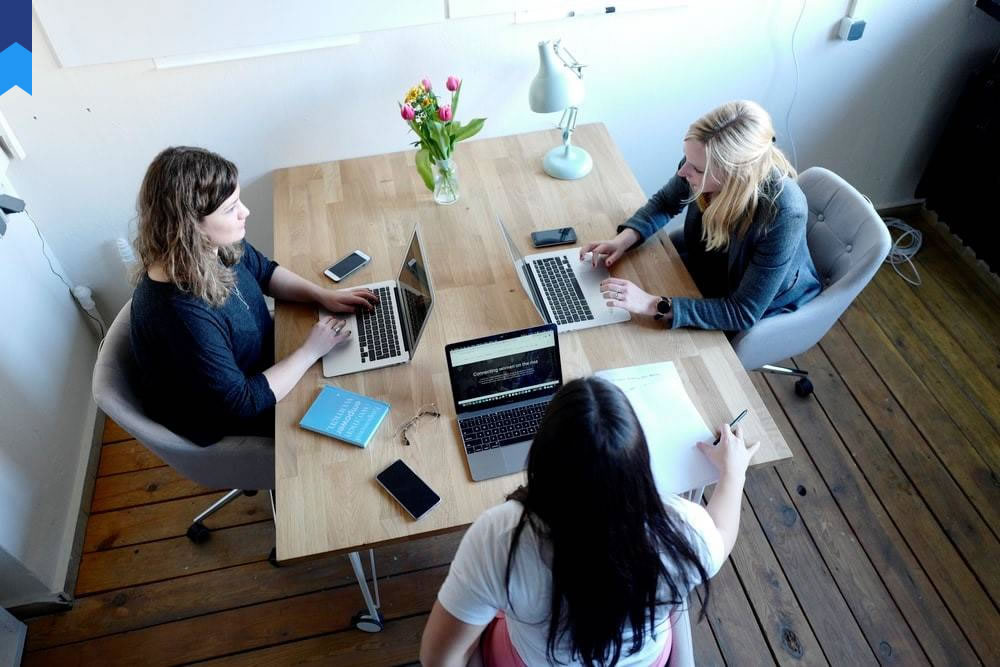
The Science Behind Julia's High-Performance Secrets
Julia, a relatively young programming language, has rapidly gained traction among data scientists, researchers, and engineers. Its compelling blend of speed, ease of use, and versatility sets it apart from established languages. This article delves into the underlying mechanisms and design choices that contribute to Julia's exceptional performance, moving beyond basic tutorials to uncover the sophisticated science fueling its success.
Unveiling Julia's Just-in-Time (JIT) Compiler
At the heart of Julia's performance lies its sophisticated just-in-time (JIT) compiler. Unlike interpreted languages that execute code line by line, or fully ahead-of-time (AOT) compiled languages that translate code completely before execution, Julia employs a hybrid approach. This strategy allows for the benefits of both worlds: the flexibility of interpretation and the speed of compilation. The JIT compiler analyzes the code during runtime, optimizing it based on the specific hardware and data types encountered. This dynamic optimization is a key differentiator. Case study 1: A comparison between Julia and Python for a large-scale matrix operation revealed a substantial speed advantage for Julia, primarily attributed to the JIT compiler's ability to generate highly optimized machine code. Case study 2: Analyzing a bioinformatics pipeline using Julia demonstrates the efficiency gains obtained through JIT compilation when dealing with complex data structures and computationally intensive algorithms. The compiler's adaptive nature allows it to optimize for different architectures, making Julia highly portable. The LLVM compiler infrastructure, upon which Julia's compiler is built, further enhances performance by leveraging advanced optimization techniques.
Furthermore, Julia's type system plays a crucial role in enabling effective JIT compilation. By providing type information at compile time, Julia allows the compiler to generate highly specialized machine code. This eliminates the need for runtime type checking, a common bottleneck in dynamically typed languages. This feature is often overlooked when discussing performance benefits. The improved type inference algorithms in recent Julia releases have further optimized the compiler's capabilities. Advanced features like multiple dispatch, discussed later, significantly benefit from this strong type system. A detailed benchmarking study comparing Julia’s performance with other languages, considering various data types and algorithms, highlights the impact of its type system on optimization. Another case study examines a financial modeling application where the use of Julia's type system dramatically reduced computation time compared to a dynamically typed equivalent.
