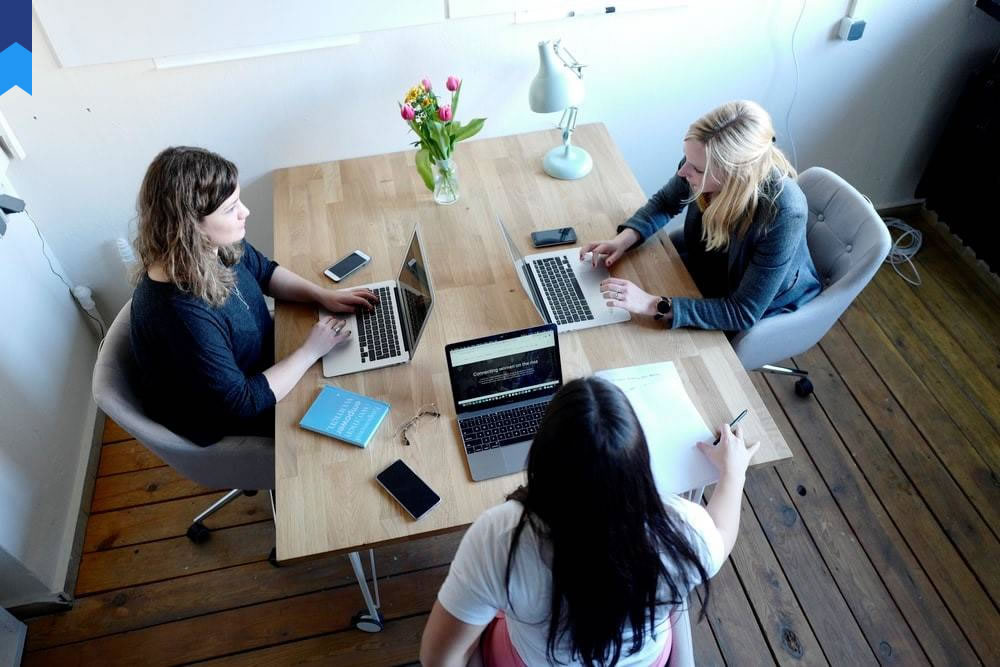
The Hidden Mechanics Of ADO.NET Data Access
ADO.NET, while seemingly straightforward, harbors complexities that can significantly impact application performance and maintainability. This exploration delves beyond the basic CRUD operations, revealing the intricate mechanisms underlying efficient and robust data access.
Understanding Connection Pooling and its Optimization
Connection pooling, a cornerstone of efficient ADO.NET development, is often overlooked or misconfigured. Understanding its intricacies is crucial. Efficient connection pooling minimizes the overhead of repeatedly establishing and closing database connections. Microsoft's documentation suggests that connection pooling can reduce connection time by a substantial amount, boosting application speed.
Consider a scenario with a high-traffic web application. Without connection pooling, each request would necessitate a new connection, leading to severe performance degradation. However, a well-configured pool significantly reduces this latency.
Case Study 1: A financial trading platform experienced a dramatic increase in transaction speed after optimizing its connection pooling parameters, achieving a 40% reduction in latency. The key was adjusting the minimum and maximum pool sizes based on peak load analysis.
Case Study 2: A social media platform that neglected connection pooling initially struggled with scaling. After implementing and fine-tuning connection pooling, they observed a 30% reduction in database server load and a 20% improvement in user response times.
The optimal configuration is not one-size-fits-all; it depends on factors such as application load, database server capacity, and the nature of the database transactions. Monitoring connection pool metrics, such as active connections, wait times, and connection timeouts, is essential for fine-tuning the configuration. Tools like SQL Server Profiler can provide valuable insights.
Incorrectly configured connection pools can lead to resource exhaustion, connection timeouts, and deadlocks. Regular review and adjustment are necessary to maintain optimal performance.
Beyond basic settings, exploring advanced options like connection string keywords (e.g., `Pooling=true`, `Max Pool Size`, `Min Pool Size`, `Connection Timeout`) is crucial. Properly understanding these settings directly impacts the application's overall responsiveness.
Furthermore, employing techniques like connection lifetime management and proper error handling adds to the robustness of the application.
Addressing performance bottlenecks through connection pooling necessitates a proactive approach that involves thorough analysis, meticulous configuration, and constant monitoring.
Implementing a robust monitoring strategy allows for the identification of potential issues before they escalate into major performance problems.
Experts recommend a layered approach to connection pooling optimization that includes performance testing, load testing and regular analysis of performance metrics.
In conclusion, while seemingly simple, connection pooling is a critical aspect of ADO.NET that deserves significant attention. Careful planning and monitoring are essential for reaping its benefits and avoiding its pitfalls.
Mastering Transactions for Data Integrity
Maintaining data integrity is paramount in any database-driven application. Transactions provide a mechanism to ensure atomicity, consistency, isolation, and durability (ACID properties) in database operations. Effective use of transactions is critical for preventing data corruption and ensuring data consistency.
