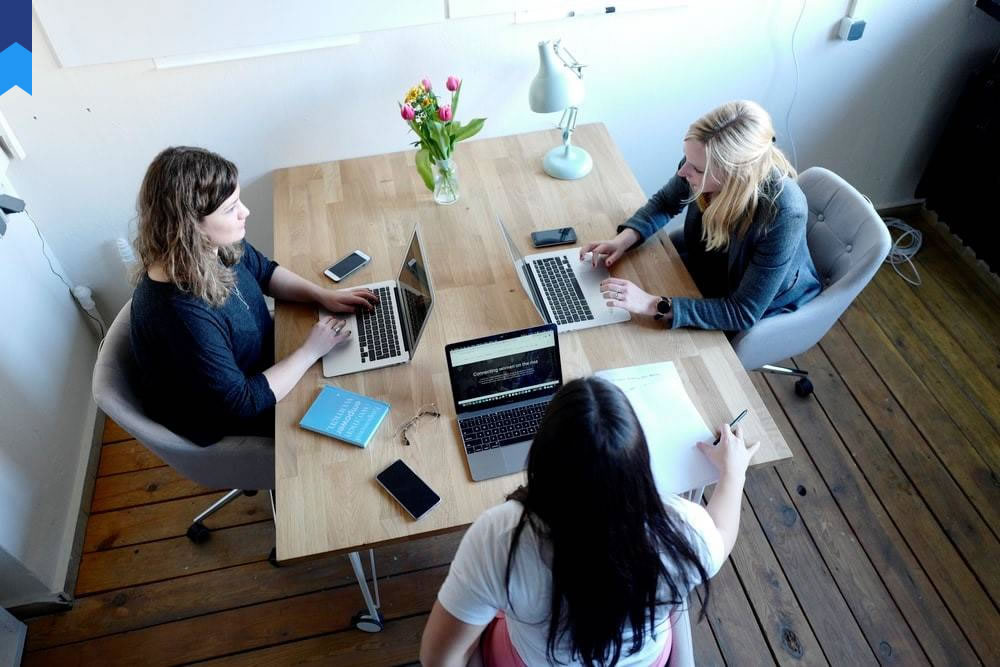
The Reality Behind Algorithm Design
The design and analysis of algorithms is a cornerstone of computer science, yet its practical application often deviates from theoretical ideals. This article delves into the complexities and realities encountered when translating theoretical algorithms into functional, efficient software.
Understanding Algorithmic Complexity in Practice
Algorithmic complexity, often expressed using Big O notation, provides a theoretical measure of an algorithm's efficiency. However, real-world scenarios rarely conform to these idealized models. Factors such as hardware limitations, data characteristics, and implementation details significantly impact actual performance. For instance, an algorithm with O(n log n) complexity might outperform an O(n) algorithm in practice if the constant factors associated with the O(n) algorithm are exceptionally large. Case study 1: A sorting algorithm with O(n log n) complexity might outperform a seemingly faster O(n) algorithm when dealing with significantly large datasets due to memory access bottlenecks. Case study 2: In a database query optimization scenario, a theoretically less efficient algorithm might perform better in a specific database system due to internal optimization techniques. The choice of data structures also plays a pivotal role. A well-chosen data structure can substantially improve algorithm efficiency, even if the underlying algorithm's complexity remains the same. Efficient memory management is also crucial, particularly when dealing with large datasets. Memory leaks or inefficient memory allocation can dramatically slow down algorithms, even those with low theoretical complexity. Consider the impact of caching strategies; careful caching can lead to significant performance gains, but poorly implemented caching can negate the efficiency of even the most optimized algorithms. The reality is that the 'best' algorithm often depends heavily on the context and must be rigorously tested.