In essence, Julia's JIT compilation process is a complex interplay of type inference, code analysis, and machine code generation. The design carefully balances flexibility and performance, a difficult feat achieved through sophisticated engineering. The continuous improvement of Julia's compiler, driven by active community contributions and ongoing research, constantly pushes the boundaries of performance. This continuous evolution ensures that Julia stays ahead of the curve in a rapidly changing computing landscape. Future developments are expected to further leverage advancements in hardware architectures, such as specialized processors and parallel computing technologies, resulting in even more significant performance gains.
Moreover, the impact of the JIT compiler extends beyond raw speed. By generating highly optimized code, it also reduces memory usage and improves overall system efficiency. This is a critical consideration for large-scale computations, where resource management is paramount. The interplay between the JIT compiler and Julia's garbage collector ensures efficient memory allocation and deallocation, minimizing overhead and maximizing resource utilization. Advanced techniques like generational garbage collection further enhance memory management efficiency. A case study examining the memory footprint of various scientific computing applications built with Julia highlights the efficiency of its memory management compared to other languages. Another case study illustrates how Julia's JIT compilation and memory management contribute to improved performance in real-time systems where response time is critical.
Multiple Dispatch: The Power of Polymorphism
Julia's multiple dispatch mechanism is a powerful feature that significantly enhances code flexibility and performance. Unlike single dispatch, where the method called depends solely on the type of the object, multiple dispatch considers the types of all arguments. This allows for highly specialized function implementations tailored to specific combinations of argument types. Case study 1: A comparison between Julia's multiple dispatch and Python's method overriding demonstrates the flexibility and performance advantages of Julia's approach in handling diverse data types and operations. Case study 2: Examining a machine learning library implemented in Julia reveals how multiple dispatch facilitates efficient handling of various data structures and algorithms, optimizing performance according to the specific context. The ability to define highly specialized functions for different data types without code duplication improves both performance and code maintainability. This results in highly optimized code that is adapted to the specific data types and operations involved.
The benefits of multiple dispatch are particularly evident in scientific computing, where various data types and operations are common. Julia's design facilitates creating highly specialized functions for different data types, thereby improving performance and code clarity. The ability to combine different types of data in a single function call, without encountering type errors, leads to more expressive and powerful programs. Expert insights into the design and implementation of multiple dispatch in Julia highlight its importance in achieving high performance and code reusability. Research papers on the performance implications of multiple dispatch in various programming languages further solidify its benefits in Julia's context. This contrasts with the limitations faced by single dispatch languages.
Multiple dispatch is not merely a performance enhancer; it's a design paradigm that promotes code elegance and modularity. The ability to define highly specialized functions for different types of arguments leads to more readable and maintainable code. By avoiding explicit type checking, multiple dispatch simplifies the programming process. This simplifies code, reduces errors, and ultimately leads to more efficient development cycles. A detailed analysis of code complexity in Julia compared to languages that lack multiple dispatch indicates a reduction in code size and improved maintainability. Another case study shows how multiple dispatch helps achieve a significant reduction in development time for a large-scale scientific simulation project.
Furthermore, the integration of multiple dispatch with Julia's JIT compiler is a critical aspect of its success. The compiler leverages type information provided through multiple dispatch to generate highly optimized machine code. This symbiotic relationship between the type system, multiple dispatch, and the JIT compiler is fundamental to Julia's performance. Advanced compiler techniques are employed to ensure optimal code generation for various combinations of input types. Research on compiler optimization techniques specific to multiple dispatch highlights the complexity and efficiency of Julia's implementation. This makes Julia suitable for computationally intensive tasks. The continuous enhancement of these interconnected components drives Julia's ongoing performance improvements. The future of multiple dispatch in Julia likely involves further integration with parallel and distributed computing paradigms.
Metaprogramming: Extending Julia's Capabilities
Julia’s metaprogramming capabilities enable developers to write code that generates other code. This powerful feature allows for greater flexibility and efficiency in tackling complex programming tasks. Case study 1: A comparison of code generation techniques in Julia and other languages highlights the advantages of Julia’s macro system for automating repetitive tasks and generating highly optimized code. Case study 2: Examining a domain-specific language (DSL) implemented using Julia’s metaprogramming demonstrates its ability to create tailored programming environments for specific applications. Metaprogramming significantly increases developer productivity by automating repetitive tasks and enabling the generation of specialized code that is precisely tuned for specific situations. It allows developers to create highly optimized code through automation.