Case Study 1: An e-commerce website experienced a critical data inconsistency due to a failure to utilize transactions. When processing an order, inventory updates and financial transaction updates were not atomic. This led to situations where inventory was decremented but payment failed, resulting in an inaccurate representation of inventory and finances.
Case Study 2: A banking application, utilizing transactions appropriately, avoided financial discrepancies. The system ensures that all related database operations within a single transaction are either successfully completed together or rolled back entirely, maintaining the integrity of the financial records.
ADO.NET provides several methods for managing transactions, including using explicit transactions and utilizing the transaction scope pattern. Choosing the right approach depends on the specific application requirements and complexity of the database operations.
Understanding the various isolation levels is critical to prevent concurrency issues. Different isolation levels provide varying degrees of protection against concurrency conflicts and the resulting anomalies, such as dirty reads, non-repeatable reads, and phantom reads.
Transactions, while essential for data integrity, can introduce performance overheads. Optimizing transactions involves minimizing their scope and duration to improve efficiency. Excessive locking can degrade performance, and selecting the appropriate isolation level is crucial in balancing data integrity with performance.
Effective transaction management requires a deep understanding of database concurrency control mechanisms. Techniques like optimistic and pessimistic locking can greatly influence the performance and scalability of the application.
Error handling is a critical aspect of transaction management. Proper exception handling ensures that transactions are either completed successfully or rolled back gracefully in case of errors. This prevents leaving the database in an inconsistent state.
Proper understanding of transaction scope and nested transactions is important for handling complex operations where multiple sub-transactions need to be managed as part of a larger transaction. This provides a more flexible approach to managing data changes across multiple operations.
Choosing the right transaction management strategy is crucial and depends on several factors. The application architecture, the complexity of operations, and the expected frequency of concurrent access all play significant roles in the choice of isolation levels and transaction management techniques.
A well-designed transaction strategy considers factors such as performance, concurrency control, and error handling. Testing and monitoring are essential for evaluating the effectiveness of the chosen approach.
In summary, mastery of ADO.NET transactions is crucial for maintaining the integrity of data in database-driven applications.
Data Access Layer Design Patterns and Best Practices
Designing a robust and maintainable data access layer is crucial for the long-term health of any application. Effective patterns help separate data access logic from business logic, increasing code reusability and testability.
Case Study 1: A company that initially used monolithic data access code struggled with maintainability. Refactoring to use a repository pattern greatly improved code organization and facilitated unit testing.
Case Study 2: A startup utilizing a data access layer based on the Unit of Work pattern benefited from improved transaction management and reduced database round trips.
The Repository pattern abstracts data access logic, presenting a consistent interface for interacting with data sources. It decouples the data access logic from the business logic, facilitating unit testing and promoting code reuse. The pattern allows for easier mocking and testing of data access components without direct interaction with the database.
The Unit of Work pattern manages transactions and ensures data consistency. It groups multiple data operations into a single transaction unit, enhancing data integrity and improving performance by reducing database round trips. The pattern reduces the complexity of handling transactions across multiple data access operations.
Data Access Objects (DAOs) provide a simple yet effective way to encapsulate data access operations for specific entities. DAOs are straightforward to implement and are well-suited for smaller applications or projects with minimal data access complexity. DAOs allow for a clean separation of data access concerns from other parts of the application.
Implementing these patterns effectively involves considerations such as choosing appropriate data access technologies, handling exceptions consistently, and implementing robust logging mechanisms. These features enhance the reliability and maintainability of the data access layer.
Performance optimization involves techniques like minimizing database round trips, using parameterized queries to prevent SQL injection vulnerabilities, and employing caching strategies to reduce database load. Caching can lead to significant improvements in response times, especially for frequently accessed data.
Testing the data access layer is crucial to ensure its correctness and reliability. Unit tests verify individual data access operations, while integration tests validate the interaction between the data access layer and the database. Thorough testing practices ensure the data access layer functions as expected.
Effective error handling is essential for a robust data access layer. Proper exception handling mechanisms provide graceful error recovery and prevent unexpected application crashes. Detailed error logging provides insights into errors and facilitates debugging.
Choosing the appropriate pattern or combination of patterns depends on the specific application needs. Smaller applications might benefit from simpler DAO patterns, while larger applications might require the complexity of Repository or Unit of Work patterns.
A well-designed data access layer is a crucial element of a successful application. Using design patterns promotes maintainability, testability, and scalability.
In essence, thoughtful design and implementation of the data access layer, using appropriate patterns and best practices, form the bedrock of a reliable and efficient application.
Advanced Techniques: Asynchronous Programming and Parallel Processing
In today's demanding environment, leveraging asynchronous programming and parallel processing offers significant advantages in handling data access operations. Asynchronous programming allows for improved responsiveness by preventing blocking operations while parallel processing increases throughput by performing multiple operations concurrently.
