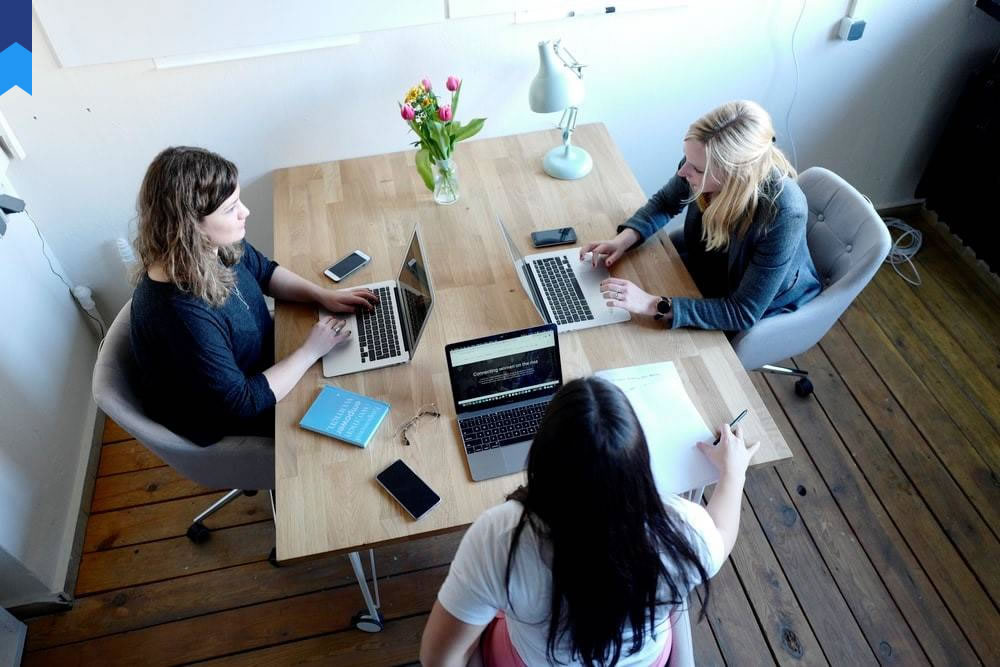
Strategic Approaches To Algorithmic Mastery: Unconventional Paths To Programming Prowess
Programming is more than just syntax; it's a craft honed through strategic thinking. This article delves into unconventional yet highly effective approaches to mastering the art of algorithmic problem-solving, moving beyond basic tutorials to reveal advanced techniques that propel programmers toward true expertise.
Unveiling the Power of Pattern Recognition
Experienced programmers don't just write code; they recognize patterns. Identifying recurring structures in problems is a key to elegant and efficient solutions. Instead of tackling each problem from scratch, seasoned programmers leverage their knowledge of established algorithms and data structures, adapting them to the specific requirements. This approach drastically reduces development time and improves code quality. For example, recognizing a problem as a variation of the knapsack problem allows one to directly apply known dynamic programming solutions, saving significant effort. Consider the case of Netflix's recommendation system. It leverages sophisticated pattern recognition algorithms to analyze user viewing history and predict future preferences, ultimately leading to personalized recommendations. This sophisticated pattern recognition is a cornerstone of their success, and it’s the kind of algorithmic mastery that this article explores.
Another illustrative example is found in fraud detection systems used by financial institutions. These systems identify fraudulent transactions by recognizing patterns in large datasets. By employing machine learning techniques to analyze transactional data, banks can effectively flag suspicious activities, minimizing financial losses. The efficacy of these systems hinges on identifying subtle but crucial patterns indicative of fraudulent behavior, demonstrating the power of pattern recognition in real-world applications. A deeper dive into algorithm design reveals the importance of using appropriate data structures; choosing the wrong one can significantly impact performance. For instance, using a linked list for frequent search operations, rather than a hash table, can lead to significantly slower execution times. Mastering the nuances of data structures is crucial for optimizing algorithms and writing efficient, scalable code. A well-designed algorithm, coupled with an appropriate data structure, can transform a complex problem into a manageable one. This is not just about theoretical understanding; it’s about practical application and a profound understanding of the computational landscape.
