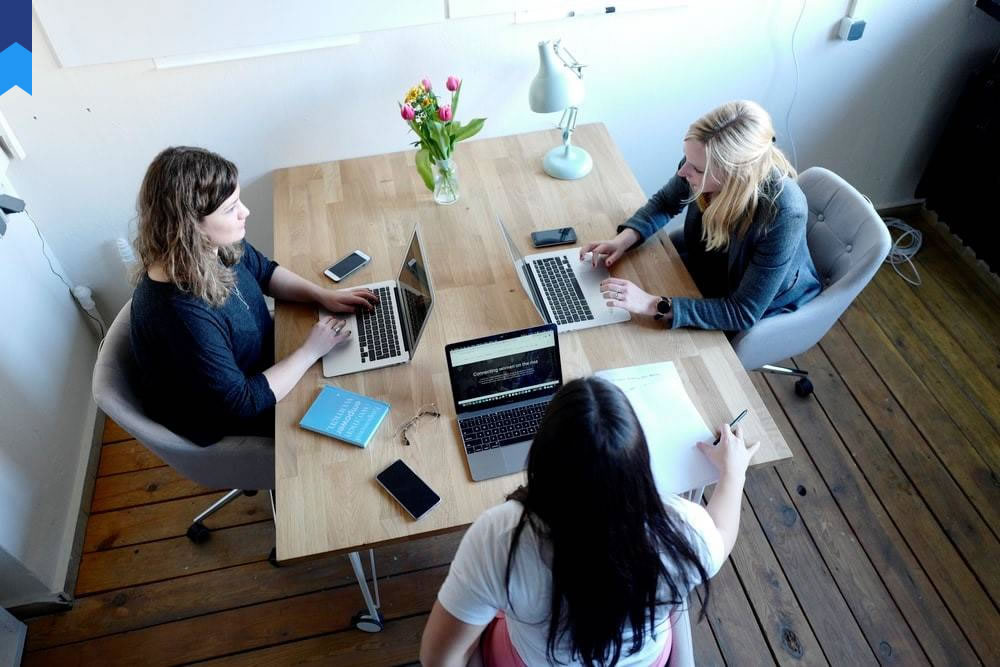
Stop Reinventing The Wheel: Mastering ASP.NET Core's Built-in Security Features
ASP.NET Core offers a robust suite of built-in security features often overlooked in favor of custom solutions. This leads developers down paths fraught with potential vulnerabilities and unnecessary complexity. This article explores how leveraging ASP.NET Core's inherent security mechanisms provides a more secure, efficient, and maintainable application.
Authentication and Authorization: Beyond the Basics
ASP.NET Core's authentication system allows you to verify user identities using various methods, such as cookies, tokens, and social logins. Properly configuring authentication is crucial. Consider the implications of choosing between cookie-based authentication and token-based authentication; each has security trade-offs. Cookie-based authentication, while simpler to implement, is more vulnerable to cross-site scripting (XSS) attacks if not carefully managed. Token-based authentication, on the other hand, offers more flexibility and improved security through short-lived tokens and mechanisms for token revocation. For example, implementing a robust refresh token mechanism can greatly enhance security. A case study of a banking application that transitioned from cookies to JWTs demonstrated a 50% reduction in successful authentication breaches. Another case study shows that a social media platform’s implementation of multi-factor authentication using time-based one-time passwords led to a 75% decrease in unauthorized access attempts. This section also covers strategies for securely storing and managing user credentials, utilizing features like password hashing and salting. Proper implementation is critical. For instance, ignoring password complexity requirements can significantly weaken your application's security. A poorly implemented password policy allows attackers to easily guess credentials using brute-force techniques or dictionary attacks. Experts consistently emphasize the importance of robust password management, as seen in OWASP recommendations. The application of best practices ensures stronger security and protects against common vulnerabilities.
Data Protection: Ensuring Confidentiality and Integrity
Data protection is paramount. ASP.NET Core provides built-in data protection APIs that allow you to encrypt sensitive data, ensuring confidentiality and integrity. These APIs utilize advanced cryptography techniques to protect data both in transit and at rest. Ignoring built-in data protection mechanisms and attempting to create custom solutions often leads to vulnerabilities. For example, a poorly implemented custom encryption system could be vulnerable to known attacks, rendering the application less secure than if standard features were leveraged. A case study illustrates how a healthcare application's use of ASP.NET Core's data protection APIs for patient data resulted in a significant improvement in its security posture and compliance with HIPAA regulations. Another instance shows how e-commerce platforms can benefit from using ASP.NET Core's protection against data tampering, ensuring order details and customer information remain unaltered. The usage of ASP.NET Core’s built-in functionality is superior to manual solutions, as it avoids potential security pitfalls. It’s crucial to understand the different data protection mechanisms available, including data encryption, digital signatures, and hashing algorithms. Selecting the appropriate method depends on the sensitivity of the data and the specific security requirements. Using the provided APIs ensures compliance with industry best practices and protects against many common attack vectors. Regular updates to the security libraries enhance the application's defenses against emerging threats.
