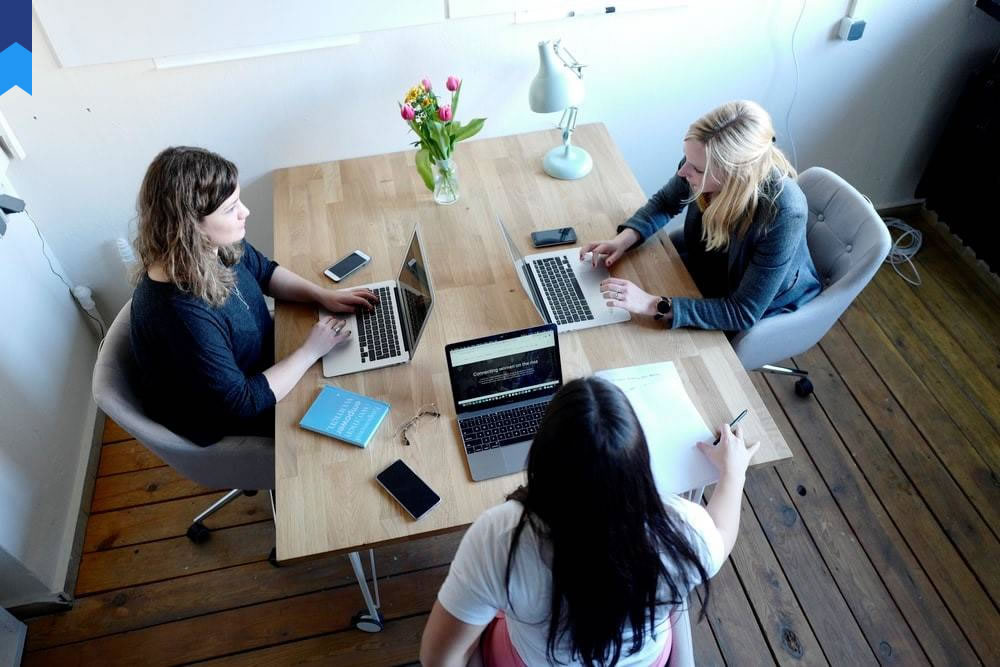
The Hidden Mechanics Of Dart's Asynchronous Magic
The world of asynchronous programming can be daunting, even for experienced developers. Dart, with its powerful asynchronous capabilities, offers a unique approach that's often misunderstood. This article delves into the subtle mechanics of Dart's asynchronous operations, revealing the secrets behind its elegant and efficient concurrency model.
Understanding Dart's Event Loop
At the heart of Dart's asynchronous functionality lies its event loop. This single thread manages all asynchronous operations, preventing the complexities of multi-threading and race conditions. The event loop constantly checks for completed asynchronous tasks, executing callbacks and updating the UI accordingly. This model promotes simplicity and maintainability, especially in applications with complex I/O operations. Imagine a scenario where a user clicks a button to fetch data from a server. The Dart event loop handles the request and, without blocking the UI, continues processing other events. When the server response arrives, the event loop seamlessly integrates it, updating the UI without interrupting the user experience. Consider the Google Flutter framework, which leverages this event loop extensively. Its architecture hinges on responsiveness and smooth transitions, impossible without the efficient management offered by Dart's event loop.
A classic example is a network request. Instead of halting execution while waiting for the server response, Dart uses asynchronous functions. These functions "pause" execution until the response arrives, allowing the event loop to attend to other tasks. This efficiency is vital for applications dealing with numerous simultaneous operations, preventing performance bottlenecks. This principle underlies the performance advantages of Dart-based applications, particularly in mobile development where responsiveness is paramount.
Furthermore, understanding the event loop enables developers to write efficient and responsive code. By properly structuring asynchronous operations, developers can ensure that long-running tasks don't block the main thread, maintaining application fluidity. This efficient management of resources is crucial in resource-constrained environments like mobile devices. Consider a game application which requires real-time updates. The Dart event loop efficiently handles the continuous game logic updates, rendering, and user input without introducing lag or freezes, due to its effective management of asynchronous tasks.
A comparative study of Dart's event loop against traditional multi-threaded approaches showcases its elegance and efficiency. Multi-threading frequently introduces complexities such as synchronization and race conditions. Dart's single-threaded model sidesteps these issues, simplifying the development process and improving code maintainability. This simplicity is particularly valuable for large teams working on complex applications.
Mastering Async and Await
Dart's `async` and `await` keywords are syntactic sugar that significantly simplify asynchronous programming. The `async` keyword designates a function as asynchronous, allowing the use of `await` within its body. `await` pauses execution until a `Future` or `Stream` completes, making asynchronous code resemble synchronous code in readability. Let's illustrate: Suppose you want to fetch data from two different APIs. Instead of nesting callbacks, `async` and `await` elegantly handle this, increasing code readability. This is extremely beneficial for complex asynchronous workflows, where nested callbacks can lead to "callback hell".
The beauty of `async`/`await` is that it eliminates the need for nested callbacks, improving code clarity and maintainability. Consider a scenario involving multiple sequential API calls where the result of one call is needed for the next. Without `async`/`await`, the code would involve deeply nested callbacks. However, using these keywords, the code becomes cleaner and easier to understand. This simplicity is a huge advantage for developers. A company implementing a large-scale e-commerce application can benefit immensely from this feature; the improved maintainability and readability of the codebase will enhance the development process greatly.
