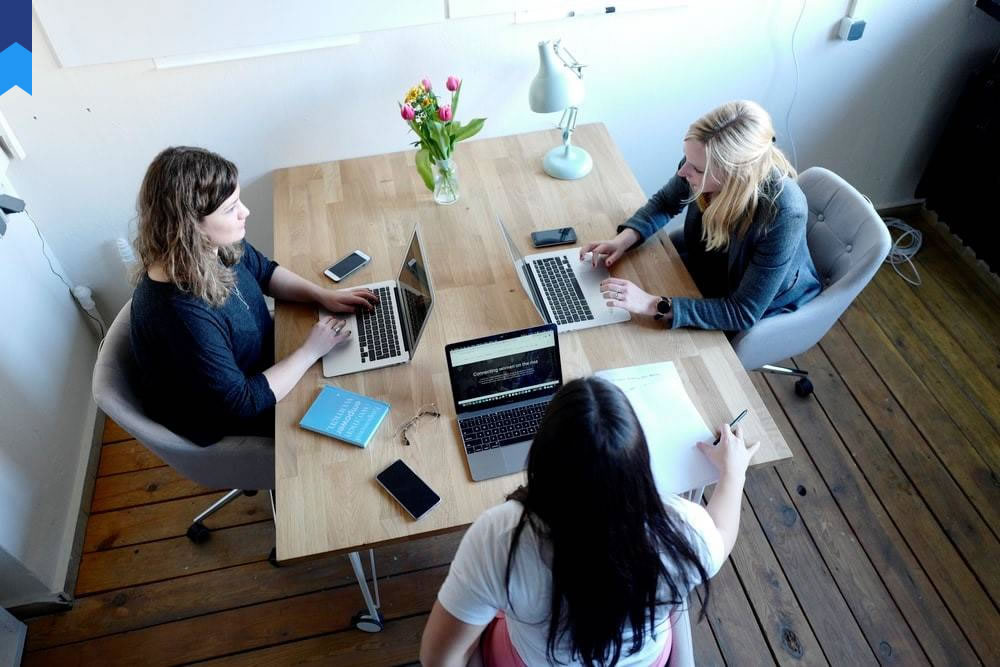
The Reality Behind Programming How-Tos
Introduction: The allure of "easy" programming tutorials often masks the complex realities of software development. This article delves beyond the surface of typical how-to guides, exposing the often-overlooked challenges, unexpected pitfalls, and nuanced techniques that truly define successful coding. We'll explore practical aspects, innovative approaches, and the crucial context missing from simplified instructions, offering a more realistic perspective for aspiring and seasoned programmers alike. This journey will equip you with a deeper understanding, enabling you to navigate the intricacies of coding with greater confidence and efficiency.
Mastering the Fundamentals: Beyond Syntax and Semantics
Effective programming transcends mere memorization of syntax and semantics. It demands a profound comprehension of underlying concepts. Consider data structures: while learning to declare an array seems straightforward, mastering its efficient use in varied scenarios requires a deeper grasp of algorithmic complexities. A simple example: choosing between a linked list and an array depends on whether frequent insertions/deletions or random access are prioritized. Failing to understand this fundamental distinction can lead to inefficient, slow, and ultimately unsuccessful programs.
Case Study 1: A social media platform initially implemented user lists using arrays, resulting in slow search times as user counts grew. Switching to a more efficient data structure dramatically improved performance. Case Study 2: A game development team struggled with AI pathfinding until they fully understood graph algorithms and implemented A* search, significantly enhancing game responsiveness.
Furthermore, understanding algorithmic efficiency is paramount. The difference between O(n) and O(n²) algorithms can mean the difference between a program that runs smoothly and one that crawls to a halt. This is where practice and experimentation truly come into play. It isn't enough to simply understand the concept; you need to apply it. The more you experiment with different approaches and analyze their performance, the better you’ll become at choosing the most efficient solution for a given task.
Beyond the theoretical, practical experience is vital. Many tutorials focus solely on idealized code, neglecting to account for real-world scenarios, such as error handling, data validation, and security considerations. For instance, a naive approach to user input can leave a program vulnerable to injection attacks. Robust error handling and proper input sanitization are crucial but often overlooked in basic tutorials. Learning these nuances through hands-on experience is essential for creating secure and reliable software.
Debugging: The Art of Problem-Solving
Debugging is an integral part of programming. Beginners often underestimate the time and effort it demands. Effective debugging requires systematic thinking, logical deduction, and a good understanding of program flow. It's not merely about finding errors; it's about understanding why they occurred and preventing similar mistakes in the future. Using a debugger effectively, understanding stack traces, and learning to interpret log files are invaluable skills often glossed over in basic tutorials. Mastering these skills differentiates a competent coder from an expert.
Case Study 1: A team of developers encountered a segmentation fault in their C++ application. By using a debugger and examining the memory contents at the point of failure, they identified a memory leak that was causing the issue. Case Study 2: A JavaScript developer found inconsistent behavior in their application. By carefully examining the console logs, they noticed timing issues that were leading to unexpected results, fixing the issue by applying asynchronous programming techniques.
Beyond traditional debugging tools, innovative techniques, such as logging and code instrumentation, offer powerful approaches for detecting and analyzing subtle problems. These methods enable programmers to monitor program behavior dynamically, providing insights that would be difficult to obtain through traditional debugging alone. For instance, implementing custom logging can highlight crucial events and conditions, making it easier to identify the root cause of complex issues.