The ability to spot recurring themes isn't just a skill; it's a habit cultivated through consistent practice and exposure to diverse programming challenges. By continually challenging oneself with intricate problems and reflecting on the solutions, programmers gradually sharpen their pattern recognition abilities. This iterative process, akin to a craftsman refining their skills, is what transforms a novice coder into a seasoned expert. Consider the case of Google's search algorithm, which is constantly being refined to better recognize patterns in user queries and deliver more relevant search results. The constant adaptation and improvement of such systems is a testament to the ongoing process of pattern recognition and refinement inherent in algorithmic mastery. A crucial aspect is the ability to decompose complex problems into smaller, manageable subproblems, which are then individually solved before integrating them into a comprehensive solution. This approach, often called divide and conquer, is fundamental to efficient algorithmic design and problem-solving. It’s akin to building a complex machine from a series of interconnected, simpler components.
Furthermore, efficient algorithm design often involves employing mathematical and logical reasoning to create optimal solutions. This often requires a deep understanding of discrete mathematics, graph theory, and other mathematical concepts that underpin computational complexity analysis. Mastering these areas is crucial for optimizing algorithms and achieving scalability. For instance, understanding the properties of different graph traversal algorithms, such as breadth-first search and depth-first search, enables the development of efficient algorithms for navigation systems, network analysis, and other applications. The application of this mathematical reasoning is often subtle, but its impact on performance is profound, highlighting the importance of a solid mathematical foundation in software engineering. Many advanced algorithms are based on complex mathematical principles, and a strong grasp of these principles is essential for understanding, adapting, and improving them. Finally, understanding time and space complexity analysis is paramount in designing efficient algorithms. Being able to analyze the resource consumption of an algorithm allows programmers to make informed decisions about algorithmic choices, optimize performance, and avoid bottlenecks. Tools like Big O notation provide a powerful framework for assessing algorithmic efficiency. Through rigorous testing and optimization, the algorithmic process can yield significant performance gains and create scalable systems.
The Art of Abstraction and Modular Design
Abstraction is the backbone of elegant code. Instead of grappling with minute details, programmers create higher-level representations that capture the essence of the problem. This promotes code readability, maintainability, and reusability. For instance, instead of writing the code for network communication directly, one can utilize an abstraction layer, such as a networking library, that handles low-level details. This abstraction shields the application from the complexities of network protocols. Consider the case of operating systems, which abstract the underlying hardware from applications, allowing developers to focus on functionality rather than low-level hardware management. Without abstraction, software development would be infinitely more complex and less efficient.
Modular design complements abstraction by breaking down complex programs into smaller, independent modules. Each module encapsulates a specific functionality, facilitating code organization, debugging, and testing. This approach is crucial for larger projects where multiple developers collaborate simultaneously. A well-defined modular design allows for parallel development and simplifies integration, reducing development time and enhancing collaboration. The construction of modern software, especially large-scale applications, heavily relies on modular design principles, ensuring robustness and maintainability. Consider large-scale enterprise resource planning (ERP) systems which are comprised of numerous interconnected modules, each responsible for a specific business function. The modular nature of such systems allows for flexible adaptation to changing business needs and easy integration of new features.
Furthermore, the concept of encapsulation, a key principle of object-oriented programming, directly relates to modularity. It involves bundling data and the methods that operate on that data within a single unit, restricting direct access to the internal state of the object. This shields the internal workings of a module from external interference, enhancing security and preventing unintended side effects. A common example of this principle is seen in database systems, where database tables encapsulate data and the methods for accessing and modifying that data. This protects the integrity of the data and ensures that changes are made in a controlled manner. The proper implementation of encapsulation results in a secure and robust system. Effective implementation requires careful consideration of access modifiers (public, private, protected) to control visibility and prevent unintended manipulation of internal data. It is critical for protecting data integrity and facilitating secure and predictable operation.
The use of design patterns, pre-defined solutions to recurring design problems, further enhances modularity and code reusability. These patterns provide established blueprints for common programming tasks, eliminating the need to reinvent the wheel. Many design patterns promote modularity through well-defined interfaces and interactions between components. For example, the Model-View-Controller (MVC) pattern separates the application's data (model), user interface (view), and logic (controller) into distinct modules, promoting flexibility and maintainability. The adoption of design patterns is essential in large-scale projects, enabling streamlined development, better collaboration, and the creation of robust, maintainable applications.
