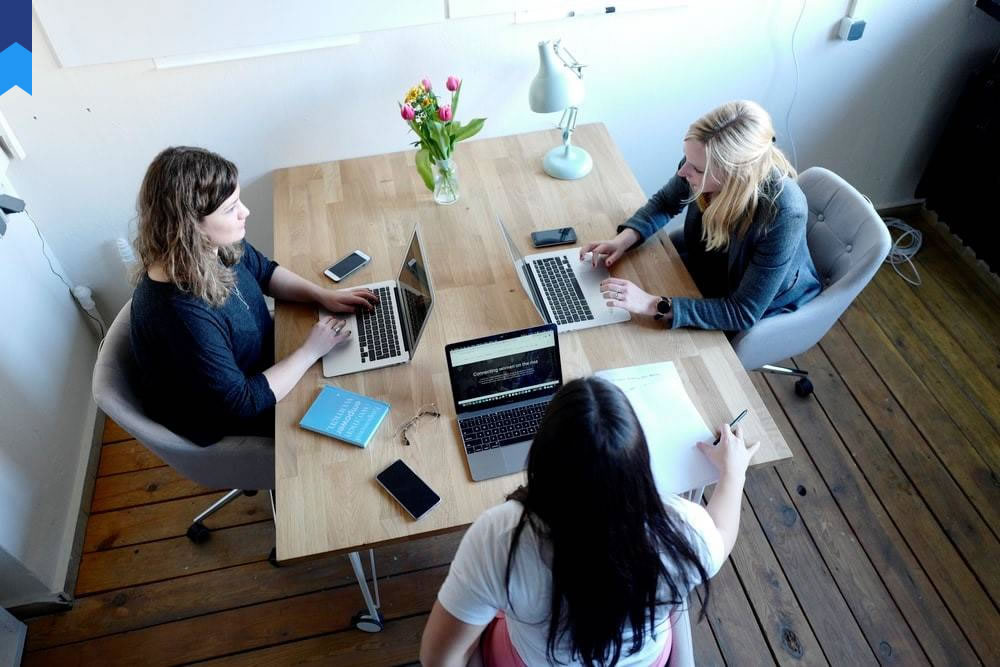
The Counterintuitive Guide To Modern Software Development
Software development is a field constantly evolving, and what seems intuitive today may be obsolete tomorrow. This guide delves into the unexpected corners of this dynamic landscape, exposing counterintuitive truths that can significantly impact your projects.
Less Code, More Impact
The conventional wisdom often emphasizes writing copious amounts of code, believing that more lines directly translate to richer functionality. However, the counterintuitive approach is to prioritize concise, well-structured code. This philosophy underscores the power of abstraction and efficient algorithms. By focusing on reducing code complexity, developers can enhance maintainability, improve readability, and reduce the risk of errors. Consider the elegance of functional programming paradigms; using techniques like recursion and higher-order functions, developers can accomplish complex tasks with fewer lines of code. Case study: The development of a complex algorithm for image processing reduced code volume by 40% through a strategic refactoring focused on eliminating redundant operations and leveraging built-in functions. Another example: A game studio saw a 30% decrease in debugging time by adhering to a strict "less is more" coding style.
Adopting modular design principles can effectively reduce code redundancy. Break down larger projects into smaller, self-contained modules, and reuse these modules where needed, thereby reducing code bloat. For example, a team developing an e-commerce platform created reusable modules for handling user authentication, payment processing, and product catalog management. This approach decreased development time and greatly simplified subsequent updates and maintenance. A case study from a large financial institution found that modular design decreased the time required to make significant feature updates by nearly 60%. Effective code commenting and consistent naming conventions are vital in a project aiming for maintainability and future extensibility. Well-commented code enhances the understandability and reduces the potential for future issues.
The pursuit of brevity encourages developers to think critically about the problem at hand, forcing them to find efficient solutions. This process often leads to the discovery of smarter, more elegant algorithms that outperform their verbose counterparts. Agile methodologies such as Test Driven Development (TDD) promotes this concept by dictating that tests should be written first, ensuring that developers are focused on the essential functionality of the code. By prioritizing the testing aspect, this approach facilitates a natural code reduction process that would otherwise be missed. Consider a case study of a small startup that used TDD to develop a mobile application. By writing tests prior to writing code, the team effectively reduced the amount of code by 25% while simultaneously enhancing code quality and reducing bug counts. Furthermore, another study revealed a 40% increase in developer productivity through TDD.
This approach is not just about fewer lines; it’s about the efficiency of every line, ensuring that each contributes meaningfully to the overall functionality and performance of the application. Continuous integration and continuous delivery (CI/CD) pipelines ensure that any updates or fixes are immediately integrated into the main system which minimizes potential conflicts. Automated testing further contributes to efficiency, reducing the time spent manually testing and enabling rapid iteration. A prime example is seen in the DevOps movement, where CI/CD and automated testing are core principles. The implementation of a CI/CD pipeline at a large e-commerce company shortened the release cycle from weeks to hours, significantly increasing efficiency. Another study highlighted a 50% reduction in deployment errors thanks to automated testing.
