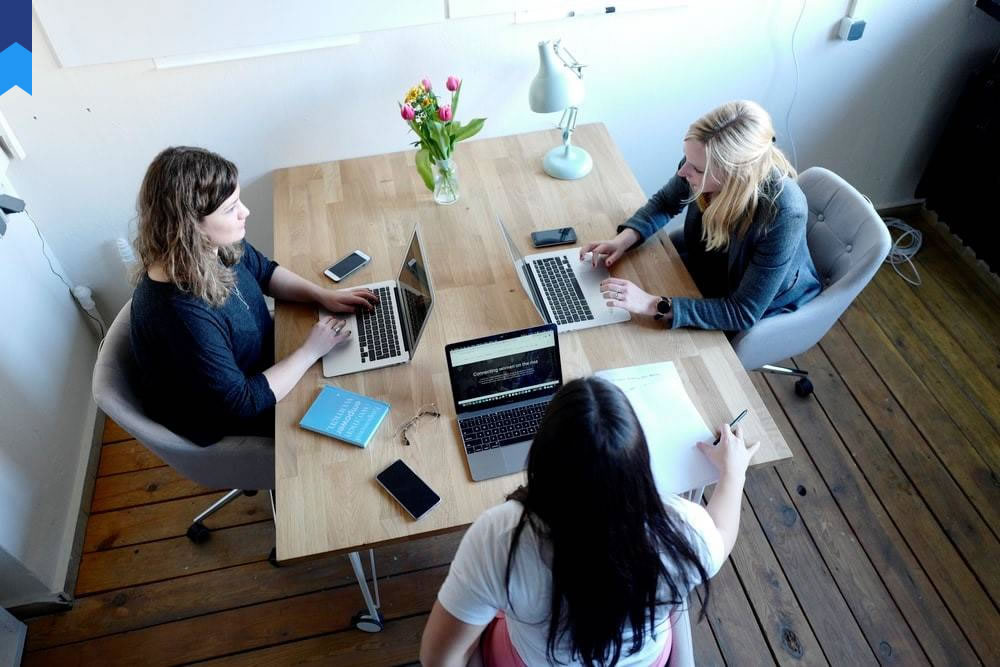
The Counterintuitive Guide To Mastering Data Structures & Algorithms
Introduction
Data structures and algorithms are the cornerstones of efficient programming. However, the path to mastery often feels counterintuitive, filled with unexpected twists and turns. This guide challenges conventional wisdom, revealing unconventional approaches and surprising insights into optimizing your code. We will explore practical applications, innovative techniques, and real-world case studies to illuminate the often-hidden depths of this crucial field. Prepare to rethink your approach and unlock a new level of proficiency.
Unlocking the Power of Recursion: Beyond the Basics
Recursion, often feared by novice programmers, is a powerful tool that can elegantly solve complex problems. The counterintuitive aspect lies in its seemingly circular nature—a function calling itself. However, understanding the base case and the recursive step is key. For example, consider the classic factorial calculation. A straightforward iterative approach is possible, but a recursive solution is often more concise and easier to understand. This approach directly mirrors the mathematical definition of a factorial. Case study one: Analyzing a directory tree. A recursive function easily traverses a directory structure, listing all files and subdirectories. Case study two: Implementing a quicksort algorithm. Quicksort's elegant efficiency is rooted in its recursive partitioning strategy. However, poorly implemented recursion can lead to stack overflow errors—a counterintuitive consequence of attempting to improve efficiency. Properly handling the base case and recursive step prevents this. Choosing the appropriate pivot element is critical to maintaining efficiency, highlighting another counterintuitive aspect— seemingly minor design choices impacting overall performance.
Mastering recursion requires understanding its limitations. Deep recursion can lead to significant performance overhead due to function call overhead and stack space consumption. In such cases, an iterative approach might be more efficient. However, for problems that naturally lend themselves to recursive solutions, it's an indispensable tool. This highlights the counterintuitive nature of optimization: the "best" solution is context-dependent. It’s not always about choosing the fastest algorithm, but the algorithm best suited to the task and available resources. Efficient memory management techniques, such as tail recursion optimization (where the compiler can transform the recursive call into an iterative loop), also play a vital role. It's important to balance elegance with practicality.