Mastering Data Structures and Algorithms
Proficient programmers possess an intimate understanding of data structures. Choosing the right data structure significantly impacts an algorithm's performance. Arrays are efficient for accessing elements by index, but linked lists excel when frequent insertions and deletions are needed. Hash tables are indispensable for fast lookups, while trees are perfect for hierarchical data organization. The selection of the optimal data structure is critical to the efficiency of the entire algorithm. This isn't just a theoretical understanding; it's about practical application and a deep appreciation of the computational trade-offs involved. Consider the development of a social networking application: the efficient retrieval and management of user connections are crucial; a well-designed data structure, such as a graph, becomes essential for managing user relationships efficiently.
Algorithm design is equally important. Mastering various algorithmic paradigms, such as divide and conquer, dynamic programming, greedy algorithms, and backtracking, empowers programmers to tackle diverse problems. Understanding the strengths and weaknesses of each paradigm allows for informed decision-making. This understanding extends beyond simply memorizing algorithms; it involves comprehending their underlying principles and applying them creatively. For instance, the application of dynamic programming is crucial in solving optimization problems where optimal substructure exists, a characteristic that is not immediately obvious in many cases. Recognizing this characteristic and selecting the appropriate algorithmic approach is a key skill for efficient coding. Consider the task of finding the shortest path between two nodes in a graph; Dijkstra's algorithm, a classic graph traversal algorithm, provides an efficient solution. Mastering such algorithms is essential for solving real-world problems effectively.
The analysis of algorithmic efficiency is paramount. Big O notation provides a powerful framework for assessing the scalability and performance of algorithms. Understanding time and space complexity helps programmers make informed decisions about algorithm selection and optimization. This is critical for building scalable and performant applications, especially when dealing with large datasets. Consider the case of a search engine where efficient retrieval of information from a vast database is paramount. The choice of algorithms directly influences the speed and responsiveness of the search results. An efficient algorithm, properly chosen and implemented, can make the difference between a responsive system and a sluggish one.
Moreover, the ability to optimize code for specific hardware architectures and software environments is crucial. This involves understanding cache mechanisms, memory management, and other low-level details that impact performance. Modern programming languages often offer tools for profiling and performance analysis, assisting programmers in identifying bottlenecks and optimizing their code. A well-optimized code can dramatically improve execution speed and reduce resource consumption. This is especially crucial in resource-constrained environments such as embedded systems or mobile applications. Furthermore, it's not enough to write efficient code; the code must also be correct. Thorough testing and debugging are essential for ensuring the reliability and accuracy of software applications. Techniques such as unit testing, integration testing, and system testing can help identify and fix errors, preventing unexpected behavior and improving the overall robustness of the application.
The Significance of Testing and Debugging
Thorough testing is not an afterthought; it's an integral part of the development process. Unit testing, integration testing, and system testing are crucial for identifying bugs early and ensuring code quality. Unit tests verify individual modules, integration tests check interactions between modules, and system tests evaluate the complete system. A robust testing strategy significantly reduces the risk of deploying faulty software. Consider the development of safety-critical systems, such as medical devices or aircraft control software, where thorough testing is essential to prevent catastrophic failures. The cost of inadequate testing in such critical systems can be immense, emphasizing the importance of a well-defined testing strategy.
Debugging is the art of identifying and fixing errors in code. Effective debugging techniques, such as using debuggers, logging, and print statements, help programmers track down the root cause of problems. A deep understanding of the program's logic is crucial for effective debugging. Modern IDEs provide powerful debugging tools, such as breakpoints and step-through execution, making the process more efficient. Consider the example of a large-scale e-commerce platform, where a bug in the payment processing system could result in significant financial losses. The prompt identification and resolution of bugs in such a system are crucial to maintain the operational integrity of the platform.
