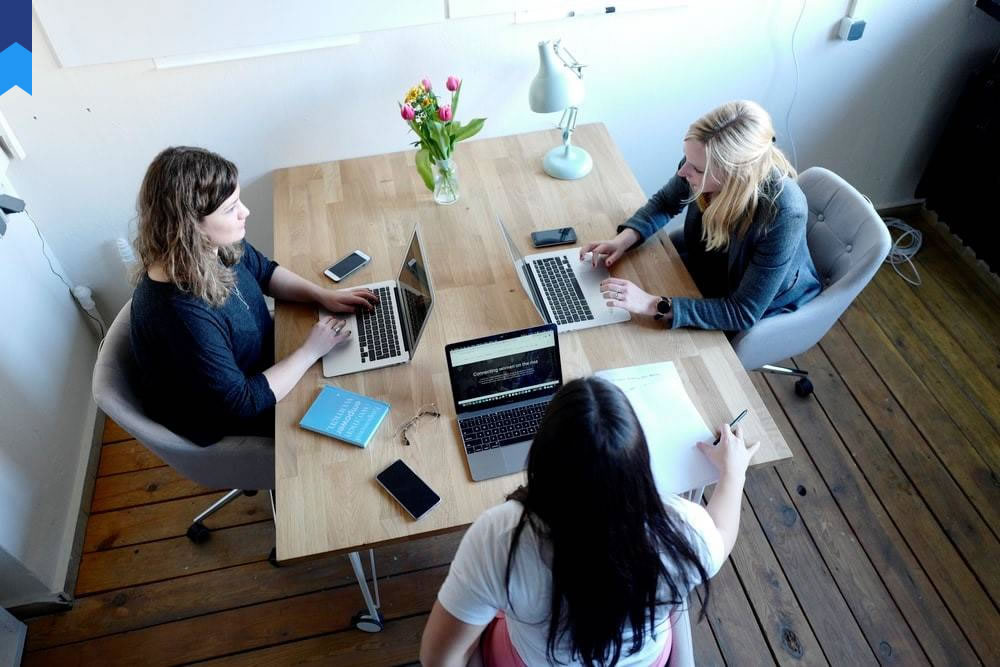
Smart BackboneJS Architecture Decisions
Backbone.js, a lightweight JavaScript framework, offers a powerful yet flexible approach to structuring complex web applications. However, its flexibility can be a double-edged sword, leading to architectural decisions that either streamline development or create maintainability nightmares. This article delves into the crucial architectural choices developers face when employing Backbone.js, emphasizing strategies that promote scalability, testability, and long-term maintainability.
Backbone Model Design: Mastering Data Representation
The Backbone Model forms the cornerstone of your application's data layer. Effective model design is crucial for managing data integrity and simplifying data interactions. Consider using a normalized data structure to avoid redundancy and facilitate efficient data manipulation. Employing inheritance to create base models allows for code reuse and maintainability. For instance, a base `BaseModel` can handle common functionalities like validation and persistence, while specialized models extend it to represent specific data entities.
Case study 1: A large-scale e-commerce platform might have a `Product` model as a base model. Then, specialized models like `ClothingProduct`, `ElectronicsProduct`, and `BookProduct` could inherit from it, adding specific attributes and behaviors.
Case study 2: A social networking application could have a base `User` model. Specialized models like `AdminUser` and `RegularUser` could then inherit and implement specific permissions and functionalities.
Careful consideration of attribute naming conventions improves consistency and readability. Avoid abbreviations unless they are universally understood. Extensive use of comments can make the code easier to understand in the long run. Validations should be implemented to ensure data integrity; this prevents corrupted data from reaching your application's backend.
Always thoroughly document your model's attributes, their data types, and any constraints. This documentation serves as an invaluable resource during future development and maintenance. Properly chosen attributes can minimize data redundancy and enhance data consistency across your entire application.
Furthermore, ensure models are designed for extensibility. Future feature additions should not require massive changes to existing models. Proper planning ensures that adding new features is a simple process.
A well-structured model architecture increases efficiency by minimizing redundant data and maximizing data integrity.
By adhering to clear naming conventions, incorporating comprehensive validations, and utilizing inheritance effectively, developers can build a robust and scalable model layer that supports the entire application.
Adopting best practices like using a dedicated model layer for data management significantly improves the organization and efficiency of the entire development process.
Consistent use of these strategies helps prevent common problems associated with poorly designed data models and fosters a clean and maintainable codebase.
Remember to utilize the built-in Backbone.js features to fully leverage its capabilities and to write clean, well-organized code.
View Organization: The Art of Presentation
Backbone Views handle the presentation logic, connecting your Models to the user interface. Structuring your views effectively is essential for maintainability and scalability. Employing a modular approach, breaking down complex views into smaller, reusable components, is vital. Each component should handle a specific portion of the UI, making it easier to understand, test, and maintain. Consider using a templating engine like Underscore.js or Handlebars.js to separate presentation logic from view code.
Case study 1: A complex dashboard could be broken into individual widgets, each representing a specific metric or data visualization, rendering each as a separate view.
