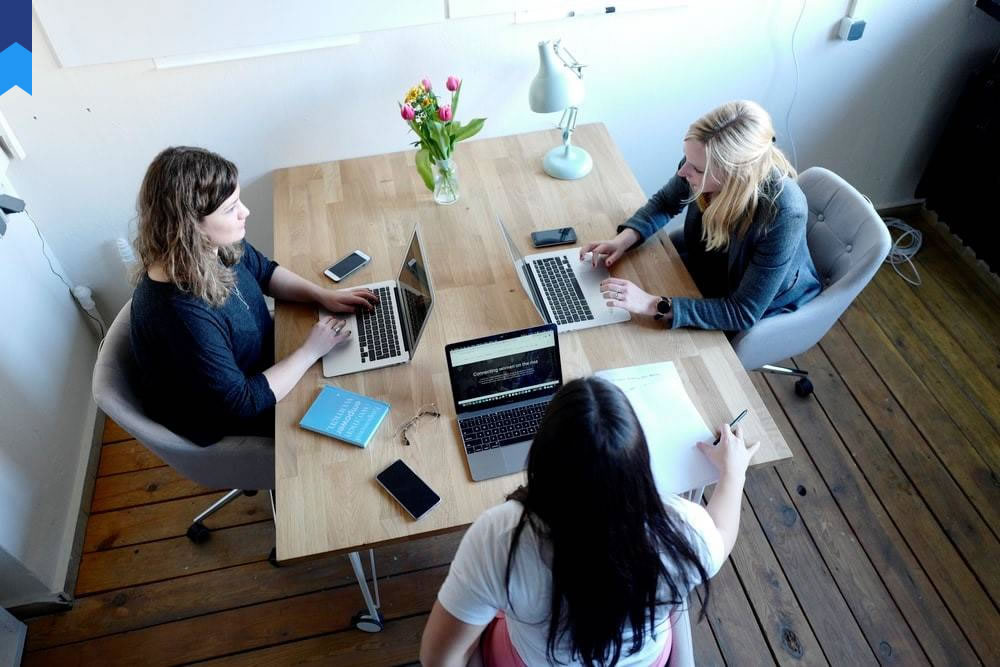
The Science Behind ASP.NET Mastery: Unlocking Advanced Techniques
ASP.NET, a robust framework for building web applications, often presents challenges beyond the basics. This article delves into the scientific principles underlying advanced ASP.NET programming, uncovering hidden potential and challenging conventional wisdom.
Mastering Asynchronous Programming: The Key to Scalability
Asynchronous programming is no longer a luxury; it's a necessity for building scalable and responsive ASP.NET applications. Understanding the intricacies of asynchronous operations, leveraging async and await keywords effectively, and managing task parallelism are crucial. Consider a scenario with a high-traffic e-commerce website: asynchronous processing of orders, inventory checks, and payment gateways prevents blocking the main thread, ensuring smooth operation even under peak load. A poorly designed synchronous system would experience significant performance degradation, leading to frustrated users and lost revenue. Case study: Netflix uses asynchronous programming extensively in its backend systems to handle millions of concurrent users. Another example: a large online gaming platform relies on asynchronous tasks to manage real-time interactions and prevent latency issues. Effective use of asynchronous patterns reduces response times, increases concurrency, and improves overall application efficiency. Experts advocate a shift towards asynchronous design as a fundamental best practice for modern web application architecture. The difference between well-structured async code and poorly implemented sync code can mean the difference between a smooth user experience and a complete system failure under load. Properly managing asynchronous operations requires careful consideration of thread pools, exception handling, and deadlock prevention. Failure to properly manage these aspects can lead to unexpected performance bottlenecks or application crashes. Advanced concepts such as parallel LINQ (PLINQ) and Task Parallel Library (TPL) provide further tools for harnessing the power of multi-core processors. Efficient asynchronous programming unlocks the full potential of modern hardware, improving response times and scaling capabilities dramatically. Ignoring this aspect leads to applications struggling under even moderate load. Properly using asynchronous programming in ASP.NET allows developers to build more efficient, scalable applications that gracefully handle increased traffic demands. This capability is fundamental to the success of modern high-traffic web applications. Consider the complexities involved in handling long-running tasks, such as file uploads or database operations. These tasks should not block the main thread, and this is achieved through asynchronous programming. Ignoring this best practice results in unresponsive applications and frustrated users.
Dependency Injection: Decoupling for Enhanced Testability and Maintainability
Dependency Injection (DI) is a design pattern that promotes loose coupling, enhancing testability and maintainability. Instead of creating objects directly within a class, dependencies are injected from the outside. This allows for easier unit testing, as mock objects can be substituted for dependencies. A practical example involves a class that interacts with a database. Instead of creating a database connection object within the class, the connection is injected as a dependency. This allows for testing the class without an actual database connection, using mock objects that simulate the database behavior. Case Study 1: A large-scale enterprise application uses DI to modularize its components, simplifying testing and maintenance. Case Study 2: A microservices architecture relies heavily on DI for efficient communication between services. The benefits extend beyond testing. DI improves code reusability and maintainability by reducing the tight coupling between components. Changes in one component have less impact on other parts of the application. Choosing the right DI container, such as Autofac or Unity, is crucial for effective implementation. These containers manage object lifecycles and resolve dependencies automatically. Understanding the principles of DI allows developers to create robust, flexible, and easily testable applications, contributing significantly to long-term maintainability. Misunderstanding or neglecting DI can lead to monolithic, untestable, and difficult-to-maintain code. The implications of poor DI practices manifest as complex debugging sessions, increased development time, and higher risk of introducing bugs. Proper implementation requires a deep understanding of interfaces, abstract classes, and the inversion of control principle. The resulting architecture is more modular and allows for easier integration with new components and technologies. DI also facilitates easier integration of external services and libraries. It helps improve the overall maintainability and scalability of the application, even as its complexity increases.