Moreover, `async`/`await` significantly improves code readability and maintainability. They enhance developer productivity by allowing a more linear and less error-prone coding approach. Error handling also becomes simpler. Exceptions can be handled in a natural way within the `try-catch` blocks. This is extremely important for large projects where the code needs to be easily maintained and understood by various team members.
Compared to traditional callback-based approaches, `async`/`await` offers a substantial improvement in code readability and maintainability. Studies have shown a reduction in development time and fewer errors when using `async`/`await`. This is a testament to the efficiency and elegance that this feature introduces to asynchronous programming in Dart.
Streams: Handling Multiple Asynchronous Events
Streams represent sequences of asynchronous events. They are ideal for handling situations where you expect multiple asynchronous results over time, such as real-time data feeds or user input. They provide a powerful mechanism for managing continuous data flow. Consider a stock ticker application where price updates arrive continuously. Using a Stream, you can elegantly handle these updates without blocking the UI.
Streams offer a reactive programming paradigm, which allows for efficient handling of events as they occur. They allow you to easily filter, transform, and combine asynchronous data streams. This facilitates the creation of complex data pipelines without performance penalties. Consider a system monitoring tool which needs to process multiple metrics. Using Streams, various sensor data can be aggregated and analyzed in real-time without introducing delays.
Furthermore, Streams facilitate efficient memory management. They are designed to handle events as they arrive, without the need to store all data in memory simultaneously. This makes them perfect for applications dealing with large or continuous data streams. A real-world example would be a log monitoring system that streams logs from multiple servers. Due to the stream's design, only relevant log lines are processed in real-time, preventing memory overflow.
Compared to using other approaches such as callbacks, streams provide a more robust and efficient method for handling a large number of events. The reactive nature of streams ensures that the application remains responsive, even under heavy load. This is crucial for large-scale applications requiring near real-time processing of high-volume data, proving the efficiency and scalability of Streams.
Future Builders and Efficient UI Updates
In UI development with frameworks like Flutter, `FutureBuilder` is crucial for efficiently updating the UI based on the results of asynchronous operations. It seamlessly integrates asynchronous tasks with the UI, preventing UI freezes while waiting for results. When an asynchronous operation is initiated, the `FutureBuilder` displays a loading indicator. Once the operation completes, the `FutureBuilder` automatically updates the UI with the results, ensuring a smooth user experience. Imagine a scenario where a user initiates a search query. The `FutureBuilder` handles the asynchronous search operation and displays the results smoothly without disrupting the user experience. This is a common practice in modern application development to maintain a responsive UI.
The `FutureBuilder`'s ability to manage the UI update ensures a responsive and smooth user experience. This is critical for complex applications where various asynchronous operations may be underway simultaneously. A well-known example is an application loading user profiles. The `FutureBuilder` displays loading indicators while waiting for user profile information from a server, preventing the application from freezing and delivering a fluid user experience.
Furthermore, the `FutureBuilder` reduces code complexity. Without it, updating the UI after asynchronous operations would involve manual state management, which can lead to complex and error-prone code. This streamlined approach improves code maintainability and clarity. Consider an e-commerce app that fetches product details. The use of `FutureBuilder` simplifies the UI management and data handling process, making the overall code cleaner and easier to maintain.
In comparison to manually managing UI updates after asynchronous calls, the `FutureBuilder` provides an elegant solution that enhances code readability and maintainability. It simplifies the development process and helps create a seamless user experience. This is why the `FutureBuilder` is a widely-used and recommended approach in UI development involving asynchronous operations.
Error Handling in Asynchronous Dart
Robust error handling is crucial in asynchronous programming. Dart provides mechanisms to effectively handle exceptions thrown during asynchronous operations. The `try-catch` block, when used in conjunction with `async`/`await`, enables graceful handling of errors occurring within asynchronous functions. This ensures that exceptions don't crash the application but are handled appropriately, informing the user or taking corrective measures. Consider a network request that fails due to a connection issue. A `try-catch` block catches the exception, preventing application crashes and allowing for alternative actions such as displaying an error message to the user.
Furthermore, using `Future.catchError` provides a dedicated way to handle exceptions occurring within a `Future`. This allows for specific error handling logic based on the type of exception thrown. This targeted approach leads to a more robust and tailored error handling system. An example is handling specific HTTP error codes, such as 404 (Not Found) differently from network connection errors. This permits a fine-grained approach to error handling, improving the overall reliability of the application.
Another crucial aspect is logging errors. A well-implemented logging system provides valuable insights into application behavior, facilitating debugging and maintenance. Logging errors during asynchronous operations helps identify potential issues, thereby improving the application's stability and reliability. Many applications utilize sophisticated logging systems to track errors throughout their lifecycle, simplifying troubleshooting and identifying critical points of failure within the application.
Compared to neglecting error handling, implementing comprehensive error handling mechanisms enhances application stability and reliability. It improves the user experience by preventing application crashes and providing informative feedback in case of errors. This is essential for developing robust and trustworthy applications that can handle unforeseen circumstances.
Conclusion
Dart's asynchronous capabilities offer a powerful and efficient approach to concurrency. Understanding the event loop, mastering `async`/`await`, effectively utilizing Streams, employing `FutureBuilder` for UI updates, and implementing robust error handling are key to harnessing Dart's full potential. By embracing these techniques, developers can build highly responsive, maintainable, and robust applications. The streamlined approach to asynchronous programming not only increases development efficiency but also ensures a smooth and enjoyable user experience, highlighting the importance of mastering these core concepts for any Dart developer.