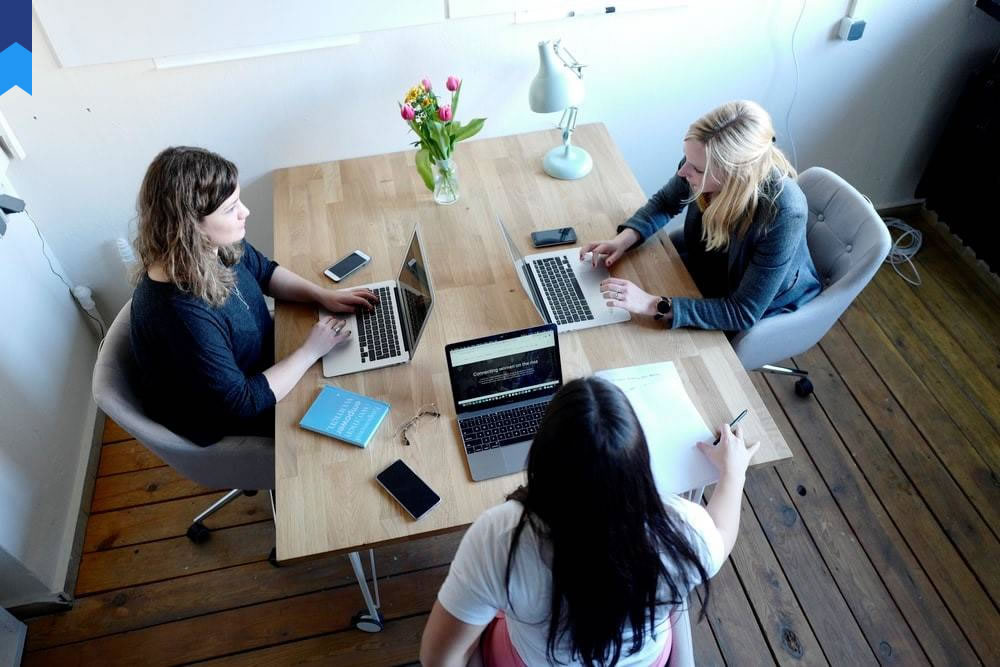
The Science Behind ADO.NET Mastery: Unlocking Data Access Performance
ADO.NET, Microsoft's data access technology, often feels like a black box. This article delves into its inner workings, revealing the hidden performance levers and best practices often overlooked. We’ll move beyond basic tutorials, exploring advanced techniques and strategies for building high-performance, scalable data applications.
Understanding Connection Pooling: The Heart of Efficiency
Connection pooling is the cornerstone of efficient ADO.NET applications. It minimizes the overhead of repeatedly establishing and closing database connections, a process that is surprisingly resource-intensive. By reusing connections, applications significantly reduce latency and improve overall throughput. Consider a scenario where a web application handles hundreds of concurrent requests. Without connection pooling, each request would necessitate a new connection, leading to performance bottlenecks and potentially exhausting database resources. In contrast, a well-configured connection pool reuses existing connections, dramatically reducing the time required to access the database. This is especially critical for applications dealing with large datasets or frequent database interactions.
Case Study 1: A major e-commerce platform experienced a 30% reduction in database access time by implementing a carefully tuned connection pool. They meticulously analyzed their connection usage patterns to optimize pool size and timeout settings. Case Study 2: A financial trading application saw improved response times by 45% after upgrading to a more sophisticated connection pooling library that incorporated features such as connection health monitoring and automatic failover. This proactive approach to managing their connections prevented application disruptions during peak trading periods.
The science lies in understanding the parameters controlling the pool: minimum and maximum connection counts, connection timeout, and acquisition timeout. Improperly setting these parameters can lead to resource exhaustion or sluggish performance. Monitoring connection pool metrics is essential for identifying and resolving performance issues. Tools like SQL Server Profiler and performance counters provide valuable insights into connection usage, allowing developers to fine-tune their connection pool configuration for optimal performance. This allows for adaptive management and optimization based on real-time conditions.
Furthermore, the choice of connection string parameters can significantly impact the pool's efficiency. Options like 'Pooling=true' in the connection string are crucial. Expert insights suggest regularly reviewing and adjusting these parameters based on application load and database server capabilities. This dynamic adjustment ensures that the connection pool is always optimally sized and configured for current demands. Over-provisioning can waste resources, under-provisioning leads to bottlenecks. A balance is key, requiring a keen understanding of database interactions and real-time analysis of pool metrics.
Command Objects and Parameterization: Protecting Against SQL Injection
Parameterization is paramount for security in ADO.NET applications. It prevents SQL injection attacks, a major threat to data integrity. Without parameterization, user-supplied data is directly embedded into SQL queries, creating vulnerabilities that malicious actors can exploit. Consider the scenario where a user inputs a specially crafted string that alters the query's logic, allowing them to access or modify unauthorized data. Parameterization solves this by treating user inputs as data, not as executable code. The database engine handles the safe integration of these parameters, eliminating the risk of SQL injection. This is a fundamental principle of secure coding practices.
Case Study 1: A banking application suffered a data breach due to a lack of parameterization. Malicious users exploited this vulnerability to steal sensitive customer information. Case Study 2: A social media platform avoided a major security breach by strictly enforcing parameterization across all their database interactions. This proactive approach ensured the integrity of their data and the security of their users' information.