The Unexpected Bottlenecks
Even well-designed algorithms can encounter unexpected bottlenecks. Network latency, I/O operations, and system resource contention can dramatically affect performance. These factors are often neglected in theoretical analyses but significantly influence real-world execution times. Case study 1: Consider a distributed algorithm designed for high throughput. Network latency can drastically reduce performance if not properly accounted for during design and testing. Case study 2: In machine learning, the training of complex models often involves significant I/O operations for reading and writing data. Inefficient I/O management can lead to unacceptable training times. It's crucial to profile the execution to pinpoint these bottlenecks. Profiling tools can help identify specific code sections consuming excessive time or resources, guiding optimization efforts towards the most impactful areas. Careful code optimization techniques, such as loop unrolling, instruction-level parallelism, and memory alignment, can further enhance performance. However, it's important to remember that premature optimization can be detrimental, and a focus on clean, well-structured code is often more effective in the long run. Modern compilers also play a key role, performing many optimizations that were previously performed manually. Understanding the capabilities of the compiler can inform design decisions and reduce the need for manual optimization.
Navigating the Trade-offs
Algorithm design often involves trade-offs between different aspects of performance, such as time complexity, space complexity, and code complexity. The optimal algorithm often depends on the specific constraints of the problem. Case study 1: In a real-time system, a slightly less efficient algorithm with lower space complexity might be preferred over a more efficient algorithm requiring significantly more memory. Case study 2: For a computationally intensive task on a resource-constrained device, a simpler algorithm might be preferred over a more complex, but theoretically more efficient algorithm. Furthermore, the maintainability and readability of the code must also be considered. A highly optimized but complex algorithm may be difficult to maintain and debug, leading to higher long-term costs. Therefore, a balance must be struck between performance and maintainability, with the optimal solution dependent on the specific context. This requires careful consideration of the project's goals and the long-term costs involved. Prioritizing well-structured and readable code often pays off in the long run, even if it sacrifices some initial performance gains. It is crucial to develop a clear understanding of the trade-offs involved before selecting an algorithm and to regularly evaluate these trade-offs throughout the development process.
The Role of Data
Data characteristics significantly influence algorithm performance. An algorithm that performs well with uniformly distributed data might perform poorly with highly skewed data. Case study 1: Consider a sorting algorithm designed for uniformly distributed data. The performance might degrade considerably when applied to data with a highly skewed distribution. Case study 2: In machine learning, the choice of algorithm might be dictated by the nature of the data. For instance, algorithms that work well with structured data might not perform as well with unstructured data. The choice of data structures is also crucial; selecting a data structure that is well-suited to the data can greatly improve efficiency. Algorithms need to be robust to handle various data qualities, including noise, outliers, and missing values. Robust algorithms can effectively deal with these issues, while less robust algorithms might produce incorrect or misleading results. Preprocessing the data can also enhance algorithm performance. Techniques like normalization, feature scaling, and dimensionality reduction can significantly improve the performance of many algorithms. The preprocessing stage often involves a significant portion of the overall development effort, and careful planning is essential to ensure effectiveness.
Beyond Asymptotic Analysis
While asymptotic analysis provides a valuable framework for understanding algorithm efficiency, it does not capture the full picture. Constant factors and lower-order terms can significantly affect performance in practice. Case study 1: An algorithm with O(n^2) complexity might outperform an algorithm with O(n log n) complexity for small input sizes due to larger constant factors associated with the O(n log n) algorithm. Case study 2: In a real-time system, an algorithm with slightly higher complexity but predictable performance might be preferred over an algorithm with lower asymptotic complexity but highly variable performance. Moreover, implementation details such as caching, compiler optimizations, and hardware architecture greatly influence performance. Testing and profiling are crucial for verifying the algorithm's actual performance in a specific environment. Comparative benchmarks provide valuable insights into the real-world performance of different algorithms. Such empirical evaluations help identify the algorithm that performs best under specific constraints. Understanding these factors, along with asymptotic analysis, is essential for making informed decisions about algorithm selection and optimization.
Conclusion
The reality of algorithm design and analysis goes beyond theoretical complexities. Practical considerations like hardware limitations, data characteristics, and unexpected bottlenecks significantly impact performance. Successful algorithm implementation demands a holistic approach that considers both theoretical analysis and empirical evaluation. A deep understanding of these realities, coupled with rigorous testing and profiling, is crucial for creating efficient and reliable software solutions. The choice of algorithm often involves trade-offs, and selecting the optimal solution requires careful consideration of the specific context and constraints. Focusing on clean, maintainable code, combined with effective data preprocessing and optimization techniques, ensures robust and scalable software systems.