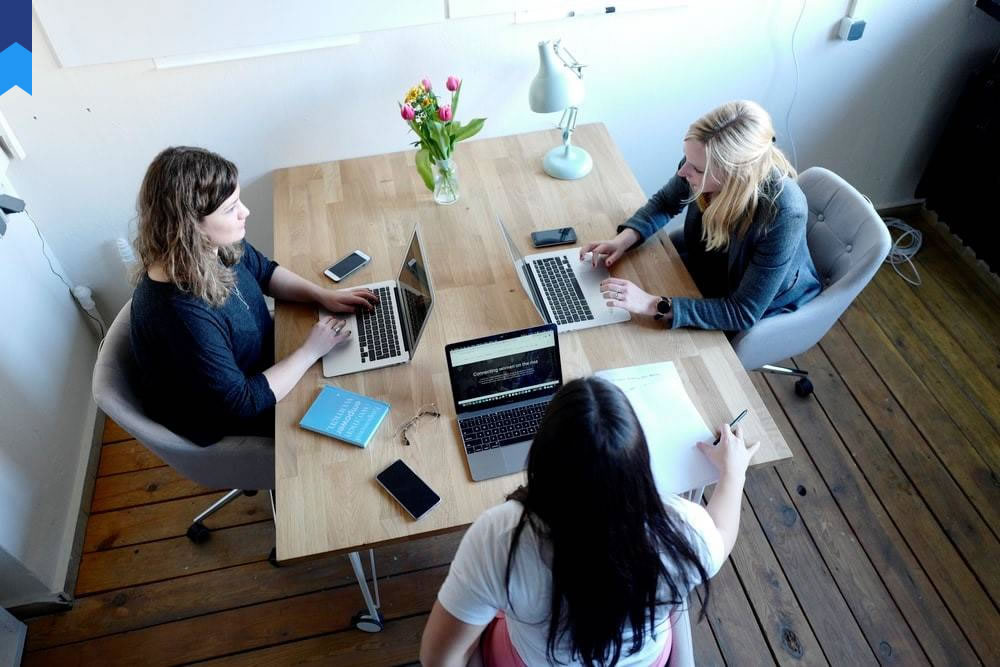
Stop Reinventing The Wheel: Mastering Reusable Code Components For Accelerated Development
Software development often feels like a constant race against time. Deadlines loom, features pile up, and the pressure to deliver quickly is immense. This relentless pace often leads developers down a path of reinventing the wheel, repeatedly writing similar code for different projects. This article explores how mastering reusable code components can dramatically accelerate development, reduce errors, and improve overall software quality. We'll delve into specific techniques, best practices, and potential pitfalls to avoid, showcasing real-world examples and case studies.
The Hidden Costs of Code Duplication
Code duplication, while seemingly harmless in the short term, introduces significant hidden costs. Maintaining duplicated code becomes a nightmare. When a bug is discovered, it needs to be fixed in every instance of the duplicated code, increasing the risk of errors and significantly extending the debugging process. This also impacts project timelines and budgets, leading to delays and cost overruns. A recent study by the Standish Group revealed that 65% of software projects go over budget, largely due to unforeseen complexities and rework stemming from duplicated code. Let's consider two case studies: Company A, which suffered a major security breach due to a missed bug fix in a duplicated authentication module; and Company B, which witnessed significant project delays due to the laborious process of correcting a simple error in 5 different parts of their codebase.
Beyond the immediate maintenance burden, duplicated code hinders scalability and maintainability. As the project grows, managing consistent updates and ensuring feature parity across different sections becomes an arduous task. This inconsistency can lead to a fractured codebase, making future development exponentially more difficult and impacting overall software performance. Imagine the complexity of managing and updating a large application with thousands of lines of duplicated code – a logistical nightmare. This leads to increased developer frustration, decreased productivity, and a higher likelihood of project failure. Consider another two case studies: Company C, which failed to scale its application due to unmaintainable duplicated code; and Company D, which experienced significant performance degradation as a result of inconsistent updates across several duplicated modules.