Furthermore, understanding when recursion is beneficial requires careful consideration of the problem. Problems with inherent self-similarity, such as tree traversal or fractal generation, often have elegant recursive solutions. However, problems better suited to iterative approaches should not be forced into a recursive mold. This nuanced understanding is crucial for effective algorithm design. Recursion, while seemingly complex, can be a powerful tool when wielded thoughtfully and appropriately. The seeming complexity is an illusion, as many algorithms become beautifully clear with recursion.
The counterintuitive nature of algorithm design highlights the importance of careful consideration of the problem space and available resources. Recursive functions, despite their initial complexity, elegantly solve problems that are difficult to solve iteratively. Understanding these counterintuitive aspects is key to mastery.
Dynamic Programming: The Art of Memoization
Dynamic programming often feels counterintuitive. Instead of directly solving a problem, we solve many smaller overlapping subproblems and store their solutions. This memoization, the act of storing previously computed results, is what makes dynamic programming so effective. A classic example is the Fibonacci sequence. A naive recursive implementation is exponentially slow, while a dynamic programming approach with memoization achieves linear time complexity. This demonstrates the power of trading space for time. Case study one: The knapsack problem. This classic optimization problem, involving maximizing the value of items within a weight constraint, benefits enormously from a dynamic programming approach. The optimal solution is built up from solutions to smaller subproblems. Case study two: Sequence alignment in bioinformatics. The Needleman-Wunsch algorithm uses dynamic programming to efficiently align biological sequences, a task crucial in genomics research. The algorithm's efficiency hinges on the memoization of alignment scores between sub-sequences.
The counterintuitive nature of dynamic programming arises from its seemingly inefficient approach. By solving multiple subproblems, it appears wasteful. However, this apparent inefficiency is offset by the avoidance of redundant computations. The trade-off between space and time efficiency is central to the approach. When solving large problems, the time saved by memoization far outweighs the space used to store intermediate results. Understanding this trade-off is crucial. The efficiency gains are dramatic, transforming exponentially complex problems into polynomial time solutions. It’s a counterintuitive optimization technique that rewards a seemingly inefficient initial strategy.
Beyond simple memoization, dynamic programming incorporates clever techniques like tabulation (building solutions bottom-up) and top-down approaches. These strategies, while different in implementation, all stem from the same core principle: breaking a complex problem into simpler, overlapping subproblems and storing their solutions. The choice between tabulation and top-down approaches is often dependent on problem characteristics and programming language features. For instance, certain languages excel at recursive implementations while others are more efficient with iterative ones. This reflects another layer of counterintuitive complexity, showcasing that optimal coding style depends on the interplay between algorithm and implementation.
Advanced dynamic programming techniques involve optimizing memoization strategies. Techniques such as using hash tables instead of arrays can significantly impact performance. Further optimizations, such as advanced memory management techniques, might be required depending on the scale of the problem and complexity of the subproblems. The space-time trade-off is a constant consideration, and choosing the optimal balance depends on the specific requirements and constraints of the problem. The seemingly complex approach of dynamic programming often yields the most efficient and elegant solutions.
Graph Algorithms: Navigating Complexity
Graphs, structures representing relationships between entities, are ubiquitous in computer science. Algorithms operating on graphs often involve counterintuitive approaches. The shortest path problem, for example, seemingly simple, requires algorithms like Dijkstra's algorithm, a sophisticated approach involving priority queues. This highlights the counterintuitive reality that simple problems can demand complex solutions. Case study one: Network routing. Protocols like OSPF (Open Shortest Path First) rely on graph algorithms like Dijkstra's to find the optimal paths for data packets across a network. This highlights the practical importance of these algorithms in large-scale systems. Case study two: Social network analysis. Analyzing connections in social networks involves graph algorithms, helping to understand community structures and influence propagation. Understanding the structure of these networks is crucial for various applications.
The counterintuitive aspect of graph algorithms lies in the often-unexpected complexity. Simple questions like "what is the shortest path?" can lead to surprisingly sophisticated algorithms. The choice of the correct algorithm is paramount, influenced by factors like the graph's structure (directed, undirected, weighted), the type of shortest path being sought (single-source, all-pairs), and computational constraints. These considerations might lead to the selection of seemingly complex algorithms over simpler ones. These unexpected algorithm complexities directly contradict the initial problem's seeming simplicity.