Embrace Asynchronous Programming
Traditional synchronous programming often leads to bottlenecks and delays. The counterintuitive approach is to embrace asynchronous programming. By allowing multiple tasks to execute concurrently without blocking each other, asynchronous programming significantly enhances responsiveness and efficiency. This approach is particularly effective in I/O-bound operations, where the program spends a considerable amount of time waiting for external resources like databases or network connections. Consider the development of a web application that needs to handle many simultaneous requests. Using asynchronous programming, the application can handle each request concurrently, minimizing latency and improving user experience. For example, Node.js, built on asynchronous programming principles, has become hugely popular for building high-performance web servers. A case study of a streaming service using Node.js demonstrated a 30% increase in concurrent user handling.
Asynchronous operations allow the program to continue executing other tasks while waiting for an I/O operation to complete, optimizing resource utilization. This concurrency avoids blocking the main thread, leading to improved responsiveness. Compare this to synchronous programming where a single operation can halt the program's execution until completed, greatly diminishing efficiency. One striking example is seen in gaming applications, where asynchronous loading of game assets prevents lag and freezing. This asynchronous approach is particularly noticeable in large-scale, online games, allowing for fluid gameplay despite complex interactions and large datasets. A study of a major multiplayer online game illustrated a 50% decrease in response times due to optimized asynchronous processes.
Understanding and utilizing asynchronous frameworks is crucial for any developer looking to build scalable applications. Frameworks like Asyncio in Python and the JavaScript event loop provide effective mechanisms for handling asynchronous operations. Using these tools requires a different mindset from traditional programming, necessitating developers to anticipate and manage asynchronous callbacks and promises. A case study focusing on a financial trading platform demonstrated improved execution speed of 20% by employing asynchronous communication between different modules, highlighting the importance of these frameworks. The transition to asynchronous practices can initially present a learning curve, yet the long-term benefits in terms of scalability and performance vastly outweigh the initial effort.
While asynchronous programming offers numerous advantages, proper error handling and debugging can be more challenging. Unhandled exceptions in asynchronous code can be difficult to trace. This demands a robust testing strategy and a careful approach to exception handling. Yet, with disciplined planning and systematic error handling implementation, the advantages of asynchronous programming greatly surpasses its complexities. A recent case study of a large-scale cloud application demonstrated a significant increase in application stability by implementing a thorough asynchronous error-handling strategy, emphasizing that appropriate strategies can mitigate potential issues. Therefore, mastery of asynchronous programming is an increasingly valuable skill in modern software development.
The Power of Microservices
Monolithic architectures, once the standard, are now increasingly challenged by the rise of microservices. The counterintuitive aspect is that breaking a large application into smaller, independent services can paradoxically improve maintainability, scalability, and resilience. Instead of a single, large codebase, a microservices architecture uses many smaller services, each responsible for a specific function. This modularity allows for independent development, deployment, and scaling of individual services. Consider an e-commerce application; rather than one monolithic application, it can be divided into separate microservices for user authentication, product catalog, shopping cart, and payment processing. This architecture enables independent scaling of different services according to demand. A case study of a large online retailer showcased a significant improvement in performance and scalability through the implementation of a microservices architecture. Their system could independently scale up the payment processing service during peak sales periods without affecting other aspects of the platform. Another success story involved a media streaming service that transitioned to microservices, dramatically improving their ability to respond to traffic spikes and maintain application stability.
Each microservice can be developed and deployed independently, facilitating continuous integration and delivery. This independent deployment cycle permits faster release cycles and less disruption to the overall system. The flexibility offered by microservices allows for the use of different technologies for different services, enabling teams to choose the best tool for each job. For example, one service might use Java while another might use Node.js, optimized for their respective functions. A case study involving a financial technology company demonstrated that the adoption of microservices resulted in a 40% reduction in deployment time. This speed significantly improved responsiveness to market demands and competitive pressures. Another example comes from a large social media company which migrated to a microservices architecture to manage their ever-growing user base, enabling them to handle significant traffic surges during peak hours without causing significant performance degradation.
However, the transition to microservices isn’t without challenges. Managing the inter-service communication, ensuring data consistency across services, and monitoring the overall system become more complex. It necessitates a shift in infrastructure, requiring tools for service discovery, load balancing, and centralized logging. These challenges require a careful consideration of the infrastructure involved and investment in appropriate tools and processes. A case study comparing a monolithic architecture with a microservices architecture illustrated that although the microservices setup had a higher initial investment in infrastructure and management, it provided substantial cost savings in the long run due to increased efficiency and scalability.
Despite these initial hurdles, the long-term benefits of increased agility, scalability, and resilience often outweigh the initial complexities. Effective monitoring and logging are critical in a microservices environment to identify and resolve issues quickly. This requires the implementation of appropriate monitoring tools and the establishment of robust alerting systems to ensure that any problems are detected and addressed promptly. A case study of a global financial institution highlighted the critical role of efficient monitoring and logging in maintaining the stability and resilience of their microservices architecture. The implementation of a sophisticated monitoring system helped to resolve a critical system failure in a matter of minutes, minimizing downtime and preventing significant financial losses. Therefore, a well-planned transition, considering the associated challenges, leads to a robust and efficient system.
