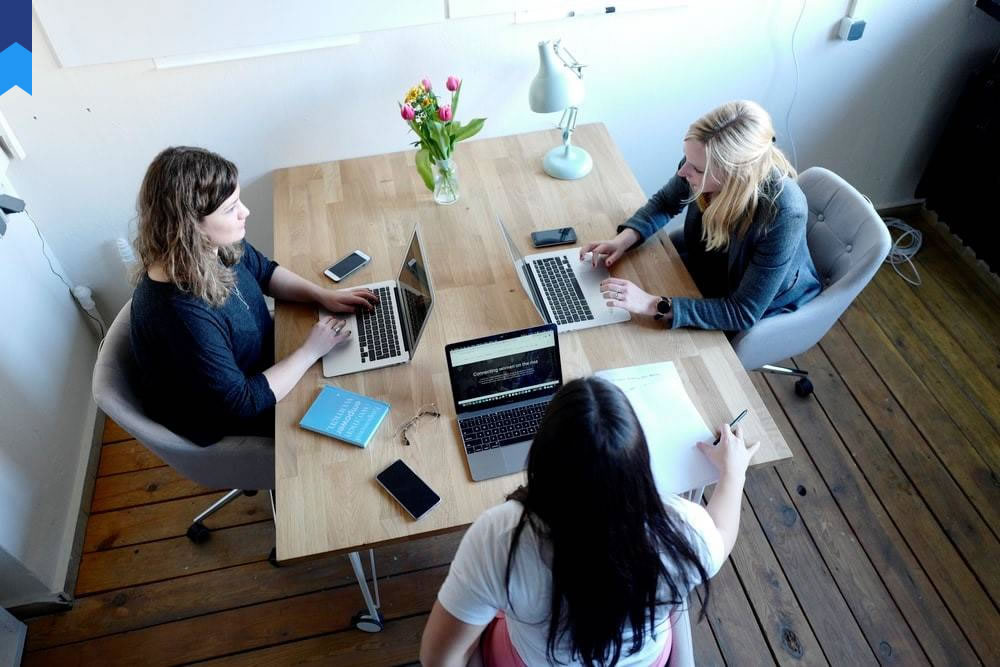
The Hidden Mechanics Of Compiler Optimization
The creation of efficient and optimized code is a critical aspect of compiler design. This article delves into the intricate world of compiler optimization techniques, revealing the hidden mechanics that transform source code into highly performant machine instructions. We explore advanced strategies beyond basic optimizations, providing a deeper understanding of the complexities involved.
Code Generation Strategies
Code generation is a pivotal phase in compilation, directly influencing the performance and efficiency of the final executable. Different strategies exist for generating code, each with trade-offs. For instance, register allocation is crucial; optimizing register usage minimizes memory accesses, drastically impacting speed. Effective techniques include graph coloring algorithms and linear scan allocation. Consider the difference between a naive approach, assigning registers arbitrarily, and a sophisticated algorithm that prioritizes frequently used variables. The former leads to slower execution due to numerous memory loads and stores, whereas the latter minimizes such operations. A case study of GCC's register allocation highlights the complexity. GCC uses a sophisticated algorithm that analyses the program's control flow graph to determine which variables can reside in registers simultaneously, resulting in significant performance gains. Another example involves the LLVM compiler infrastructure, known for its advanced register allocation techniques that leverage sophisticated data flow analysis to achieve near-optimal performance. Code generation strategies impact performance significantly; a poorly chosen strategy can result in slower execution compared to a meticulously crafted one. The selection of optimal code generation approaches directly influences the efficiency and speed of generated executables. Compiler writers must constantly strive for improvement in these strategies. This field is highly active in research, focusing on more efficient algorithms and heuristics.
