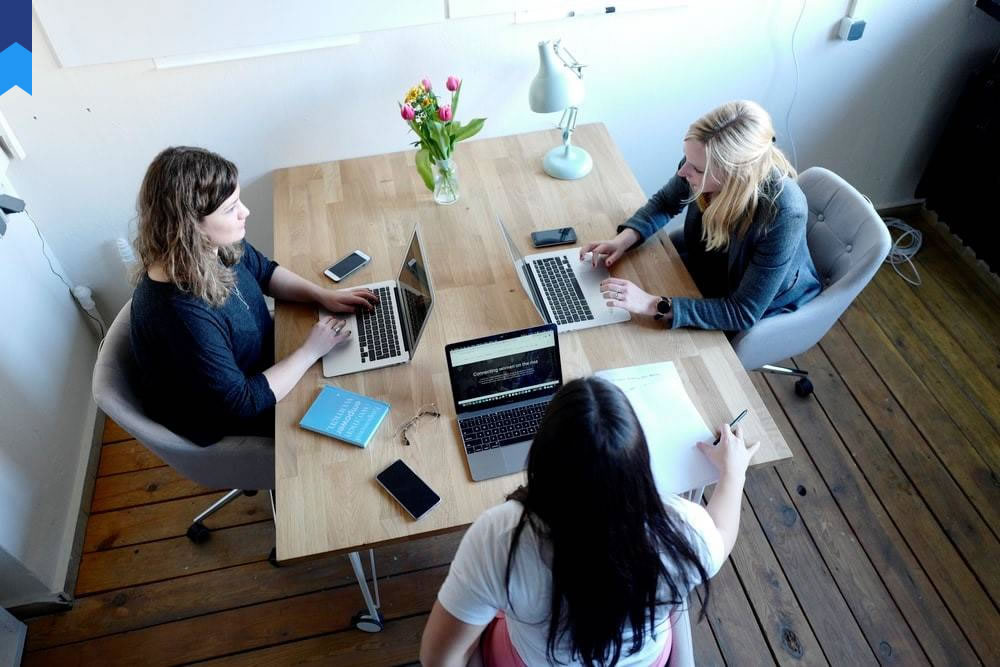
The Reality Behind Kotlin's Hidden Power
Kotlin, the increasingly popular programming language, often receives praise for its conciseness and elegance. But beneath the surface lies a world of powerful, often overlooked features that can significantly impact the efficiency and robustness of your projects. This article delves into the practical aspects of Kotlin, revealing the true scope of its capabilities beyond the beginner tutorials.
Kotlin's Coroutines: Mastering Asynchronous Programming
Asynchronous programming is crucial for modern applications to handle I/O operations without blocking the main thread. Kotlin's coroutines offer an elegant and efficient solution. Unlike traditional threads, coroutines are lightweight and manage concurrency effectively. Consider the case of a network request in an Android app; a coroutine can fetch data in the background without freezing the UI. A well-structured coroutine can significantly enhance the user experience, preventing freezes and lag. The use of `async` and `await` keywords provides an intuitive mechanism to handle asynchronous operations in a sequential manner. Furthermore, structured concurrency, a new feature, significantly simplifies error handling and resource management in coroutines, ensuring easier debugging and preventing leaks.
Case Study 1: A popular e-commerce app used coroutines to handle background tasks such as fetching product images and user data. The result was a noticeably smoother user experience, with reduced loading times and improved responsiveness. Case Study 2: A social media client implemented coroutines for handling real-time updates and notifications, significantly improving the app's responsiveness and efficiency.
The benefits of using coroutines extend beyond responsiveness. Efficient resource management is another critical advantage. Unlike threads, which consume significant system resources, coroutines are lightweight, allowing applications to handle a large number of concurrent operations without incurring heavy overhead. This is especially important in resource-constrained environments like mobile devices. Furthermore, the use of coroutine scopes simplifies the management of coroutine lifecycles, preventing resource leaks and ensuring clean shutdown of asynchronous operations.