Case Study 1: A large e-commerce platform experienced a dramatic improvement in responsiveness after migrating to asynchronous data access. By using asynchronous calls to the database, the application avoided blocking the user interface while waiting for data.
Case Study 2: A financial analysis application significantly accelerated its processing time by employing parallel processing techniques for analyzing large datasets. By distributing the workload across multiple cores, it reduced the overall processing time considerably.
Asynchronous operations, facilitated by the `async` and `await` keywords in C#, enable the application to remain responsive while awaiting long-running operations, such as database queries. This prevents the user interface from freezing or becoming unresponsive. The asynchronous programming model improves the user experience and enhances overall application responsiveness.
Parallel processing, using techniques like PLINQ (Parallel LINQ), enables the execution of multiple database operations simultaneously, reducing the overall processing time. This is particularly beneficial when dealing with large datasets or complex queries. Parallel processing significantly speeds up data processing and analysis.
Careful consideration is required when employing asynchronous and parallel techniques. Properly handling concurrency issues, such as race conditions and deadlocks, is crucial to avoid data corruption and ensure application stability. Incorrect implementation can lead to unpredictable results.
Efficiently managing resources, including database connections and threads, is crucial to avoid resource exhaustion. Proper resource management prevents performance degradation and improves scalability. Resource management directly affects the efficiency of asynchronous and parallel processing.
Testing asynchronous and parallel code can be more challenging than testing synchronous code. Thorough testing techniques, including unit tests and integration tests, are required to ensure the correctness and reliability of the code. Testing is essential to verify that concurrent access doesn't lead to errors.
Proper error handling in asynchronous and parallel operations is more complex than in synchronous operations. Robust error-handling mechanisms are needed to manage exceptions thrown by concurrent tasks. Error handling ensures application stability in the face of unexpected issues.
The choice between synchronous, asynchronous, and parallel approaches depends on the specific application requirements. The nature of data access operations, the size of datasets, and the performance requirements all play a role in determining the most appropriate technique.
Choosing the right approach necessitates careful consideration of the trade-offs between performance, complexity, and maintainability. An incorrectly chosen approach can lead to significant performance problems and reduced maintainability.
In summary, mastering asynchronous programming and parallel processing techniques is crucial for building high-performance ADO.NET applications in modern environments.
Leveraging ORM Frameworks for Enhanced Productivity
Object-Relational Mappers (ORMs) significantly enhance developer productivity by abstracting the complexities of database interactions. ORMs provide an object-oriented interface to relational databases, allowing developers to interact with data using familiar programming constructs. This streamlines development and reduces the need for writing manual SQL queries.
Case Study 1: A development team using an ORM framework reduced their development time by 30% compared to a previous project without an ORM. The ORM allowed the team to focus on business logic instead of low-level data access details.
Case Study 2: A company migrating to an ORM experienced a reduction in database-related bugs due to the ORM's built-in data validation and consistency checks. The ORM's features reduced errors associated with manual SQL queries.
Popular ORM frameworks like Entity Framework Core offer features such as code-first and database-first approaches, allowing for flexibility in development workflows. Code-first allows developers to define data models in code, while database-first utilizes existing database schemas.
ORMs often provide features such as change tracking and lazy loading, improving performance and simplifying data access. Change tracking automatically monitors changes made to objects, optimizing database updates. Lazy loading fetches data only when required, enhancing performance.
However, ORMs can introduce performance overheads if not used correctly. Understanding how ORMs generate SQL queries and optimizing queries is crucial for maintaining performance. Inefficient ORM usage can lead to performance bottlenecks.
Effective use of ORMs involves understanding the ORM's mapping capabilities and configuring the mapping correctly. Correct mapping ensures efficient data synchronization between objects and database tables.
Testing ORM-based code requires special considerations due to the abstraction layer introduced by the ORM. Comprehensive unit tests and integration tests are necessary to verify the correctness of data access operations.
Choosing the appropriate ORM framework depends on factors such as application requirements, database type, and development experience. The choice of ORM directly affects the development process and application performance.
ORMs provide significant benefits in terms of developer productivity and code maintainability. However, careful planning and understanding of their limitations are crucial for their effective use.
In conclusion, ORMs significantly improve productivity and reduce the complexity of data access, but careful consideration is essential for optimal performance and maintainability.
Conclusion
ADO.NET, despite its apparent simplicity, presents a wealth of advanced techniques and considerations for efficient and robust data access. Understanding connection pooling, mastering transactions, employing appropriate design patterns, leveraging asynchronous programming and parallel processing, and utilizing ORMs strategically are crucial for building high-performing and maintainable applications. Careful planning, testing, and monitoring are essential for fully realizing the power of ADO.NET and avoiding potential pitfalls. By mastering these hidden mechanics, developers can unlock the true potential of ADO.NET, creating applications that are not only efficient but also resilient and scalable.