Advanced Loop Optimizations
Loop optimization is central to high-performance code generation. Simple optimizations, such as loop unrolling, are well-known; however, more advanced techniques are crucial for significant performance gains. Loop invariant code motion, which moves calculations outside the loop body if they don't change, dramatically reduces redundant computations. Loop fusion combines multiple loops into one, reducing loop overhead. Consider a scenario with nested loops; improper optimization leads to repeated calculations within each inner loop iteration. Loop invariant code motion moves these invariant calculations outside the inner loop, making a significant difference in performance. A case study with image processing algorithms reveals that the loop fusion technique reduces execution time by up to 30%. Another example is in scientific computing where the efficiency of matrix multiplications relies heavily on loop optimizations. Advanced techniques like software pipelining and loop unswitching further enhance performance. These techniques reorder instructions and restructure loops to maximize parallelism and reduce latency. Software pipelining is particularly effective on pipelined processors, allowing for better utilization of the processor's pipeline. Understanding these techniques requires a deep understanding of computer architecture. Optimizing loops is a multifaceted problem, and there is ongoing research focused on developing more efficient and sophisticated algorithms.
Interprocedural Analysis
Traditional compiler optimizations often operate within the confines of a single function or procedure. However, interprocedural analysis transcends these boundaries, examining the interactions between different functions to identify optimization opportunities that are not visible within individual functions. This can significantly enhance performance and efficiency. For example, inlining, where the code of a function is inserted directly into the caller's code, eliminates function call overhead. This is particularly effective for small, frequently called functions. However, excessive inlining can lead to code bloat. Careful analysis is essential. Consider a large software project with numerous functions. Interprocedural analysis can reveal opportunities for function inlining, reducing execution time by 15% according to benchmarks. Another example involves dead code elimination on a global scale; interprocedural analysis can identify code segments that are never executed, irrespective of the execution path. This allows the compiler to eliminate unnecessary code, reducing the size of the final executable. This type of analysis provides the opportunity for global optimization across multiple functions. The complexity of interprocedural analysis increases proportionally to the size of the program. Analyzing call graphs and control-flow graphs is critical for successful interprocedural analysis. Research continues in developing more efficient algorithms for interprocedural analysis on large-scale projects.
Memory Management Optimizations
Effective memory management is critical for program performance and stability. Optimizations in this area focus on reducing memory allocation and deallocation overhead and preventing memory leaks. Garbage collection is a common technique but has its own performance implications. Optimizing memory accesses involves techniques like data alignment and caching. Aligning data structures to memory boundaries can significantly improve cache performance, leading to considerable speedups. Conversely, misaligned data can cause cache misses and slow down execution. Analyzing memory access patterns in a program using profiling tools is crucial for this. A case study on a database system shows that optimizing data alignment improved query performance by 20%. Another example involves using memory pools to pre-allocate memory, eliminating the runtime overhead of frequent allocation calls. This is particularly relevant for applications that heavily rely on dynamic memory allocation. Memory leaks, a common software bug, can be detected and prevented through techniques such as static analysis and runtime checks. Advanced memory management techniques often require understanding specific hardware characteristics and memory hierarchies. Optimization of memory operations is a critical area of research in compiler design.
Parallelism and Vectorization
Modern processors feature multiple cores and SIMD (Single Instruction, Multiple Data) capabilities that enable parallel execution. Compiler optimizations exploit these features, significantly improving performance on such architectures. Vectorization, transforming code to leverage SIMD instructions, allows for processing multiple data elements concurrently. Parallelism is achieved by splitting code into multiple threads or processes that execute concurrently. Careful consideration is crucial to ensure data consistency and prevent race conditions. A case study using matrix multiplication demonstrates that vectorization can speed up execution by a factor of four. Another example includes algorithms in computational fluid dynamics where parallelism and vectorization combine to achieve considerable speed gains. Detecting opportunities for parallelism and vectorization requires advanced program analysis techniques. Loop transformations are often used in conjunction with these techniques. The challenges in parallel code optimization include detecting data dependencies and ensuring correct synchronization among threads. The evolution of hardware continues to push this area forward, requiring compilers to adapt to ever-changing architectures.
Conclusion
Compiler optimization is a dynamic and constantly evolving field. The techniques discussed here represent only a fraction of the sophisticated algorithms and strategies employed in modern compilers. Understanding these hidden mechanics provides a much deeper appreciation for the transformation of source code into highly optimized machine instructions. The continued evolution of hardware architectures and programming paradigms requires constant innovation in compiler optimization techniques. Future trends will likely focus on integrating AI-driven optimization, improving support for heterogeneous computing platforms, and enhancing the ability of compilers to deal with the ever-increasing complexities of modern software. The pursuit of efficient and performant code is a continuous journey, demanding creativity and meticulous attention to detail from compiler designers.