Caching Strategies for Optimal Performance: Beyond Simple In-Memory Solutions
Caching is fundamental to high-performance ASP.NET applications. While in-memory caching is a common starting point, understanding advanced caching strategies like distributed caching, output caching, and content delivery networks (CDNs) is vital. A poorly designed caching strategy can lead to stale data and increased database load. Case Study 1: An e-commerce platform leverages Redis as a distributed cache to handle high read loads and reduce database pressure. Case Study 2: A news website uses output caching to store frequently accessed pages, minimizing database queries. Beyond simple in-memory solutions, exploring distributed caching technologies like Redis or Memcached enhances scalability and performance. These technologies provide a shared cache across multiple servers, enabling efficient data access in distributed environments. Output caching, which stores the rendered output of a page, further optimizes performance by bypassing the rendering process for subsequent requests. Content Delivery Networks (CDNs) extend caching to a global scale, delivering content from geographically closer servers to users, significantly reducing latency. The choice of caching strategy depends on several factors, including the type of data, access patterns, and scalability requirements. Effective caching significantly improves application responsiveness and reduces database load, enhancing user experience and reducing infrastructure costs. Ignoring proper caching strategies results in slow response times, increased server load, and a less responsive application. Understanding the intricacies of cache invalidation, cache eviction policies, and cache dependency management is crucial to preventing data inconsistencies. Properly implementing caching can reduce the load on databases by orders of magnitude, leading to improved scalability and decreased infrastructure costs. Additionally, it leads to faster page load times, a crucial factor in user satisfaction and search engine ranking.
Security Best Practices: Defending Against Modern Threats
Security is paramount in any web application. Beyond basic authentication and authorization, understanding modern security threats, such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF), is essential. Case Study 1: A financial institution uses multi-factor authentication and robust input validation to protect user accounts. Case Study 2: A social media platform employs a comprehensive security strategy, including regular security audits and penetration testing. ASP.NET provides built-in security features, but developers must diligently implement best practices to prevent vulnerabilities. Input validation is crucial to prevent SQL injection and XSS attacks. Parameterized queries help to protect against SQL injection by treating user input as data rather than code. Properly configured authentication and authorization mechanisms are vital for controlling access to application resources. Regular security audits and penetration testing identify and address vulnerabilities before they are exploited. Understanding OWASP (Open Web Application Security Project) top 10 vulnerabilities provides a framework for addressing common security threats. Employing secure coding practices, using HTTPS, and regularly patching vulnerabilities are essential for maintaining a secure application. Neglecting security can lead to data breaches, financial losses, and reputational damage. A robust security strategy involves a multi-layered approach, combining preventative measures, detection mechanisms, and response plans. This includes using strong passwords, implementing rate limiting to prevent brute force attacks, and monitoring application logs for suspicious activity. Developers should regularly update their knowledge of the latest security threats and best practices to adapt to the ever-evolving landscape.
Optimizing Performance: Beyond Basic Profiling
Performance optimization in ASP.NET goes beyond basic profiling. Understanding advanced techniques like code analysis, database optimization, and efficient resource utilization is crucial for building high-performance applications. Case Study 1: An e-commerce site optimizes its database queries to improve page load times significantly. Case Study 2: A social media platform uses load balancing and caching strategies to distribute traffic and improve performance under high load. Effective performance optimization starts with identifying bottlenecks using profiling tools. Understanding the execution path, memory usage, and database query performance is critical. Database optimization, including indexing, query tuning, and database schema design, can drastically improve query performance. Efficient resource utilization involves minimizing memory allocation, optimizing network calls, and leveraging asynchronous programming to improve concurrency. Code analysis tools can identify areas for improvement, such as inefficient algorithms or excessive memory allocation. Regular performance testing under various load conditions is essential to identify potential bottlenecks and ensure the application's responsiveness under different circumstances. Addressing performance bottlenecks proactively ensures optimal application performance, improving user experience and minimizing infrastructure costs. Ignoring performance considerations can lead to slow response times, increased server load, and reduced scalability. The application may struggle to handle increased traffic, leading to poor user experiences and business disruptions. A holistic approach to performance optimization involves continuous monitoring and fine-tuning to maintain optimal performance.
Conclusion
Mastering ASP.NET involves understanding the underlying principles and advanced techniques beyond basic tutorials. This article has explored key areas, emphasizing the scientific approach to building robust, scalable, and secure applications. By understanding asynchronous programming, dependency injection, caching strategies, security best practices, and performance optimization, developers can unlock the full potential of the ASP.NET framework. Continuous learning and adaptation are vital in the ever-evolving world of software development. Embracing these advanced concepts transforms ASP.NET development from simply writing code into a process of building high-quality, efficient, and secure software systems. The future of ASP.NET development lies in the mastery of these techniques and the ongoing adaptation to new technologies and best practices.