Furthermore, effective debugging often involves a combination of systematic approaches and creative problem-solving. Tracing execution flow, analyzing log files, and reproducing errors systematically are essential steps. However, sometimes it also requires intuition and experience to identify the root cause of a complex issue. Modern debugging tools provide extensive visualization capabilities, allowing developers to inspect the state of variables, memory, and other system resources, which helps in identifying subtle issues that may not be evident otherwise. Consider the case of complex data structures and algorithms, where debugging can require a deep understanding of the underlying logic and the ability to trace the flow of data through the system. A methodical and analytical approach is essential for effective debugging.
Moreover, the adoption of code review practices further enhances code quality and reduces the likelihood of errors. Peer review helps identify potential issues before they reach production, improving code maintainability and reducing technical debt. Code review also offers a valuable learning opportunity for developers, exposing them to various coding styles and best practices. Consider the development of large-scale software projects where multiple developers collaborate simultaneously. Code review is essential in ensuring consistency, maintainability, and avoiding conflicting implementations. This process significantly increases the overall quality of the code and makes the code easier to maintain and debug in the long run.
Collaboration and Community Engagement
Effective collaboration is paramount in software development. Working effectively in teams requires clear communication, well-defined roles, and a shared understanding of goals. Version control systems, such as Git, are indispensable for managing code changes and facilitating collaboration. The use of collaborative coding platforms and tools further streamlines the development process. Effective collaboration is not just about writing code together; it's about fostering a supportive and inclusive environment where team members can learn from each other and share expertise. Consider the development of large-scale software projects, where teams of developers work together across geographical locations. Effective communication and collaboration are critical to the success of such projects.
Engaging with the broader programming community provides invaluable opportunities for learning and growth. Online forums, communities, and open-source projects offer platforms for sharing knowledge, seeking assistance, and contributing to collaborative efforts. Participating in such communities enhances one's skills and understanding, exposing individuals to diverse perspectives and best practices. Consider the numerous open-source projects that rely on contributions from a global community of developers. Such projects demonstrate the power of community engagement in driving software innovation and collaboration.
Furthermore, actively participating in online forums and knowledge-sharing platforms helps connect with experienced programmers, learn from their expertise, and gain insights into various aspects of software development. Sharing one's knowledge and experiences also contributes to the growth of the community and fosters a sense of collective learning. Consider the various online forums and communities dedicated to specific programming languages or technologies. These platforms provide valuable resources and opportunities for learning and collaboration. Moreover, participation in hackathons and coding competitions can enhance one's skills and provide valuable experience working on challenging projects within a time-constrained environment. These events often bring together talented individuals from various backgrounds, facilitating collaborative problem-solving and idea generation.
Finally, continuous learning is crucial for staying current in a rapidly evolving field. Exploring new technologies, attending workshops, and pursuing advanced education are essential for maintaining a competitive edge. This constant learning process keeps one informed about the latest trends, tools, and techniques, ensuring relevance and adaptability in the field. Consider the continuous evolution of programming languages, frameworks, and tools. Staying up-to-date is paramount for software developers to remain proficient and relevant in the industry. This ongoing learning journey is crucial for sustained professional growth and continued success in the ever-changing world of software development.
Conclusion
Mastering algorithmic problem-solving is a journey of continuous learning and strategic refinement. This article has explored unconventional yet highly effective approaches, emphasizing the significance of pattern recognition, abstraction, data structures, rigorous testing, and collaborative engagement. By integrating these strategic approaches into one's development process, programmers can elevate their skills, enhance code quality, and achieve true algorithmic mastery. The path to algorithmic prowess is not a linear progression; it's an iterative journey of experimentation, learning, and adaptation. Embracing these principles will not only improve one's coding skills but also foster a deeper understanding and appreciation for the elegant power of algorithms.
The ability to design efficient and scalable algorithms is crucial for the development of modern software systems, impacting areas ranging from artificial intelligence and machine learning to embedded systems and data science. Through consistent practice and a commitment to continuous learning, programmers can unlock their potential and achieve remarkable results. The journey towards algorithmic mastery is a continuous process of growth and refinement, requiring dedication, persistence, and a passion for solving complex problems. By embracing these principles, programmers can not only enhance their technical skills but also cultivate a deeper understanding and appreciation for the elegance and power of algorithms. The future of software development hinges on the ability to create efficient and innovative solutions, and the mastery of algorithmic principles is the key to unlocking this potential.