Beyond shortest paths, graph algorithms deal with various problems, such as minimum spanning trees (Prim's and Kruskal's algorithms) and topological sorting (finding a linear ordering of nodes in a directed acyclic graph). Each of these problems has its own set of efficient algorithms, often counterintuitive in their approach. Understanding these algorithms demands a deep grasp of graph theory and algorithmic design. Furthermore, optimizing these algorithms for specific graph structures is crucial. For instance, algorithms designed for sparse graphs might perform poorly on dense graphs, demonstrating again the importance of carefully considering the characteristics of the input data. These intricate aspects highlight the counterintuitive nature of the optimization process.
The practical applications of graph algorithms are vast, ranging from GPS navigation (finding optimal routes) to recommendation systems (finding users with similar preferences) and network security (detecting vulnerabilities). The efficiency of these algorithms is not just a theoretical concern but directly impacts the performance and scalability of real-world systems. Further exploration often unveils counterintuitive optimization strategies that enhance performance based on the structure of the specific graph. This underlines the need to go beyond basic understanding and delve into the advanced techniques that make these algorithms truly effective. The seeming simplicity of graph theory masks significant challenges requiring advanced algorithms.
Greedy Algorithms: The Power of Local Optimization
Greedy algorithms, which make locally optimal choices at each step, often defy intuition. They might not always yield the globally optimal solution but often provide surprisingly good approximations with significantly reduced computational cost. This local-to-global optimization trade-off is the counterintuitive core of greedy algorithms. Case study one: Huffman coding. This data compression technique uses a greedy approach to build an optimal prefix-free binary code. The algorithm's efficiency is in its simplicity and local optimization strategy. Case study two: Scheduling problems. Many scheduling problems can be approached with greedy algorithms, where tasks are assigned in order of priority or deadline, leading to efficient though not always optimal schedules. The simplicity of these algorithms is a major benefit in time-constrained situations.
The counterintuitive effectiveness of greedy algorithms arises from their simplicity. Instead of exploring all possible solutions, a greedy algorithm makes a locally optimal choice at each step, dramatically reducing computation time. While this approach does not guarantee a globally optimal solution, it often provides a solution that is "good enough" for many practical purposes. This highlights the tension between optimality and efficiency. It’s a common trade-off in algorithm design: a slightly suboptimal solution might be significantly more efficient to compute.
The choice of which greedy strategy to use depends heavily on the problem being solved. Different greedy approaches exist, each making locally optimal choices based on different criteria. This selection process is often more nuanced than it first appears and requires careful consideration of the problem's characteristics. Understanding these subtle differences is key to applying greedy algorithms effectively. Furthermore, it's crucial to analyze the performance of greedy algorithms, comparing their solutions to globally optimal solutions (if known) to assess their effectiveness in the context of a specific problem.
The analysis of greedy algorithms often involves proving that their solutions are within a certain approximation factor of the optimal solution. This demonstrates their theoretical robustness, even when they don't achieve perfect optimality. This theoretical understanding is critical for practical applications, where a guarantee on solution quality (even if not perfectly optimal) is often more valuable than the potential computational gains of a more complex algorithm that might not deliver. The counterintuitive simplicity of these approaches makes them attractive tools for solving many practical optimization problems efficiently.
Conclusion
Mastering data structures and algorithms requires a willingness to embrace the counterintuitive. This journey involves challenging assumptions, exploring unconventional approaches, and understanding the intricate trade-offs involved in algorithm design. While basic overviews provide a foundation, true mastery lies in grappling with the unexpected complexities and nuances of these core concepts. This guide has attempted to shed light on some of these counterintuitive aspects, highlighting how unconventional approaches can lead to elegant and efficient solutions. The path to mastery is not linear; it involves continuous learning, experimentation, and a willingness to challenge conventional wisdom.
The counterintuitive nature of optimization often involves exploring seemingly inefficient strategies that unexpectedly yield significant improvements. Understanding these trade-offs is crucial for becoming a proficient programmer. The choice of algorithm often depends on subtle factors relating to the input data and available resources. The ultimate goal is not just to find a solution, but to find the best solution given the constraints. By embracing these counterintuitive aspects, you can unlock a deeper understanding of data structures and algorithms and become a more effective programmer.