In practice, debugging often involves a combination of approaches. Trial and error, systematic investigation, and leveraging the power of debugging tools and logging all play a crucial role in effectively resolving bugs. This iterative process strengthens problem-solving skills and cultivates a deeper understanding of the code’s behavior.
Version Control and Collaboration: The Team Dynamics of Coding
Version control systems (VCS) like Git are indispensable for modern software development, enabling collaborative coding and efficient management of code changes. Tutorials often present a simplistic view, focusing on basic commands. However, the true power of VCS lies in advanced techniques like branching, merging, and resolving conflicts. These skills are critical for team collaboration, where multiple developers work concurrently on the same codebase. Mastering branching strategies, such as feature branches and Gitflow, is essential for a smooth workflow.
Case Study 1: A large software project utilized a simple linear workflow, leading to frequent merge conflicts and code integration issues. Switching to a more robust branching strategy dramatically improved team efficiency. Case Study 2: A small team of developers initially struggled with managing multiple versions of their project. After adopting Git and learning effective branching strategies, they were able to streamline their collaboration and improve their workflow significantly.
Collaboration tools and communication strategies are equally important. Effective communication among team members is crucial to avoid conflicts and ensure everyone is on the same page. Using a collaborative code review process allows for peer feedback and knowledge sharing, leading to better code quality and preventing potential problems early on. Furthermore, using issue trackers to manage bugs and feature requests facilitates efficient organization and enables tracking of progress across the project.
Beyond individual skills, successful collaboration requires a cohesive team environment. Building a culture of mutual respect, open communication, and shared responsibility is crucial to maximize team effectiveness and product quality. This involves establishing clear communication channels, adopting a collaborative code review process, and fostering a positive and supportive work environment.
Testing and Quality Assurance: Ensuring Robustness and Reliability
Writing robust and reliable software requires a rigorous testing strategy. Many basic tutorials neglect the importance of comprehensive testing, leading to software with hidden flaws. Effective testing involves unit tests, integration tests, system tests, and user acceptance testing (UAT). Each level serves a distinct purpose, targeting different aspects of the software. Unit testing focuses on individual components, integration testing verifies interactions between components, and system testing evaluates the entire system as a whole. UAT involves end-users verifying the system meets its intended requirements.
Case Study 1: A company released a new software application without sufficient testing. Consequently, they faced a wave of user complaints and had to issue multiple patches to fix critical bugs. Case Study 2: A software development team implemented a rigorous testing strategy, resulting in a more robust and reliable product with significantly fewer bugs after release.
Choosing the right testing methodologies and frameworks is paramount. Understanding the trade-offs between different testing frameworks and adapting the strategy based on the project's needs is vital. Test-driven development (TDD) is a popular approach where tests are written before the code itself, guiding the development process and ensuring testability from the outset. This results in more modular, well-documented code that is easier to test and maintain.
Furthermore, automating testing processes significantly enhances efficiency and scalability. Automated tests run quickly and reliably, allowing for continuous integration and continuous deployment (CI/CD) pipelines. This enables faster feedback loops, allowing teams to identify and address issues early in the development cycle. A well-defined testing strategy is essential for developing high-quality, reliable software, and it is an area often underestimated in basic tutorials.
Deployment and Maintenance: Beyond the "Hello World" Stage
Deploying and maintaining software is a significant aspect of the development lifecycle, often overlooked in introductory tutorials. Deployment involves transferring the finished software to the target environment, which could be a server, cloud platform, or mobile device. This requires understanding different deployment strategies, such as rolling deployments, blue-green deployments, and canary deployments. Each strategy offers different trade-offs in terms of risk and downtime. Choosing the appropriate strategy depends on the specific application and its requirements.
Case Study 1: A web application experienced significant downtime during a deployment due to insufficient planning and testing. Employing a more robust deployment strategy would have mitigated the risk. Case Study 2: A mobile application developer implemented continuous integration and continuous delivery (CI/CD) which automated the build, test, and deployment processes, enabling faster release cycles and improved efficiency.
Maintaining software involves monitoring its performance, addressing bugs, and implementing new features or updates. This requires ongoing effort and a structured approach. Monitoring tools and techniques are essential for identifying performance bottlenecks and potential problems early. Proactive maintenance prevents issues from escalating and minimizes disruptions. Effective monitoring also includes logging, error tracking, and performance analytics.
Furthermore, planning for scalability and future growth is crucial. Software should be designed with scalability in mind from the outset, anticipating future growth and changes. This might involve choosing appropriate technologies and architectures that can handle increased load and user traffic. A well-planned and maintained software system is the hallmark of a successful project, and this stage is often underrepresented in typical tutorials.
Conclusion: The reality of computer programming extends far beyond the simplified examples found in basic tutorials. This article has explored some of the often-overlooked complexities, showcasing the importance of a deeper understanding of fundamental concepts, debugging strategies, collaborative practices, testing methodologies, and deployment considerations. Mastering these aspects is not just about writing functional code; it's about creating robust, reliable, maintainable, and scalable software solutions. By embracing a holistic approach and incorporating practical experience, programmers can bridge the gap between theoretical knowledge and real-world software development, leading to greater success and a more rewarding career.