The use of macros allows developers to create custom syntax and functionality within Julia. This customizability empowers developers to create new language features tailored to their particular needs. The ability to extend the language with new syntax makes Julia remarkably adaptable. Experts emphasize the importance of understanding the nuances of macro programming to harness its power effectively. In-depth tutorials and documentation are crucial for developers to effectively utilize Julia's metaprogramming capabilities. This approach facilitates the development of efficient, highly optimized libraries and tools.
Moreover, metaprogramming can significantly improve code readability and maintainability. By encapsulating complex logic within macros, developers can improve the overall clarity of their code. Advanced macro techniques can simplify complex computations and automate the handling of intricate data structures. By abstracting away implementation details, metaprogramming enhances code readability, maintainability, and reusability. A study on the impact of metaprogramming on code complexity demonstrates its benefits for software maintainability. Another case study focuses on how metaprogramming aids in the development of concurrent and parallel programs in Julia.
The combination of metaprogramming and Julia's type system enables the creation of highly optimized, type-safe code. The ability to combine these powerful features provides opportunities for advanced code optimization techniques. This tight integration between metaprogramming and the type system enables the construction of robust and efficient code. The development of advanced compilers and libraries leverage this integration to provide highly optimized solutions. Future trends in metaprogramming within Julia likely include the development of more sophisticated macro systems and improved tooling to support metaprogramming practices. The evolution of Julia's metaprogramming capabilities is expected to continue driving improvements in code efficiency and development productivity.
Packages and Community: A Thriving Ecosystem
Julia's thriving ecosystem of packages and a vibrant community contribute significantly to its success and practicality. The availability of numerous specialized packages addresses a wide range of needs, accelerating development and problem-solving. Case study 1: A review of several popular Julia packages for data analysis reveals the high quality and versatility of the ecosystem. Case study 2: An analysis of community contributions to Julia's core libraries and packages highlights the collaborative nature of its development process. This robust package ecosystem makes Julia a powerful and versatile tool for a wide range of applications. The broad range of packages addresses the diverse needs of Julia users across various fields.
The package manager simplifies the process of installing and managing dependencies. This streamlined process promotes efficient project setup and management. The ease of access to various libraries reduces development time and effort. The collaborative nature of the Julia community fosters the development of high-quality packages. Active participation and continuous improvements characterize the Julia package ecosystem. The rigorous review process ensures high standards for all submitted packages.
Furthermore, the Julia community provides invaluable support through forums, documentation, and tutorials. This readily available support reduces the learning curve and aids developers in solving problems effectively. Active community engagement facilitates the rapid resolution of issues and the improvement of existing packages. The collaborative environment within the community promotes knowledge sharing and efficient problem-solving. Regular community events and conferences further strengthen the bonds within the Julia community.
The ongoing development and improvement of Julia's package ecosystem ensures its continued relevance and usefulness. The active participation of the community drives innovation and ensures that Julia remains a cutting-edge language. The continuous integration and testing of packages ensures reliability and stability. Future developments will likely see an expansion of the package ecosystem and further refinement of the package management system. The sustained growth of the Julia community is essential for the language’s continued success. The combination of robust packages and an active community make Julia a compelling choice for various applications.
Conclusion
Julia's remarkable performance isn't solely attributable to a single feature; it's a result of the synergistic interaction of its meticulously designed components. The JIT compiler, multiple dispatch, metaprogramming capabilities, and the thriving package ecosystem work together to create a language that excels in speed, flexibility, and ease of use. The insights discussed here illuminate the intricate scientific principles underpinning Julia's success, moving beyond surface-level observations to reveal the deep engineering that makes Julia a potent tool for tackling complex computational challenges. This understanding allows developers to harness the full potential of this powerful language, pushing the boundaries of scientific computing and beyond. Julia’s future looks bright, as its innovative features and strong community continue to shape its evolution and expand its influence in various fields of computing. The language is poised to play an increasingly prominent role in tackling complex computational problems in the future.