Advanced techniques like `Dispatchers` allow you to control where coroutines execute (e.g., on the main thread, a background thread, or a dedicated thread pool), enabling fine-grained control over resource utilization. Efficiently managing multiple coroutines through proper scoping practices is essential to avoid race conditions and other concurrency issues. Proper error handling within coroutines using `try-catch` blocks ensures resilience, providing a better user experience and avoiding crashes. The integration with other Kotlin features like flows enhances the power and flexibility of coroutines, enabling sophisticated asynchronous programming.
Industry experts consistently highlight the importance of asynchronous programming for building responsive and scalable applications. The use of Kotlin coroutines provides a practical and efficient approach. Effective utilization of Kotlin's coroutine features significantly reduces development time and improves the overall quality of the application. Mastering coroutines is not simply a technical skill; it's essential for creating modern, high-performing applications.
Delegated Properties: Simplifying Object Management
Kotlin's delegated properties provide a powerful mechanism to simplify object management and code readability. Instead of explicitly handling property access and modification, developers can delegate these tasks to other objects. For instance, a lazy property can be delegated to a `lazy` delegate, which only initializes the property when it’s first accessed. This is especially useful for expensive computations or operations that should only happen when needed. Observing property changes can be simplified through delegates like `ObservableProperty`, which trigger an action whenever the property value changes.
Case Study 1: A mapping application used delegated properties to efficiently manage map data, loading only the required sections when they are in view. Case Study 2: A game development studio implemented delegated properties to manage game resources, freeing up memory and enhancing performance. This streamlined the management of large datasets and improved the application’s responsiveness.
Delegated properties offer several significant benefits. They enhance code readability by abstracting away the underlying implementation details. They promote maintainability by centralizing property management in a single location, reducing code duplication and simplifying modifications. They are also reusable, as the same delegate can be used across multiple properties. The use of delegates such as `vetoable` properties offers control over property assignment, enabling validation or side effects before modifications are made. This improves data integrity and application robustness.
Advanced usage involves creating custom delegates tailored to specific needs, enabling flexibility and customization. Understanding the different delegate types and their applications is crucial for optimizing object management. Combining delegated properties with other Kotlin features like data classes and extension functions further enhances their effectiveness. This approach results in more concise and expressive code while ensuring high performance. The ability to integrate with various libraries expands the potential applications of this feature.
Experts recommend using delegated properties wherever appropriate to enhance code quality. Effective utilization of delegated properties results in cleaner, more maintainable code. By understanding and applying these features, developers can create robust, scalable, and highly efficient applications. The adoption of delegated properties is a key aspect of modern Kotlin programming best practices.
Functional Programming with Kotlin: Achieving Elegance and Efficiency
Kotlin embraces functional programming paradigms, offering tools like higher-order functions, lambdas, and immutable data structures that promote code clarity, testability, and concurrency. Higher-order functions, which take functions as arguments or return them, enable elegant and concise expressions of complex logic. Lambdas, anonymous functions, allow developers to define functions inline, improving code readability and reducing boilerplate. Immutability helps prevent unintended side effects and improves concurrent code safety.
Case Study 1: A data analysis company utilized Kotlin's functional features to streamline data processing pipelines, resulting in improved efficiency and reduced errors. Case Study 2: A financial modeling firm employed functional programming to build robust and reliable algorithms, enhancing accuracy and reducing risks.
The benefits of functional programming in Kotlin are substantial. Increased code readability is a significant advantage; functional code is often more concise and easier to understand than its imperative counterpart. Enhanced testability stems from the use of pure functions, which lack side effects and make unit testing straightforward. Concurrency safety is promoted because immutable data structures and pure functions eliminate race conditions and other concurrency issues, simplifying parallel computations. The efficient use of resources results from leveraging immutable data structures and reducing the overhead associated with mutable state.
Advanced functional programming techniques, such as monads and functors, enhance the expressiveness and power of Kotlin's functional capabilities. Mastering these techniques requires a deeper understanding of functional principles but yields significant benefits. The integration of functional programming with other Kotlin features creates a synergistic effect, resulting in highly efficient and robust code. Careful consideration of function purity and immutability is essential for fully realizing the advantages of functional programming.
Industry best practices emphasize the importance of functional programming in modern software development. Experts advocate for the use of functional programming techniques to build more maintainable and efficient applications. The adoption of functional approaches is not merely a style choice; it is a crucial step towards building high-quality software. By incorporating functional principles, developers can significantly improve code clarity, testability, and performance.