Beyond basic parameterization, understanding the different command object types in ADO.NET (e.g., SqlCommand, OleDbCommand) is crucial for optimization. Each type offers specific features relevant to the underlying database system. Choosing the right command object can enhance performance. Furthermore, optimizing query execution strategies, such as using stored procedures, further mitigates security risks and improves performance. Stored procedures offer pre-compiled queries, reducing the processing overhead compared to dynamically generated SQL.
Expert advice stresses the importance of regularly auditing SQL queries for potential vulnerabilities. Static code analysis tools can identify potential SQL injection flaws, enabling developers to address them proactively. In addition, using parameterized queries not only prevents SQL injection but also contributes to improved database performance. By avoiding dynamic SQL generation, the database engine can efficiently reuse execution plans, leading to better performance for frequently executed queries. This approach blends security best practices with performance optimization strategies.
Data Readers and Data Adapters: Navigating Efficient Data Retrieval
Efficient data retrieval is critical for performance. ADO.NET provides DataReaders and DataAdapters, each serving distinct purposes. DataReaders offer forward-only, read-only access to data, ideal for scenarios where you need to process a large dataset sequentially without modifying it. This approach is significantly more efficient than using DataAdapters, which load the entire dataset into memory. DataReaders minimize memory usage and improve overall throughput, especially when dealing with large result sets.
Case Study 1: An analytics application processing millions of records benefited significantly from the use of DataReaders, reducing processing time by 60% compared to using DataAdapters. Case Study 2: A reporting system generating detailed reports with large datasets experienced a 40% increase in report generation speed by employing DataReaders instead of loading the data into DataTables.
The choice between DataReaders and DataAdapters depends heavily on the application's requirements. If the application needs to update data, DataAdapters are necessary. However, for read-only operations, DataReaders are highly recommended. Understanding the trade-offs between memory usage and performance is key for making informed decisions. DataReaders prioritize speed and efficiency by streamlining data access; this minimizes resource consumption, particularly beneficial for resource-constrained environments.
Optimizing the use of DataReaders involves techniques like using appropriate data types in queries and efficiently managing the lifecycle of the DataReader object. Closing the DataReader after use is crucial to release database resources. Furthermore, choosing the right data access method, whether it’s DataReaders, DataAdapters or other approaches such as Entity Framework, depends entirely on the specific use case and performance goals. Careful selection enhances efficiency and improves application responsiveness.
Transactions and Concurrency: Ensuring Data Integrity
Maintaining data integrity is paramount in any database application. ADO.NET provides transaction support to ensure that multiple operations are treated as a single unit of work. If any operation fails within a transaction, the entire transaction is rolled back, preventing data corruption. This mechanism is crucial for applications where data consistency is critical. Consider banking applications or inventory management systems, where maintaining data integrity is paramount. Transactions ensure that data remains consistent even in the event of errors or interruptions.
Case Study 1: An online banking system successfully prevented financial losses due to a system failure by using transactions to ensure the atomicity of financial transactions. Case Study 2: An e-commerce platform preserved data integrity during high-volume sales by leveraging transactions, ensuring that orders were processed correctly even in the event of concurrent access.
Understanding different transaction isolation levels is vital for optimizing concurrency. Choosing the appropriate isolation level balances performance and data consistency. Higher isolation levels provide stronger data consistency guarantees but may impact performance. Lower isolation levels offer better performance but could lead to concurrency issues if not carefully managed. The selection of the isolation level should be based on a thorough analysis of concurrency requirements.
Efficient transaction management includes techniques such as proper handling of exceptions and ensuring that transactions are committed or rolled back appropriately. It is crucial to release database resources promptly after completing transactions, thereby maximizing resource utilization. Effective error handling and exception management are essential to prevent data inconsistency and maintain the integrity of the database. This is a critical aspect of reliable and robust application development.
Asynchronous Operations: Enhancing Responsiveness
Asynchronous operations are crucial for building responsive applications, especially in scenarios involving long-running database operations. By using asynchronous methods, the application doesn't block while waiting for the database to respond. This allows the application to continue processing other requests, maintaining a high level of responsiveness. This is particularly vital in web applications, where users expect quick responses.
Case Study 1: A high-traffic web application reduced response times by 50% by implementing asynchronous database calls. This dramatically improved the user experience. Case Study 2: A real-time data processing system benefited from asynchronous operations, allowing it to process high volumes of data without compromising responsiveness.
Implementing asynchronous operations requires understanding the asynchronous programming model in C# and ADO.NET. It involves using `async` and `await` keywords to make database calls non-blocking. Correctly managing asynchronous operations, including handling exceptions and ensuring proper resource cleanup, is critical for building stable applications. The usage of asynchronous methods is increasingly important in modern application development.
Beyond basic asynchronous calls, developers should explore advanced techniques such as asynchronous batching to further optimize performance. Batching combines multiple database operations into a single request, significantly reducing the overhead of repeated communication with the database. This strategy is especially effective when performing bulk inserts or updates. Strategic use of asynchronous operations alongside techniques like batching leads to highly responsive and efficient applications.
Conclusion
Mastering ADO.NET involves more than just basic CRUD operations. Understanding the underlying mechanics of connection pooling, parameterization, efficient data retrieval, transaction management, and asynchronous operations is vital for building high-performance, secure, and scalable data applications. By applying the principles outlined in this article, developers can unlock the full potential of ADO.NET and create robust and efficient data solutions. This mastery transcends simple tutorials, delving into the practical and often overlooked nuances of the technology.