The cost of code duplication extends beyond the immediate project. It impacts team morale, leading to burnout and frustration amongst developers. This, in turn, translates to reduced innovation and a decline in the overall quality of the software produced. In a competitive market, this lack of efficiency can be the difference between success and failure. Let's consider two more case studies: Company E, which experienced high developer turnover due to the stress of managing duplicated code; and Company F, which lost market share due to a delay in releasing a critical feature update as a result of fixing issues in replicated code segments.
Adopting reusable components significantly reduces these costs. It allows for a streamlined development process, leading to increased productivity and reduced maintenance overhead. By centralizing code, updates can be applied in a single location, ensuring consistency and minimizing the risk of errors. By adopting a component-based approach, teams can achieve significant cost savings while improving the overall quality and scalability of their software. Examples such as React, Angular, and Vue.js show how well-designed reusable components are vital in modern web development, leading to faster development cycles and more robust applications.
Designing Effective Reusable Components
Creating effective reusable components requires careful planning and design. The key is to create modular, self-contained units with well-defined interfaces. This ensures that they can be easily integrated into various parts of the application without causing conflicts or unexpected behavior. Components should adhere to established design patterns and best practices to maintain consistency and improve readability. This improves overall code quality and reduces the likelihood of integration issues. Clear documentation is also critical, allowing other developers to understand how to use and integrate these components effectively. Lack of clear documentation often leads to confusion, increased development time, and ultimately, frustration amongst developers. Consider the case of a component designed with insufficient documentation, leading to weeks of debugging when integrated into a larger project.
The use of version control systems like Git is paramount in managing reusable components. This allows for easy tracking of changes, collaboration among developers, and the ability to revert to previous versions if necessary. Version control is an invaluable tool for managing both the development and deployment of reusable components. For example, a poorly managed version control system may result in conflicts and integration issues when multiple developers simultaneously work on the same component, leading to delays and errors. A well-managed system, however, enhances collaboration, reduces risks, and makes the process streamlined and organized.
Choosing the right programming language and framework is also vital for creating effective reusable components. Certain languages and frameworks lend themselves better to component-based development. Languages with strong modularity features, like JavaScript or Python, are ideal for building reusable components. Frameworks like React, Angular, and Vue.js provide structures and tools specifically designed for component-based development. For example, using a language lacking modularity characteristics could impede building effective components, possibly leading to code that's difficult to maintain and reuse. Properly selecting a language and framework ensures that the components are well-structured, maintainable, and easily reusable.
Testing is essential to ensure the reliability and stability of reusable components. Thorough testing, including unit tests, integration tests, and end-to-end tests, can significantly reduce the risk of bugs and improve the overall quality of the components. Failing to test reusable components adequately might introduce bugs into other parts of the application where the components are used. Therefore, testing ensures the reliability of reusable components, enhancing the software’s robustness. A comprehensive testing strategy ensures that components are free from errors, improving overall software reliability and scalability.
Implementing Reusable Components: Best Practices
Effective implementation involves careful consideration of several crucial factors. Firstly, components should be designed with a clear separation of concerns, ensuring each component handles a specific task without interfering with others. This modularity improves maintainability and scalability. This principle promotes cleaner code and allows for more focused testing. For instance, a component responsible for user authentication should be separate from a component managing user profiles. This separation makes maintenance and updates less cumbersome, minimizing risk of introducing bugs when modifying one component.
Secondly, a consistent naming convention should be enforced across all reusable components. This improves readability and reduces confusion amongst developers. A standardized naming convention enhances the understandability of the codebase, leading to better collaboration amongst developers. For example, using a consistent naming scheme (e.g., prefixing component names) allows developers to quickly identify and understand the functionality of various components, aiding in rapid development and error mitigation.
Thirdly, using dependency injection promotes loose coupling between components, making them more flexible and reusable. Loosely coupled components are easier to test and maintain. Dependency injection prevents tight coupling, improving reusability across projects and reducing the potential for errors. Consider a scenario where a component depends on another specific component without dependency injection; any changes made to the dependent component would invariably affect the dependent, making the code brittle.