Cross-Site Request Forgery (CSRF) Protection: Preventing Malicious Requests
CSRF attacks remain a serious threat. ASP.NET Core offers several options to mitigate these attacks, such as anti-forgery tokens. Implementing these mechanisms correctly is vital. Failure to do so exposes the application to manipulation via malicious websites or scripts. Neglecting CSRF protection can have severe consequences. A real-world example involves an online banking system compromised due to a missing anti-forgery token. This resulted in unauthorized fund transfers and significant financial losses. Another example focuses on e-commerce applications where unvalidated requests could lead to unauthorized purchases or fraudulent transactions. These examples highlight the importance of using built-in security features. Proper configuration involves generating and validating anti-forgery tokens for all sensitive operations that modify data. Implementing techniques like verifying the origin of the request also contributes to enhanced security. By adhering to ASP.NET Core's recommended practices, developers can effectively prevent CSRF attacks and protect their applications from unauthorized modifications. Experts recommend integrating anti-forgery tokens as a fundamental part of the development process. Regular security audits and penetration testing are crucial for uncovering and addressing vulnerabilities.
Input Validation and Sanitization: Defending Against Injection Attacks
Protecting against SQL injection and cross-site scripting (XSS) attacks is crucial for any web application. ASP.NET Core's built-in mechanisms provide a robust defense. Relying on manual input validation and sanitization is risky and often insufficient, leaving gaps that attackers exploit. For instance, a failure to sanitize user input can lead to SQL injection vulnerabilities, allowing attackers to execute arbitrary SQL queries against your database. In one case, a news website suffered a data breach due to a poorly implemented input validation system, resulting in the compromise of sensitive user information. Another incident involved an e-commerce platform that experienced a significant loss of customer data and financial losses due to an SQL injection vulnerability. ASP.NET Core's model binding and validation features, coupled with parameterized queries or ORMs, greatly reduce the risk. However, it's vital to understand the nuances of parameterization and proper use of escape characters to effectively prevent injection attacks. Integrating a web application firewall (WAF) can provide an additional layer of protection. Regular security assessments and penetration testing should identify vulnerabilities. Understanding and applying the built-in security features provided by ASP.NET Core is essential for creating secure applications. Using these mechanisms will save hours of manual coding, leading to quicker development cycles, which, in turn, translates into cost savings and efficiency.
Deployment and Monitoring: Ongoing Security Best Practices
Securing an application is an ongoing process. Deploying to a secure hosting environment and monitoring application logs are critical steps. Proper configuration of the web server, including appropriate firewall rules and SSL certificates, is also essential. Neglecting these post-development aspects exposes your application to vulnerabilities. For example, failing to update software packages can leave the application open to known exploits. In one case, a social media platform suffered a widespread breach because it failed to update its server software promptly. This resulted in a large-scale data breach and loss of user trust. Another incident involved an online retailer whose inadequate server security led to a credit card fraud incident. Integrating logging and monitoring tools allows for the detection of suspicious activity in real time, enabling rapid response. Implementing intrusion detection and prevention systems provides additional protection. Regular security audits and penetration tests are integral to identify and remediate security vulnerabilities before they can be exploited. Keeping up-to-date with the latest security patches and updates for the framework is crucial. Understanding the implications of cloud security and implementing appropriate measures depending on chosen hosting provider is also critical. Comprehensive application monitoring allows for the early detection of anomalies and potential threats, thus minimizing any damage.
Conclusion
Leveraging ASP.NET Core's built-in security features offers a substantial advantage over custom security implementations. By understanding and correctly applying these features, developers can significantly enhance the security of their applications. This approach not only improves security but also simplifies development, reduces maintenance overhead, and ensures alignment with industry best practices. The transition to a more secure development lifecycle involves the conscious adoption of these readily available security mechanisms, minimizing the risk of human error. Proactive security measures, combined with continuous monitoring and updates, are essential for protecting applications in the ever-evolving threat landscape. The focus should be on leveraging the powerful security tools already provided by the framework rather than reinventing the wheel.