Case study 2: A blog post page could be split into separate views: the header (title, author, date), the content area, and the comments section. Each could be a separate, reusable view.
Maintain separation of concerns by keeping the view focused solely on rendering and updating the UI. Avoid placing business logic within views. Instead, delegate such tasks to models or controllers. Leverage event-driven communication between models and views, enabling seamless data updates based on model changes. This approach promotes clean, reusable, and easily testable code.
Consistent use of naming conventions for view elements makes the code easily understandable. Comments are also crucial for readability. Proper use of CSS can enhance the separation of presentation logic from view code, promoting a cleaner and more maintainable codebase.
Choosing a templating engine adds to the clarity of view code, making it cleaner and more readable. Effective use of events reduces complexity and improves code readability.
Adhering to these principles will improve the quality of code and overall efficiency of the software.
Utilizing best practices in view organization is crucial for creating a scalable and maintainable application.
Properly structured views enhance the overall developer experience and reduce the time needed to complete projects.
Employing a modular approach promotes code reusability and prevents repetitive code, simplifying the development process.
Effective use of event handling leads to more responsive and dynamic applications, enhancing user experience.
Router Management: Navigating Your Application
The Backbone Router manages application navigation and URL routing. Define routes that map URLs to specific views and actions. A well-structured router ensures a seamless user experience, allowing users to navigate through different sections of your application effortlessly. Ensure clear naming conventions for routes to aid in understanding and maintenance.
Case study 1: An e-commerce application might have routes for product listings, individual product pages, shopping carts, and checkout processes.
Case study 2: A social media platform could use routes for user profiles, news feeds, message threads, and search results.
Leveraging parameterized routes enables flexible navigation by passing data dynamically. This eliminates the need for hardcoding specific URLs and makes your application more versatile. Properly using events allows the router to communicate effectively with views.
Clear documentation of routes is essential for maintaining the router's functionality. This documentation is crucial for understanding how routes interact with views and other parts of the application. Good code commenting makes the router's actions easy to understand.
Using best practices in route management improves the quality of code and creates a more consistent application.
Robust routers improve application responsiveness and provide a better user experience.
A modular approach to route design helps in managing complex applications more effectively.
Thorough testing of routers is crucial for preventing unexpected application behavior.
Well-organized routers make applications easier to debug and maintain.
Collection Strategies: Data Aggregation and Management
Backbone Collections provide a structured way to manage multiple models. Effective collection usage simplifies data manipulation and interaction. Using nested collections allows for complex data structures that can effectively represent relationships between models. The proper use of collection methods like `fetch`, `add`, and `remove` provides efficient data management.
Case study 1: An e-commerce application could use collections to manage lists of products, orders, or shopping carts.
Case study 2: A social media platform may use collections to represent users' news feeds, lists of followers, or comments on posts.
Implement custom comparator functions to efficiently sort and filter collections. This enhances application performance, enabling quicker response times. Careful consideration of when to fetch data from the server minimizes unnecessary network requests.
Thorough documentation of collections is essential. This improves maintainability and aids in understanding the role of each collection in the application. Comments on functions and their purposes are also crucial for readability.
Using best practices in collection management improves application efficiency and data integrity.
Well-structured collections ensure seamless interactions between the data layer and user interface.
Proper use of built-in Backbone methods minimizes coding effort and maximizes code reusability.
Efficient use of collections prevents issues like data inconsistency and reduces overall application complexity.
Choosing appropriate collection strategies reduces the risk of performance problems.
Testing and Maintainability: Ensuring Long-Term Success
Testing and maintaining Backbone.js applications is crucial for long-term success. Writing unit tests for models, views, routers, and collections ensures code quality and prevents regressions. Employing a testing framework like Jasmine or Mocha simplifies the testing process, making it easier to catch bugs early.
Case study 1: Testing a model's validation logic ensures that only valid data is stored.
Case study 2: Testing a view's rendering logic prevents rendering errors in the UI.
Implementing continuous integration and delivery (CI/CD) pipelines automates testing and deployment processes, ensuring efficient development cycles. Refactoring improves code readability and maintainability over time. The use of linters and code formatters ensures code consistency across the project.
Proper code documentation and comments help in understanding and maintaining the application. Thorough documentation for future developers is vital to the long-term success of the project. Clear documentation also simplifies the troubleshooting of potential errors.
Prioritizing testing and maintainability results in more robust and scalable applications.
Well-tested applications have reduced bugs and higher reliability.
A well-maintained application is easily adaptable to future requirements and changes.
Good maintainability reduces development costs and improves team productivity.
Thorough testing and regular code reviews are crucial for identifying and resolving issues promptly.
Conclusion
Building robust and scalable Backbone.js applications requires careful consideration of architectural choices. From meticulous model design to well-structured views and a streamlined router, each decision impacts the application's maintainability, performance, and overall success. By embracing best practices such as modularity, separation of concerns, and a robust testing strategy, developers can create applications that are not only functional but also easily maintainable and adaptable to future needs. Remember, proactive planning and a commitment to code quality are key ingredients in creating a successful Backbone.js application.