Lastly, regular code reviews are crucial to ensure the quality and maintainability of reusable components. Code reviews can catch potential issues early on, preventing them from impacting the larger application. Peer reviews improve code quality, consistency, and maintainability by allowing multiple developers to review the code, spotting potential problems and suggesting improvements. A scenario where code reviews were absent might lead to accumulation of subtle bugs that could only be detected during production, leading to serious complications and unnecessary expenses. Code reviews are essential for maintaining high-quality reusable components.
Leveraging Existing Component Libraries
Taking advantage of existing component libraries significantly reduces development time and effort. Numerous open-source and commercial libraries provide pre-built components that can be readily integrated into projects. Leveraging these libraries allows developers to focus on the unique aspects of their application, rather than reinventing the wheel for common functionalities. This can drastically reduce development time and improve productivity, enabling faster time-to-market. This practice is highly efficient, considering the time and effort required to build, test, and maintain a component from scratch.
However, it's important to carefully evaluate the quality and suitability of these libraries before integration. Security vulnerabilities and compatibility issues can be significant problems if not addressed properly. Choosing a library with a strong community and active maintenance is essential. Due diligence in reviewing a library’s features, documentation, and user reviews is vital to avoid integrating an unsuitable or potentially harmful component into a project. A poorly chosen library can lead to security breaches, compatibility issues, or even introduce unexpected bugs and performance issues.
Thorough testing is crucial when using external components. Integrating a component without sufficient testing can introduce unexpected bugs or security vulnerabilities. Testing should cover both the functionality and security of the component to ensure it integrates seamlessly with the application. This mitigates risks and maintains the integrity of the software. A detailed testing strategy will ensure compatibility and performance, and will minimize the risks associated with using components from external sources.
Proper licensing and attribution must be carefully considered when using external components. Understanding the licensing terms prevents legal issues and ensures compliance. This crucial step safeguards against potential legal ramifications and ensures ethical practices. Failure to adhere to licensing requirements could lead to legal issues and reputational damage for the organization.
Future Trends and Implications
The future of software development increasingly relies on the effective use of reusable components. The rise of microservices architectures emphasizes the importance of well-defined, independent components that can be easily deployed and scaled. Microservices-based architecture is becoming increasingly prevalent, with many organizations opting to adopt this approach for its ability to allow for independent scaling and deployment of individual components. This approach leverages reusable components to a significant extent.
The increasing popularity of low-code/no-code platforms relies heavily on reusable components to provide developers with pre-built functionalities that can be easily assembled to create applications. These platforms, while sometimes limited in scope, allow for rapid application development and deployment, making them popular choices for many businesses. Many such platforms depend entirely on well-designed and well-documented reusable components for their efficacy.
Artificial Intelligence (AI) and Machine Learning (ML) are poised to further revolutionize the creation and management of reusable components. AI-powered tools can assist in automating the process of creating, testing, and deploying components, potentially leading to significant efficiency gains. AI can facilitate automatic code generation, bug detection, and even component recommendation based on project needs. This could drastically reduce development time and improve component quality.
The adoption of reusable components is no longer a matter of choice but a necessity for building scalable, maintainable, and cost-effective software. Mastering this crucial aspect of software development is essential for success in today's dynamic and competitive software landscape. As software systems become increasingly complex, the ability to effectively leverage reusable components becomes crucial for building resilient and adaptable systems. The future of software development is likely to be further defined by the extent to which organizations master the effective use of reusable code components.
Conclusion
Mastering reusable code components is not just a best practice; it's a necessity for modern software development. By understanding the hidden costs of code duplication, designing effective components, implementing best practices, and leveraging existing libraries, development teams can significantly accelerate their workflows, improve software quality, and reduce long-term maintenance burdens. The future of software development hinges on the efficient and effective use of reusable components, promising faster development cycles, increased scalability, and enhanced overall software quality. Embracing this paradigm shift is crucial for any organization looking to thrive in the ever-evolving landscape of software engineering. The adoption of robust, reusable components will continue to drive innovation and efficiency in the years to come.