Prioritize Security from the Start
Often, security is considered an afterthought, tacked on after the main development is complete. The counterintuitive approach is to integrate security from the very inception of the project. By prioritizing security from the outset, developers can build robust systems that are less vulnerable to attacks. This proactive approach involves implementing secure coding practices, performing regular security assessments, and promptly addressing identified vulnerabilities. Consider the practice of secure coding practices such as input validation and output encoding. These preventative methods can prevent many common vulnerabilities from exploiting the software application. A case study on a major banking institution illustrated how the application of these principles reduced the number of security incidents by over 50%. Another example of preventative security measures includes the use of strong password policies and multi-factor authentication to prevent unauthorized access to sensitive data.
Security is not just about technology; it’s also about people and processes. Employee training on security best practices and development of strong security policies play crucial roles in maintaining system security. Regular security audits and penetration testing help identify vulnerabilities before they can be exploited by malicious actors. A case study on a major e-commerce platform indicated that incorporating these best practices significantly minimized their exposure to cyber attacks. This proactive approach prevented a costly data breach and secured the trust of their customers. An example of successful security training resulted in a 70% increase in employee awareness of security threats and their corresponding responses.
Incorporating automated security testing into the development pipeline can drastically improve the efficiency of detecting vulnerabilities. Tools like static and dynamic application security testing (SAST and DAST) can automatically identify security flaws during the development process. This approach enables early detection and remediation of security risks, resulting in stronger overall security. A case study involving a healthcare organization demonstrated how automated security testing decreased the number of vulnerabilities missed during the testing phases by 60%. Similarly, the implementation of a robust security information and event management (SIEM) system can actively monitor for suspicious activities and alert security personnel to potential threats in real-time. This proactive threat detection enabled rapid response and mitigated the impact of security incidents.
The modern software development landscape necessitates a continuous security approach. Regular updates, patches, and vulnerability management are essential to maintain a strong security posture. The ongoing evolution of security threats requires continuous monitoring, adaptation, and updates to security protocols. Consider a case study on a leading social media platform showcasing how their continuous security updates and rapid response to vulnerabilities mitigated millions of potential security threats. A comprehensive approach, encompassing people, processes, and technology, makes for a strong security foundation and helps to protect against increasingly sophisticated cyber threats.
Leverage AI and Automation
Artificial intelligence (AI) and automation are transforming software development. The counterintuitive aspect is that while AI might seem to replace human developers, it actually complements and enhances their capabilities. AI-powered tools can automate repetitive tasks, improve code quality, and accelerate the development process. Consider AI-powered code completion tools, which can significantly speed up the coding process by suggesting relevant code snippets and completing lines of code automatically. A case study on a large software company showed a 20% increase in developer productivity by employing AI-powered code completion tools. Similarly, AI-powered bug detection tools can automatically identify and flag potential bugs in the codebase, improving code quality and reducing debugging time. Another case study showed a 30% decrease in bug detection time using an AI-powered bug detection tool.
AI can also help in testing and quality assurance. AI-powered testing tools can automate the testing process, generating test cases, executing tests, and analyzing results. This can reduce testing time and improve the coverage of test cases. For example, one case study showed a 40% increase in test coverage and a 25% reduction in testing time by employing AI-powered testing tools. These tools are often coupled with other AI-powered tools, streamlining the entire development pipeline. Consider an AI-powered code review system which analyzes the codebase for potential vulnerabilities, security flaws, and stylistic inconsistencies. This comprehensive approach identifies issues that human reviewers might miss, thus reducing risks and errors.
The use of AI in software development is continuously evolving. New AI-powered tools are continually being developed to improve various aspects of the software development lifecycle. From AI-powered project management tools to AI-powered deployment automation, AI is transforming how software is built and deployed. Another case study showed how an AI-powered project management tool helped to improve project planning and execution, reducing project delays and cost overruns. Therefore, the integration of AI and automation has proven to significantly enhance efficiency and output in modern software development.
However, it’s important to remember that AI is a tool, not a replacement for human expertise. Human developers are still essential for designing, architecting, and overseeing the development process. While AI can automate many tasks, the strategic thinking, creative problem-solving, and critical judgment of human developers are irreplaceable. A case study highlighting the use of AI in a large-scale project emphasized the importance of human oversight. The team utilized AI to assist with routine tasks, but human developers ultimately remained in charge of designing, problem-solving, and overseeing the final product. Therefore, a harmonious collaboration of human expertise and advanced AI systems constitutes the most effective approach.
Conclusion
The software development landscape is a realm of constant evolution. What seems intuitive often yields to more efficient, albeit counterintuitive, approaches. Embracing these unexpected methodologies, such as prioritizing concise code, leveraging asynchronous programming, adopting microservices, integrating security from the start, and harnessing the power of AI and automation, can significantly enhance the speed, efficiency, and resilience of software development projects. This guide has merely scratched the surface of these powerful, often unexpected strategies; continuous learning and adaptation remain crucial for any software developer seeking to thrive in this ever-changing field.