Extension Functions: Extending Class Functionality Without Inheritance
Kotlin's extension functions allow adding new functionality to existing classes without modifying their original source code or using inheritance. This is incredibly useful for extending standard library classes or third-party libraries without altering their definitions. For instance, you can add a new function to the `String` class to easily reverse a string, or to the `List` class to perform a custom sort. This capability significantly enhances code reusability and reduces boilerplate.
Case Study 1: A development team used extension functions to add custom validation rules to form input fields, simplifying data entry and improving the overall user experience. Case Study 2: A game developer utilized extension functions to enhance the functionality of existing game objects, reducing development time and improving efficiency.
The advantages of extension functions are numerous. Improved code organization is a major benefit, as extension functions can be grouped logically within the same file, reducing clutter and improving readability. Code reusability is enhanced, as extension functions can be reused across multiple projects and contexts. Reduced code duplication minimizes the risk of errors and simplifies maintenance. The ability to extend existing libraries without modification provides flexibility and avoids compatibility issues.
Advanced techniques involve using extension functions with generics for increased flexibility and reusability. The careful naming of extension functions is important to avoid conflicts and maintain clarity. Combining extension functions with other Kotlin features like higher-order functions and lambdas can significantly enhance their usefulness. Effective use of extension functions reduces boilerplate and enhances productivity.
Many industry experts recommend using extension functions to enhance code organization and maintainability. Effective utilization of extension functions leads to cleaner, more efficient, and more maintainable code. By understanding and applying this powerful feature, developers can significantly improve their productivity and the quality of their projects. The adoption of extension functions is a significant aspect of modern Kotlin development best practices.
Data Classes and Data Structures: Efficient Data Management
Kotlin's data classes simplify the creation of classes that primarily hold data. They automatically generate boilerplate code, such as `equals()`, `hashCode()`, `toString()`, and `copy()`, reducing development time and improving code readability. Effective use of data classes enhances the overall efficiency of data management within applications. Kotlin also provides a comprehensive suite of data structures, including lists, sets, and maps, enabling efficient organization and manipulation of data.
Case Study 1: A social media platform used data classes to represent user profiles, streamlining data access and manipulation. Case Study 2: An e-commerce website used data classes and lists to represent product catalogs and shopping carts, making data management more efficient.
The benefits of data classes and effective data structures are clear. Improved developer productivity is a key advantage, as the automatic generation of boilerplate code saves significant development time and effort. Enhanced code readability results from the concise syntax of data classes, making code easier to understand and maintain. Increased efficiency in data management stems from the use of optimized data structures and built-in functions for data manipulation. The integration with other Kotlin features further enhances the power and flexibility of data management within applications.
Advanced techniques involving sealed classes, which restrict the types of data that can be held within a variable, enhance data integrity and type safety. The careful selection of data structures, considering factors such as access patterns and data size, is crucial for optimal performance. The utilization of Kotlin's collection functions, which provide efficient methods for searching, filtering, and manipulating data, further improves data handling. Mastering these techniques optimizes performance and enhances code maintainability.
Industry best practices advocate for using data classes and appropriate data structures to optimize data management. Experts emphasize the importance of selecting the right data structure for a given task to enhance performance. By applying these techniques, developers can create more efficient, robust, and maintainable applications. The adoption of these features is essential for modern Kotlin development.
Conclusion
Kotlin's power extends far beyond its concise syntax and elegant design. By mastering the features explored in this article—coroutines, delegated properties, functional programming, extension functions, and data classes—developers can unlock a new level of efficiency and create robust, scalable applications. These tools are not merely syntactic sugar; they represent a fundamental shift in how developers approach problem-solving and code organization, leading to cleaner, more maintainable, and ultimately more successful projects. The key lies in understanding not just the *what* but the *why* and *how* of these features, leading to a deeper appreciation of Kotlin's true potential.
The future of Kotlin is bright, with continued innovation and community support driving its growth. Mastering these advanced aspects of the language is crucial for developers seeking to build high-quality, high-performance applications. Continuous learning and exploration of the ever-evolving ecosystem surrounding Kotlin will remain essential for staying at the forefront of software development.