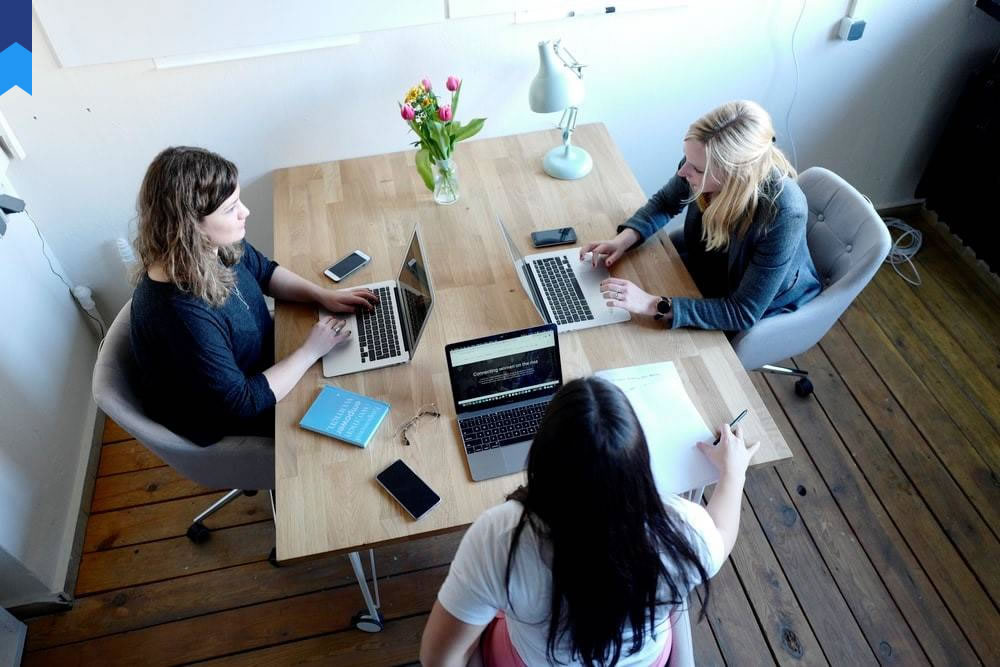
Strategic Approaches To Compiler Optimization
Introduction
Compiler optimization is a critical aspect of software development, significantly impacting performance, energy efficiency, and code size. This article delves into strategic approaches to compiler optimization, moving beyond basic overviews to explore sophisticated techniques and innovative strategies. We will examine various optimization methods, considering their strengths, weaknesses, and practical applications. The goal is to equip developers and compiler designers with a deeper understanding of how to achieve optimal code generation and execution.
Loop Optimization Techniques
Loop optimization is paramount for performance enhancement. Loop unrolling replicates loop bodies, reducing loop overhead. Consider a simple loop adding numbers: for(int i=0; i<100; i++) sum += i;
Unrolling could yield faster execution. Loop fusion combines adjacent loops accessing the same data structures, minimizing memory accesses. For instance, two loops iterating through an array for addition and multiplication can be fused into one. Loop invariant code motion moves calculations outside loops if their results are constant within the loop. If a calculation like x*y
is within a loop and x and y are constant, it is moved outside. Strength reduction replaces expensive operations with cheaper ones. For example, calculating x*2
is faster than x<<1
. Case study: The LLVM compiler employs sophisticated loop optimization techniques, significantly improving the performance of C++ applications. Another case study: The GCC compiler's loop optimization strategies have proven effective in numerous open-source projects. Advanced techniques include software pipelining and loop tiling, which enhance performance through more intricate manipulation of loop iterations and data access patterns. The effective use of these techniques can significantly impact overall system performance.
Data Flow Analysis and Optimization
Data flow analysis is the bedrock of many compiler optimizations. Reaching definitions analysis determines where a variable's value is defined and used. Live variable analysis identifies variables whose values are used after their definitions. These analyses are critical for various optimizations. Dead code elimination removes code that doesn't affect the program's output. Constant propagation replaces variable uses with their constant values. Copy propagation replaces variable assignments with their copied values. Constant folding evaluates constant expressions during compilation. Case study: The Java Virtual Machine (JVM) uses extensive data flow analysis for just-in-time compilation. Case study: Modern C++ compilers rely on sophisticated data flow analysis to perform advanced optimizations like inlining and function specialization. These techniques drastically impact performance, reducing runtime and resource consumption. Accurate and efficient data flow analysis is critical for developing high-performance compilers. Effective implementation of these techniques require careful handling of complex control flow structures and data dependencies.
Register Allocation and Scheduling
Efficient register allocation is crucial for maximizing performance. Register allocation assigns variables to CPU registers, minimizing memory accesses. Graph coloring algorithms are often used to solve this problem. Register spilling moves variables to memory when registers are exhausted. Register scheduling reorders instructions to improve performance, reducing pipeline stalls and instruction dependencies. List scheduling, priority-based scheduling, and other sophisticated algorithms are employed to address various scheduling challenges. Case study: The performance of embedded systems compilers relies heavily on intelligent register allocation. Case study: High-performance computing (HPC) compilers use advanced register scheduling techniques to optimize parallel code execution. Compiler optimization techniques significantly influence overall system efficiency. Careful consideration of hardware limitations and architectural nuances are key to maximizing performance gains. Register allocation and scheduling are tightly intertwined, necessitating sophisticated algorithms for efficient implementation.
Interprocedural Optimization
Interprocedural optimization involves optimizing across multiple functions. Inlining replaces function calls with the function body, reducing overhead. Function specialization creates specialized versions of functions for specific argument types or call sites. Cross-function dead code elimination removes unused code across multiple functions. Case study: Modern C++ compilers utilize interprocedural optimization for significant performance improvements. Case study: Many Java compilers perform interprocedural analysis to optimize the code within large applications. Interprocedural analysis can reveal optimization opportunities often missed by intraprocedural analysis. This level of optimization is more complex, but the potential gains in performance are substantial, particularly in complex applications. Proper handling of function pointers and indirect calls adds substantial complexity to the implementation of this optimization strategy.
Advanced Optimization Techniques
Beyond the basics, advanced techniques offer further performance gains. Auto-vectorization transforms loops to utilize vector instructions, leveraging SIMD capabilities. Automatic parallelization transforms sequential code into parallel code, exploiting multi-core processors. Link-time optimization (LTO) performs optimization across multiple object files during linking. Profile-guided optimization (PGO) uses profiling data to guide optimization decisions, enhancing performance for specific workloads. Case study: Modern compilers employ auto-vectorization to optimize numerical computations. Case study: Large-scale scientific applications benefit significantly from automatic parallelization. These sophisticated techniques demand detailed understanding of the target architecture and program behavior. The effective use of advanced techniques necessitates thorough profiling and careful consideration of trade-offs between optimization complexity and performance gains. The continuous evolution of hardware architectures requires ongoing research and development in this field.
Conclusion
Compiler optimization is a multifaceted field, with numerous techniques offering substantial performance improvements. The strategies discussed – loop optimization, data flow analysis, register allocation and scheduling, interprocedural optimization, and advanced techniques – are crucial for creating efficient and high-performing software. Understanding these techniques and their interplay is essential for both compiler designers and software developers seeking to maximize the performance of their applications. The constant evolution of hardware and software necessitates ongoing research and development of even more sophisticated optimization strategies in the future. Effective optimization necessitates a thorough understanding of both the compiler and the target hardware architecture.